1.盛最多水的容器
已解答
中等
相关标签
相关企业
提示
给定一个长度为 n
的整数数组 height
。有 n
条垂线,第 i
条线的两个端点是 (i, 0)
和 (i, height[i])
。
找出其中的两条线,使得它们与 x
轴共同构成的容器可以容纳最多的水。
返回容器可以储存的最大水量。
**说明:**你不能倾斜容器。
示例 1:
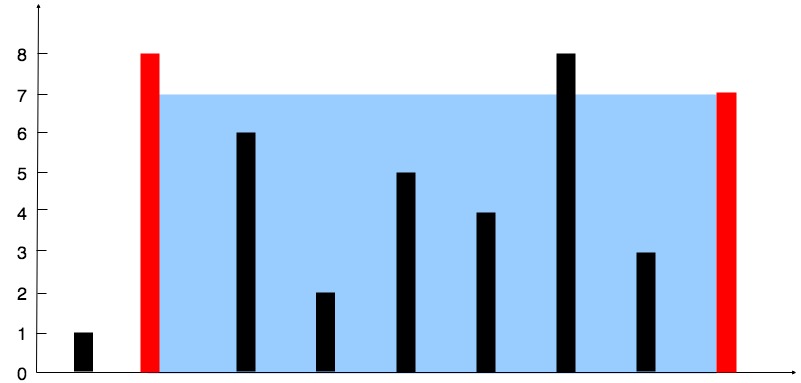
输入:[1,8,6,2,5,4,8,3,7]
输出:49
解释:图中垂直线代表输入数组 [1,8,6,2,5,4,8,3,7]。在此情况下,容器能够容纳水(表示为蓝色部分)的最大值为 49。
示例 2:
输入:height = [1,1]
输出:1
提示:
n == height.length
2 <= n <= 105
0 <= height[i] <= 104
java
public static void main(String[] args) {
int[] nums = {1,8,6,2,5,4,8,3,7};
int maxArea = maxArea3(nums);
System.out.println(maxArea);
}
public static int maxArea3(int[] height) {
int left = 0, right = height.length - 1;//左指针,右指针
int res = 0;//maxArea
while(left < right){//左指针<右指针
int w = right - left;//x
int h = Math.min(height[left], height[right]);//y
res = Math.max(res, w * h);
while(left < right && height[left] <= h) left++;//移动左指针,寻找比当前y值更大的y值
while(left < right && height[right] <= h) right--;//移动右指针,寻找比当前y值更大的y值
}
return res;
}
//1-my(2)
public static int maxArea2(int[] height) {
int maxArea = 0;
for (int i = 0; i < height.length; i++) {//左指针
for (int j = height.length-1; j >= 0 && j>=i ; j--) {//右指针
int x = j - i;
int y = Math.min(height[i],height[j]);
int area = x * y;
maxArea = Math.max(maxArea,area);
if (height[i] < height[j]){
break;
}
}
}
return maxArea;
}
//1-my
public static int maxArea(int[] height) {
int maxArea = 0;
for (int i = height.length-1; i >= 0 ; i--) {//x轴大小
for (int j = 0; j < height.length; j++) {//index
if (j+i<height.length) {
int left = height[j];
int right = height[j + i];
int min = Math.min(left, right);
int area = min * i;
maxArea = Math.max(maxArea,area);
}
}
}
return maxArea;
}
2.三数之和
给你一个整数数组 nums
,判断是否存在三元组 [nums[i], nums[j], nums[k]]
满足 i != j
、i != k
且 j != k
,同时还满足 nums[i] + nums[j] + nums[k] == 0
。请你返回所有和为 0
且不重复的三元组。
**注意:**答案中不可以包含重复的三元组。
示例 1:
输入:nums = [-1,0,1,2,-1,-4]
输出:[[-1,-1,2],[-1,0,1]]
解释:
nums[0] + nums[1] + nums[2] = (-1) + 0 + 1 = 0 。
nums[1] + nums[2] + nums[4] = 0 + 1 + (-1) = 0 。
nums[0] + nums[3] + nums[4] = (-1) + 2 + (-1) = 0 。
不同的三元组是 [-1,0,1] 和 [-1,-1,2] 。
注意,输出的顺序和三元组的顺序并不重要。
示例 2:
输入:nums = [0,1,1]
输出:[]
解释:唯一可能的三元组和不为 0 。
示例 3:
输入:nums = [0,0,0]
输出:[[0,0,0]]
解释:唯一可能的三元组和为 0 。
提示:
3 <= nums.length <= 3000
-105 <= nums[i] <= 105
my(1-3)
java
public static List<List<Integer>> threeSum3(int[] nums) {
Arrays.sort(nums);
Set<List<Integer>> temp = new HashSet<>();
for (int i = 0; i < nums.length && nums[i] <= 0 ; i++) {
for (int j = i+1; j < nums.length; j++) {
for (int k = j+1; k < nums.length; k++) {
if (i!=j && j!=k && k!= i && nums[i]+ nums[j]+nums[k]==0 ){
List<Integer> inner = new ArrayList<>();
inner.add(nums[i]);
inner.add(nums[j]);
inner.add(nums[k]);
temp.add(inner);
}
}
}
}
return new ArrayList<>(temp);
}
public static List<List<Integer>> threeSum2(int[] nums) {
Set<List<Integer>> temp = new HashSet<>();
for (int i = 0; i < nums.length; i++) {
for (int j = 0; j < nums.length; j++) {
for (int k = 0; k < nums.length; k++) {
if (i!=j && j!=k && k!= i && nums[i]+ nums[j]+nums[k]==0 ){
List<Integer> inner = new ArrayList<>();
inner.add(nums[i]);
inner.add(nums[j]);
inner.add(nums[k]);
inner = inner.stream().sorted().collect(Collectors.toList());
temp.add(inner);
}
}
}
}
return new ArrayList<>(temp);
}
public static List<List<Integer>> threeSum(int[] nums) {
//请你返回所有和为 0 且不重复的三元组 的下标组合
List<List<Integer>> res = new ArrayList<>();
Map<Integer,List<Integer>> map = new HashMap<>();
for (int left = 0; left < nums.length; left++) {
for (int right = nums.length - 1; right >=0; right--) {
int temp = 0 - nums[left] - nums[right];
List<Integer> mapOrDefault = map.getOrDefault(temp, new ArrayList<>());
if (mapOrDefault.size()==0){
mapOrDefault.add(left);
mapOrDefault.add(right);
map.put(temp,mapOrDefault);
}
}
}
for (int i = 0; i < nums.length; i++) {
List<Integer> mapOrDefault = map.getOrDefault(nums[i], new ArrayList<>());
if (mapOrDefault.size()==2){
mapOrDefault.add(i);
int size = mapOrDefault.stream().collect(Collectors.toSet()).size();
if (size==3) {
res.add(mapOrDefault);
}
}
}
return res;
}
others-1
java
public static void main(String[] args) {
int[] nums1 = {-1,0,1,-2,0,2,-2,-1,0};
int[] nums2 = {0,0,0};
int[] nums = {-1,0,1,2,-1,-4,-2,-3,3,0,4};
List<List<Integer>> threeSum = findTriplets(nums);
System.out.println(threeSum);
}
public static List<List<Integer>> findTriplets(int[] nums) {
Set<List<Integer>> result = new HashSet<>(); // 用于去重
Arrays.sort(nums); // 先排序数组,方便去重和双指针
for (int i = 0; i < nums.length - 2; i++) {
if (i > 0 && nums[i] == nums[i - 1]) continue; // 跳过重复的 i
int left = i + 1;
int right = nums.length - 1;
while (left < right) {//i!=j && j!=k && k!= i
int sum = nums[i] + nums[left] + nums[right];
if (sum == 0) { // 三数之和为 0,nums[i]+ nums[j]+nums[k]==0
List<Integer> inner = new ArrayList<>();
inner.add(nums[i]);
inner.add(nums[left]);
inner.add(nums[right]);
result.add(inner); // 自动去重
left++;
right--;
} else if (sum < 0) {
left++;
} else {
right--;
}
}
}
return new ArrayList<>(result);
}
others-2
java
public static List<List<Integer>> findTriplets2(int[] nums) {
return new AbstractList<List<Integer>>() {
// Declare List of List as a class variable
private List<List<Integer>> list;
// Implement get method of AbstractList to retrieve an element from the list
public List<Integer> get(int index) {
// Call initialize() method
initialize();
// Return the element from the list at the specified index
return list.get(index);
}
// Implement size method of AbstractList to get the size of the list
public int size() {
// Call initialize() method
initialize();
// Return the size of the list
return list.size();
}
// Method to initialize the list
private void initialize() {
// Check if the list is already initialized
if (list != null)
return;
// Sort the given array
Arrays.sort(nums);
// Create a new ArrayList
list = new ArrayList<>();
// Declare required variables
int l, h, sum;
// Loop through the array
for (int i = 0; i < nums.length; i++) {
// Skip the duplicates
if (i != 0 && nums[i] == nums[i - 1])
continue;
// Initialize l and h pointers
l = i + 1;
h = nums.length - 1;
// Loop until l is less than h
while (l < h) {
// Calculate the sum of three elements
sum = nums[i] + nums[l] + nums[h];
// If sum is zero, add the triple to the list and update pointers
if (sum == 0) {
list.add(getTriple(nums[i], nums[l], nums[h]));
l++;
h--;
while (l < h && nums[l] == nums[l - 1])
l++;
while (l < h && nums[h] == nums[h + 1])
h--;
} else if (sum < 0) {
// If sum is less than zero, increment l
l++;
} else {
// If sum is greater than zero, decrement h
h--;
}
}
}
}
};
}
private static List<Integer> getTriple(int i, int j, int k){
return new AbstractList<Integer>() {
private int[] data;
// Constructor to initialize the triple with three integers
// Method to initialize the list
private void initialize(int i, int j, int k) {
if (data != null)
return;
data = new int[] { i, j, k };
}
// Implement get method of AbstractList to retrieve an element from the triple
public Integer get(int index) {
// Call initialize() method
initialize(i, j, k);
return data[index];
}
// Implement size method of AbstractList to get the size of the triple
public int size() {
// Call initialize() method
initialize(i, j, k);
return 3;
}
};
}