二维码QR码,是有两种实现效果,一种底层的,而另一种就是封装好的。
目录
[QRCodeGenerator 和 QRCodeEncoder 的区别](#QRCodeGenerator 和 QRCodeEncoder 的区别)
[右键=> 引用= > 管理Nuget程序包](#右键=> 引用= > 管理Nuget程序包)
[3、QRCodeGenerator 的思路流程及功能实现](#3、QRCodeGenerator 的思路流程及功能实现)
[4、QRCodeEncoder 的思路流程及功能](#4、QRCodeEncoder 的思路流程及功能)
[7、 二维码图片合并](#7、 二维码图片合并)
先看效果
he
QRCodeGenerator 和 QRCodeEncoder 的区别
1、功能上的区别
- QRCodeGenerator
- 通常是一个基础类,负责生成二维码的核心逻辑
- 专注于将输入数据(文本或URL)转换为二维码的矩阵(二维码的点阵结构)
- 可能不直接生成图像,提供二维码的原始数据结构
- QRCodeEncoder
- 高层次的封装类,基于 QRCodeGenerator 的功能
- 将二维码的矩阵数据进一步处理,将其转换为图像(PNG/JPG等)
- 处理一些额外的逻辑,比如错误校正、编码模式选择等
2、使用场景的区别
- QRCodeGenerator
- 直接操作二维码的矩阵数据,需要用到 QRCodeGenerator
- 适合开发者需要更底层控制的场景
- QRCodeEncoder
- 快速生成二维码图像
- 适合开发者快速完成二维码生成场景
3、实现细节的区别
- QRCodeGenerator
- 是一个基础的类,专注于二维码生成的核心算法
- 可能不处理图像渲染或高级编码逻辑
- QRCodeEncoder
- 一个封装类,调用了 QRCodeGenerator 的功能,并在此基础上添加了额外的处理
- 可能直接返回二维码的图像处理
总结
- QRCodeGenerator:更底层,专注于生成二维码的矩阵数据
- QRCodeEncoder:更高层,封装了二维码生成的完整流程,可能直接生成图像
1.先创建控件
button SaveFileDialog textBox
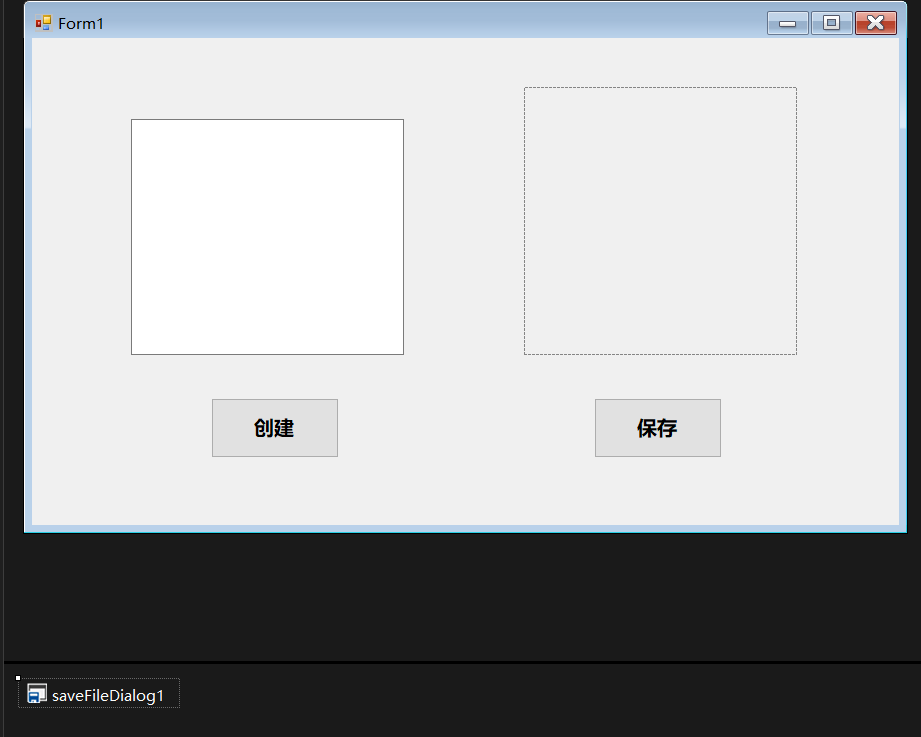
2.添加引用(两个)
右键=> 引用= > 管理Nuget程序包
ThoughtWorks.QRCode ====> QRCodeEncoder
QRCode =====> QRCodeGenerator
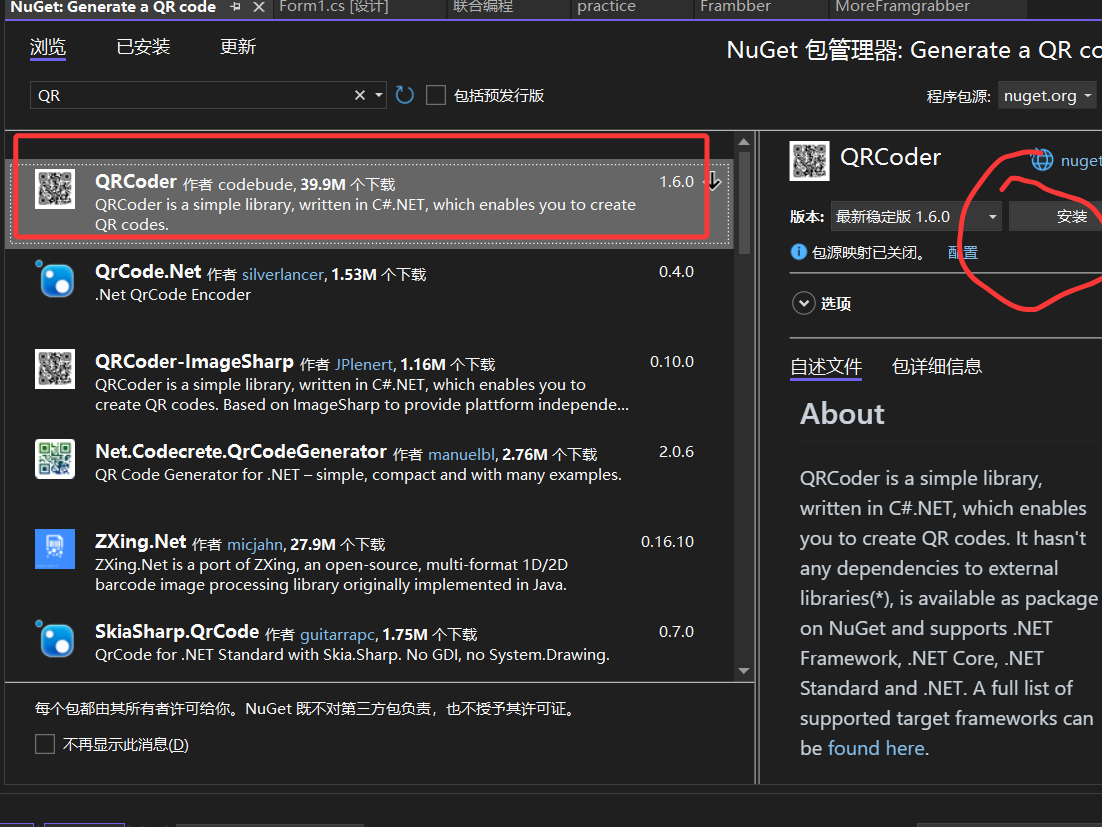
3、QRCodeGenerator 的思路流程及功能实现
- 创建一个二维码生成器
- 用生成器生成二维码数据(设置错误校正级别为 Q)
- 用生成的二维码数据创建一个二维码对象
- 生成二维码图像并保存为 PNG 文件
- 从保存的文件中加载图像
- 将图像显示在 Windows 窗体的 PictureBox 控件中
二维码创建
cs
string s=textBox1.Text.Trim();
if (string.IsNullOrEmpty(s)|| s.Length<5)
{
MessageBox.Show("内容长度不得为空且长度不能小于5个字符");
return;
}
//用来生成二维码的核心工具
QRCodeGenerator codeGenerator = new QRCodeGenerator();
//使用 codeGenerator 生成二维码数据(包含生成二维码所需的数据)
QRCodeData qRCodeData = codeGenerator.CreateQrCode(s, QRCodeGenerator.ECCLevel.Q);
//使用 QRCodeData 对象创建一个 QRCode 对象
QRCode qRCode = new QRCode(qRCodeData);
//创建二维码图像
//参数1:缩放因子 参数2:前景色 参数3:背景色 参数4:是否启用纯色
Bitmap bitmap=qRCode.GetGraphic(15,Color.Black,Color.White,true);
string filepath = @"QRCode" + s.Substring(s.Length - 4, 4) + ".png";
bitmap.Save(filepath);
//Image.FromFile 从文件路径加载图像
Image imagePic=Image.FromFile(filepath);
//将加载的二维码显示在PictureBox控件中
pictureBox1.Image=imagePic;

4、QRCodeEncoder 的思路流程及功能
- 创建二维码编译器对象·
- 设置二维码的背景色和前景色
- 使用编码器将用户输入的文本编码为二维码图像
- 将生成的二维码图像显示在 PictureBox 控件中
二维码创建
cs
string s = textBox1.Text.Trim();
if (string.IsNullOrEmpty(s) || s.Length < 5)
{
MessageBox.Show("内容长度不得为空且长度不能小于5个字符");
return;
}
QRCodeEncoder qr = new QRCodeEncoder();
qr.QRCodeBackgroundColor = Color.White;
qr.QRCodeForegroundColor = Color.Black;
var qrcode = qr.Encode(s, Encoding.Default);
pictureBox1.Image = qrcode;
5、图片保存
cs
saveFileDialog1.Filter = "Jpg 图片|*.jpg|Bmp 图片|*.bmp|Gif 图片|*.gif|Png 图片|*.png|Wmf 图片|*.wmf";
saveFileDialog1.FilterIndex= 0;
if(pictureBox1.Image==null)
{
MessageBox.Show("没有课预览的图片", "提示", MessageBoxButtons.OK, MessageBoxIcon.Error);
}
else
{
if(saveFileDialog1.ShowDialog()== DialogResult.OK)
{
pictureBox1.Image.Save(saveFileDialog1.FileName, System.Drawing.Imaging.ImageFormat.Jpeg);
}
}
6、图像保存集合
给你们封装了一个类,可以根据我之前的教程给他封装成dll,进行引用
cs
using System;
using System.Collections.Generic;
using System.Drawing.Imaging;
using System.Drawing;
using System.IO;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using QRCoder;
namespace Generate_a_QR_code
{
public class QRCoderHelper
{
/// <summary>
/// 创建二维码返回文件路径名称
/// </summary>
/// <param name="plainText">二维码内容</param>
public string CreateQRCodeToFile(string plainText)
{
try
{
string filename = "";
if (string.IsNullOrEmpty(plainText))
{
return null;
}
//二维码文件目录
string filePath = "D://Image//QR";
if (!Directory.Exists(filePath))
{
Directory.CreateDirectory(filePath);
}
//创建二维码文件路径名称
filename = filePath + DateTime.Now.ToString("yyyyMMddHHmmss") + new Random().Next(100, 1000) + ".jpeg";
QRCodeGenerator qRCodeGenerator = new QRCoder.QRCodeGenerator();
//QRCodeGenerator.ECCLevel:纠错能力,Q级:纠错25%的数据码字
QRCodeData qR = qRCodeGenerator.CreateQrCode(plainText, QRCodeGenerator.ECCLevel.Q);
QRCode qRCode = new QRCode(qR);
Bitmap bitmap = qRCode.GetGraphic(15);
bitmap.Save(filename, ImageFormat.Jpeg);
return filename;
}
catch (Exception ex)
{
throw new Exception("Exception in the method of creating a QR code to return a file path name", ex);
}
}
/// <summary>
/// 创建二维码返回byte数组
/// </summary>
/// <param name="plainText">二维码内容</param>
/// <returns></returns>
/// <exception cref="Exception"></exception>
public byte[] CreateQRCodeToBytes(string plainText)
{
try
{
if (string.IsNullOrEmpty(plainText))
{
return null;
}
QRCodeGenerator qRCodeGenerator = new QRCoder.QRCodeGenerator();
//QRCodeGenerator.ECCLevel:纠错能力,Q级:约可纠错25%的数据码字
QRCodeData qRCodeData = qRCodeGenerator.CreateQrCode(plainText, QRCodeGenerator.ECCLevel.Q);
QRCode qRCode = new QRCode(qRCodeData);
Bitmap bitmap = qRCode.GetGraphic(15);
MemoryStream ms = new MemoryStream();
bitmap.Save(ms, ImageFormat.Jpeg);
byte[] arr = new byte[ms.Length];
ms.Position = 0;
ms.Read(arr, 0, (int)ms.Length);
ms.Close();
return arr;
}
catch (Exception ex)
{
throw new Exception("Exception in the method that creates a QR code and returns a byte array", ex);
}
}
/// <summary>
/// 创建二维码 返回 Base64字符串
/// </summary>
/// <param name="plainText">二维码内容</param>
/// <param name="hasEdify"></param>
/// <returns></returns>
/// <exception cref="Exception"></exception>
public string CreateQRCodeToBase64(string plainText, bool hasEdify = true)
{
try
{
string result = "";
if (string.IsNullOrEmpty(plainText))
{
return "";
}
QRCodeGenerator qRCodeGenerator = new QRCoder.QRCodeGenerator();
//QRCodeGenerator.ECCLevel:纠错能力,Q级:约可纠错25%的数据码
QRCodeData qRCodeData = qRCodeGenerator.CreateQrCode(plainText, QRCodeGenerator.ECCLevel.Q);
QRCode qRCode = new QRCode(qRCodeData);
Bitmap bitmap = qRCode.GetGraphic(15);
MemoryStream ms = new MemoryStream();
bitmap.Save(ms, ImageFormat.Jpeg);
byte[] arr = new byte[ms.Length];
ms.Position = 0;
ms.Read(arr, 0, (int)ms.Length);
ms.Close();
if (hasEdify)
{
result = "data:image/jpeg;base64" + Convert.ToBase64String(arr);
}
else
{
result = Convert.ToBase64String(arr);
}
return result;
}
catch (Exception ex)
{
throw new Exception("create a QR code to return a Base64 string method exception", ex);
}
}
}
}
cs
QRCoderHelper qrHelper = new QRCoderHelper();
string s = "https://mpbeta.csdn.net/mp_blog/creation/editor/147076940?spm=1000.2115.3001.4503";
string filename=qrHelper.CreateQRCodeToFile(s);
7、 二维码图片合并
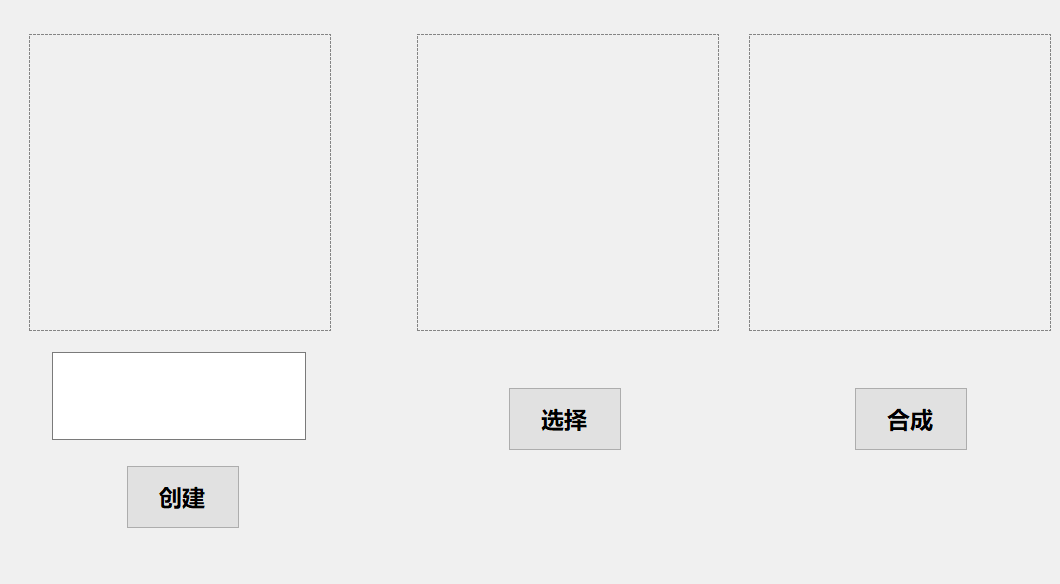
8、封装(代码)
cs
Bitmap Merge_images(Image p1, Image p2)
{
Bitmap bitmap = new Bitmap(p1.Width, p1.Height);
Graphics g = Graphics.FromImage(bitmap);
g.DrawImage(p1, 0, 0, p1.Width, p2.Height);
g.DrawImage(p2, (float)0.375 * p1.Width, (float)0.375 * p1.Height, (float)0.25 * p1.Width, (float)0.25 * p1.Height);
return bitmap;
}
cs
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
using ThoughtWorks.QRCode.Codec;
namespace Generate_a_QR_code
{
public partial class Form2 : Form
{
public Form2()
{
InitializeComponent();
}
private void button1_Click(object sender, EventArgs e)
{
QRCodeEncoder qr = new QRCodeEncoder();
qr.QRCodeBackgroundColor = Color.White;
qr.QRCodeForegroundColor = Color.Black;
var qrcode = qr.Encode(textBox1.Text, Encoding.Default);
pictureBox1.Image = qrcode;
}
private void button2_Click(object sender, EventArgs e)
{
OpenFileDialog openFileDialog = new OpenFileDialog();
if (openFileDialog.ShowDialog() == DialogResult.OK)
{
pictureBox2.Image = Image.FromFile(openFileDialog.FileName);
}
}
private void button3_Click(object sender, EventArgs e)
{
pictureBox3.Image = Merge_images(pictureBox1.Image, pictureBox2.Image);
}
Bitmap Merge_images(Image p1, Image p2)
{
Bitmap bitmap = new Bitmap(p1.Width, p1.Height);
Graphics g = Graphics.FromImage(bitmap);
g.DrawImage(p1, 0, 0, p1.Width, p2.Height);
g.DrawImage(p2, (float)0.375 * p1.Width, (float)0.375 * p1.Height, (float)0.25 * p1.Width, (float)0.25 * p1.Height);
return bitmap;
}
}
}
9、结果显示
