rust
use tokio::{sync::oneshot, time::{sleep, Duration}};
async fn check_for_one() {
// This loop will continuously print "write" every second until interrupted
loop {
println!("write");
sleep(Duration::from_secs(1)).await; // Non-blocking sleep in async context
}
}
#[tokio::main]
async fn main() {
// Create a oneshot channel
let (tx1, rx1) = oneshot::channel::<&str>();
// Spawn a task that sends a message after 2 seconds
tokio::spawn(async move {
sleep(Duration::from_secs(2)).await;
let _ = tx1.send("one");
});
// Use tokio::select! to wait for either the print task or the message on rx1
tokio::select! {
_ = check_for_one() => {
// This branch will continuously print "write" every second
println!("check_for_one completed");
}
val = rx1 => {
// This branch will be executed once the message is received from rx1
match val {
Ok(val) => println!("rx1 completed first with {:?}", val),
Err(e) => println!("Failed to receive from rx1: {:?}", e),
}
}
}
println!("main thread exiting");
}
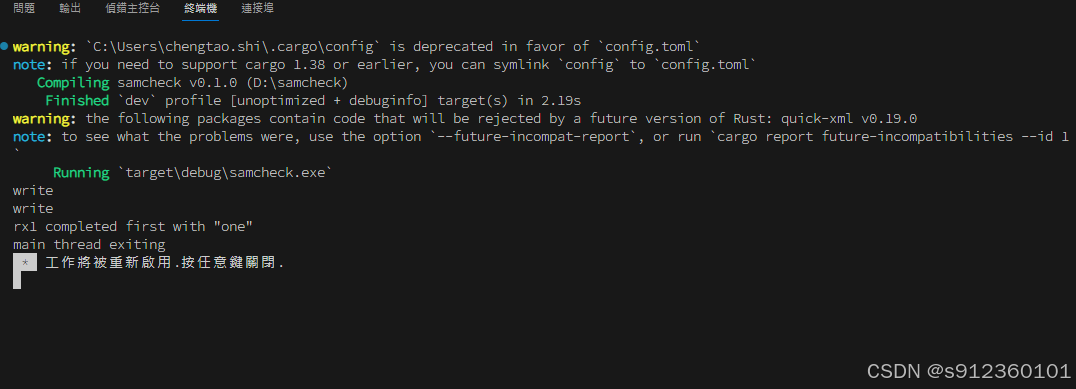
只要有一个异步任务完成,就会退出select! 。