1.两数之和
题目
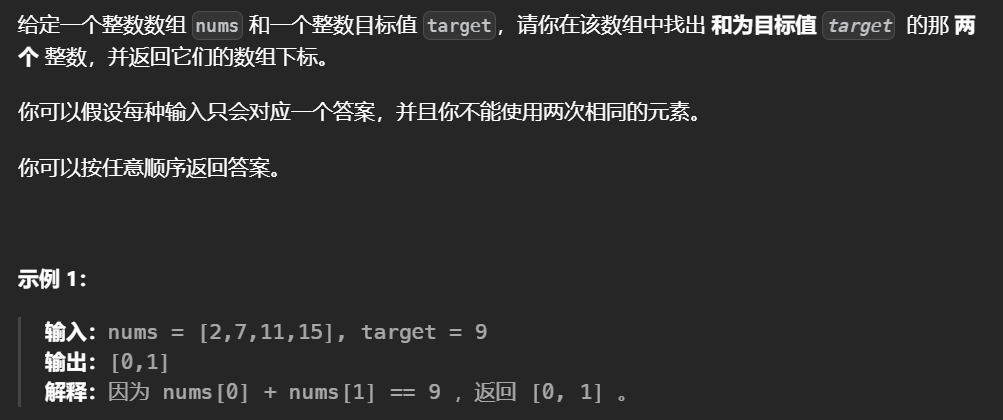
解析
- 枚举右指针 j,同时记录进哈希表,对于遍历的 nums[j],寻找哈希表中是否有 target - nums[j];
- 与双指针算法的不同点在于,本题需要记录下标,而双指针算法需要有序;
- 时间复杂度:O(n);空间复杂度:O(n);空间换时间
代码
cpp
class Solution {
public:
vector<int> twoSum(vector<int>& nums, int target) {
// 时间复杂度:O(n)
// 空间复杂度:O(n)
unordered_map<int,int> idx;
for(int j = 0; ;j ++){
auto it = idx.find(target - nums[j]);
if(it != idx.end()) { // 找到了
return {it -> second,j};
}
idx[nums[j]] = j;// 保存 nums[j] 和 j
}
}
};
2.好数对的数目
题目
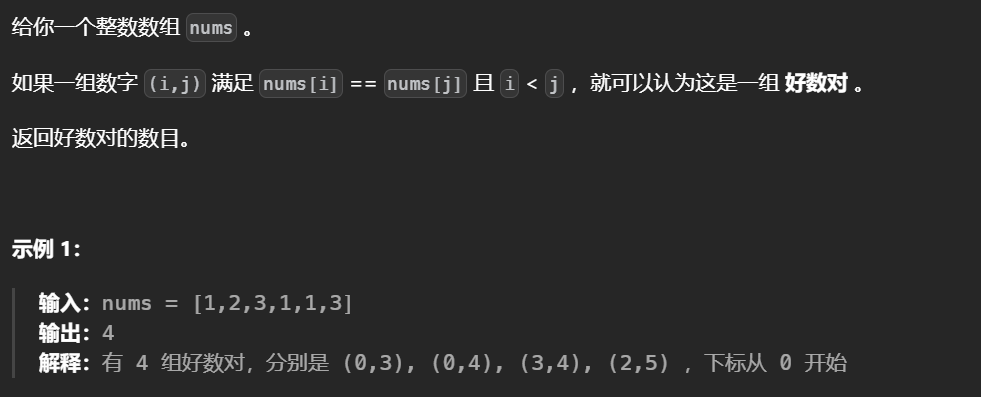
解析
- 同理可得
代码
cpp
class Solution {
public:
int numIdenticalPairs(vector<int>& nums) {
// 时间复杂度:O(n)
// 空间复杂度:O(n)
int res = 0;
unordered_map<int,int> cnt;
for(int j = 0;j < nums.size();j ++){
if(cnt[nums[j]]) res += cnt[nums[j]];
cnt[nums[j]] ++;
}
return res;
}
};
3.可互换矩形的组数
题目
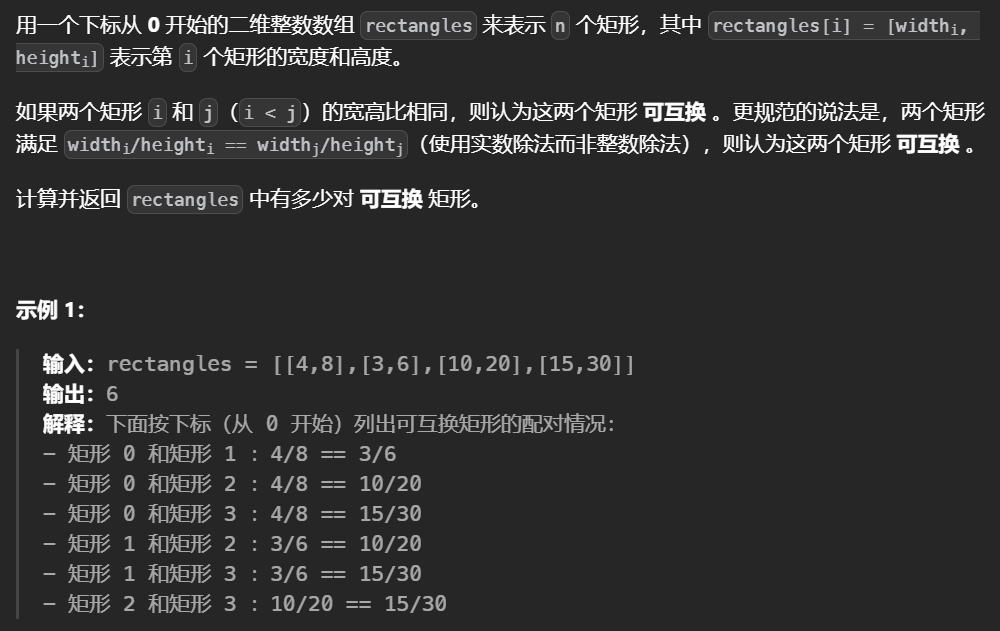
解析
- 同理可得,注意计算要 * 1.0;
代码
cpp
class Solution {
public:
long long interchangeableRectangles(vector<vector<int>>& rectangles) {
// 时间复杂度:O(n)
// 空间复杂度:O(n)
long long ans = 0;
unordered_map<double,int> cnt;
for(int j = 0;j < rectangles.size();j ++){
double x = rectangles[j][1] * 1.0 / rectangles[j][0];
if(cnt[x]) ans += cnt[x];
cnt[x] ++;
}
return ans;
}
};