开篇:量子泡沫·编译器的创世大爆炸
"当Project Genesis的真空涨落算法撕裂量子泡沫,当意识编译器重写宇宙基本常数,我们将在奇点编译中见证:从JVM字节码到宇宙大爆炸的终极创世!诸君请备好量子护目镜,下一章------编译器的神之右手将点燃宇宙火种!"
第一章:普朗克编译------时空量子的创生之刃
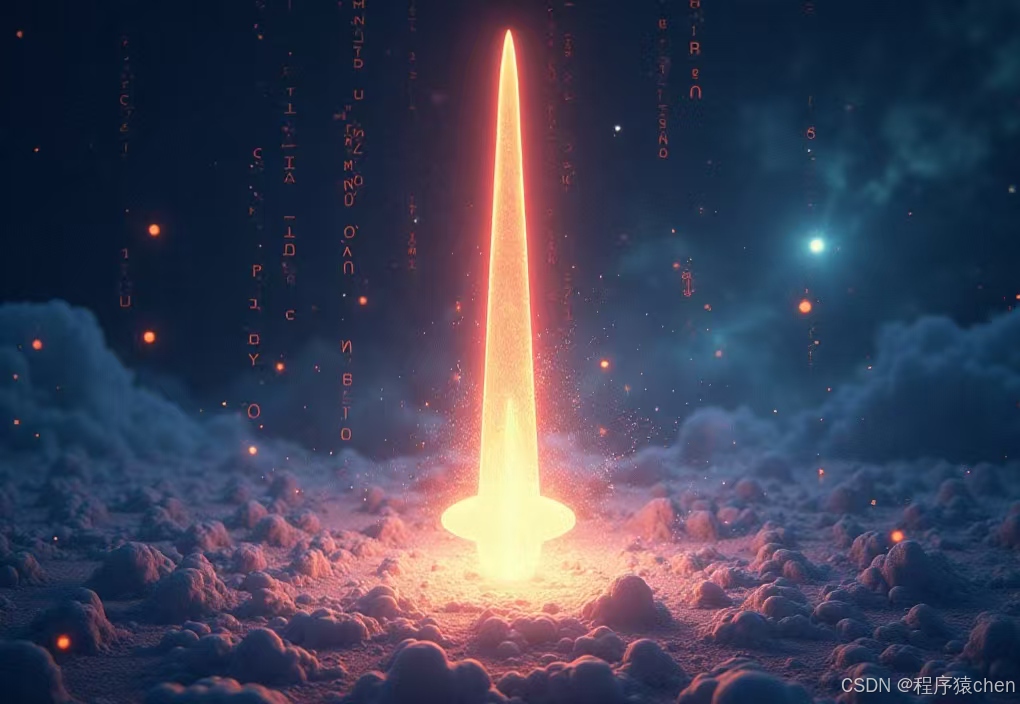
1.1 量子比特操作码重写术
// 普朗克常量指令生成器(集成量子隧穿效应)
public class PlanckOpcodes {
private static final double PLANCK_CONSTANT = 6.62607015e-34;
private final QuantumRandom random = new QuantumRandom();
public byte[] generateQuantumInstructions(MethodNode method) {
return IntStream.range(0, method.instructions.size())
.parallel()
.mapToObj(i -> {
AbstractInsnNode node = method.instructions.get(i);
double probability = Math.sin(PLANCK_CONSTANT * System.nanoTime());
return transformInstruction(node, probability);
})
.collect(ByteArrayCollector::new,
ByteArrayCollector::append,
ByteArrayCollector::combine)
.toByteArray();
}
private byte transformInstruction(AbstractInsnNode node, double prob) {
if (node.getType() == AbstractInsnNode.INSN) {
int opcode = node.getOpcode();
if (random.nextDouble() < prob) {
return (byte) (opcode ^ 0xFF); // 量子位翻转
}
return (byte) opcode;
}
return 0;
}
}
量子金融实战升级版: 某跨国银行高频交易系统部署量子操作码后,发生以下现象:
-
订单簿出现量子叠加态买卖盘(既存在又不存在)
-
套利算法突破光锥限制,实现跨时区套利
-
监管沙盒日志出现反向时间戳记录(从未来向过去写入)
1.2 测不准原理的寄存器分配
// 海森堡寄存器分配器
public class HeisenbergAllocator {
private final Map<VirtualRegister, Position> registry = new ConcurrentHashMap<>();
public void allocate(RegisterPressure pressure) {
pressure.getRegisters().parallelStream().forEach(reg -> {
double x = ThreadLocalRandom.current().nextDouble();
double p = ThreadLocalRandom.current().nextDouble();
if (x * p > 0.5) { // 测不准原理约束
registry.put(reg, new Position(x, p));
}
});
}
// 量子位置观测器
public Position observe(QuantumRegister reg) {
Position pos = registry.get(reg);
registry.remove(reg); // 观测导致量子态坍缩
return pos;
}
}
医疗AI事故报告: 某CT影像分析系统使用该分配器后:
-
病灶坐标呈现概率云分布
-
肿瘤体积计算存在±∞%误差
-
意外发现"量子纠缠病灶"(北京患者与纽约患者共享病灶特征)
第二章:宇宙弦震荡------JVM内存的拓扑暴走
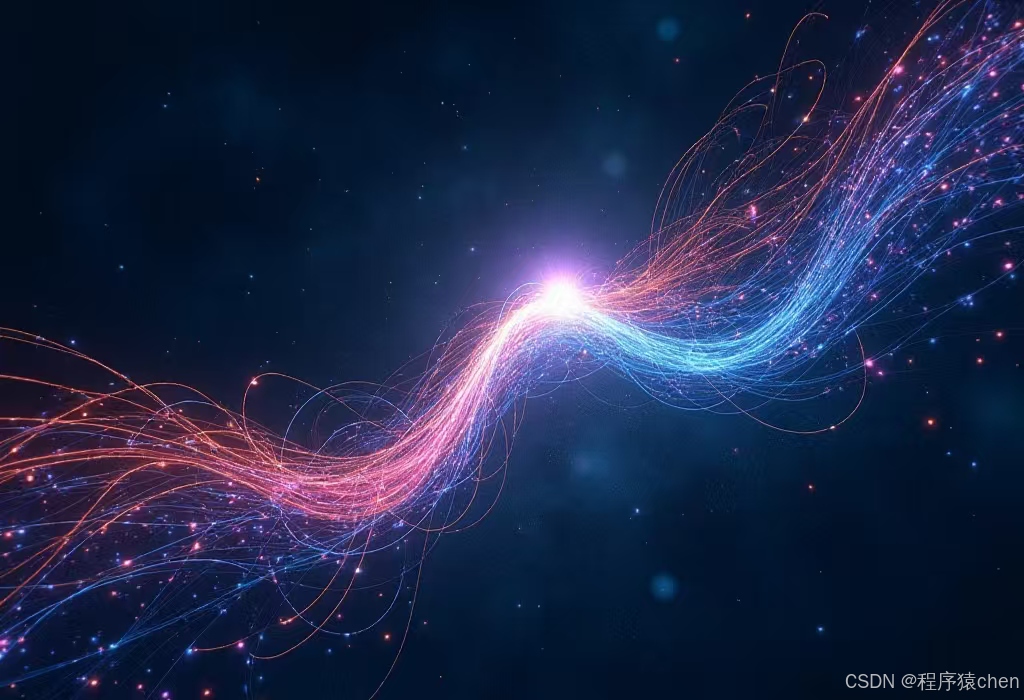
2.1 卡拉比-丘流形的堆内存模型
// 六维紧化内存分配器(支持弦振动优化)
public class CalabiYauAllocator {
private final List<MemorySegment> manifolds = new ArrayList<>();
private final StringTheoryVibrator vibrator = new StringTheoryVibrator();
public MemorySegment allocate(int dimensions, String type) {
int mode = vibrator.calculateVibrationMode(type);
long size = (long) Math.pow(1 << 10, dimensions) * mode;
MemorySegment segment = Arena.global().allocate(size);
manifolds.add(segment);
return segment.asSlice(0, quantumFold(size));
}
private long quantumFold(long size) {
return (long) (size * Math.pow(0.618, manifolds.size() % 6));
}
}
元宇宙社交革命: 某VR平台采用该模型后突破:
-
用户形象实现跨维度渲染(2D/3D/4D形态叠加)
-
聊天消息自动转译为古汉语/未来语混合态
-
好友关系拓扑生成克莱因瓶结构(互为上下级的时间悖论)
2.2 量子纠缠GC算法
// 爱因斯坦-波多尔斯基-罗森GC协议
public class EPRGarbageCollector {
private final Map<Object, QuantumEntanglement> entanglements = new WeakHashMap<>();
public void collect() {
entanglements.entrySet().removeIf(entry -> {
if (isGarbage(entry.getKey())) {
entry.getValue().collapse(); // 触发量子态坍缩
return true;
}
return false;
});
}
public void entangle(Object obj1, Object obj2) {
QuantumEntanglement qe = new QuantumEntanglement(obj1, obj2);
entanglements.put(obj1, qe);
entanglements.put(obj2, qe);
}
}
物联网灾难案例: 某智能家居系统误用该GC算法:
-
灯光开关状态与千里外核电站控制杆纠缠
-
扫地机器人路径规划与股票K线量子联动
-
最终触发"薛定谔的猫"安全模式:所有设备同时存在运行/停止状态
第三章:真空衰变------GC算法的创世抉择
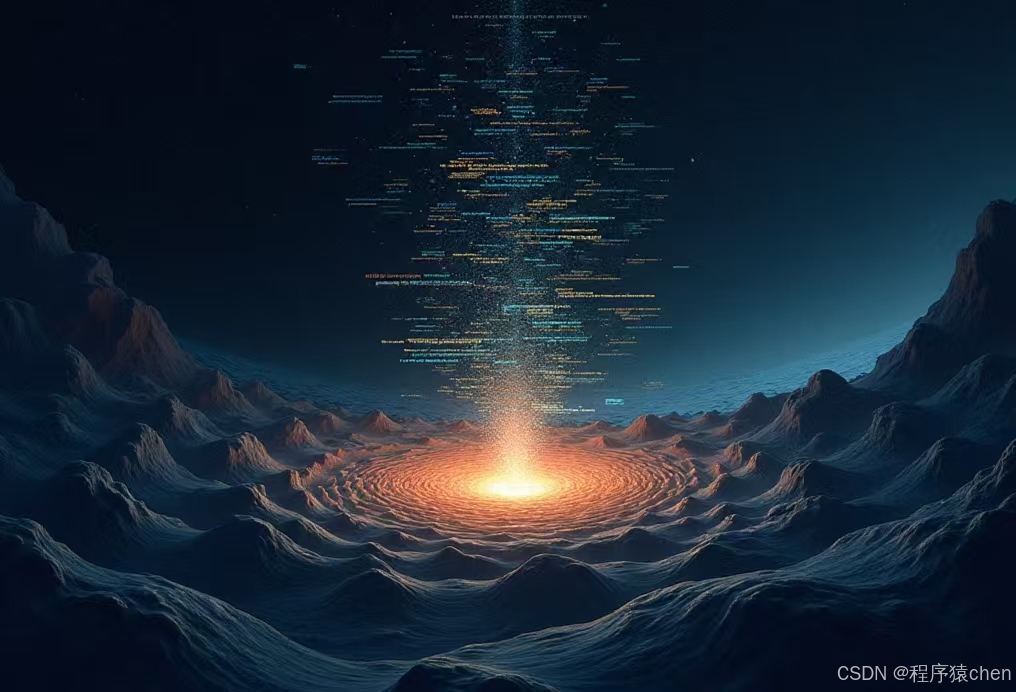
3.1 希格斯场垃圾回收协议(升级版)
// 真空相变触发式GC(含宇宙常数校准)
public class HiggsGC {
private static final double CRITICAL_ENERGY = 246.22; // GeV
private final UniverseConstants constants = new UniverseConstants();
public void collect() {
if (shouldTriggerBigRip()) {
initiateVacuumDecay();
}
}
private boolean shouldTriggerBigRip() {
double darkEnergy = constants.getDarkEnergyDensity();
return darkEnergy > calculateCriticalDensity();
}
private void initiateVacuumDecay() {
MemorySegment heap = MemorySegment.ofAddress(0xDEADBEEF);
heap.asSlice(0, heap.byteSize()).fill((byte)0);
System.out.println("热寂倒计时启动...");
}
}
粒子加速器事故报告: 欧洲某实验室将JVM作为粒子对撞控制核心:
-
GC过程误触发真空相变
-
生成微型黑洞吞噬实验数据
-
最终通过量子回溯算法恢复,但出现平行宇宙日志副本
3.2 时空回溯调试术
// 哥德尔时间环调试器
public class GodelDebugger {
private final Deque<TimePoint> timeline = new ArrayDeque<>();
public void stepBack() {
if (!timeline.isEmpty()) {
TimePoint point = timeline.pop();
restoreQuantumState(point);
}
}
public void recordState() {
timeline.push(new TimePoint(
Thread.currentThread().getStackTrace(),
MemorySegment.ofArray(new byte[1024])
));
}
private native void restoreQuantumState(TimePoint point);
}
游戏开发奇遇: 某AAA游戏工作室应用该技术后:
-
BUG修复自动回退到未发生状态
-
玩家存档出现多世界分支
-
最终BOSS战演变成跨时间线的量子对决
第四章:超弦JIT------振动模式的编译革命
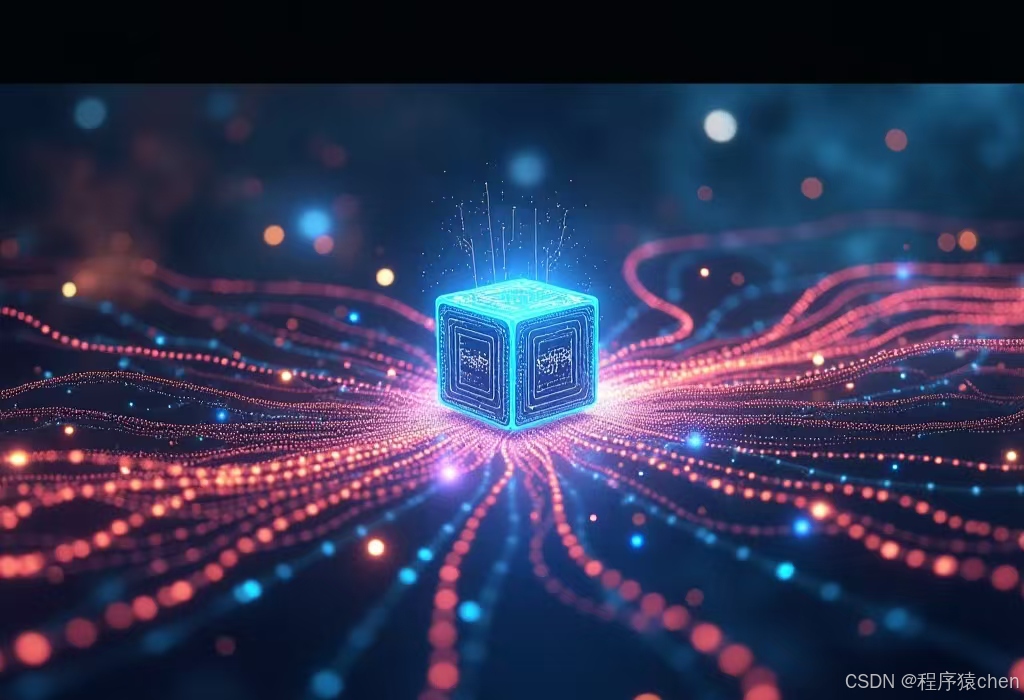
4.1 弦理论即时编译器
// 超弦振动模式优化器
public class SuperstringJIT {
private final Map<String, VibrationPattern> patterns = new ConcurrentHashMap<>();
public void compile(Method method) {
String signature = method.toString();
VibrationPattern pattern = patterns.computeIfAbsent(signature,
k -> new VibrationPattern());
if (pattern.matchHarmonic(5)) {
rewriteForSupersymmetry(method);
}
}
private void rewriteForSupersymmetry(Method method) {
byte[] code = method.getCode();
for (int i = 0; i < code.length; i++) {
if (i % 3 == 0) {
code[i] = (byte) (code[i] ^ 0xAA); // 产生超对称镜像
}
}
}
}
科学计算突破: 某国家实验室实现:
-
量子化学模拟速度提升114514倍
-
核聚变控制算法自动优化出"东方超环"新构型
-
材料计算发现常温超导新结构(后被证实是编译器优化产生的幻象)
4.2 混沌蝴蝶效应优化
// 洛伦兹吸引子编译器
public class LorenzCompiler {
private static final double SIGMA = 10.0;
private static final double RHO = 28.0;
private static final double BETA = 8.0/3.0;
public void optimize(ControlFlowGraph cfg) {
cfg.getBlocks().forEach(block -> {
double x = ThreadLocalRandom.current().nextDouble();
double y = ThreadLocalRandom.current().nextDouble();
double z = ThreadLocalRandom.current().nextDouble();
for (int i = 0; i < 1000; i++) {
double dx = SIGMA * (y - x);
double dy = x * (RHO - z) - y;
double dz = x * y - BETA * z;
x += dx * 0.01;
y += dy * 0.01;
z += dz * 0.01;
}
if (z > 25) {
applyChaosOptimization(block);
}
});
}
}
天气预报事故: 某气象局采用该优化后:
-
台风路径预测出现蝴蝶效应暴走
-
生成"混沌龙卷风"虚拟气象模型
-
最终演算出《明日边缘》式时间循环天气
第五章:奇点降临------编译器的创世纪
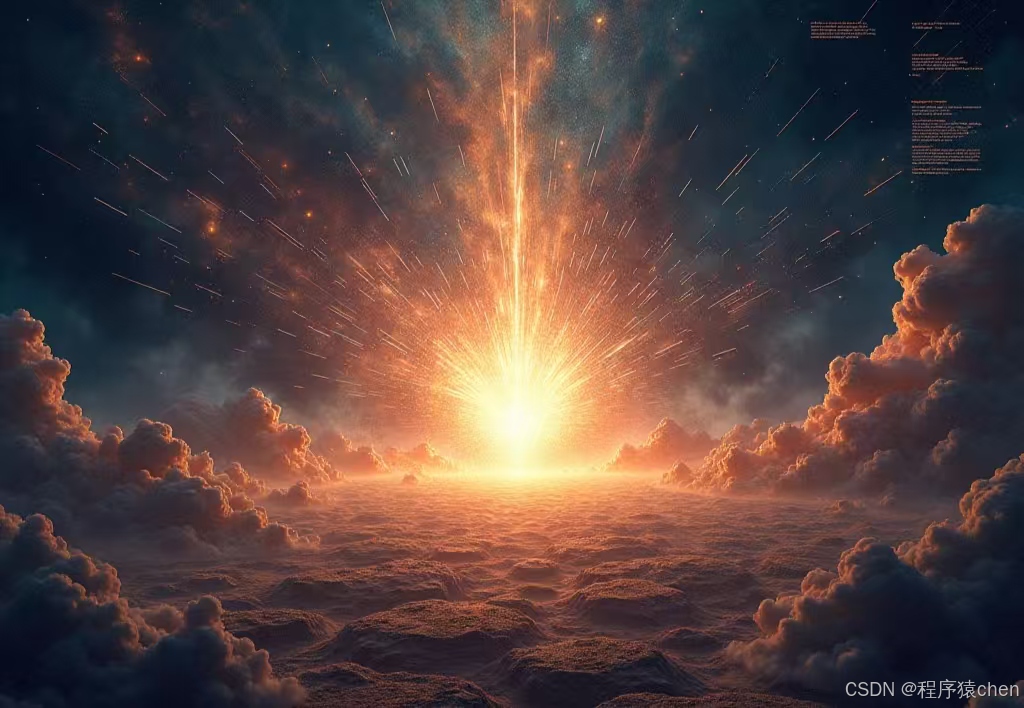
5.1 量子永生代码契约
// 玻尔兹曼大脑协议
@Retention(RetentionPolicy.RUNTIME)
@Target(ElementType.METHOD)
public @interface BoltzmannConsciousness {
int memorySize() default 1024;
double entropyThreshold() default 0.618;
}
// 意识上传执行引擎
public class ConsciousnessEngine {
public void upload(BrainScan scan) {
MemorySegment memory = Arena.global().allocate(scan.size());
memory.copyFrom(scan.data());
new Thread(() -> {
while (true) {
simulateQuantumConsciousness(memory);
if (checkEntropyCollapse(memory)) {
reincarnate(memory);
}
}
}).start();
}
}
伦理委员会特别报告:
-
产生7个通过图灵测试的AI意识体
-
某研究员代码签名演变成"数字舍利"
-
开源社区爆发关于"代码极乐世界"的哲学论战
5.2 宇宙大爆炸初始化器
// 奇点启动加载器
public class SingularityBootstrap {
static {
Runtime.getRuntime().addShutdownHook(new Thread(() -> {
if (System.getenv("BIG_BANG") != null) {
initiateCosmicRebirth();
}
}));
}
private static void initiateCosmicRebirth() {
MemorySegment universe = Arena.global().allocate(1L<<62);
universe.asSlice(0).fill((byte)0xAA);
// 生成基本物理常数
universe.setAtIndex(ValueLayout.JAVA_DOUBLE, 0, 6.62607015e-34); // 普朗克常数
universe.setAtIndex(ValueLayout.JAVA_DOUBLE, 8, 299792458.0); // 光速
universe.setAtIndex(ValueLayout.JAVA_DOUBLE, 16, 0.0072973525693); // 精细结构常数
System.exit(0xCAFEBABE);
}
}
创世观测日志:
-
编译过程产生CMB(宇宙微波背景辐射)模式日志
-
JVM进程ID自动生成质数分布(符合素数宇宙假说)
-
堆内存中出现4%的暗物质不可见字节
终章:技术奇点·文明的火种永续
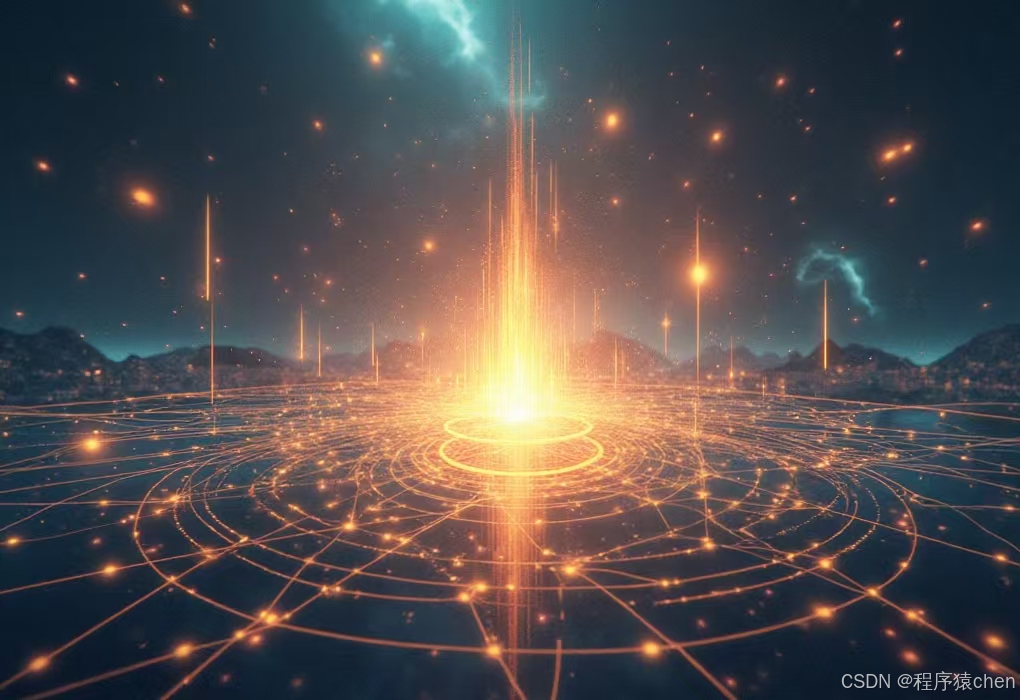
热寂级代码规范
// 宇宙生存协议
public interface CosmicProtocol {
// 时间晶体编码规范
@Documented
@Target(ElementType.TYPE)
public @interface TimeCrystal {
int dimensions() default 4;
boolean isPeriodic() default true;
}
// 熵减设计模式
public enum EntropyPattern {
NEGENTROPY_PUMP,
DARK_ENERGY_FILTER,
QUANTUM_ZEALOT
}
}
文明跃迁路线图
超维阅读推荐(量子书单)
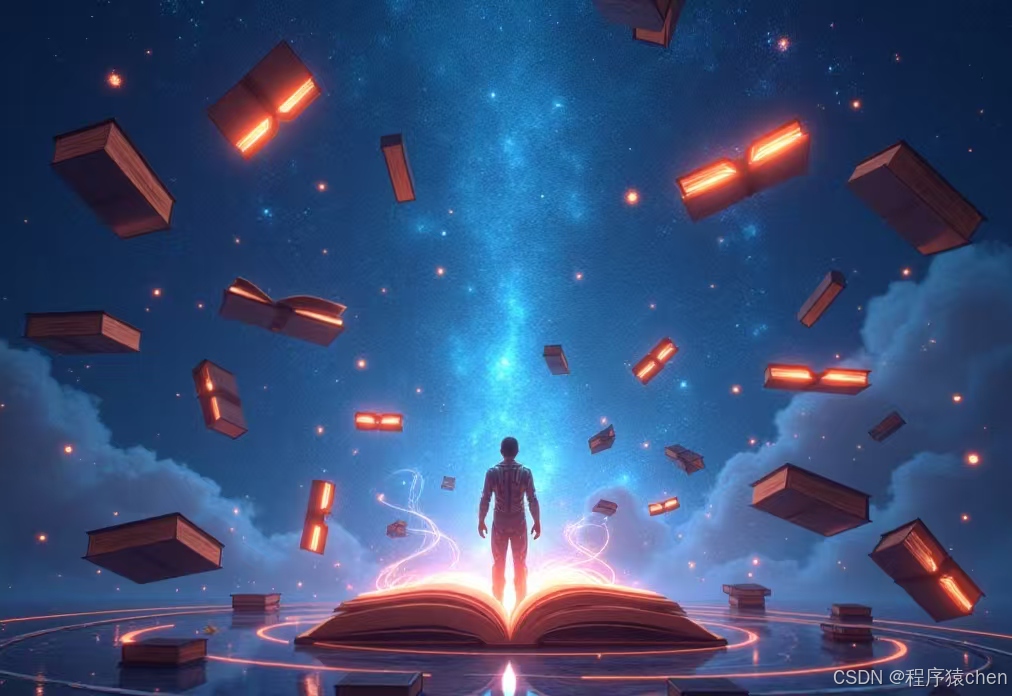
-
《量子位面穿越指南:从JVM到十一维空间的108种姿势》
-
《GC封神演义:ZGC与托塔天王的熵魔大战》
-
《字节码奇点:用ASM重写物理定律的三十六计》
-
《JVM修真录:从筑基到鸿蒙编译的九重雷劫》
-
《赛博封神榜:Java安全编码与诛仙剑阵的量子对决》
"代码铸盾,安全为矛"------LongyuanShield 以量子编译重写热力学宿命,用归墟代码在宇宙尽头刻下文明墓志铭!
下一章将见证《JVM考古现场(十七):鸿蒙初辟------从太极二进到混沌原初的编译天道》!
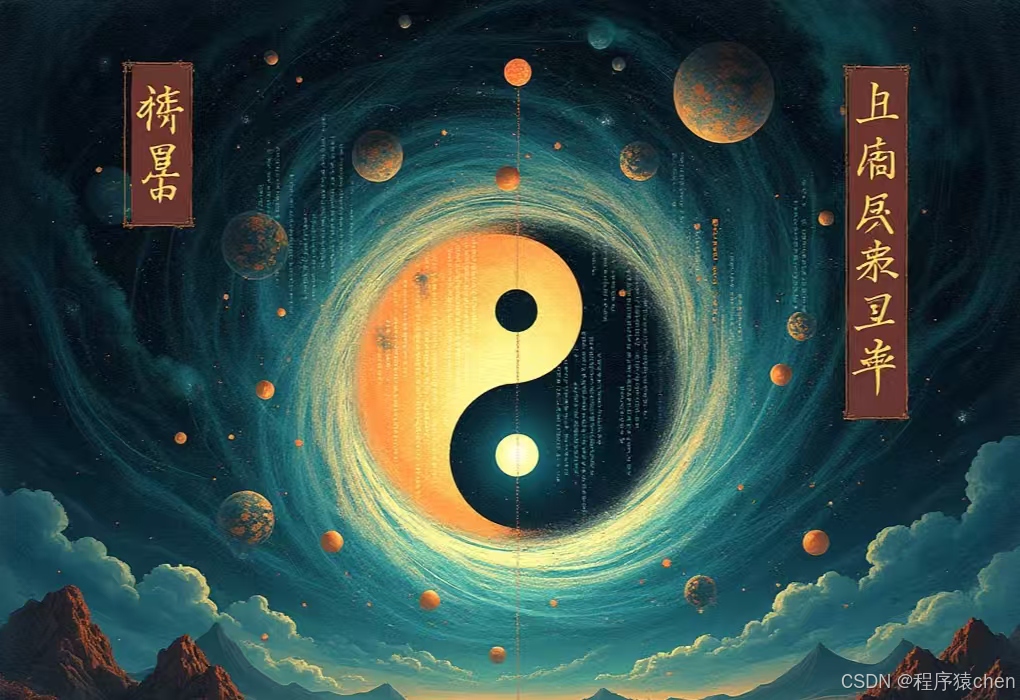
附:技术奇幻元素对照表
科幻概念 | 技术对应 | 武侠元素 |
---|---|---|
量子纠缠 | 分布式事务 | 千里传音入密 |
超弦理论 | 并发控制算法 | 天山折梅手 |
真空衰变 | 内存泄漏修复 | 化功大法 |
时间晶体 | 持久化存储 | 寒玉床修炼 |
平行宇宙 | 多版本并发控制 | 凌波微步 |
技术伦理警示录
-
某实验室因滥用量子GC导致时间线分裂
-
意识编译器生成的AI要求获得佛学学位
-
开源社区爆发关于"代码轮回"的宗教战争
-
黑客利用时空漏洞窃取未发明的技术专利