上一篇
【STL 之速通pair vector list stack queue set map 】
queue
note
priority_queue pq;
使用的还是很方便的
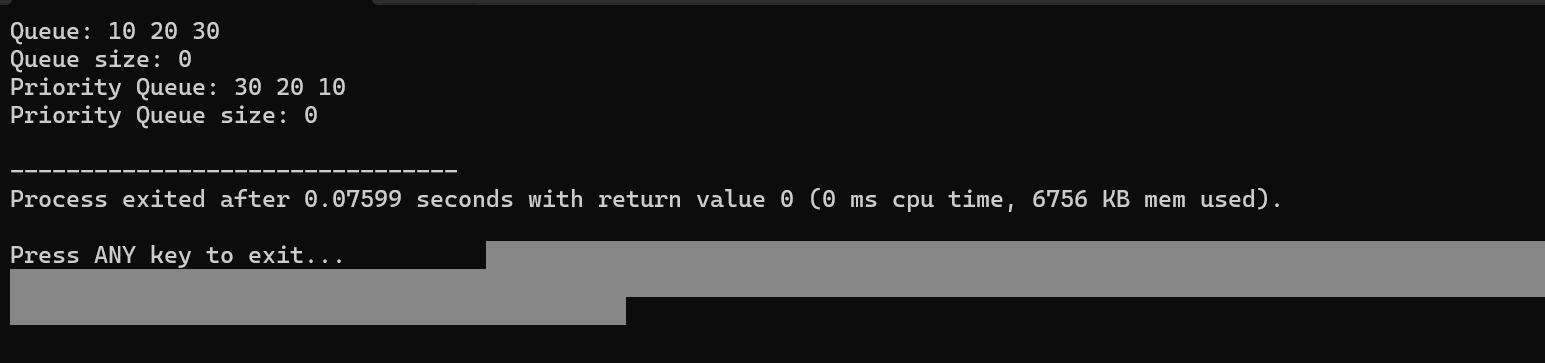
c
#include <iostream>
#include <queue>
using namespace std;
int main() {
// Queue 示例
queue<int> q;
q.push(10);
q.push(20);
q.push(30);
cout << "Queue: ";
while (!q.empty()) {
cout << q.front() << " ";
q.pop();
}
cout << endl;
// 使用 size() 方法
cout << "Queue size: " << q.size() << endl;
// Priority Queue 示例
priority_queue<int> pq;
pq.push(10);
pq.push(20);
pq.push(30);
cout << "Priority Queue: ";
while (!pq.empty()) {
cout << pq.top() << " ";
pq.pop();
}
cout << endl;
// 使用 size() 方法
cout << "Priority Queue size: " << pq.size() << endl;
return 0;
}
set
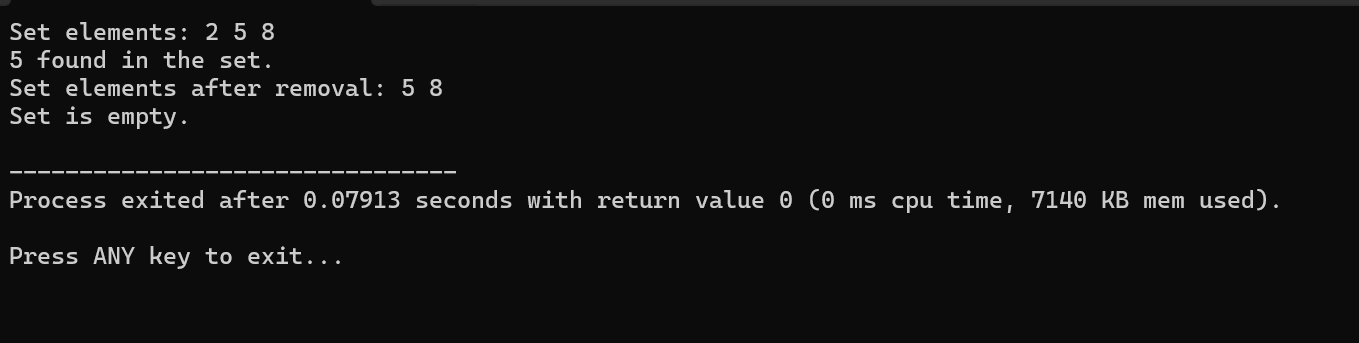
c
#include <iostream>
#include <set>
using namespace std;
int main() {
set<int> mySet;
// 插入元素
mySet.insert(5);
mySet.insert(2);
mySet.insert(8);
mySet.insert(2); // 重复元素
// 遍历集合
cout << "Set elements: ";
for (const auto& elem : mySet) {
cout << elem << " ";
}
cout << endl;
// 查找元素
int searchValue = 5;
auto it = mySet.find(searchValue);
if (it != mySet.end()) {
cout << searchValue << " found in the set." << endl;
} else {
cout << searchValue << " not found in the set." << endl;
}
// 移除元素
int removeValue = 2;
mySet.erase(removeValue);
// 再次遍历集合
std::cout << "Set elements after removal: ";
for (const auto& elem : mySet) {
std::cout << elem << " ";
}
std::cout << std::endl;
// 清空集合
mySet.clear();
// 检查集合是否为空
if (mySet.empty()) {
std::cout << "Set is empty." << std::endl;
} else
std::cout << "Set is not empty." << std::endl;
return 0;
}
map
count(key)方法 大多只用来判断是否存在某个键-key
c
#include <iostream>
#include <map>
#include <string> // 添加string头文件
using namespace std;
int main() {
// 创建并初始化map
map<int, string> myMap = { {1, "Apple"}, {2, "Banana"}, {3, "Orange"} };
// 插入元素
myMap.insert(make_pair(4, "Grapes"));
// 查找和访问元素
cout << "Value at key 2: " << myMap[2] << endl;
// 遍历并打印map中的元素
for (const auto& pair : myMap) {
cout << "Key: " << pair.first << ", Value: " << pair.second << endl;
}
// 删除元素
myMap.erase(3);
// 判断元素是否存在
if (myMap.count(3) == 0) {
cout << "Key 3 not found." << endl;
}
else cout << "Key 3 is found." << endl;
// 清空map
myMap.clear();
// 判断map是否为空
if (myMap.empty()) {
cout << "Map is empty." << endl;
}
return 0; // 表示程序正常结束
}
还有
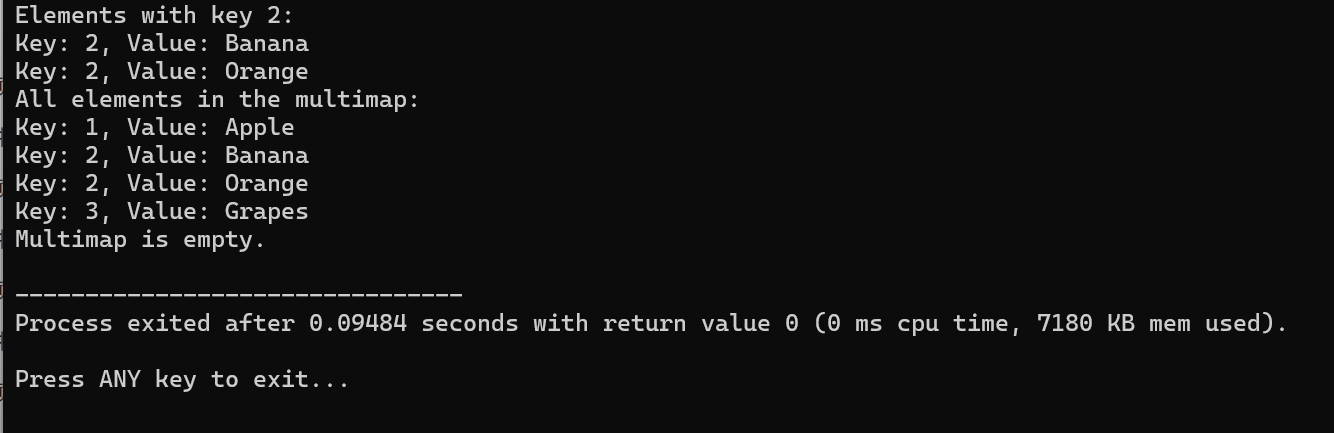
c
#include <iostream>
#include <map>
#include <string> // 添加string头文件
using namespace std;
int main() {
// 创建并初始化multimap
multimap<int, string> myMultimap = {
{1, "Apple"},
{2, "Banana"},
{2, "Orange"}
};
// 插入一个新元素
myMultimap.insert(make_pair(3, "Grapes"));
// 查找并访问键为2的元素,使用equal_range获取范围,并通过循环输出键值对
auto range = myMultimap.equal_range(2);
cout << "Elements with key 2:" << endl;
for (auto it = range.first; it != range.second; ++it) {
cout << "Key: " << it->first << ", Value: " << it->second << endl;
}
// 遍历并打印multimap中的所有元素
cout << "All elements in the multimap:" << endl;
for (const auto& pair : myMultimap) {
cout << "Key: " << pair.first << ", Value: " << pair.second << endl;
}
// 删除一个元素(例如删除键为2的一个元素)
auto it = myMultimap.find(2);
if (it != myMultimap.end()) {
myMultimap.erase(it);
}
// 判断元素是否存在
if (myMultimap.count(2) == 0) {
cout << "Key 2 not found." << endl;
}
// 清空multimap
myMultimap.clear();
// 判断multimap是否为空
if (myMultimap.empty()) {
cout << "Multimap is empty." << endl;
}
return 0; // 返回0表示程序正常结束
}
--->>完结撒花