一、当前页面直接获取
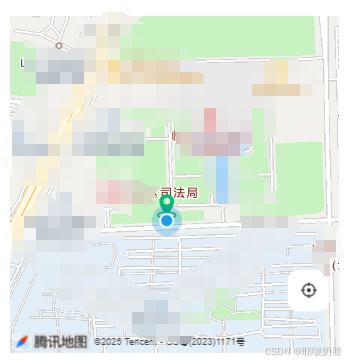
html
<view class="map">
<map id="myMap" style="width: 100%; height: 40vh;" :latitude="latitude"
:longitude="longitude" :markers="markers" :scale="scale" :show-location="true" />
<!-- 定位图标 -->
<view class="location_center" @click="moveToLocation">
<image style="width: 32rpx;height: 32rpx;" src="/static/images/map/location_center.png"
mode="aspectFill">
</image>
</view>
</view>
javascript
// 定义地图中心经纬度
const latitude = ref(30.2672);
const longitude = ref(120.1531);
// 定义地图缩放级别
const scale = ref(16);
// 定义标记点数组
const markers = ref([{
id: 1,
latitude: 30.2672,
longitude: 120.1531,
iconPath: '/static/images/map/marker.png', // 电动车图标路径
width: 30, // 图标宽度
height: 30 // 图标高度
}, {
id: 2,
latitude: 35.08729,
longitude: 118.40184,
iconPath: '/static/images/map/marker.png', // 电动车图标路径
width: 30, // 图标宽度
height: 30 // 图标高度
}, {
id: 3,
latitude: 36.08729,
longitude: 119.40184,
iconPath: '/static/images/map/marker.png', // 电动车图标路径
width: 30, // 图标宽度
height: 30 // 图标高度
}]);
const _mapContext = ref(null)
onLoad(() => {
location.init()
console.log(location.state.latitude);
console.log(location.state.longitude);
// getCurrentLocation(); // 在页面加载时调用获取位置的函数
})
onReady(() => {
_mapContext.value = uni.createMapContext("myMap"); // 在页面初次渲染完成后初始化地图上下文
})
// 获取当前位置经纬度的函数
const getCurrentLocation = () => {
uni.getLocation({
type: 'wgs84', // 返回的坐标类型,这里使用 WGS84 坐标
success: (res) => {
// 获取成功,将经纬度赋值给变量
latitude.value = res.latitude;
longitude.value = res.longitude;
console.log('纬度:', res.latitude);
console.log('经度:', res.longitude);
},
fail: (err) => {
// 获取失败,打印错误信息
console.error('获取位置失败:', err);
uni.showToast({
title: '获取位置失败,请检查权限',
icon: 'none'
});
}
});
}
// 定位移动到当前位置
const moveToLocation = () => {
// console.log(latitude.value,longitude.value);
_mapContext.value.moveToLocation({
latitude: latitude.value,
longitude: longitude.value
})
}
二、封装的组件
locationStore.js文件
javascript
import { defineStore } from 'pinia';
import { authorization } from '@/libs/common/location';
import { ref, reactive } from 'vue';
export const locationStore = defineStore('location', () => {
const state = reactive({
latitude: '',
longitude: '',
isLoading: true, // 表示是否正在加载位置信息
error: null, // 存储可能出现的错误信息
status: '定位中' // 新增状态,用于显示定位状态信息
});
const isInit = ref(false);
/**
* 位置初始化
*/
async function init() {
try {
const location = await authorization();
state.latitude = location.latitude;
state.longitude = location.longitude;
state.status = '定位成功'; // 定位成功,更新状态
isInit.value = true;
state.isLoading = false;
state.error = null;
} catch (err) {
state.error = err;
state.status = '定位失败,请开启定位权限'; // 定位失败,更新状态
state.isLoading = false;
console.error('获取位置信息失败:', err);
}
}
return {
init,
state,
isInit
};
});
使用
html
<view class="map">
<map id="myMap" style="width: 100%; height: 40vh;" :latitude="latitude"
:longitude="longitude" :markers="markers" :scale="scale" :show-location="true" />
<!-- 定位图标 -->
<view class="location_center" @click="moveToLocation">
<image style="width: 32rpx;height: 32rpx;" src="/static/images/map/location_center.png"
mode="aspectFill">
</image>
</view>
</view>
javascript
<script setup>
import {
onLoad,
onReady,
} from '@dcloudio/uni-app'
import {
ref,
} from "vue";
import {
locationStore
} from '@/store/locationStore.js'
const location = locationStore()
// 地图相关start
// 定义地图中心经纬度
const latitude = ref(33.2672);
const longitude = ref(121.1531);
// 定义地图缩放级别
const scale = ref(16);
// 定义标记点数组
const markers = ref([{
id: 1,
latitude: 30.2672,
longitude: 120.1531,
iconPath: '/static/images/map/marker.png', // 电动车图标路径
width: 30, // 图标宽度
height: 30 // 图标高度
}, {
id: 2,
latitude: 35.08729,
longitude: 118.40184,
iconPath: '/static/images/map/marker.png', // 电动车图标路径
width: 30, // 图标宽度
height: 30 // 图标高度
}, {
id: 3,
latitude: 36.08729,
longitude: 119.40184,
iconPath: '/static/images/map/marker.png', // 电动车图标路径
width: 30, // 图标宽度
height: 30 // 图标高度
}]);
const _mapContext = ref(null)
// 地图相关end
onLoad(async () => {
try {
// 等待 init 方法中的异步操作完成
await location.init();
// 此时可以获取到最新的经纬度
latitude.value = location.state.latitude;
longitude.value = location.state.longitude;
} catch (error) {
console.error('位置初始化失败:', error);
}
})
onReady(() => {
_mapContext.value = uni.createMapContext("myMap"); // 在页面初次渲染完成后初始化地图上下文
})
// 定位移动到当前位置
const moveToLocation = () => {
// console.log(latitude.value,longitude.value);
_mapContext.value.moveToLocation({
latitude: latitude.value,
longitude: longitude.value
})
}
</script>
css
.map {
position: relative;
margin-top: 30rpx;
// 定位图标
.location_center {
position: absolute;
bottom: 5vh;
right: 20rpx;
width: 80rpx;
height: 80rpx;
border-radius: 20rpx;
display: flex;
align-items: center;
justify-content: center;
background-color: #ffffff;
image {
width: 80rpx;
height: 80rpx;
}
}
}