1.安装opencv, 官网:https://opencv.org/releases/
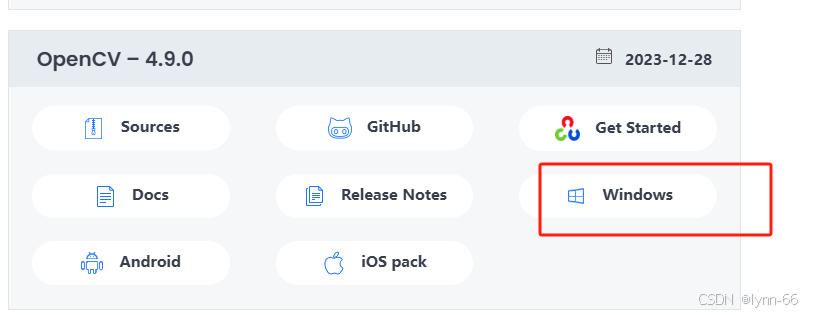
- 把opencv安装路径下的dll文件复制到jdk的bin目录下
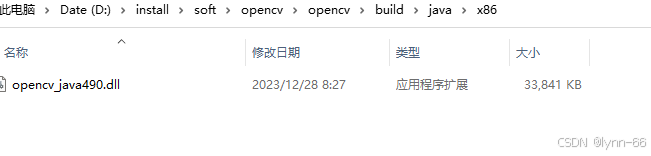
3.创建java的maven工程,配置opencv的jar包
<dependencies>
<dependency>
<groupId>org.openpnp</groupId>
<artifactId>opencv</artifactId>
<version>4.9.0-0</version> <!-- 根据实际版本调整 -->
</dependency>
</dependencies>
4.下载官网预训练的人脸检测模型文件(或者下载附件):
haarcascade_frontalface_default.xml
官网git地址:
opencv/data/haarcascades at 4.x · opencv/opencv · GitHub
5.编写代码
package org.example;
import org.opencv.core.*;
import org.opencv.imgcodecs.Imgcodecs;
import org.opencv.imgproc.Imgproc;
import org.opencv.objdetect.CascadeClassifier;
public class FaceRead {
public static void main(String[] args) {
// 加载OpenCV库
System.loadLibrary(Core.NATIVE_LIBRARY_NAME);
// 读取图片
String imagePath = "D:\\temp\\face\\1.jpg";
Mat image = Imgcodecs.imread(imagePath);
if (image.empty()) {
System.out.println("Could not open or find the image!");
System.exit(0);
}
// 加载预训练的人脸检测模型
String xmlPath = "D:\\temp\\opencv-4.x\\opencv-4.x\\data\\haarcascades\\haarcascade_frontalface_default.xml";
CascadeClassifier faceDetector = new CascadeClassifier(xmlPath);
if (faceDetector.empty()) {
System.out.println("Could not load the face detector model!");
System.exit(0);
}
// 检测人脸
MatOfRect faceDetections = new MatOfRect();
faceDetector.detectMultiScale(image, faceDetections);
// 遍历检测到的人脸
for (Rect rect : faceDetections.toArray()) {
// 在人脸周围绘制矩形框
Imgproc.rectangle(image, new Point(rect.x, rect.y), new Point(rect.x + rect.width, rect.y + rect.height), new Scalar(0, 255, 0), 3);
}
// 保存结果图片
String outputPath = "D:\\temp\\face\\output_image.jpg";
Imgcodecs.imwrite(outputPath, image);
System.out.println("Face detection complete. Result saved to " + outputPath);
}
}