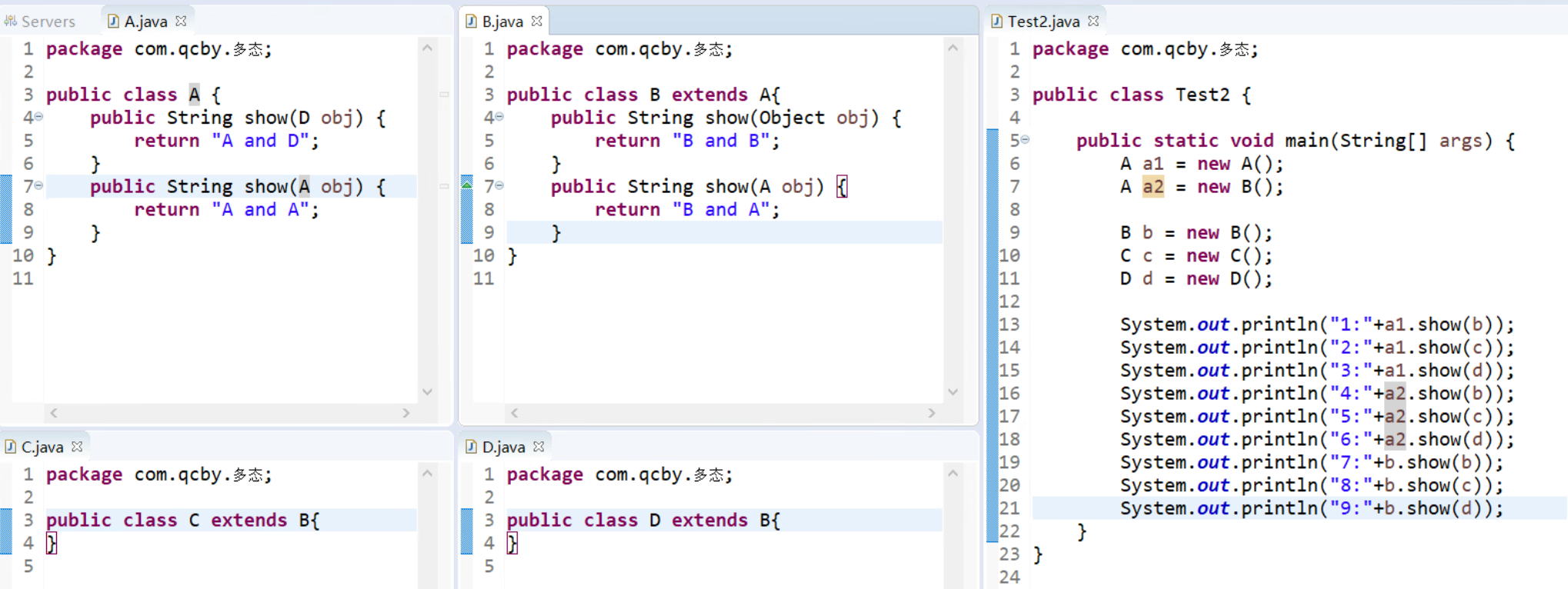
1:A and A
2:A and A
3: A and D
4: B and A
5: B and A
6:A and D
7:B and A
8: B and A
9:A and D
java
package 第四章对象和类;
public class ForthThir {
//多态:父类的引用指向子类的对象,只能调父类的方法和子类重写的方法,不能调子类独有的方法;子类则可以调父类独有的方法
//条件:必须继承关系、必须要重写,父类的引用指向子类对象
/*
* public class Parent{
* public void eat(){System.out.println("Parent"); }
* }
*
* public class Child1 extends Parent{
* public void eat(){System.out.println("Child1");}
* }
*
* public class Child2 extends Parent{
* public void eat(){System.out.println("Child2");}
* }
*
* public class Grand1 extends Child1{
* public void eat(){System.out.println("Grand1");}
* }
*
*
* public class Test{
* public static void main(String[] args){
* Parent xx=new Parent();
* xx.eat();
* xx=new Child1();
* xx.eat();//执行Child1里的方法
*
*
* aa(new Parent());
* aa(new Child1());
* }
* public static void aa(Parent xx){
* xx.eat();
* }
///强制类型转换 大碗不能自动转成小碗
* Parent xx =new Grand1();
* Child1 yy=(Child1)xx;
* Child2 yy=(Child2)xx;//运行报错,xx内核为Grand类型数据
*
* 引用类型到底能不能转,做判断:
* instanceof 判断xx是否位Child2类型的对象,或者Child2子孙后代的对象
* if(xx instanceof Child2){
* Child2 yy=(Child2)xx;
*
* }
*
*
* int a=32;
* short b=(short)a;
*
* int[] arr={100,2457,120,22};
* for(int i=0;i<arr.length;i++){
* char j =(char)arr[i];
* System.out.println(j);
* }
* }
*/
//基本数据类型
/*
* int a=10;
* Integer b=10;
*
* int Integer String
*
* int->Integer new Integer():
* int a=10;
* Integer a_p=new Integer(a);
*
* Integer->int intValue()
* Integer a_p=new Integer(4);
* int a=a_p.intValue();
*
* Integer->String toString();
* Integer a_p=new Integer(4);
*
*
*
* String->/integer:new Integer()
* String a="888";
* Integer b=new Integer(a)
*
* int->String String.valueOf()
* int a=10;
* String b=String.valueOf(a);
*
* String->int Integer.parseInt()
* String a="999";
* int b=Integer.parseInt(a);
*
*/
//装箱:基本类型->包装类
/*
* int a=10;
* Integer i=Integer.valueOf(a);
* 或Integer j=new Integer(a);
*/
//拆箱 包装类->基本类型
/*
* Integer k=new Integer(10);
* int b=k.intValue();
*/
//自动装箱 直接把一个int类型的数据直接赋值到Integer(java 5之后)
/*
* int c=20;
* Integer p_auto=a;
* 或 Integer m=100;
*/
//自动拆箱(把一个包装类型的数据变成基本类型数据)
/*
* Integer l=new Integer(10);
* int d_auto=l;
*/
//128陷阱
/*
* Integer num1=100;/=150 调的是valueOf方法,避免频繁创建
* Integer num2=100;/=150
* System.out.println(num1==num2);true/false 超过128就是false
* Integer num3=new Integer(100);
* Integer num4=new Integer(100);
* System.out.println(num3==num4);false
*
*
*/
}
java
package com.qc.Object;
public class Test {
public static void main(String[] args) {
Object aa=new Object();
aa.equals(obj);
aa.hashCode();
//equals()比较内存地址指向是否相同
//getClass()获取类的信息 反射邻域
//hashCode()获取散列码 根据地址生成
//哈希表:数组+链表结构
String a="ok";
String b=new String("ok");
Person x1=new Person();
Person x2=new Person();
System.out.println(x1.hashCode());
//x1,x2的hashCode不同;a,b的hashCode相同,因为String重写了方法
//(Objects里)equals判断是否为同一个对象;hashCode判断是否是同一类 大部分类在重写equals方法时会重写hashCode方法
//如果两个对象相同(equals方法返回true),那么他们的hashCode值一定相同
//如果两个对象不同(equals方法返回false),他们的hashCode值可能相同 可能不同
//如果两个对象hashCode值相同(存在哈希冲突),他们可能相同可能不同(equals方法可能返回false,可能返回true)
//如果两个对象hashCode值不同,那么他们两个肯定不同(equals方法返回false)
//面试题1:两个不同的对象,hashCode有没有可能相同? 可能,有极小概率
//面试题2:重写equals方法为什么要重写hashCode方法?一定要重写吗?
//跟hashmap 如果不使用hashmap则不需要重写hashCode;equals和hashCode在hashmap里是配合使用的
//hashmap比较key值的时候使用equals方法比较是否重复,存入values时要使用hashCode确定位置
//访问权限修饰符(修饰变量/方法) 当前类 同包 子类 跨包 其他包
//public ✔ ✔ ✔ ✔
//protected ✔ ✔ ✔ ✖
//默认 不写 ✔ ✔ ✖ ✖
//private ✖ ✖ ✖ ✖
//wait() 线程进入等待状态 让出CPU和锁
//notify() 唤醒等待的线程
//toString() 输出对象的类型和内存地址
//hashCode()
}
}
"128陷阱"是Java中关于整数缓存机制的一个经典问题,主要涉及Integer
类的缓存行为和自动装箱/拆箱机制。
-
Integer缓存机制:
-
Java对
-128
到127
之间的Integer
对象进行了缓存 -
当使用自动装箱或
Integer.valueOf()
方法时,会优先从缓存中获取对象
-
底层原理
-
IntegerCache类:
-
Java在
Integer
类内部维护了一个静态的IntegerCache
类 -
默认缓存了-128到127之间的Integer对象
-
-
自动装箱行为:
-
当使用
Integer i = 100
这样的语法时,实际调用的是Integer.valueOf(100)
-
valueOf()
方法会先检查是否在缓存范围内
-
目的:
- 减少对象创建:对于常用的小整数(-128~127),通过缓存复用对象,避免重复创建