前言
在现代软件开发中,网络通信是构建分布式系统和实现服务间交互的核心组成部分。无论是微服务架构中的服务调用、RESTful API的集成,还是基础的HTTP请求处理,开发者都需要依赖高效、稳定且功能丰富的工具来实现网络通信。HttpClient作为Java平台中一个强大的HTTP客户端库,提供了全面的API支持,能够高效地处理HTTP请求与响应,并支持异步通信等现代网络协议特性。本文将系统性地介绍HttpClient的核心概念、使用方法,并通过实战案例探讨其在实际项目中的实践与应用场景。
一、HttpClient简介
Java 11的HttpClient(位于java.net.http包中)是Java标准库中引入的现代化HTTP客户端API,它旨在替代传统的HttpURLConnection,通过更简洁、灵活且功能丰富的设计,满足现代应用对高性能网络通信的需求。作为Java原生支持的HTTP客户端,它不仅支持HTTP/1.1和HTTP/2协议,还兼容WebSocket通信,并通过异步非阻塞模型显著提升高并发场景下的吞吐效率。
HttpClient的核心特性如下:
-
原生支持HTTP/2协议:HttpClient默认启用HTTP/2协议,可自动协商协议版本(如服务端不支持HTTP/2则降级为HTTP/1.1)。通过多路复用(Multiplexing)技术,单连接可并行处理多个请求/响应,减少TCP连接数,并且可以结合头部压缩(HPACK)技术,显著降低网络开销,提升通信效率。
-
异步非阻塞通信模型:提供sendAsync()方法实现异步请求处理,基于CompletableFuture和响应式编程模型,允许开发者通过回调或链式操作处理响应。非阻塞I/O机制避免线程资源浪费,适用于高并发场景(如微服务调用、实时数据处理)。
-
高度可扩展的配置机制:通过HttpClient.Builder链式接口,开发者可灵活配置以下参数:连接超时(connectTimeout)、代理服务器(proxy)、SSL/TLS上下文(sslContext)、重定向策略(followRedirects)、认证机制(authenticator)等。并且其还支持自定义拦截器(如日志、重试逻辑)以扩展功能。
-
简洁直观的API设计:API分层清晰,核心类(HttpClient、HttpRequest、HttpResponse)职责明确:
- HttpRequest.Builder构建请求,支持设置URI、请求头、请求体(支持多种数据格式,如字符串、文件、字节流)。
- HttpResponse.BodyHandlers提供响应体处理模板(如字符串、文件、JSON反序列化),例如发送GET请求仅需数行代码即可完成构建、发送和响应解析。
-
与Java生态无缝集成:作为Java标准库的一部分,无需引入第三方依赖,天然兼容java.util.concurrent、java.nio等模块,并可通过ServiceLoader机制扩展协议实现(如自定义HTTP版本)。
二、HttpClient基础使用
1. 创建HttpClient实例
HttpClient实例的创建支持两种核心方式:通过默认工厂方法快速构建基础实例,或通过构建器(HttpClient.Builder)实现深度定制化配置,开发者可根据项目需求选择合适的方式。
创建默认实例:
通过HttpClient.newHttpClient()静态方法可直接获取预配置的HttpClient实例,该实例采用以下默认参数:
arduino
// 创建默认配置的HttpClient实例
HttpClient defaultClient = HttpClient.newHttpClient();
通过构建器定制化配置:
通过HttpClient.newBuilder()获取构建器对象,可逐项设置关键参数以创建定制的客户端实例,以下是典型配置示例:
java
import java.net.InetSocketAddress;
import java.net.http.HttpClient;
import java.security.NoSuchAlgorithmException;
import java.time.Duration;
import java.net.ProxySelector;
import java.net.Authenticator;
import java.util.concurrent.Executors;
import javax.net.ssl.SSLContext;
public class CustomHttpClientExample {
public static void main(String[] args) throws NoSuchAlgorithmException {
HttpClient customClient = HttpClient.newBuilder()
// 强制使用HTTP/2(若服务端不支持则抛出异常)
.version(HttpClient.Version.HTTP_2)
// 设置TCP连接超时为15秒
.connectTimeout(Duration.ofSeconds(15))
// 自动跟随所有重定向
.followRedirects(HttpClient.Redirect.ALWAYS)
// 设置代理
.proxy(ProxySelector.of(new InetSocketAddress("proxy.example.com", 8080)))
// 自定义SSL上下文(如TLS配置)
.sslContext(SSLContext.getDefault())
// 设置认证处理器(如Basic Auth)
.authenticator(Authenticator.getDefault())
// 指定异步执行器(Java 19 +)
.executor(Executors.newVirtualThreadPerTaskExecutor())
.build();
}
}
关键配置项详解:
配置方法 | 功能说明 | 默认值 |
---|---|---|
.version(Version) | 指定HTTP协议版本(HTTP_1.1/HTTP_2) | 自动协商(优先HTTP/2) |
.connectTimeout(Duration) | 设置TCP连接建立超时时间 | 无超时 |
.followRedirects(策略) | 定义重定向处理策略(ALWAYS/NEVER/NORMAL) | NEVER(不自动重定向) |
.proxy(ProxySelector) | 设置代理服务器地址及端口 | 系统默认代理 |
.sslContext(SSLContext) | 配置SSL/TLS上下文(如证书验证、加密套件) | 系统默认SSL上下文 |
.authenticator(Authenticator) | 设置认证处理器(如Basic Auth、OAuth2 Token注入) | 无 |
.executor(Executor) | 指定异步请求执行器(可集成虚拟线程、线程池等) | ForkJoinPool.commonPool() |
注意事项:
- 协议版本:若需强制使用HTTP/2,需确保服务端支持,否则抛出IOException。推荐优先使用自动协商(默认行为)。
- 超时控制:生产环境务必设置connectTimeout,避免因网络问题导致线程阻塞。
- 异步执行器:在Java 19+项目中,可通过Executors.newVirtualThreadPerTaskExecutor()启用虚拟线程,显著提升异步请求吞吐量。
2. 发送GET请求
发送GET请求是HttpClient最常见的用法之一,可以通过HttpRequest.newBuilder()创建一个HttpRequest,然后使用HttpClient发送请求并获取响应。
java
import java.net.http.HttpClient;
import java.net.URI;
import java.net.http.HttpRequest;
import java.net.http.HttpResponse;
public class HttpClientExample {
public static void main(String[] args) throws Exception {
HttpClient httpClient = HttpClient.newHttpClient();
HttpRequest request = HttpRequest.newBuilder()
.uri(URI.create("https://jsonplaceholder.typicode.com/posts/1"))
.GET()
.build();
HttpResponse<String> response = httpClient.send(request, HttpResponse.BodyHandlers.ofString());
System.out.println("Status Code: " + response.statusCode());
System.out.println("Response Body: " + response.body());
}
}
这段代码演示了如何使用java.net.http.HttpClient API来发送一个HTTP GET请求到指定的URL(在这个例子中是jsonplaceholder.typicode.com/posts/1),并接收服务器的响应。程序首先创建了一个HttpClient实例,然后构建了一个HttpRequest对象,设置了请求的URI和HTTP方法(GET)。接着程序使用HttpClient的send方法发送请求,并指定响应体应该被解析为字符串。最后程序打印出响应的状态码和响应体,其中响应体包含了从服务器获取的数据(在这个例子中是一个JSON格式的帖子信息)。
jsonplaceholder.typicode.com/posts/1 的内容如下:

运行结果如下:
swift
Status Code: 200
Response Body: {
"userId": 1,
"id": 1,
"title": "sunt aut facere repellat provident occaecati excepturi optio reprehenderit",
"body": "quia et suscipit\nsuscipit recusandae consequuntur expedita et cum\nreprehenderit molestiae ut ut quas totam\nnostrum rerum est autem sunt rem eveniet architecto"
}
3. 发送POST请求
发送POST请求与GET请求类似,只是需要在请求体中包含要发送的数据,通常POST请求用于提交表单数据或JSON数据。
java
import java.net.URI;
import java.net.http.HttpClient;
import java.net.http.HttpRequest;
import java.net.http.HttpResponse;
import java.nio.charset.StandardCharsets;
public class HttpClientExample {
public static void main(String[] args) throws Exception {
HttpClient httpClient = HttpClient.newHttpClient();
String json = "{"title":"foo","body":"bar","userId":1}";
HttpRequest request = HttpRequest.newBuilder()
.uri(URI.create("https://jsonplaceholder.typicode.com/posts"))
.header("Content-Type", "application/json")
.POST(HttpRequest.BodyPublishers.ofString(json, StandardCharsets.UTF_8))
.build();
HttpResponse<String> response = httpClient.send(request, HttpResponse.BodyHandlers.ofString());
System.out.println("Status Code: " + response.statusCode());
System.out.println("Response Body: " + response.body());
}
}
这段代码展示了如何使用java.net.http.HttpClient API来发送一个HTTP POST请求。代码中首先创建了一个HttpClient实例,然后构建了一个HttpRequest对象,设置了请求的URI为jsonplaceholder.typicode.com/posts ,请求头Content-Type为application/json,以及一个JSON格式的请求体。请求体包含了标题(title)、正文(body)和用户ID(userId)。接着使用HttpClient的send方法发送请求,并指定响应体应该被解析为字符串。最后程序打印出响应的状态码和响应体。
运行结果:
css
Status Code: 201
Response Body: {"title":"foo","body":"bar","userId":1,"id":101}
4. 异步请求
HttpClient支持异步请求,可以在不阻塞主线程的情况下发送请求并处理响应,可以通过sendAsync方法可以发送异步请求,并返回一个CompletableFuture对象。
java
import java.net.URI;
import java.net.http.HttpClient;
import java.net.http.HttpRequest;
import java.net.http.HttpResponse;
import java.util.concurrent.CompletableFuture;
public class HttpClientExample {
public static void main(String[] args) {
HttpClient httpClient = HttpClient.newHttpClient();
HttpRequest request = HttpRequest.newBuilder()
.uri(URI.create("https://jsonplaceholder.typicode.com/posts/1"))
.GET()
.build();
CompletableFuture<HttpResponse<String>> future = httpClient.sendAsync(request, HttpResponse.BodyHandlers.ofString());
future.thenApply(HttpResponse::body)
.thenAccept(System.out::println)
.join();
}
}
这段代码通过Java的HttpClient API异步发送一个HTTP GET请求到jsonplaceholder.typicode.com/posts/1 ,并处理响应。它创建了HttpClient和HttpRequest对象来配置请求,之后使用httpClient.sendAsync方法发送请求,并指定响应体应被解析为字符串。接着通过链式调用thenApply和thenAccept方法,在响应完成时提取响应体并将其打印到控制台,并且使用join()方法被调用以阻塞主线程,直到异步操作完成,确保响应体被打印出来。
运行结果:
swift
{
"userId": 1,
"id": 1,
"title": "sunt aut facere repellat provident occaecati excepturi optio reprehenderit",
"body": "quia et suscipit\nsuscipit recusandae consequuntur expedita et cum\nreprehenderit molestiae ut ut quas totam\nnostrum rerum est autem sunt rem eveniet architecto"
}
三、HttpClient实战案例
1. 调用RESTful API案例
在实际开发中,经常需要调用外部的RESTful API来获取数据或执行某些操作,RESTful API通常返回JSON格式的数据,开发中可以使用Jackson或Gson等库来解析JSON响应,下面是一个使用HttpClient调用GitHub API获取用户信息并且使用Jackson解析GitHub API返回的JSON数据的案例:
java
import com.fasterxml.jackson.databind.ObjectMapper;
import java.net.URI;
import java.net.http.HttpClient;
import java.net.http.HttpRequest;
import java.net.http.HttpResponse;
public class GitHubApiExample {
public static void main(String[] args) throws Exception {
HttpClient httpClient = HttpClient.newHttpClient();
HttpRequest request = HttpRequest.newBuilder()
.uri(URI.create("https://api.github.com/users/octocat"))
.GET()
.build();
HttpResponse<String> response = httpClient.send(request, HttpResponse.BodyHandlers.ofString());
ObjectMapper objectMapper = new ObjectMapper();
GitHubUser user = objectMapper.readValue(response.body(), GitHubUser.class);
System.out.println("User Login: " + user.getLogin());
System.out.println("User Name: " + user.getName());
}
}
class GitHubUser {
private String login;
private String name;
public String getLogin() {
return login;
}
public void setLogin(String login) {
this.login = login;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
}
该案例是利用HttpClient API向GitHub的API发送HTTP GET请求,以获取用户octocat的信息。程序首先通过导入必要的类来准备环境,包括Jackson库的ObjectMapper类用于JSON数据的转换。在GitHubApiExample类的main方法中,创建了一个HttpClient实例,并构建了一个指向api.github.com/users/octoc... 的HttpRequest对象。之后使用httpClient.send方法同步发送请求,并指定响应体应解析为字符串。接收到的响应体通过Jackson的ObjectMapper实例被转换为GitHubUser类的实例,该类定义了两个私有字段login和name,以及相应的getter和setter方法。最后程序打印出用户的登录名和姓名。
api.github.com/users/octoc... 的内容如下:
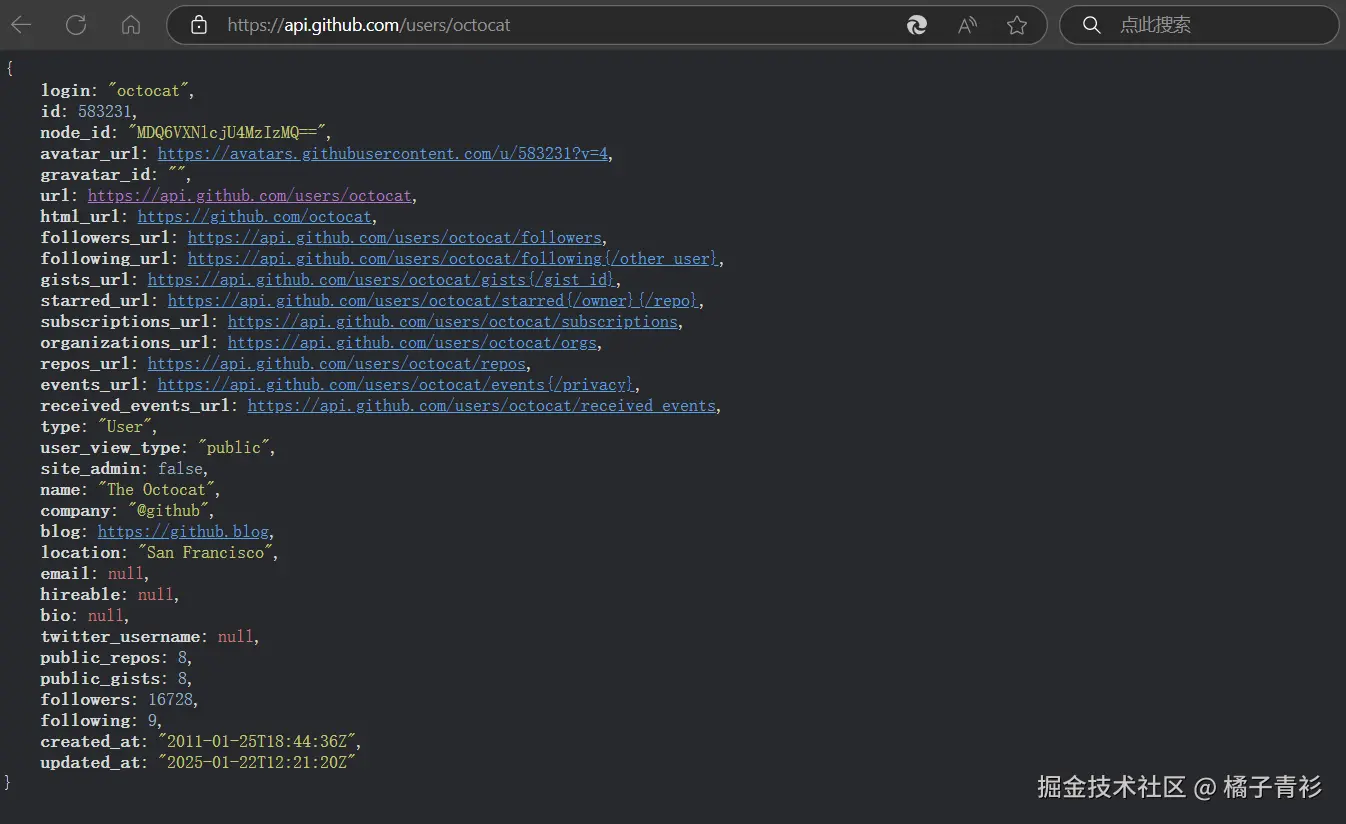
运行结果:
sql
User Login: octocat
User Name: The Octocat
2. 处理异常和重试机制
在实际应用中,网络请求可能会因为各种原因失败,为了提高系统的健壮性,通常需要实现重试机制,下面是一个简单的重试机制实现:
java
import java.net.URI;
import java.net.http.HttpClient;
import java.net.http.HttpRequest;
import java.net.http.HttpResponse;
import java.time.Duration;
import java.util.concurrent.atomic.AtomicInteger;
public class GitHubApiExample {
private static final int MAX_RETRIES = 3;
public static void main(String[] args) throws Exception {
HttpClient httpClient = HttpClient.newHttpClient();
HttpRequest request = HttpRequest.newBuilder()
.uri(URI.create("https://api.github.com/users/octocat"))
.GET()
.build();
AtomicInteger retryCount = new AtomicInteger(0);
while (retryCount.get() < MAX_RETRIES) {
try {
HttpResponse<String> response = httpClient.send(request, HttpResponse.BodyHandlers.ofString());
System.out.println("Status Code: " + response.statusCode());
System.out.println("Response Body: " + response.body());
break;
} catch (Exception e) {
retryCount.incrementAndGet();
System.out.println("Retry attempt: " + retryCount.get());
if (retryCount.get() == MAX_RETRIES) {
throw e;
}
// 等待2秒后重试
Thread.sleep(Duration.ofSeconds(2).toMillis());
}
}
}
}
该案例利用Java的HttpClient API来向GitHub的API发送HTTP GET请求,以获取用户octocat的信息。程序中定义了一个最大重试次数MAX_RETRIES为3的私有静态常量,并在main方法中创建了一个HttpClient实例和一个指向api.github.com/users/octoc... 的HttpRequest对象。为了处理可能的网络或API访问问题,程序实现了一个重试机制:使用AtomicInteger来线程安全地计数重试次数,并在一个while循环中尝试发送请求。如果请求成功,则打印状态码和响应体并跳出循环;如果发生异常,则增加重试次数,并在达到最大重试次数前等待2秒后再次尝试,如果重试次数达到限制,则重新抛出异常终止程序(最多重试 3 次,并在每次重试之间等待 2 秒)。
如果一次请求成功(即返回状态码为 200),程序将输出以下内容:
vbscript
Status Code: 200
Response Body: {
"login": "octocat",
"id": 583231,
"node_id": "MDQ6VXNlcjU4MzIzMQ==",
"avatar_url": "https://avatars.githubusercontent.com/u/583231?v=4",
"gravatar_id": "",
"url": "https://api.github.com/users/octocat",
"html_url": "https://github.com/octocat",
"followers_url": "https://api.github.com/users/octocat/followers",
"following_url": "https://api.github.com/users/octocat/following{/other_user}",
"gists_url": "https://api.github.com/users/octocat/gists{/gist_id}",
"starred_url": "https://api.github.com/users/octocat/starred{/owner}{/repo}",
"subscriptions_url": "https://api.github.com/users/octocat/subscriptions",
"organizations_url": "https://api.github.com/users/octocat/orgs",
"repos_url": "https://api.github.com/users/octocat/repos",
"events_url": "https://api.github.com/users/octocat/events{/privacy}",
"received_events_url": "https://api.github.com/users/octocat/received_events",
"type": "User",
"user_view_type": "public",
"site_admin": false,
"name": "The Octocat",
"company": "@github",
"blog": "https://github.blog",
"location": "San Francisco",
"email": null,
"hireable": null,
"bio": null,
"twitter_username": null,
"public_repos": 8,
"public_gists": 8,
"followers": 16728,
"following": 9,
"created_at": "2011-01-25T18:44:36Z",
"updated_at": "2025-01-22T12:21:20Z"
}
如果请求失败(例如由于网络问题或服务器错误),程序将尝试重试最多 3 次,每次重试时,程序会打印当前的重试次数,如果所有重试都失败,最终会抛出异常。假设请求失败了两次,第三次成功,则输出如下:
vbscript
Retry attempt: 1
Retry attempt: 2
Status Code: 200
Response Body: {
"login": "octocat",
"id": 583231,
"node_id": "MDQ6VXNlcjU4MzIzMQ==",
"avatar_url": "https://avatars.githubusercontent.com/u/583231?v=4",
"gravatar_id": "",
"url": "https://api.github.com/users/octocat",
"html_url": "https://github.com/octocat",
"followers_url": "https://api.github.com/users/octocat/followers",
"following_url": "https://api.github.com/users/octocat/following{/other_user}",
"gists_url": "https://api.github.com/users/octocat/gists{/gist_id}",
"starred_url": "https://api.github.com/users/octocat/starred{/owner}{/repo}",
"subscriptions_url": "https://api.github.com/users/octocat/subscriptions",
"organizations_url": "https://api.github.com/users/octocat/orgs",
"repos_url": "https://api.github.com/users/octocat/repos",
"events_url": "https://api.github.com/users/octocat/events{/privacy}",
"received_events_url": "https://api.github.com/users/octocat/received_events",
"type": "User",
"user_view_type": "public",
"site_admin": false,
"name": "The Octocat",
"company": "@github",
"blog": "https://github.blog",
"location": "San Francisco",
"email": null,
"hireable": null,
"bio": null,
"twitter_username": null,
"public_repos": 8,
"public_gists": 8,
"followers": 16728,
"following": 9,
"created_at": "2011-01-25T18:44:36Z",
"updated_at": "2025-01-22T12:21:20Z"
}
总结
HttpClient是Java中一个功能强大且灵活的HTTP客户端库,适用于各种网络通信场景,本文从HttpClient的基础使用入手,逐步深入到实战案例,展示了如何在实际项目中使用HttpClient进行网络请求、处理JSON响应以及实现重试机制。通过掌握HttpClient,开发者可以更加高效地处理网络通信,提升应用的性能和可靠性。