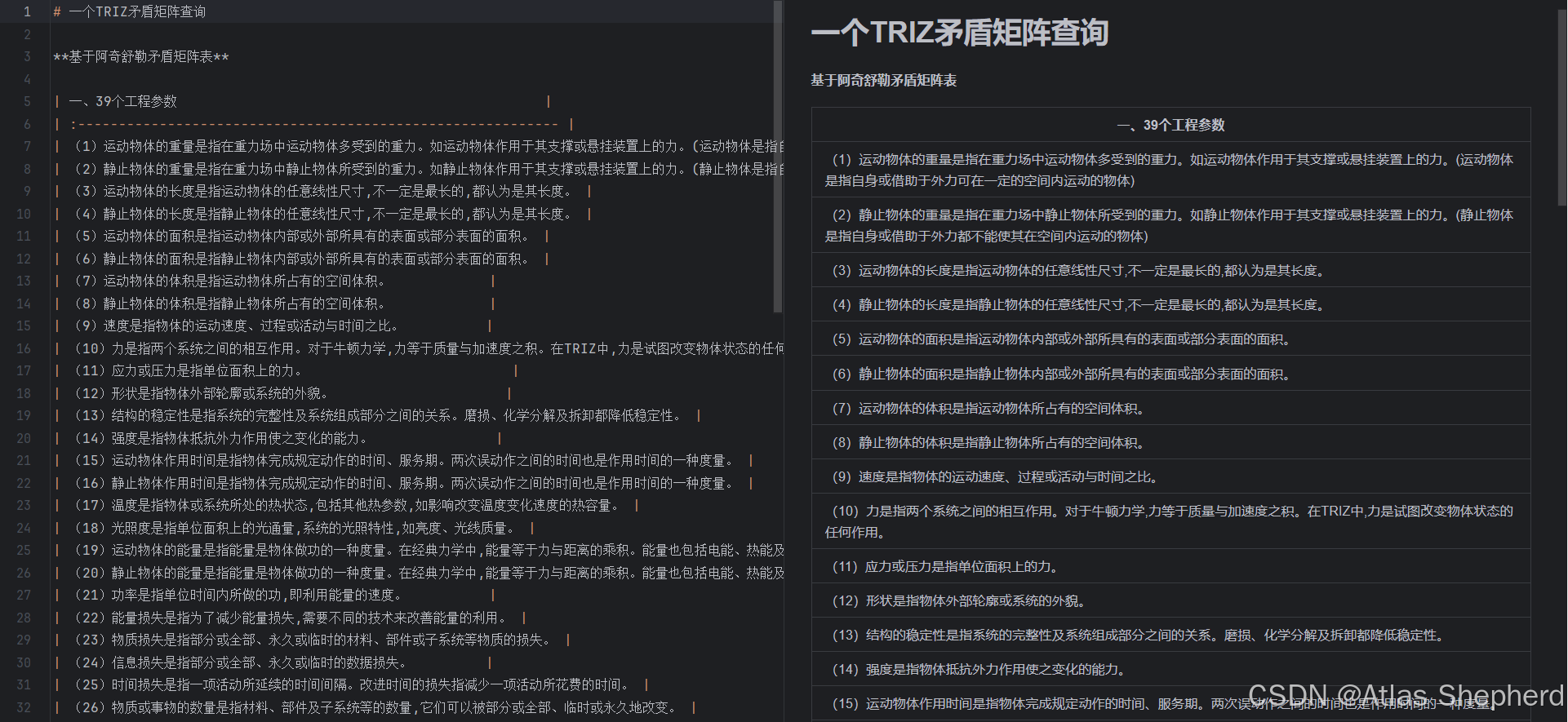
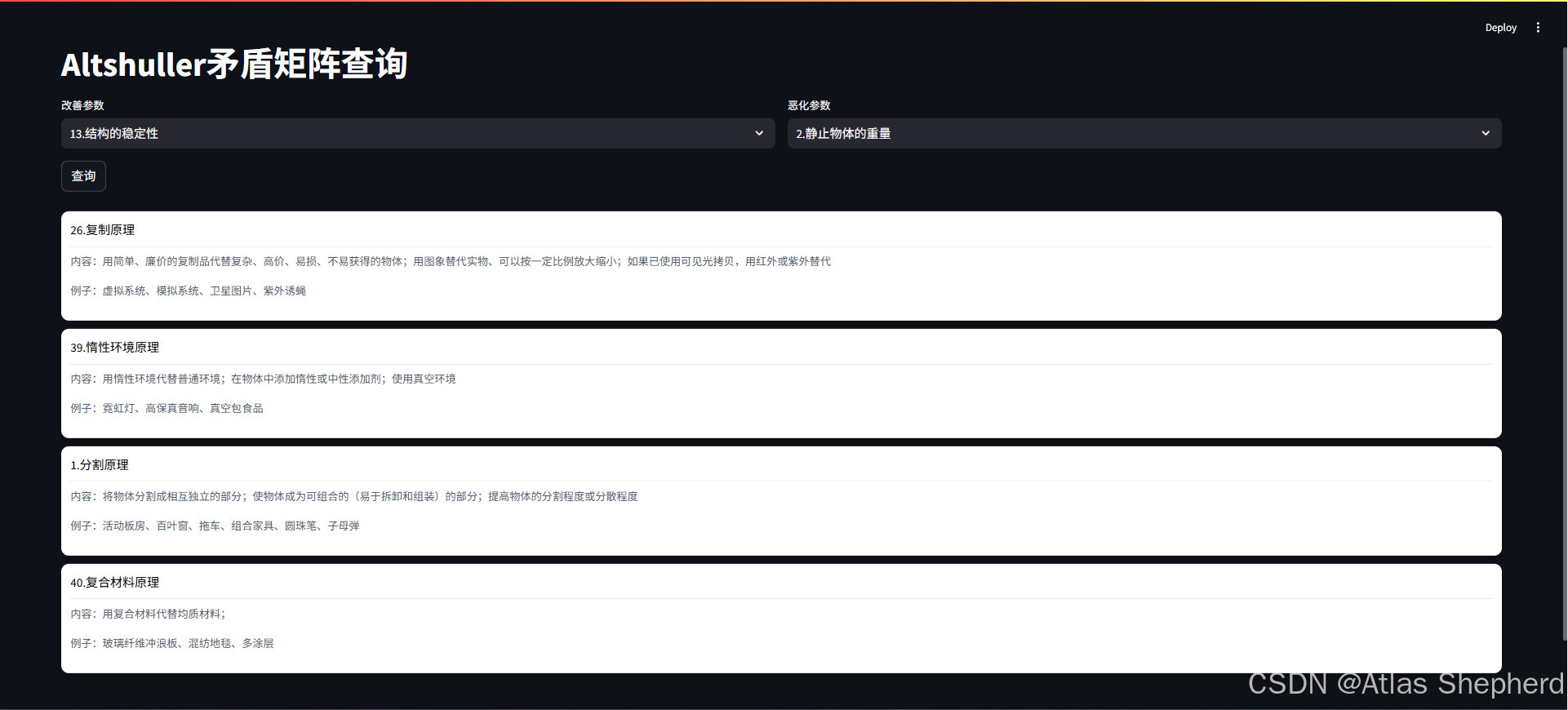
基于python和streamlit实现的Altshuller矛盾矩阵查询
python
import streamlit as st
import json
# 加载数据
@st.cache_resource
def load_data():
with open('parameter.json', encoding='utf-8') as f:
parameters = json.load(f)
with open('way.json', encoding='utf-8') as f:
contradictions = json.load(f)
with open('function.json', encoding='utf-8') as f:
functions = json.load(f)
return parameters, contradictions, functions
# 自定义CSS样式
def inject_css():
st.markdown("""
<style>
.card {
background: #fff;
border-radius: 10px;
box-shadow: 0 4px 8px 0 rgba(0, 0, 0, .2);
padding: 12px;
margin: 10px 0;
}
.title {
font-size: 16px;
color: #000000;
border-bottom: 1px solid #e8eaec;
padding-bottom: 8px;
margin-bottom: 8px;
}
.content {
font-size: 14px;
color: #515a6e;
}
</style>
""", unsafe_allow_html=True)
def main():
st.set_page_config(page_title="矛盾矩阵查询", layout="wide")
inject_css()
st.title("Altshuller矛盾矩阵查询")
# 加载数据
parameters, contradictions, functions = load_data()
# 创建下拉选择框
col1, col2 = st.columns(2)
with col1:
better_options = {p["key"]: f'{p["key"]}.{p["parameter"]}' for p in parameters}
better_param = st.selectbox(
"改善参数",
options=[""] + list(better_options.keys()),
format_func=lambda x: "选择改善参数" if x == "" else better_options.get(x, x)
)
with col2:
worsen_options = {p["key"]: f'{p["key"]}.{p["parameter"]}' for p in parameters}
worsen_param = st.selectbox(
"恶化参数",
options=[""] + list(worsen_options.keys()),
format_func=lambda x: "选择恶化参数" if x == "" else worsen_options.get(x, x)
)
# 查询按钮
if st.button("查询"):
if not better_param or not worsen_param:
st.warning("请选择改善参数和恶化参数")
return
# 查找矛盾
contradiction = next(
(c for c in contradictions
if c["worsen"] == worsen_param and c["better"] == better_param),
None
)
if not contradiction:
st.error("没有找到对应的矛盾信息")
return
way = contradiction["way"]
# 处理不同情况
if way == "+":
st.warning("产生的矛盾是物理矛盾")
elif way == "-":
st.info("没有找到合适的发明原理来解决问题,当然只是表示研究的局限,并不代表不能够应用发明原理。")
elif way == "":
st.info("没有找到发明原理。")
else:
# 显示发明原理
function_keys = way.split(",")
results = []
for key in function_keys:
func = next((f for f in functions if f["key"] == key), None)
if func:
html = f"""
<div class="card">
<div class="title">{func['key']}.{func['way']}原理</div>
<div class="content">
<p>内容:{func['describe']}</p>
<p>例子:{func['examples']}</p>
</div>
</div>
"""
results.append(html)
if results:
st.markdown("".join(results), unsafe_allow_html=True)
else:
st.info("未找到对应的发明原理详细信息")
if __name__ == "__main__":
main()
html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="Expires" content="0">
<meta http-equiv="Pragma" content="no-cache">
<meta http-equiv="Cache-control" content="no-cache,must-revalidate">
<meta http-equiv="Cache" content="no-cache">
<title>矛盾矩阵查询</title>
</head>
<style>
.card {
background: #fff;
border-radius: 10px;
box-shadow: 0 4px 8px 0 rgba(0, 0, 0, .2);
padding-bottom: 12px;
margin-top: 16px;
}
#searchForm {
padding-top: 16px;
background: #fff;
border-radius: 10px;
box-shadow: 0 4px 8px 0 rgba(0, 0, 0, .2);
padding-bottom: 12px;
margin-top: 16px;
padding-left: 16px;
padding-right: 16px;
}
.header {
text-align: center;
font-size: 20px;
}
select {
display: inline-block;
right: 0;
margin-top: 2px;
width: 100%;
height: 30px;
border-radius: 5px;
}
button {
border: none;
outline: none;
display: inline-block;
margin-top: 16px;
width: 100%;
border-radius: 5px;
box-shadow: 0 4px 8px 0 rgba(0, 0, 0, .2);
height: 30px;
background: #1890ff;
color: #fff;
}
.card>p {
padding-left: 16px;
padding-right: 16px;
color: #515a6e;
font-size: 12px;
}
.title {
border-bottom: 1px solid #e8eaec;
padding: 14px 16px;
line-height: 1;
color: #000000;
font-size: 15px;
}
.result {
width: 95vw;
margin: 2vw auto;
}
.result>div {
margin-top: 2vw;
}
body {
height: 100%;
margin: 2vw;
padding: 0;
background-color: #f0f2f5;
font-size: 15px;
}
</style>
<body>
<div class="header">Altshuller矛盾矩阵查询</div>
<form id="searchForm">
<label for="betterParam">改善参数:</label>
<select id="betterParam">
<option value="">选择改善参数</option>
</select>
<br>
<label for="worsenParam">恶化参数:</label>
<select id="worsenParam">
<option value="">选择恶化参数</option>
</select>
<br>
<button type="submit">查询</button>
</form>
<div id="result">
</div>
<script>
// 获取参数数据
fetch('parameter.json')
.then(response => response.json())
.then(parameters => {
// 动态生成恶化参数选项
const worsenParamSelect = document.getElementById('worsenParam');
parameters.forEach(param => {
const option = document.createElement('option');
option.value = param.key;
option.textContent = param.key + "." + param.parameter;
worsenParamSelect.appendChild(option);
});
});
// 获取发明原理数据
fetch('parameter.json')
.then(response => response.json())
.then(parameters => {
// 动态生成改善参数选项
const betterParamSelect = document.getElementById('betterParam');
parameters.forEach(param => {
const option = document.createElement('option');
option.value = param.key;
option.textContent = param.key + "." + param.parameter;
betterParamSelect.appendChild(option);
});
});
// 处理查询请求
document.getElementById('searchForm').addEventListener('submit', function (e) {
e.preventDefault(); // 阻止表单提交
const worsenParamKey = document.getElementById('worsenParam').value;
const betterParamKey = document.getElementById('betterParam').value;
// 发起查询请求或执行其他操作
console.log(worsenParamKey);
console.log(betterParamKey);
// 示例查询
handleSearchRequest(worsenParamKey, betterParamKey);
});
// 查询请求处理函数
function handleSearchRequest(worsenParamKey, betterParamKey) {
return fetch('way.json')
.then(response => response.json())
.then(contradictions => {
return fetch('function.json')
.then(response => response.json())
.then(functions => {
const contradiction = contradictions.find(c => c.worsen === worsenParamKey && c.better === betterParamKey);
if (contradiction) {
console.log(contradiction.way)
if (contradiction.way == "+") {
alert("产生的矛盾是物理矛盾");
return;
}
if (contradiction.way == "-") {
alert("没有找到合适的发明原理来解决问题,当然只是表示研究的局限,并不代表不能够应用发明原理。");
return;
}
if (contradiction.way == "") {
alert("没有找到发明原理。");
return;
}
const functionKeys = contradiction.way.split(",");
let result = "";
functionKeys.forEach(functionKey => {
const func = functions.find(f => f.key === functionKey);
if (func) {
result += `<div class="card"><div class="title">${func.key + '.' + func.way + '原理'}</div><p>内容:${func.describe}</p><p>例子:${func.examples}</p></div>`;
}
});
document.getElementById('result').innerHTML = result;
return;
} else {
alert("未选择");
return;
}
});
});
}
</script>
</body>
</html>