1.Mysql数据表users如下:
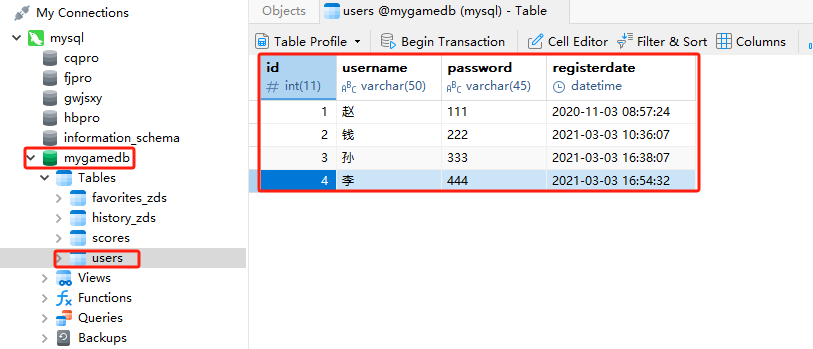
2.即将导入的Excel表格如下:
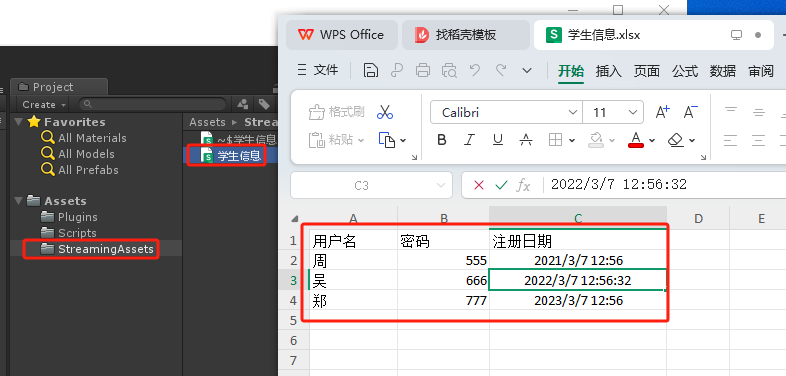
3.代码如下:
cs
using System;
using System.Data;
using System.IO;
using Excel;
using MySql.Data.MySqlClient;
using UnityEngine;
using UnityEditor;
public class ImportExcel
{
// 数据库连接字符串,需根据实际情况修改
private const string ConnectionString = "server=localhost;database=mygamedb;uid=root;pwd=123456;";
[MenuItem("Excel操作/导入Excel数据到MySQL")]
static void ImportExcelToMySQL()
{
string excelPath = Application.streamingAssetsPath + "/学生信息.xlsx";
try
{
// 读取Excel数据
DataRowCollection dataRows = ReadExcel(excelPath);
if (dataRows != null && dataRows.Count > 0)
{
// 连接MySQL数据库并插入数据
InsertDataToMySQL(dataRows);
}
}
catch (Exception e)
{
Debug.LogError("导入数据时出错:" + e.Message);
}
}
private static DataRowCollection ReadExcel(string path)
{
try
{
using (FileStream stream = File.Open(path, FileMode.Open, FileAccess.Read))
{
IExcelDataReader excelReader = ExcelReaderFactory.CreateOpenXmlReader(stream);
DataSet result = excelReader.AsDataSet();
return result.Tables[0].Rows;
}
}
catch (Exception e)
{
Debug.LogError("读取Excel文件时出错:" + e.Message);
return null;
}
}
private static void InsertDataToMySQL(DataRowCollection dataRows)
{
using (MySqlConnection connection = new MySqlConnection(ConnectionString))
{
try
{
connection.Open();
// 查询当前最大的id值
string str_总数据条数 = "SELECT COUNT(*) AS total_records FROM users";
MySqlCommand maxIdCommand = new MySqlCommand(str_总数据条数, connection);
object obj_总数据条数 = maxIdCommand.ExecuteScalar();
Debug.Log(Convert.ToInt32(obj_总数据条数));
// 插入语句包含id字段,数据库自增长会自动赋值
string insertQuery = "INSERT INTO users (id, username, password, registerdate) VALUES (@id, @username, @password, @registerdate)";
using (MySqlCommand command = new MySqlCommand(insertQuery, connection))
{
// 从第二行开始插入数据,跳过标题行
for (int i = 1; i < dataRows.Count; i++)
{
DataRow row = dataRows[i];
command.Parameters.Clear();
command.Parameters.AddWithValue("@id", Convert.ToInt32(obj_总数据条数)+i);
command.Parameters.AddWithValue("@username", row[0]);
command.Parameters.AddWithValue("@password", row[1]);
// 处理日期格式,假设Excel中的日期格式可直接转换
DateTime registerDate;
if (DateTime.TryParse(row[2].ToString(), out registerDate))
{
command.Parameters.AddWithValue("@registerdate", registerDate);
}
else
{
Debug.LogWarning("无法解析日期:" + row[2] + ",使用默认值");
command.Parameters.AddWithValue("@registerdate", DateTime.Now);
}
command.ExecuteNonQuery();
}
}
Debug.Log("数据成功导入到MySQL数据库");
}
catch (Exception e)
{
Debug.LogError("插入数据到MySQL时出错:" + e.Message);
}
}
}
}