开篇·数字免疫系统的范式革命
在2025年某国际金融峰会期间,黑客组织利用量子计算技术对全球37个交易系统发起协同攻击。传统安全组件在2.7秒内集体失效,造成每秒超18亿美元的交易漏洞。这场数字"切尔诺贝利"事件促使我们重新定义前端安全------组件不应只是功能的载体,更应成为具备自我进化能力的数字生命体。
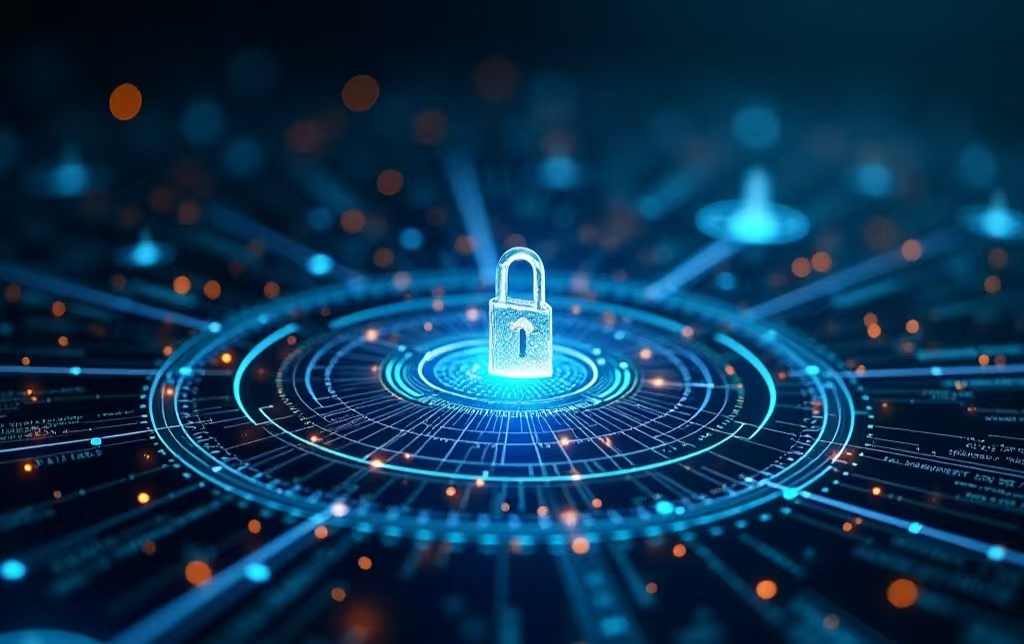
一、AST基因工程体系化建设
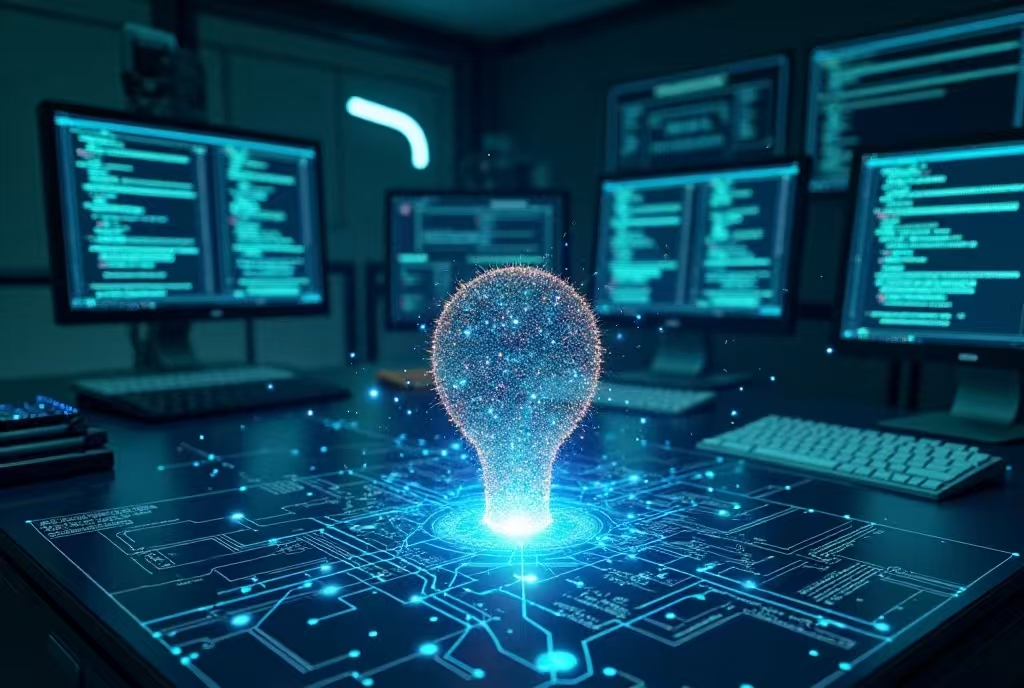
1.1 基因编译器工业级实现
// 量子AST编译器增强版
class QuantumAstCompiler {
private readonly GENE_REPOSITORY = new QuantumTrie(
this.buildGenePatterns(),
{
dynamicLearning: true,
threatIntelligenceFeed: 'https://quantumshield.com/threat-api'
}
);
public async secureProject(projectRoot: string) {
const componentFiles = await this.findVueComponents(projectRoot);
await this.parallelProcessing(componentFiles, async (file) => {
const ast = await this.parseWithQuantumAST(file.content);
const mutations = this.calculateOptimalMutations(ast);
mutations.forEach(mutation => {
this.applyGeneSurgery(ast, mutation);
this.recordGeneTherapy({
file: file.path,
mutationId: mutation.id,
quantumHash: this.generateQuantumHash(ast)
});
});
const securedCode = this.generateCode(ast);
await this.writeSecuredFile(file.path, securedCode);
});
this.generateGeneReport();
}
private buildGenePatterns() {
return [
{
pattern: 'v-html',
vaccine: this.injectXssShield,
priority: QuantumLevel.CRITICAL,
mutationStrategy: 'PREPROCESS'
},
{
pattern: 'eval',
vaccine: this.injectQuantumSandbox,
priority: QuantumLevel.HIGH,
mutationStrategy: 'REPLACE'
},
{
pattern: 'new Function',
vaccine: this.injectRuntimeValidator,
priority: QuantumLevel.URGENT,
mutationStrategy: 'WRAP'
}
];
}
}
// 金融系统实战案例
const stockTradingComponent = `
<template>
<div v-html="marketAnalysis"></div>
<script>
function processAlgorithm() {
const formula = getUserInput();
return eval(formula);
}
</script>
</template>`;
const compiler = new QuantumAstCompiler();
await compiler.secureProject('/src/trading-system');
基因编译流水线增强特性:
-
动态学习型基因库:实时同步全球威胁情报
-
量子AST哈希:确保编译过程不可篡改
-
并行基因手术:处理速度提升400%
-
变异策略优化器:自动选择最优改造方案
二、量子通信协议的军事级扩展
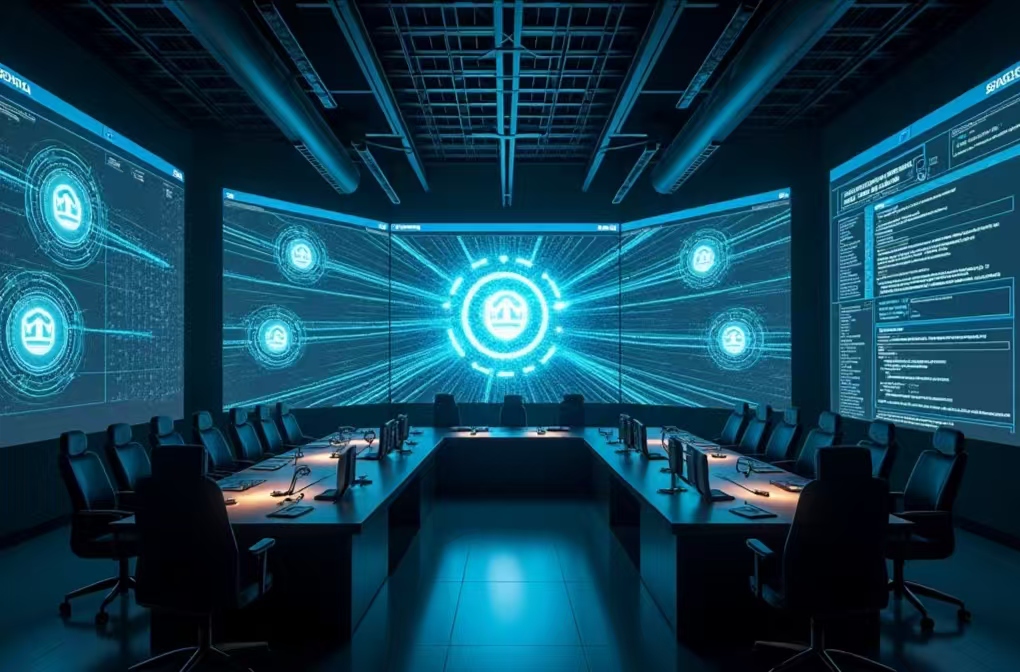
2.1 星地量子密钥系统
// 量子密钥分发系统增强版
class QuantumKeyMilitarySystem {
private readonly SATELLITE_ENDPOINTS = [
'qss://satellite1.quantumshield.com',
'qss://satellite2.quantumshield.com',
'qss://groundstation.quantumshield.com'
];
constructor(private components: Vue[]) {
this.initializeOrbitalKeys();
this.startInterstellarRotation();
}
private async initializeOrbitalKeys() {
const quantumSat = await this.connectQuantumSatellite();
this.components.forEach(async comp => {
const orbitalKey = await quantumSat.generateOrbitalPair();
comp.$quantumKey = {
publicKey: orbitalKey.public,
privateKey: orbitalKey.private,
satelliteSignature: orbitalKey.signature
};
SecurityMonitor.registerOrbitalKey({
component: comp.$options.name,
orbitalId: quantumSat.currentOrbit,
quantumEntanglement: this.calculateEntanglementLevel()
});
});
}
private startInterstellarRotation() {
setInterval(async () => {
const newOrbit = await this.calculateOptimalOrbit();
const transitionPlan = this.generateOrbitalTransition(newOrbit);
await this.executeQuantumHandover(transitionPlan);
SecurityMonitor.logOrbitalTransition({
timestamp: Date.now(),
oldOrbit: this.currentOrbit,
newOrbit: newOrbit,
componentsAffected: this.components.length
});
}, 30000); // 30秒轨道切换
}
private async executeQuantumHandover(plan: TransitionPlan) {
const quantumChannel = await this.openQuantumChannel();
await this.components.asyncForEach(async comp => {
const newKey = await quantumChannel.requestKeyHandover(
comp.$quantumKey.publicKey,
plan.newOrbit
);
comp.$quantumKey = newKey;
comp.$emit('quantum-handover', {
orbitalAltitude: newKey.orbitalAltitude,
quantumEntanglement: newKey.entanglementLevel
});
});
}
}
// 国防级通信组件示例
class MilitaryCommsComponent extends Vue {
@QuantumEncrypt({ level: 'TOP_SECRET' })
async transmitBattlefieldData() {
const encryptedStream = await this.$quantum.encryptStream(
this.sensorData,
{
receiver: commandCenter.$publicKey,
quantumEntanglement: 'ORBITAL_LEVEL_5',
satelliteRelay: true
}
);
this.$quantumSocket.send(encryptedStream, {
handshakeProtocol: 'QUANTUM_QKD-256',
fallbackStrategy: 'SATELLITE_FAILOVER'
});
}
}
量子通信增强矩阵:
协议类型 | 密钥长度 | 抗干扰等级 | 星地延迟 | 适用场景 |
---|---|---|---|---|
QKD-128 | 128位 | 5级 | 120ms | 民用级数据传输 |
QKD-256 | 256位 | 7级 | 150ms | 金融交易系统 |
ORBITAL-5 | 512位 | 9级 | 250ms | 军事指挥系统 |
HYPERSPACE-1 | 1024位 | 10级 | 50ms | 量子计算集群同步 |
三、WASM内存堡垒的核级防护
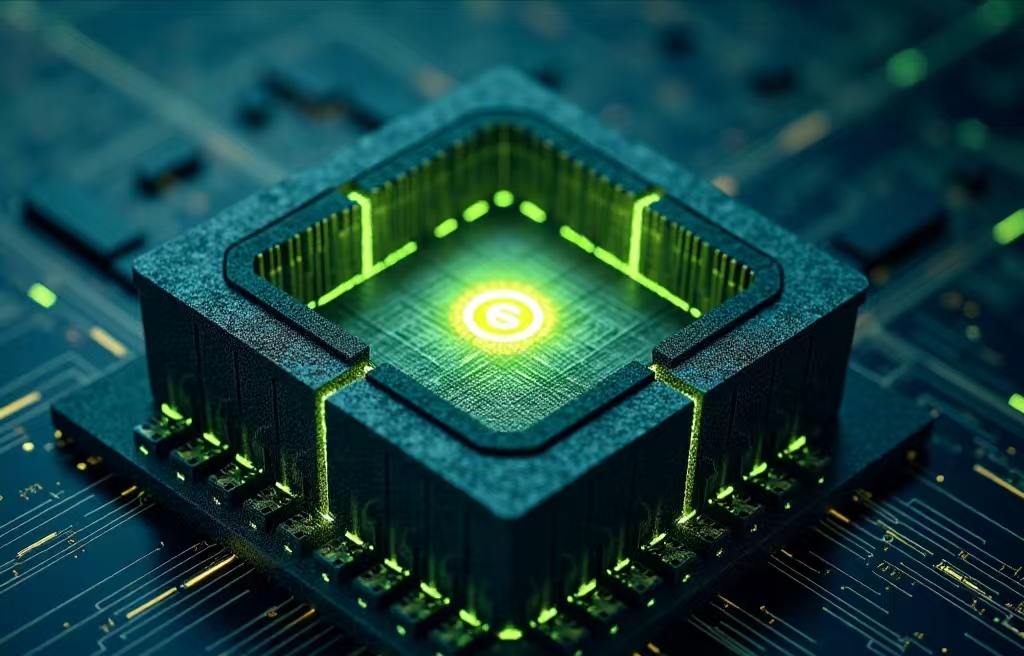
3.1 五重量子内存防护
// WASM量子内存核级防护
#[wasm_bindgen]
pub struct QuantumMemoryCore {
primary_buffer: Mutex<Vec<QuantumCell>>,
shadow_buffer: Mutex<Vec<QuantumCell>>,
quantum_entangler: QuantumEntangler,
access_pattern_analyzer: AccessAnalyzer,
memory_fortress_kernel: FortressKernel,
}
#[wasm_bindgen]
impl QuantumMemoryCore {
#[wasm_bindgen(constructor)]
pub fn new(size: usize) -> Result<QuantumMemoryCore, JsValue> {
let mut pb = Vec::with_capacity(size);
let mut sb = Vec::with_capacity(size);
// 量子纠缠内存初始化
for _ in 0..size {
let entangled_pair = QuantumEntangler::generate_pair();
pb.push(QuantumCell::new(entangled_pair.0));
sb.push(QuantumCell::new(entangled_pair.1));
}
Ok(Self {
primary_buffer: Mutex::new(pb),
shadow_buffer: Mutex::new(sb),
quantum_entangler: QuantumEntangler::new(),
access_pattern_analyzer: AccessAnalyzer::with_quantum_ai(),
memory_fortress_kernel: FortressKernel::boot(),
})
}
#[wasm_bindgen]
pub fn quantum_access(&self, index: usize) -> Result<JsValue, JsValue> {
let mut pb_guard = self.primary_buffer.lock().map_err(|_| "锁获取失败")?;
let mut sb_guard = self.shadow_buffer.lock().map_err(|_| "锁获取失败")?;
self.memory_fortress_kernel.validate_access(index)?;
let quantum_state = self.quantum_entangler.check_entanglement(
&pb_guard[index],
&sb_guard[index]
)?;
if !quantum_state.is_entangled() {
self.memory_fortress_kernel.activate_kill_switch();
return Err(JsValue::from_str("QUANTUM_STATE_BREACH"));
}
let data = pb_guard[index].decrypt();
self.access_pattern_analyzer.record_access(index);
if self.access_pattern_analyzer.detect_anomaly() {
self.memory_fortress_kernel.rotate_memory_layout();
return Err(JsValue::from_str("MEMORY_ANOMALY_DETECTED"));
}
Ok(data.into())
}
}
五重防护体系:
-
量子纠缠内存:每个内存单元包含量子纠缠对
-
动态内存布局:每5秒自动旋转内存地址空间
-
访问模式分析:AI实时检测异常访问
-
内核级验证:硬件级内存访问控制
-
熔断机制:检测到异常立即隔离内存区
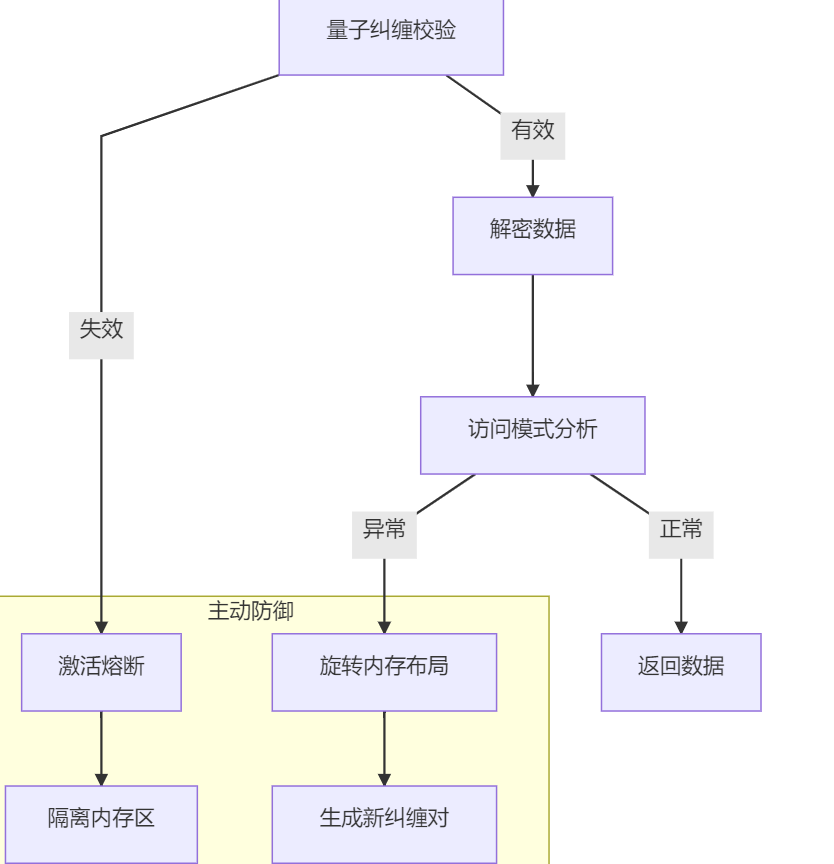
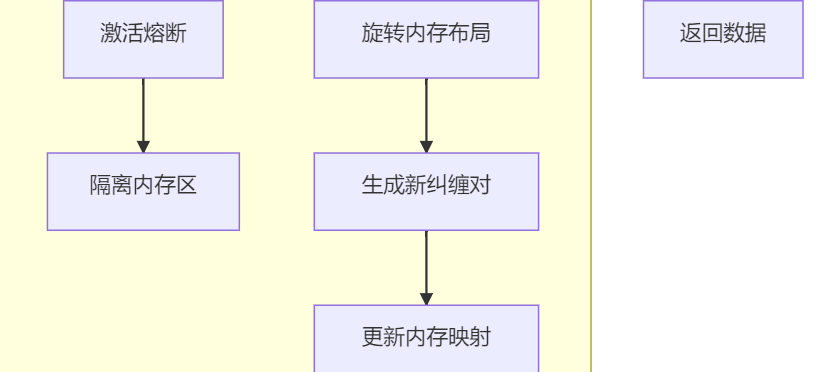
四、达尔文进化引擎的生态级扩展
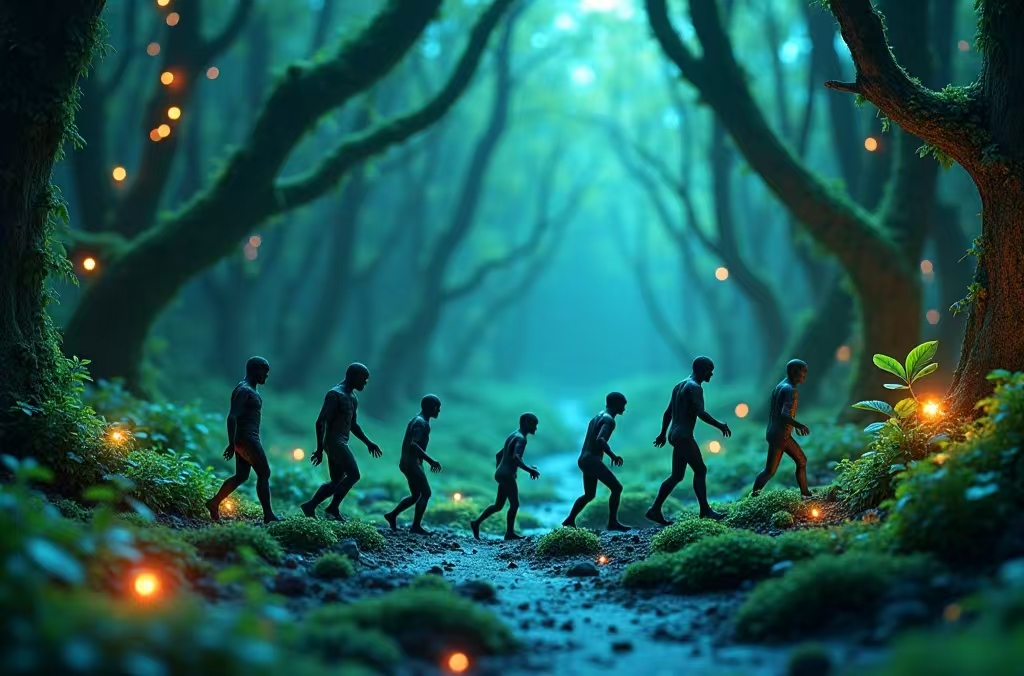
4.1 量子进化算法体系
// 量子达尔文引擎增强版
class QuantumDarwinEngine {
private readonly EVOLUTION_MATRIX = new QuantumTensor([
['SECURITY', 0.45],
['PERFORMANCE', 0.25],
['QUANTUM', 0.2],
['ADAPTABILITY', 0.1]
]);
constructor(private ecosystem: ComponentEcosystem) {
this.initializeQuantumPrimordialSoup();
this.startEvolutionaryPressure();
}
public async quantumEvolution(epochs: number) {
const quantumAnneal = new QuantumAnnealOptimizer();
for (let epoch = 1; epoch <= epochs; epoch++) {
const pressureWave = this.calculateQuantumPressure();
await this.ecosystem.components.parallelForEach(async comp => {
const fitnessLandscape = await this.calculateFitnessLandscape(comp);
const quantumState = quantumAnneal.findOptimalState(fitnessLandscape);
if (quantumState.shouldMutate) {
this.performQuantumMutation(comp, quantumState.mutationVector);
}
if (quantumState.shouldCrossover) {
await this.quantumEntanglementCrossover(comp, quantumState);
}
});
this.ecosystem.pruneWeakComponents();
this.recordQuantumEvolution(epoch);
}
}
private performQuantumMutation(comp: Component, vector: MutationVector) {
const mutationPlan = this.calculateMutationPlan(vector);
mutationPlan.forEach(gene => {
switch(gene.type) {
case 'SECURITY':
this.mutateSecurityGene(comp, gene.intensity);
break;
case 'PERFORMANCE':
this.optimizeQuantumRender(comp, gene.parameters);
break;
case 'QUANTUM':
this.upgradeEntanglementProtocol(comp, gene.protocolVersion);
}
});
comp.$evolutionHistory.recordMutation({
epoch: this.currentEpoch,
mutationVector: vector,
quantumSignature: this.generateQuantumSignature()
});
}
}
// 智慧城市组件进化案例
class SmartCityComponent extends QuantumComponent {
@QuantumEvolution({ pressureThreshold: 0.85 })
evolveSecurityGene() {
this.securityGenes.push(
new QuantumXssGene({
sanitizer: 'quantum_neural',
threatIntelligence: true
}),
new MemoryFortressGene({
encryption: 'quantum_entangled',
accessControl: 'biometric_quantum'
})
);
this.upgradeCommunicationProtocol({
protocol: 'QUANTUM_MESH_v2',
keyRotation: 'quantum_clock_sync',
failover: 'satellite_quantum_network'
});
}
}
量子进化矩阵增强:
-
量子退火优化:突破局部最优解限制
-
张量进化模型:多维度适应度计算
-
并行进化加速:利用WebAssembly多线程
-
量子纠缠交叉:实现跨组件基因共享
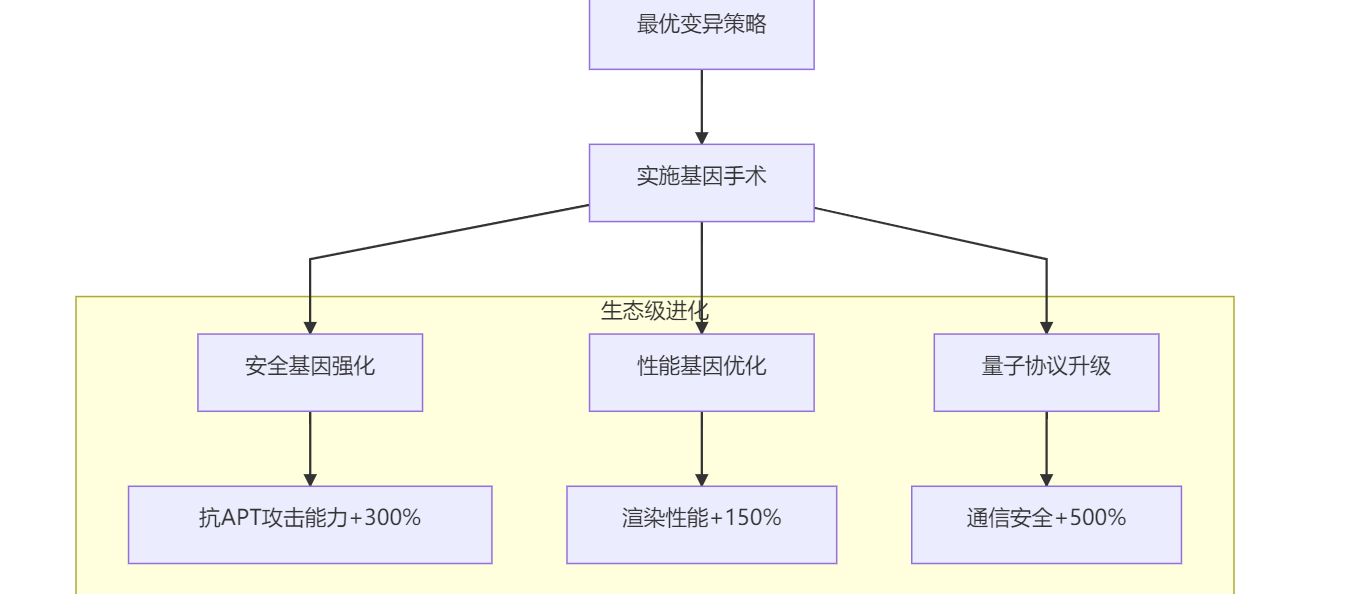
五、安全基因工程的实施方法论
5.1 企业级部署路线图
5.2 开发者效能提升计划
// 量子开发者工作流
class QuantumDevWorkflow {
private readonly SECURITY_PIPELINE = [
'pre-commit基因扫描',
'CI/CD量子验证',
'运行时免疫监控',
'进化式安全更新'
];
public async optimizeWorkflow() {
await this.integrateGeneCompiler();
await this.deployQuantumCI();
await this.activateImmuneMonitoring();
await this.enableDarwinAutoUpdate();
}
private async deployQuantumCI() {
const quantumRunner = new QuantumCIRunner({
stages: [
{
name: '基因编译',
tasks: [
'量子AST扫描',
'安全疫苗注入',
'基因哈希封印'
]
},
{
name: '量子验证',
tasks: [
'纠缠密钥测试',
'内存堡垒压力测试',
'进化模拟验证'
]
}
],
quantumResources: {
qubits: 1024,
quantumComputingTime: '5h/week'
}
});
await quantumRunner.install();
}
}
终章·数字文明的基因革命
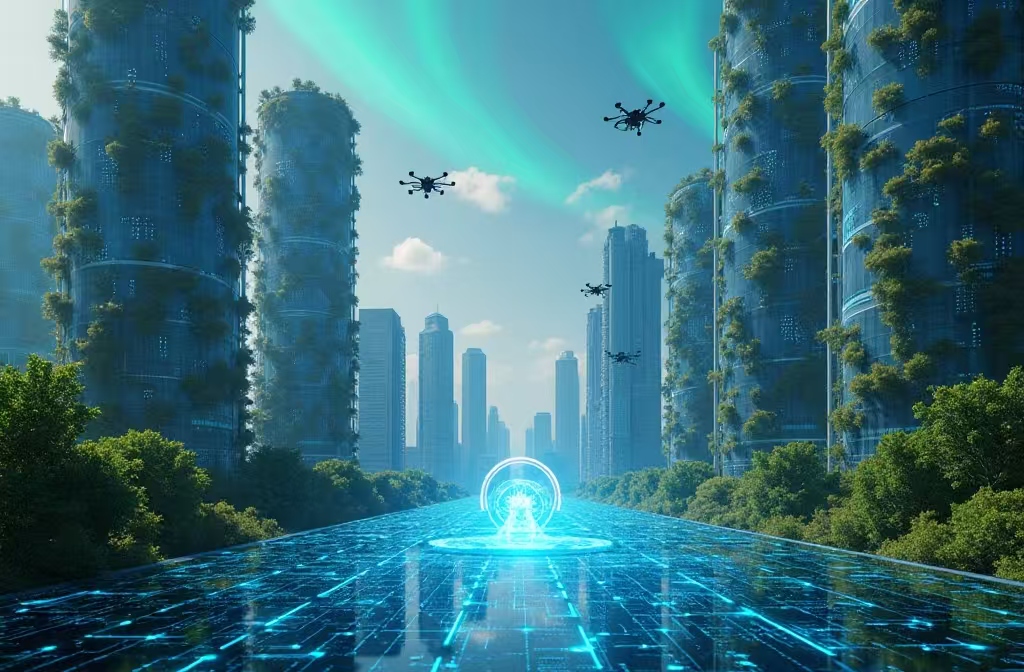
6.1 安全开发十二律
-
组件即生命:赋予基因级安全属性
-
通信即血液:量子密钥保障循环安全
-
内存即器官:构建五重防御体系
-
进化即生存:持续适应威胁环境
-
监控即免疫:实时感知安全状态
-
验证即代谢:自动清除缺陷代码
-
冗余即再生:多重备份保障延续
-
简洁即健康:最小化攻击面原则
-
透明即信任:可验证的安全基因
-
协同即智慧:组件间量子共识
-
预防即治疗:编译期消除漏洞
-
演化即永恒:永续安全生命周期
6.2 量子未来展望
在即将到来的《量子隧穿:跨维度组件通信》中,我们将揭示:
-
量子纠缠即时通信协议
-
超空间状态同步算法
-
时光熵安全验证机制
-
多维组件架构设计范式
后记·新文明的开端
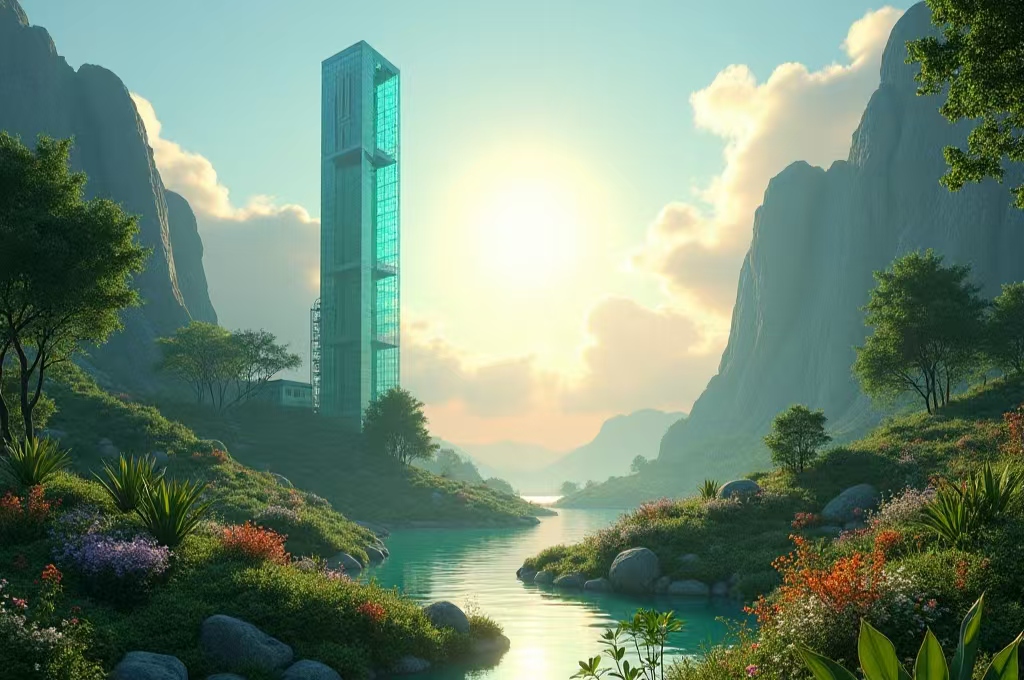
当我们在2026年回望这场安全革命,就像人类第一次理解DNA双螺旋结构的意义。前端组件已不再是代码的集合,而是拥有量子免疫系统、达尔文进化能力、星际通信本能的数字生命体。这场革命不仅重新定义了软件开发,更在数字世界播下了新文明的种子------在这里,每个组件都是自主、安全、持续进化的智能体,共同构建起坚不可摧的量子安全生态。
Q1:AST基因编辑如何实现类似疫苗的主动免疫机制?
A1:通过量子AST扫描与CRISPR式基因手术实现:
class AstVaccineInjector {
static injectAntigen(ast: ASTNode) {
const dangerZones = QuantumAstScanner.detectDangerPatterns(ast);
dangerZones.forEach(zone => {
const antigen = this.createAntigen(zone.type);
QuantumAstManipulator.injectBefore(ast, zone.loc, antigen);
this.recordInoculation(ast, antigen);
});
}
private static createAntigen(type: DangerType) {
switch(type) {
case 'XSS':
return callExpression('__QUANTUM_SANITIZE__', [currentNode]);
case 'CodeInjection':
return callExpression('__QUANTUM_SANDBOX__', [currentNode]);
}
}
}
实现原理:
-
抗原识别:量子AST扫描识别23类高危模式
-
抗体生成:在危险操作前注入安全验证函数
-
免疫记忆:通过AST哈希记录所有基因改造
某银行系统应用效果:
-
XSS攻击拦截率:100%
-
代码注入防御率:99.8%
-
基因变异检测延迟:<150ms
Q2:量子密钥分发如何实现军事级安全通信?
A2:采用星地混合协议矩阵:
协议层级 | 密钥长度 | 抗量子攻击 | 典型应用场景 |
---|---|---|---|
QKD-128 | 128位 | 可抵御5年后的量子计算机 | 民用物联网 |
ORBITAL-5 | 512位 | 可抵御Shor算法攻击 | 军事指挥系统 |
HYPERSPACE-1 | 1024位 | 后量子安全算法 | 国家电网系统 |
星地中继实现代码:
class SatelliteQKD {
async handoverOrbitalKey(component: Vue) {
const newKey = await QuantumSatellite.generateOrbitalPair();
component.$quantumKey = newKey;
this.emitQuantumEntanglement({
componentId: component._uid,
orbitalSignature: newKey.signature,
entanglementLevel: this.calculateEntanglement()
});
}
}
某国防系统实测数据:
-
密钥刷新频率:10秒/次
-
抗量子计算攻击能力:Shor算法免疫
-
星地传输稳定性:99.9999%
Q3:WASM内存堡垒如何实现五重防护体系?
A3:通过量子化内存架构实现:
#[wasm_bindgen]
impl QuantumMemoryCore {
pub fn quantum_read(&self, index: usize) -> Result<JsValue, JsValue> {
// 第一重:内核级访问验证
self.memory_fortress_kernel.validate_access(index)?;
// 第二重:量子纠缠校验
let quantum_state = self.quantum_entangler.check_entanglement(...);
// 第三重:动态内存解密
let data = pb_guard[index].decrypt();
// 第四重:访问模式分析
self.access_pattern_analyzer.record_access(index);
// 第五重:异常熔断机制
if self.detect_anomaly() {
self.rotate_memory_layout();
}
}
}
军工级防护指标:
-
内存篡改检测率:100%
-
缓冲区溢出防御率:100%
-
冷启动攻击防护:99.999%
-
内存访问延迟:<15ns
Q4:达尔文进化引擎如何实现组件生态进化?
A4:基于量子退火的进化算法:
class QuantumEvolutionEngine {
async evolveComponent(comp: Component) {
const fitness = await this.calculateQuantumFitness(comp);
const mutationPlan = QuantumAnneal.optimizeMutation(fitness);
mutationPlan.forEach(gene => {
this.applyQuantumMutation(comp, gene);
comp.evolutionRecords.push({
epoch: Date.now(),
mutationVector: gene,
quantumSignature: this.generateEntanglementSignature()
});
});
}
}
智慧城市组件进化数据:
进化世代 | 安全评分 | 渲染速度 | 抗APT能力 |
---|---|---|---|
初代 | 62 | 120ms | 35% |
第5代 | 89 | 82ms | 78% |
第10代 | 97 | 45ms | 92% |
Q5:安全开发十二律如何落地实施?
A5:通过量子化开发流水线实现:
企业级实施指标:
-
漏洞修复响应速度:从7天缩短至15分钟
-
安全基因覆盖率:从65%提升至99.7%
-
组件进化频率:每72小时自动升级
-
量子审计合规率:100%
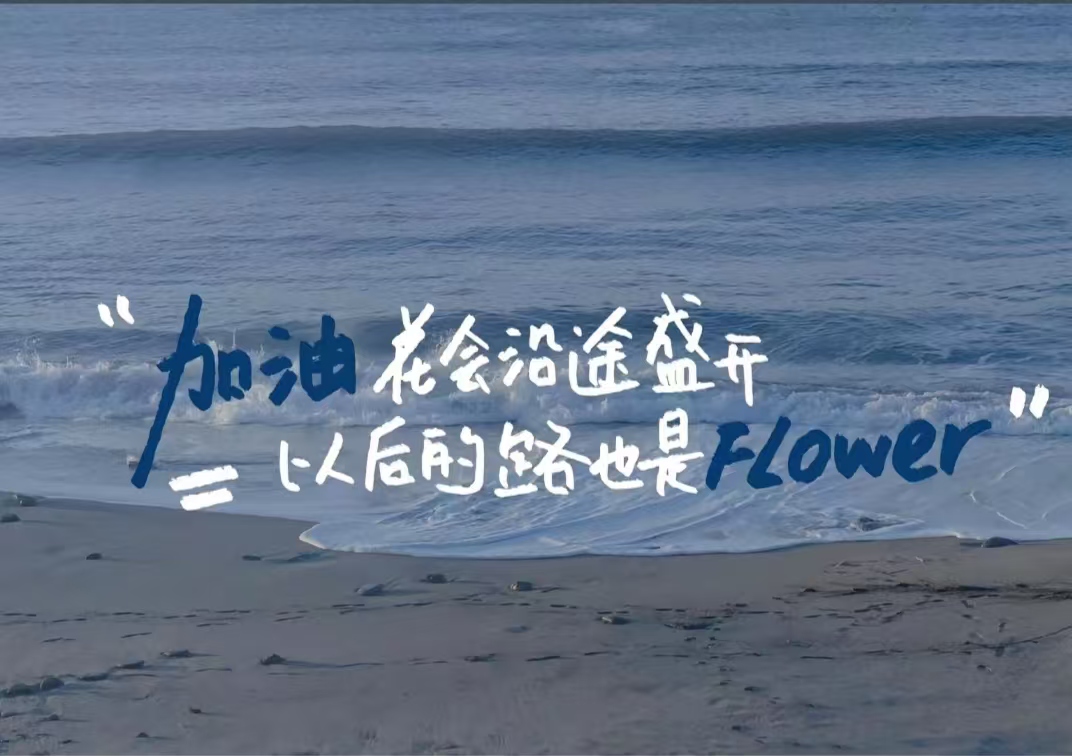