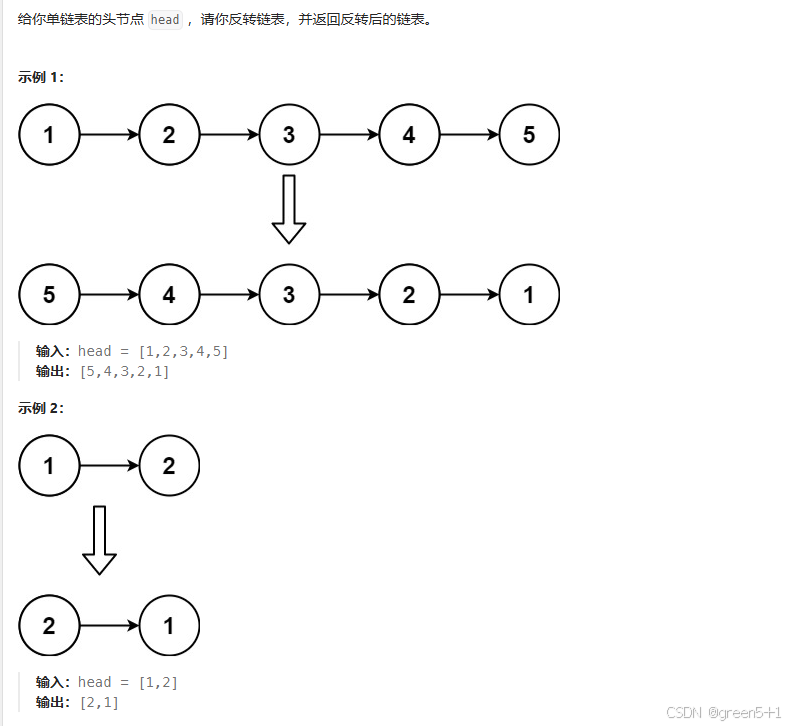
思路:关键在于,不要引起链表混乱,以及不要丢失链表,所以要注意指针的先后顺序
错误代码
c
/**
* Definition for singly-linked list.
* struct ListNode {
* int val;
* struct ListNode *next;
* };
*/
struct ListNode* reverseList(struct ListNode* head)
{
//
struct ListNode *pre=head;
struct ListNode *cur=head;
while(cur!=NULL)
{
cur=pre->next;//在这里会出现指针混淆,cur先更新为pre->next,后cur->next又指回pre,此时要把pre往后跳就会出现错误,因为逻辑上我们是希望pre跳到cur,但是cur的下一个指向了pre,所以就出现了逻辑bug
cur->next=pre;
pre=pre->next;
}
head->next=NULL;
head=cur;
return head;
}
AC代码
c
/**
* Definition for singly-linked list.
* struct ListNode {
* int val;
* struct ListNode *next;
* };
*/
struct ListNode* reverseList(struct ListNode* head)
{
//
struct ListNode *pre=NULL;
struct ListNode *cur=head;
while(head!=NULL)
{
//必须先让cur记住head->next,如果先让head->next指向null,那么head后续节点会丢失
cur=head->next;
//链表转向
head->next=pre;
//pre是后指针,跟上
pre=head;
//head指针往前跳
head=cur;
}
//循环终止是head=null,那么pre是后指针,终止的时候刚好指向最后一个节点,所以返回pre
return pre;
}