1.react生命周期(旧版)
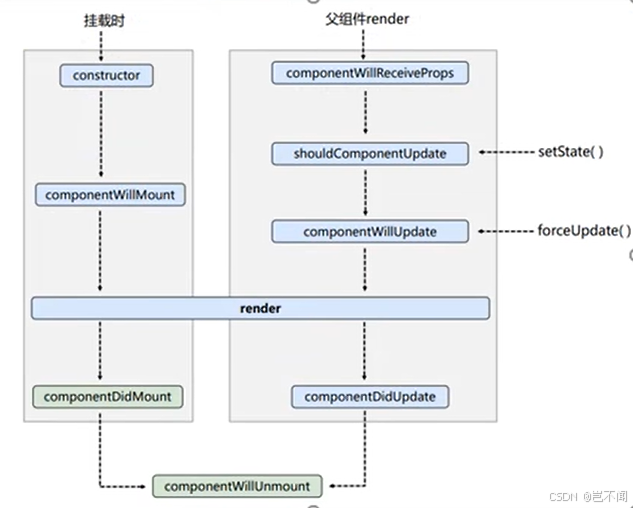
1.1react初始挂载时的生命周期
1:构造器-constructor
javascript
// 构造器
constructor(props) {
console.log('1:构造器-constructor');
super(props)
// 初始化状态
this.state = {count: 0}
}
2:组件将要挂载-componentWillMount
javascript
// 组件将要挂载
componentWillMount() {
console.log('2:组件将要挂载-componentWillMount');
}
3:开始渲染-render
javascript
render(){
console.log('3:开始渲染-render');
const {count} = this.state
return(
<div>
<h2>当前的和为{count}</h2>
<button onClick={this.add}>点我+1</button>
<button onClick={this.death}>卸载DOM</button>
</div>
)
}
4:组件挂载完成-componentDidMount
javascript
// 组件挂载完成
componentDidMount() {
console.log('4:组件挂载完成-componentDidMount');
}
5:组件卸载-componentWillUnmount
javascript
// 组件卸载
componentWillUnmount() {
console.log('5:组件卸载-componentWillUnmount');
}
代码实现演示
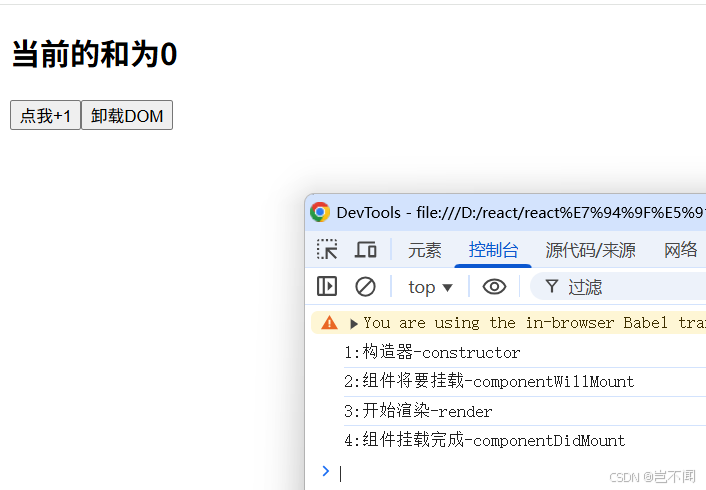
点击卸载dom后
整体代码
html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Hello,React生命周期(旧)</title>
</head>
<body>
<!-- 容器 -->
<div id="test"></div>
<!-- {/* // 引入 React核心库 */} -->
<script src="https://unpkg.com/react@16/umd/react.production.min.js"></script>
<!-- {/* // 引入 react-dom 用于支持 react 操作 DOM */} -->
<script src="https://unpkg.com/react-dom@16/umd/react-dom.production.min.js"></script>
<!-- {/* // 引入 babel:1. ES6 ==> ES5 2. jsx ==> js */} -->
<script src="https://unpkg.com/babel-standalone@6/babel.min.js"></script>
<!-- {/* // 引入 JSX 语法 */} -->
<script type="text/babel">
// 1. 创建组件
class Count extends React.Component {
// 构造器
constructor(props) {
console.log('1:构造器-constructor');
super(props)
// 初始化状态
this.state = {count: 0}
}
add=()=>{
// 获取原状态
const {count} = this.state
// 状态更新
this.setState({
count: count+1
})
}
death=()=>{
ReactDOM.unmountComponentAtNode(document.getElementById('test'))
}
// 组件将要挂载
componentWillMount() {
console.log('2:组件将要挂载-componentWillMount');
}
// 组件挂载完成
componentDidMount() {
console.log('4:组件挂载完成-componentDidMount');
}
// 组件卸载
componentWillUnmount() {
console.log('5:组件卸载-componentWillUnmount');
}
render(){
console.log('3:开始渲染-render');
const {count} = this.state
return(
<div>
<h2>当前的和为{count}</h2>
<button onClick={this.add}>点我+1</button>
<button onClick={this.death}>卸载DOM</button>
</div>
)
}
}
ReactDOM.render(<Count />,document.getElementById('test'))
</script>
</body>
</html>
1.2.react更新数据setState
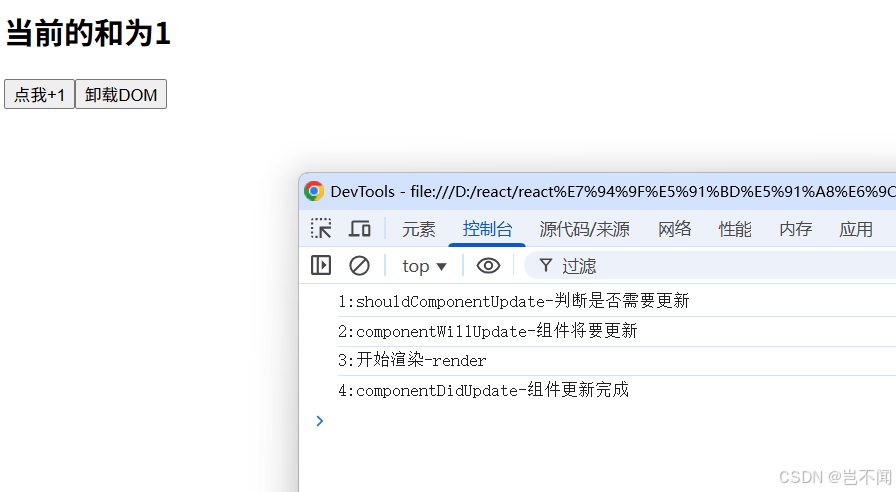
1:shouldComponentUpdate-判断是否需要更新
javascript
// 控制组件更新 默认不写此钩子函数 返回true 组件更新
shouldComponentUpdate(nextProps, nextState) {
console.log('1:shouldComponentUpdate-判断是否需要更新');
return true
}
2:componentWillUpdate-组件将要更新
javascript
// 组件将要更新
componentWillUpdate(nextProps, nextState) {
console.log('2:componentWillUpdate-组件将要更新');
}
3:开始渲染-render
javascript
render(){
console.log('3:开始渲染-render');
const {count} = this.state
return(
<div>
<h2>当前的和为{count}</h2>
<button onClick={this.add}>点我+1</button>
<button onClick={this.death}>卸载DOM</button>
</div>
)
}
4:componentDidUpdate-组件更新完成
javascript
// 组件更新完成
componentDidUpdate(prevProps, prevState) {
console.log('4:componentDidUpdate-组件更新完成');
}
5:组件卸载-componentWillUnmount
javascript
// 组件卸载
componentWillUnmount() {
console.log('5:组件卸载-componentWillUnmount');
}
整体代码
html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Hello,React生命周期(旧)</title>
</head>
<body>
<!-- 容器 -->
<div id="test"></div>
<!-- {/* // 引入 React核心库 */} -->
<script src="https://unpkg.com/react@16/umd/react.production.min.js"></script>
<!-- {/* // 引入 react-dom 用于支持 react 操作 DOM */} -->
<script src="https://unpkg.com/react-dom@16/umd/react-dom.production.min.js"></script>
<!-- {/* // 引入 babel:1. ES6 ==> ES5 2. jsx ==> js */} -->
<script src="https://unpkg.com/babel-standalone@6/babel.min.js"></script>
<!-- {/* // 引入 JSX 语法 */} -->
<script type="text/babel">
// 1. 创建组件
class Count extends React.Component {
state = {count: 0}
add=()=>{
// 获取原状态
const {count} = this.state
// 状态更新
this.setState({
count: count+1
})
}
death=()=>{
ReactDOM.unmountComponentAtNode(document.getElementById('test'))
}
// 组件卸载
componentWillUnmount() {
console.log('5:组件卸载-componentWillUnmount');
}
// 控制组件更新 默认不写此钩子函数 返回true 组件更新
shouldComponentUpdate(nextProps, nextState) {
console.log('1:shouldComponentUpdate-判断是否需要更新');
return true
}
// 组件将要更新
componentWillUpdate(nextProps, nextState) {
console.log('2:componentWillUpdate-组件将要更新');
}
// 组件更新完成
componentDidUpdate(prevProps, prevState) {
console.log('4:componentDidUpdate-组件更新完成');
}
render(){
console.log('3:开始渲染-render');
const {count} = this.state
return(
<div>
<h2>当前的和为{count}</h2>
<button onClick={this.add}>点我+1</button>
<button onClick={this.death}>卸载DOM</button>
</div>
)
}
}
ReactDOM.render(<Count />,document.getElementById('test'))
</script>
</body>
</html>
1.3.forceUpdate声明周期函数强制刷新
forceUpdate--》componentWillUpdate--》render--》componentDidUpdate--》componentWillUnmount
1.4父组件调用子组件render生命周期
componentWillReceiveProps--生命周期钩子函数---父组件调用子组件 第二次渲染,其他的调用子组件的生命周期如setState以后执行生命周期一致。
父组件A
javascript
// 创建组件A--父组件
class A extends React.Component {
// 初始化状态
state={carName:'奥迪'}
// 事件
changeCarName=()=>{
this.setState({
carName: '宝马'
})
}
render() {
return (
<div>
<div>A组件</div>
<button onClick={this.changeCarName}>修改车名</button>
{/*将修改的车名传递给子组件--B*/}
<B carName={this.state.carName} />
</div>
)
}
}
子组件B
javascript
// 创建组件B--子组件
class B extends React.Component {
// 生命周期钩子函数---父组件调用子组件 第二次渲染
componentWillReceiveProps() {
console.log('b--componentWillReceiveProps');
}
render() {
return (
// B--props 接收父组件传递的props
<div>B组件,接收父组件传递的props:{this.props.carName}</div>
)
}
}
1.5 旧版生命周期总结
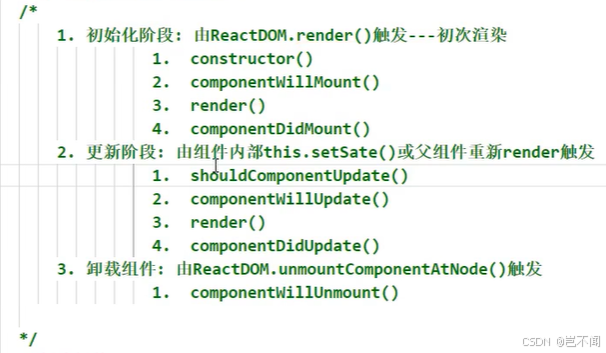
基础开发中常用的生命周期钩子:
- componentDidMount:初始化做的事情,开启定时器,发送网络请求,订阅消息。
- render:渲染结构。
- componentWillUnmount:收尾,关闭定时器,取消消息。
2.react生命周期(新版17)
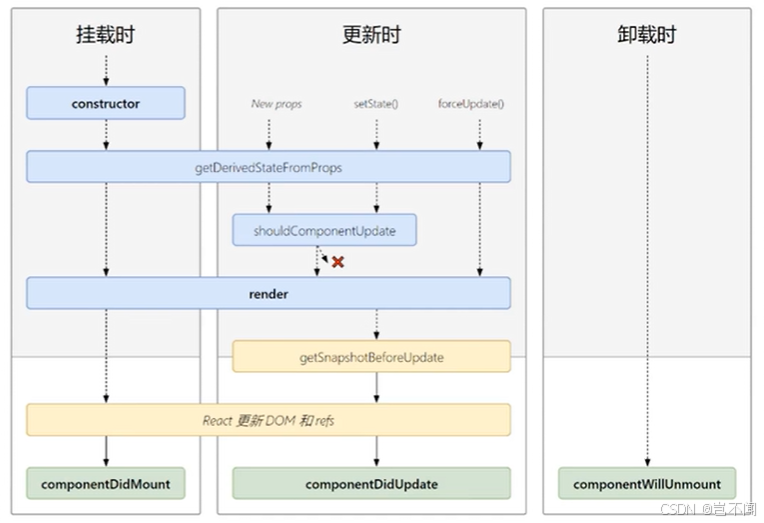
componentWillMount,componentWillReceiveProps,componentWillUpdate,在新的react17上中要加前缀UNSAFE。