文章目录
-
- 一、基础实现方案
-
- [1. 使用 WindowManager 实现全局悬浮窗](#1. 使用 WindowManager 实现全局悬浮窗)
- [2. 布局文件 (res/layout/floating_button.xml)](#2. 布局文件 (res/layout/floating_button.xml))
- [3. 圆形背景 (res/drawable/circle_background.xml)](#3. 圆形背景 (res/drawable/circle_background.xml))
- [4. 启动服务](#4. 启动服务)
- 二、权限处理
-
- [1. AndroidManifest.xml 中添加权限](#1. AndroidManifest.xml 中添加权限)
- [2. 检查并请求权限](#2. 检查并请求权限)
- 三、高级功能扩展
-
- [1. 添加动画效果](#1. 添加动画效果)
- [2. 自动吸附边缘](#2. 自动吸附边缘)
- [3. 显示/隐藏动画](#3. 显示/隐藏动画)
- 四、优化建议
- 五、替代方案
-
- [1. 使用 CoordinatorLayout + FloatingActionButton](#1. 使用 CoordinatorLayout + FloatingActionButton)
- [2. 使用第三方库](#2. 使用第三方库)
- 六、常见问题解决
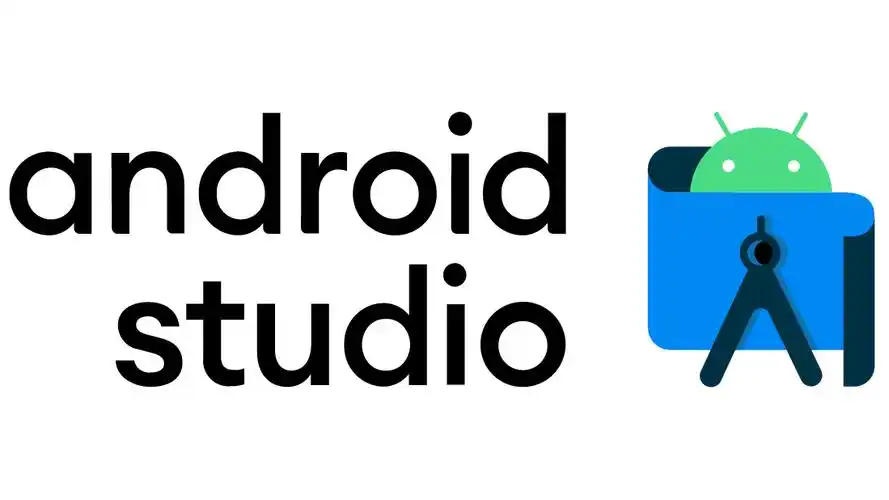
在 Android 应用中实现全局悬浮按钮是一个常见的需求,可以用于快速访问重要功能或返回顶部等操作。下面我将详细介绍如何实现一个自定义的全局悬浮按钮。
一、基础实现方案
1. 使用 WindowManager 实现全局悬浮窗
这是最灵活的实现方式,可以在任何界面显示悬浮按钮:
java
public class FloatingButtonService extends Service {
private WindowManager windowManager;
private View floatingButton;
@Override
public IBinder onBind(Intent intent) {
return null;
}
@Override
public void onCreate() {
super.onCreate();
// 创建悬浮按钮视图
floatingButton = LayoutInflater.from(this).inflate(R.layout.floating_button, null);
// 设置按钮点击事件
floatingButton.findViewById(R.id.float_button).setOnClickListener(v -> {
// 处理点击事件
Toast.makeText(this, "悬浮按钮被点击", Toast.LENGTH_SHORT).show();
});
// 设置窗口参数
WindowManager.LayoutParams params = new WindowManager.LayoutParams(
WindowManager.LayoutParams.WRAP_CONTENT,
WindowManager.LayoutParams.WRAP_CONTENT,
Build.VERSION.SDK_INT >= Build.VERSION_CODES.O ?
WindowManager.LayoutParams.TYPE_APPLICATION_OVERLAY :
WindowManager.LayoutParams.TYPE_PHONE,
WindowManager.LayoutParams.FLAG_NOT_FOCUSABLE,
PixelFormat.TRANSLUCENT);
// 设置初始位置
params.gravity = Gravity.TOP | Gravity.START;
params.x = 0;
params.y = 100;
// 获取WindowManager并添加视图
windowManager = (WindowManager) getSystemService(WINDOW_SERVICE);
windowManager.addView(floatingButton, params);
// 添加拖拽功能
addDragFeature();
}
private void addDragFeature() {
floatingButton.setOnTouchListener(new View.OnTouchListener() {
private int initialX;
private int initialY;
private float initialTouchX;
private float initialTouchY;
@Override
public boolean onTouch(View v, MotionEvent event) {
switch (event.getAction()) {
case MotionEvent.ACTION_DOWN:
initialX = params.x;
initialY = params.y;
initialTouchX = event.getRawX();
initialTouchY = event.getRawY();
return true;
case MotionEvent.ACTION_MOVE:
params.x = initialX + (int) (event.getRawX() - initialTouchX);
params.y = initialY + (int) (event.getRawY() - initialTouchY);
windowManager.updateViewLayout(floatingButton, params);
return true;
}
return false;
}
});
}
@Override
public void onDestroy() {
super.onDestroy();
if (floatingButton != null) {
windowManager.removeView(floatingButton);
}
}
}
2. 布局文件 (res/layout/floating_button.xml)
xml
<?xml version="1.0" encoding="utf-8"?>
<FrameLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="wrap_content"
android:layout_height="wrap_content">
<ImageButton
android:id="@+id/float_button"
android:layout_width="56dp"
android:layout_height="56dp"
android:background="@drawable/circle_background"
android:src="@drawable/ic_float_button"
android:elevation="8dp"
android:layout_margin="16dp" />
</FrameLayout>
3. 圆形背景 (res/drawable/circle_background.xml)
xml
<?xml version="1.0" encoding="utf-8"?>
<shape xmlns:android="http://schemas.android.com/apk/res/android"
android:shape="oval">
<solid android:color="@color/colorPrimary" />
</shape>
4. 启动服务
java
// 在需要显示悬浮按钮的地方启动服务
startService(new Intent(context, FloatingButtonService.class));
// 停止服务
stopService(new Intent(context, FloatingButtonService.class));
二、权限处理
1. AndroidManifest.xml 中添加权限
xml
<uses-permission android:name="android.permission.SYSTEM_ALERT_WINDOW" />
2. 检查并请求权限
java
// 检查悬浮窗权限
if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.M) {
if (!Settings.canDrawOverlays(this)) {
Intent intent = new Intent(Settings.ACTION_MANAGE_OVERLAY_PERMISSION,
Uri.parse("package:" + getPackageName()));
startActivityForResult(intent, OVERLAY_PERMISSION_REQ);
} else {
// 已经有权限,启动服务
startService(new Intent(this, FloatingButtonService.class));
}
} else {
// 6.0以下直接启动
startService(new Intent(this, FloatingButtonService.class));
}
三、高级功能扩展
1. 添加动画效果
java
// 在按钮点击时添加动画
floatingButton.setOnClickListener(v -> {
// 缩放动画
ObjectAnimator scaleX = ObjectAnimator.ofFloat(v, "scaleX", 1f, 0.8f, 1f);
ObjectAnimator scaleY = ObjectAnimator.ofFloat(v, "scaleY", 1f, 0.8f, 1f);
AnimatorSet animatorSet = new AnimatorSet();
animatorSet.playTogether(scaleX, scaleY);
animatorSet.setDuration(200);
animatorSet.start();
// 执行点击操作
performButtonAction();
});
2. 自动吸附边缘
java
private void autoAttachToEdge() {
int screenWidth = getResources().getDisplayMetrics().widthPixels;
int buttonWidth = floatingButton.getWidth();
if (params.x < screenWidth / 2 - buttonWidth / 2) {
// 吸附到左边
params.x = 0;
} else {
// 吸附到右边
params.x = screenWidth - buttonWidth;
}
windowManager.updateViewLayout(floatingButton, params);
}
3. 显示/隐藏动画
java
public void hideButton() {
floatingButton.animate()
.translationY(floatingButton.getHeight())
.setDuration(300)
.start();
}
public void showButton() {
floatingButton.animate()
.translationY(0)
.setDuration(300)
.start();
}
四、优化建议
-
性能优化:
- 使用轻量级的视图层级
- 避免频繁调用
updateViewLayout
- 使用硬件加速
-
内存管理:
- 在不需要时及时移除悬浮窗
- 在低内存时自动隐藏
-
用户体验:
- 添加适当的触摸反馈
- 考虑屏幕旋转时的位置调整
- 提供设置选项让用户自定义位置和行为
-
兼容性处理:
- 处理不同 Android 版本的权限差异
- 适配各种屏幕尺寸和密度
- 考虑全面屏和刘海屏的适配
五、替代方案
1. 使用 CoordinatorLayout + FloatingActionButton
如果只需要在应用内显示悬浮按钮,可以使用 Material Design 组件:
xml
<androidx.coordinatorlayout.widget.CoordinatorLayout
android:layout_width="match_parent"
android:layout_height="match_parent">
<!-- 其他内容 -->
<com.google.android.material.floatingactionbutton.FloatingActionButton
android:id="@+id/fab"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="bottom|end"
android:layout_margin="16dp"
android:src="@drawable/ic_add" />
</androidx.coordinatorlayout.widget.CoordinatorLayout>
2. 使用第三方库
一些流行的悬浮按钮库:
六、常见问题解决
-
权限问题:
- 确保已正确请求
SYSTEM_ALERT_WINDOW
权限 - 在 Android 6.0+ 上需要动态请求权限
- 某些厂商 ROM 可能需要额外的白名单设置
- 确保已正确请求
-
位置不正确:
- 检查
WindowManager.LayoutParams
的 gravity 设置 - 考虑状态栏和导航栏的高度
- 在屏幕旋转时更新位置
- 检查
-
点击穿透:
- 设置
FLAG_NOT_FOCUSABLE
可能导致点击事件穿透 - 可以通过在
onTouch
中返回true
来拦截事件
- 设置
-
内存泄漏:
- 确保在服务销毁时移除视图
- 避免在视图中持有 Activity 引用