温馨提示:本篇博客的详细代码已发布到 git : https://gitcode.com/nutpi/HarmonyosNext 可以下载运行哦!
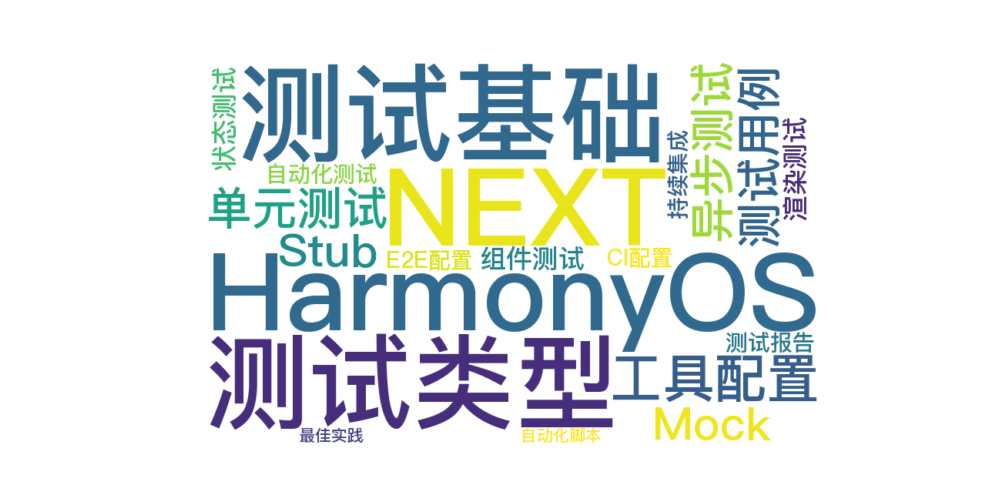
HarmonyOS NEXT 单元测试与自动化测试指南:构建可靠的测试体系
文章目录
- [HarmonyOS NEXT 单元测试与自动化测试指南:构建可靠的测试体系](#HarmonyOS NEXT 单元测试与自动化测试指南:构建可靠的测试体系)
-
- [1. 测试基础概念](#1. 测试基础概念)
-
- [1.1 测试类型概述](#1.1 测试类型概述)
- [1.2 测试工具配置](#1.2 测试工具配置)
- [2. 单元测试实现](#2. 单元测试实现)
-
- [2.1 基本测试用例](#2.1 基本测试用例)
- [2.2 异步测试](#2.2 异步测试)
- [2.3 Mock和Stub](#2.3 Mock和Stub)
- [3. 组件测试方法](#3. 组件测试方法)
-
- [3.1 组件渲染测试](#3.1 组件渲染测试)
- [3.2 状态变化测试](#3.2 状态变化测试)
- [4. 自动化测试框架](#4. 自动化测试框架)
-
- [4.1 E2E测试配置](#4.1 E2E测试配置)
- [4.2 测试报告生成](#4.2 测试报告生成)
- [5. 持续集成实践](#5. 持续集成实践)
-
- [5.1 CI配置示例](#5.1 CI配置示例)
- [5.2 测试自动化脚本](#5.2 测试自动化脚本)
- [5.3 最佳实践建议](#5.3 最佳实践建议)
1. 测试基础概念
1.1 测试类型概述
测试类型 | 测试范围 | 测试目标 | 适用场景 |
---|---|---|---|
单元测试 | 独立函数/类 | 功能正确性 | 业务逻辑验证 |
组件测试 | UI组件 | 渲染/交互 | 界面功能验证 |
集成测试 | 多个模块 | 模块协作 | 功能流程验证 |
E2E测试 | 整个应用 | 用户场景 | 完整流程验证 |
1.2 测试工具配置
typescript
// package.json
{
"devDependencies": {
"@ohos/hypium": "^1.0.0",
"@ohos/test-runner": "^1.0.0"
},
"scripts": {
"test": "ets test",
"test:unit": "ets test -u",
"test:e2e": "ets test -e"
}
}
2. 单元测试实现
2.1 基本测试用例
typescript
// utils.test.ts
import { describe, it, expect } from '@ohos/hypium';
import { formatDate, calculateTotal } from '../utils';
describe('Utils Functions', () => {
// 日期格式化测试
it('should format date correctly', () => {
const date = new Date('2024-01-01');
expect(formatDate(date)).toBe('2024-01-01');
});
// 总计计算测试
it('should calculate total correctly', () => {
const items = [
{ price: 10, quantity: 2 },
{ price: 20, quantity: 1 }
];
expect(calculateTotal(items)).toBe(40);
});
// 边界条件测试
it('should handle empty items array', () => {
expect(calculateTotal([])).toBe(0);
});
});
2.2 异步测试
typescript
// service.test.ts
import { describe, it, expect } from '@ohos/hypium';
import { UserService } from '../services/user';
describe('UserService', () => {
let userService: UserService;
beforeEach(() => {
userService = new UserService();
});
// 异步请求测试
it('should fetch user data', async () => {
const user = await userService.getUser('123');
expect(user).not.toBeNull();
expect(user.id).toBe('123');
});
// 错误处理测试
it('should handle fetch error', async () => {
try {
await userService.getUser('invalid');
fail('Should throw error');
} catch (error) {
expect(error.message).toContain('User not found');
}
});
});
2.3 Mock和Stub
typescript
// api.mock.ts
import { Mock } from '@ohos/hypium';
export class ApiClientMock {
@Mock
async get(url: string): Promise<any> {
if (url.includes('users')) {
return {
id: '123',
name: 'Test User'
};
}
throw new Error('Not found');
}
}
// service.test.ts
describe('Service with Mocks', () => {
let service: UserService;
let apiClient: ApiClientMock;
beforeEach(() => {
apiClient = new ApiClientMock();
service = new UserService(apiClient);
});
it('should use mocked API client', async () => {
const user = await service.getUser('123');
expect(user.name).toBe('Test User');
expect(apiClient.get).toHaveBeenCalledWith('/users/123');
});
});
3. 组件测试方法
3.1 组件渲染测试
typescript
// button.test.ets
import { describe, it, expect } from '@ohos/hypium';
import { CustomButton } from '../components/Button';
describe('CustomButton Component', () => {
it('should render with correct text', () => {
const button = new CustomButton({
text: 'Click Me'
});
const wrapper = mount(button);
expect(wrapper.text()).toBe('Click Me');
});
it('should handle click event', () => {
const onClick = jest.fn();
const button = new CustomButton({
text: 'Click Me',
onClick
});
const wrapper = mount(button);
wrapper.trigger('click');
expect(onClick).toHaveBeenCalled();
});
});
3.2 状态变化测试
typescript
// counter.test.ets
describe('Counter Component', () => {
it('should update count on button click', async () => {
const counter = new Counter();
const wrapper = mount(counter);
expect(wrapper.find('#count').text()).toBe('0');
await wrapper.find('button').trigger('click');
expect(wrapper.find('#count').text()).toBe('1');
});
it('should handle state updates correctly', async () => {
const counter = new Counter();
const wrapper = mount(counter);
// 多次点击测试
for (let i = 0; i < 3; i++) {
await wrapper.find('button').trigger('click');
}
expect(wrapper.find('#count').text()).toBe('3');
});
});
4. 自动化测试框架
4.1 E2E测试配置
typescript
// e2e.config.ts
export default {
specs: ['./test/e2e/**/*.test.ts'],
capabilities: {
platformName: 'HarmonyOS',
deviceType: 'phone',
app: './dist/app.hap'
},
framework: '@ohos/test-runner'
};
// login.e2e.ts
describe('Login Flow', () => {
it('should login successfully', async () => {
await element(by.id('username'))
.typeText('testuser');
await element(by.id('password'))
.typeText('password123');
await element(by.text('Login'))
.tap();
// 验证登录成功
await expect(element(by.text('Welcome')))
.toBeVisible();
});
});
4.2 测试报告生成
typescript
// reporter.config.ts
export default {
reporters: [
'default',
['@ohos/test-reporter', {
outputDir: './test-results',
filename: 'test-report.html',
includeScreenshots: true
}]
]
};
5. 持续集成实践
5.1 CI配置示例
yaml
# .github/workflows/test.yml
name: Tests
on:
push:
branches: [ main ]
pull_request:
branches: [ main ]
jobs:
test:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v2
- name: Setup Node.js
uses: actions/setup-node@v2
with:
node-version: '14'
- name: Install dependencies
run: npm install
- name: Run tests
run: npm test
- name: Upload test results
uses: actions/upload-artifact@v2
with:
name: test-results
path: test-results/
5.2 测试自动化脚本
typescript
// scripts/test-automation.ts
import { TestRunner } from '@ohos/test-runner';
async function runTests() {
const runner = new TestRunner({
// 配置测试环境
environment: {
deviceId: process.env.DEVICE_ID,
platform: 'HarmonyOS'
},
// 配置测试套件
suites: [
{
name: 'Unit Tests',
pattern: 'test/unit/**/*.test.ts'
},
{
name: 'E2E Tests',
pattern: 'test/e2e/**/*.test.ts'
}
],
// 配置报告生成
reporters: [
{
name: 'html',
outputFile: 'test-results/report.html'
},
{
name: 'junit',
outputFile: 'test-results/junit.xml'
}
]
});
try {
const results = await runner.run();
console.log('Test Results:', results);
if (results.failed > 0) {
process.exit(1);
}
} catch (error) {
console.error('Test execution failed:', error);
process.exit(1);
}
}
runTests();
5.3 最佳实践建议
-
测试策略
- 制定合理的测试计划
- 确定测试覆盖率目标
- 优先测试关键功能
-
测试用例设计
- 覆盖边界条件
- 包含错误处理
- 保持用例独立性
-
测试维护
- 定期更新测试用例
- 清理过时的测试
- 优化测试性能
-
持续集成
- 自动化测试执行
- 及时反馈测试结果
- 集成代码覆盖率报告
通过建立完善的测试体系,可以有效保证应用的质量和稳定性。在实际开发中,要根据项目特点选择合适的测试策略,并持续优化测试流程。