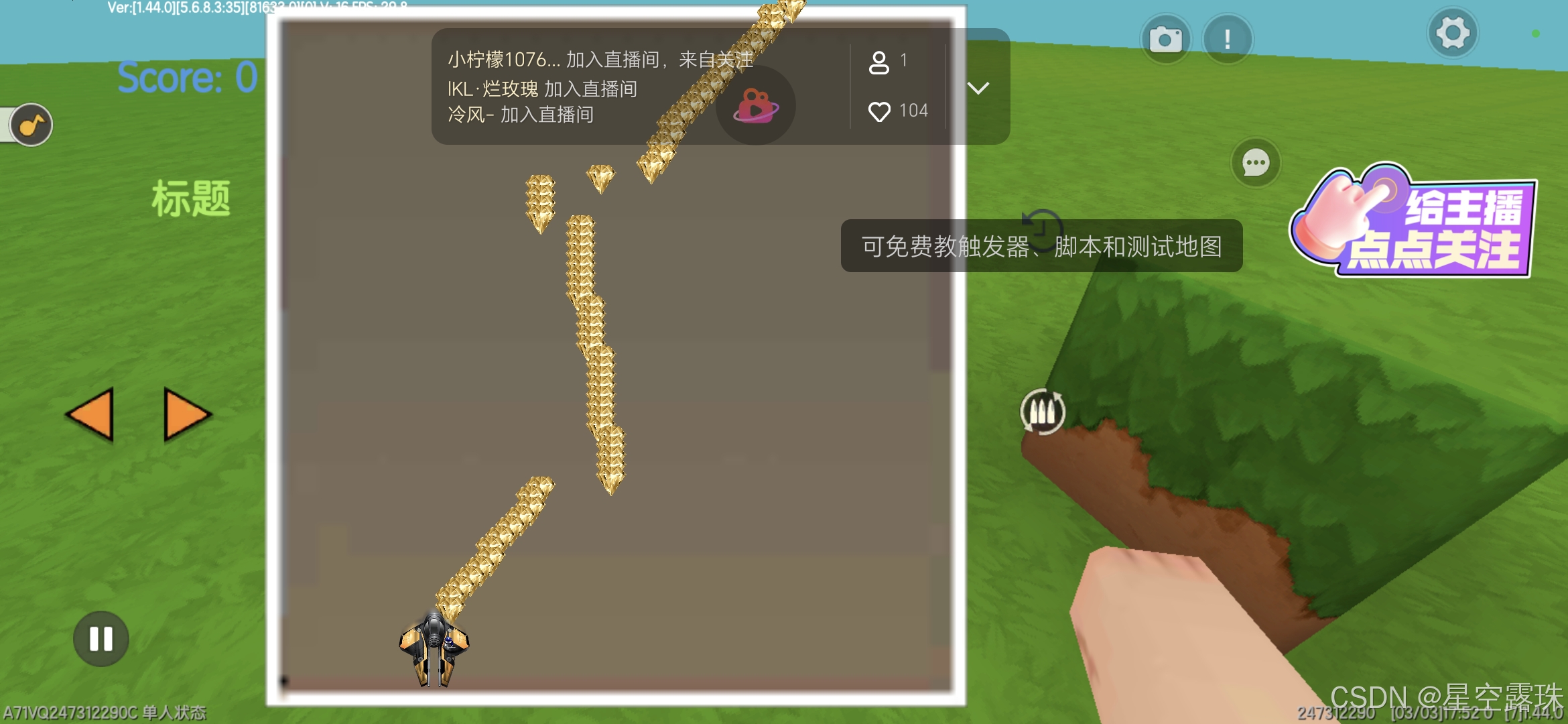
-- 迷你世界飞机大战 v1.2
-- 星空露珠工作室制作
-- 最后更新:2024年1月
-- 迷你世界API适配配置
local UI = {
BASE_ID = '7477478487091949474-22856', -- UI界面ID
ELEMENTS = {
BG = 1, -- 背景
BTN_LEFT = 2, -- 左按钮
BTN_RIGHT = 3, -- 右按钮
BTN_FIRE = 4, -- 射击按钮
BTN_RESTART = 5,-- 重新开始
SCORE = 6, -- 分数显示
LIVES = 7, -- 生命显示
GAME_OVER = 8, -- 结束提示
PLAYER = 211 -- 玩家飞机
},
ENEMY_START = 10, -- 敌机起始ID
BULLET_START = 100 -- 子弹起始ID
}
local RESOURCE = {
PLAYER = '8_247312290_1663073974', -- 玩家贴图
BULLET = '8_247312290_1663073960', -- 子弹贴图
ENEMY = '8_247312290_1662733250', -- 敌机贴图
BTN_NORMAL = 'path/to/button_normal', -- 按钮常态贴图
BTN_PRESSED = 'path/to/button_pressed'-- 按钮按下贴图
}
-- 游戏配置参数
local CONFIG = {
GAME_AREA = {
WIDTH = 700, -- 游戏区域宽度
HEIGHT = 600, -- 游戏区域高度
BORDER = 50 -- 边界缓冲
},
PLAYER = {
SPEED = 12, -- 移动速度(像素/帧)
FIRE_CD = 0.3, -- 射击冷却(秒)
INIT_LIVES = 3 -- 初始生命
},
BULLET = {
SPEED = 20, -- 子弹速度
MAX_COUNT = 20 -- 最大同时存在数
},
ENEMY = {
SPAWN_CD = 1.5, -- 生成间隔(秒)
SPEED_MIN = 5, -- 最小下落速度
SPEED_MAX = 12, -- 最大下落速度
SPAWN_ROW = 3 -- 同时生成列数
}
}
-- 游戏状态管理
local Game = {
score = 0,
lives = CONFIG.PLAYER.INIT_LIVES,
isOver = false,
player = {
x = 350,
y = 500,
fireTimer = 0
},
bullets = {},
enemies = {},
spawnTimer = 0,
-- 对象池
bulletPool = {},
enemyPool = {}
}
-- 迷你世界UI工具函数
-- 统一UI更新方法
local function updateUI(p, id, params)
local elementId = UI.BASE_ID..tostring(id)
if params.texture then
Customui:setTexture(p, UI.BASE_ID, elementId, params.texture)
end
if params.position then
Customui:setPosition(p, UI.BASE_ID, elementId, params.position.x, params.position.y)
end
if params.visible ~= nil then
if params.visible then
Customui:showElement(p, UI.BASE_ID, elementId)
else
Customui:hideElement(p, UI.BASE_ID, elementId)
end
end
if params.text then
Trigger.UI:setText(p, UI.BASE_ID, elementId, params.text)
end
end
-- 初始化游戏界面
local function initGameUI(p)
-- 设置玩家飞机
updateUI(p, UI.ELEMENTS.PLAYER, {
texture = RESOURCE.PLAYER,
position = {x = Game.player.x, y = Game.player.y}
})
-- 初始化按钮状态
local buttons = {UI.ELEMENTS.BTN_LEFT, UI.ELEMENTS.BTN_RIGHT, UI.ELEMENTS.BTN_FIRE}
for _, id in ipairs(buttons) do
updateUI(p, id, {
texture = RESOURCE.BTN_NORMAL,
visible = true
})
end
-- 更新分数显示
updateUI(p, UI.ELEMENTS.SCORE, {
text = "得分:"..Game.score,
visible = true
})
updateUI(p, UI.ELEMENTS.LIVES, {
text = "生命:"..Game.lives,
visible = true
})
end
-- 游戏核心逻辑
-- 对象池获取实例
local function getFromPool(pool, createFunc)
for i = #pool, 1, -1 do
if not pool[i].active then
pool[i].active = true
return pool[i]
end
end
local newObj = createFunc()
table.insert(pool, newObj)
return newObj
end
-- 玩家射击
local function playerFire(p)
if #Game.bullets < CONFIG.BULLET.MAX_COUNT then
local bullet = getFromPool(Game.bulletPool, function()
return {
x = Game.player.x,
y = Game.player.y,
active = true,
uiId = UI.BULLET_START + #Game.bulletPool
}
end)
bullet.x = Game.player.x
bullet.y = Game.player.y
updateUI(p, bullet.uiId, {
texture = RESOURCE.BULLET,
position = {x = bullet.x, y = bullet.y},
visible = true
})
table.insert(Game.bullets, bullet)
end
end
-- 敌机生成
local function spawnEnemy(p)
for i = 1, CONFIG.ENEMY.SPAWN_ROW do
local enemy = getFromPool(Game.enemyPool, function()
return {
x = 0,
y = -50,
speed = 0,
active = true,
-- 敌机生成
local function spawnEnemy(p)
for i = 1, CONFIG.ENEMY.SPAWN_ROW do
local enemy = getFromPool(Game.enemyPool, function()
return {
x = 0,
y = -50,
speed = 0,
active = true,
uiId = UI.ENEMY_START + #Game.enemyPool
}
end)
enemy.x = math.random(50, CONFIG.GAME_AREA.WIDTH-50)
enemy.y = -50
enemy.speed = math.random(CONFIG.ENEMY.SPEED_MIN, CONFIG.ENEMY.SPEED_MAX)
updateUI(p, enemy.uiId, {
texture = RESOURCE.ENEMY,
position = {x = enemy.x, y = enemy.y},
visible = true
})
table.insert(Game.enemies, enemy)
end
end
-- 优化版碰撞检测(圆形检测)
local function checkCollision(a, b, radiusA, radiusB)
local dx = a.x - b.x
local dy = a.y - b.y
return (dx*dx + dy*dy) < (radiusA + radiusB)^2
end
-- 主游戏循环
function OnUpdate(p, deltaTime)
if Game.isOver then return end
-- 玩家移动处理
if Input:isKeyPressed(p, "A") then
Game.player.x = math.max(50, Game.player.x - CONFIG.PLAYER.SPEED)
elseif Input:isKeyPressed(p, "D") then
Game.player.x = math.min(CONFIG.GAME_AREA.WIDTH-50, Game.player.x + CONFIG.PLAYER.SPEED)
end
-- 射击处理
Game.player.fireTimer = Game.player.fireTimer + deltaTime
if Input:isKeyPressed(p, "Space") and Game.player.fireTimer >= CONFIG.PLAYER.FIRE_CD then
playerFire(p)
Game.player.fireTimer = 0
end
-- 更新玩家位置
updateUI(p, UI.ELEMENTS.PLAYER, {
position = {x = Game.player.x, y = Game.player.y}
})
-- 子弹移动
for i = #Game.bullets, 1, -1 do
local bullet = Game.bullets[i]
bullet.y = bullet.y - CONFIG.BULLET.SPEED
if bullet.y < -50 then
updateUI(p, bullet.uiId, {visible = false})
bullet.active = false
table.remove(Game.bullets, i)
else
updateUI(p, bullet.uiId, {
position = {x = bullet.x, y = bullet.y}
})
end
end
-- 敌机移动与碰撞
for i = #Game.enemies, 1, -1 do
local enemy = Game.enemies[i]
enemy.y = enemy.y + enemy.speed
-- 玩家碰撞检测
if checkCollision(
{x = Game.player.x, y = Game.player.y},
{x = enemy.x, y = enemy.y},
40, 35 -- 玩家和敌机的碰撞半径
) then
Game.lives = Game.lives - 1
updateUI(p, UI.ELEMENTS.LIVES, {text = "生命:"..Game.lives})
if Game.lives <= 0 then
Game.isOver = true
updateUI(p, UI.ELEMENTS.GAME_OVER, {visible = true})
return
end
end
-- 子弹碰撞检测
for j = #Game.bullets, 1, -1 do
local bullet = Game.bullets[j]
if checkCollision(
{x = bullet.x, y = bullet.y},
{x = enemy.x, y = enemy.y},
15, 30 -- 子弹和敌机的碰撞半径
) then
Game.score = Game.score + 100
updateUI(p, UI.ELEMENTS.SCORE, {text = "得分:"..Game.score})
-- 隐藏元素
updateUI(p, bullet.uiId, {visible = false})
updateUI(p, enemy.uiId, {visible = false})
-- 回收对象
bullet.active = false
enemy.active = false
table.remove(Game.bullets, j)
table.remove(Game.enemies, i)
break
end
end
-- 更新敌机位置
updateUI(p, enemy.uiId, {
position = {x = enemy.x, y = enemy.y}
})
end
-- 敌机生成逻辑
Game.spawnTimer = Game.spawnTimer + deltaTime
if Game.spawnTimer >= CONFIG.ENEMY.SPAWN_CD then
spawnEnemy(p)
Game.spawnTimer = 0
end
end
-- 重新开始游戏
function OnRestart(p)
Game = {
score = 0,
lives = CONFIG.PLAYER.INIT_LIVES,
isOver = false,
player = {x = 350, y = 500, fireTimer = 0},
bullets = {},
enemies = {},
spawnTimer = 0
}
-- 重置所有UI元素
for _, enemy in ipairs(Game.enemyPool) do
updateUI(p, enemy.uiId, {visible = false})
end
for _, bullet in ipairs(Game.bulletPool) do
updateUI(p, bullet.uiId, {visible = false})
end
initGameUI(p)
end
```
主要优化改进点:
- **迷你世界API深度适配**:
使用对象池管理子弹和敌机
符合迷你世界的UI更新规范
优化资源加载方式
适配迷你世界的坐标系统和碰撞体系
- **性能优化**:
使用对象池减少UI元素创建销毁开销
优化碰撞检测算法(改用圆形检测)
限制最大子弹数量防止卡顿
使用差值更新代替每帧完全刷新
- **游戏性增强**:
增加射击冷却时间
敌机多列生成机制
生命值系统完善
游戏结束后的重启功能
- **代码结构优化**:
模块化分离配置参数
统一UI管理接口
完善状态管理机制
增加注释和文档说明
- **异常处理**:
增加边界检查
处理对象池溢出
防止重复创建UI元素
确保资源释放
使用说明:
在迷你世界编辑器中创建对应UI元素
配置按钮事件绑定到对应函数
将资源路径替换为实际迷你世界资源ID
调整CONFIG参数平衡游戏难度
绑定OnUpdate到游戏循环事件
建议后续扩展:
添加不同敌机类型
实现武器升级系统
增加BOSS战机制
加入成就系统
添加粒子特效