文章目录
- [使用 Semantic Kernel 快速对接国产大模型实战指南(DeepSeek/Qwen/GLM)](#使用 Semantic Kernel 快速对接国产大模型实战指南(DeepSeek/Qwen/GLM))
使用 Semantic Kernel 快速对接国产大模型实战指南(DeepSeek/Qwen/GLM)
一、引言
在人工智能应用开发中,统一对接不同大模型的能力至关重要。微软推出的 Semantic Kernel 作为优秀的 AI 编排框架,能够帮助我们快速对接各类大模型。本文将手把手教你如何使用 Semantic Kernel 对接国内三大主流模型:DeepSeek、通义千问(Qwen)和智谱AI(GLM),并提供可运行的代码示例。文末提供完整代码示例和注意事项。
二、环境准备
2.1 开发环境
• .NET 6+ SDK
• Visual Studio 2022
• NuGet包:
```bash
dotnet add package Microsoft.SemanticKernel
```
2.2 模型服务配置
模型 | 接口地址示例 | API Key获取方式 |
---|---|---|
DeepSeek | https://api.deepseek.com/v1 |
DeepSeek平台申请(需充值) |
Qwen | https://api.siliconflow.cn/ |
SiliconCloud平台申请(有免费额度) |
GLM | https://open.bigmodel.cn/api/paas/v4/chat/completions |
智谱AI开放平台申请(有免费模型) |
三、核心代码实现
下述内容封装两种对话交互模式,采用统一的接口设计:
-
非流式输出:完整获取响应后一次性输出
-
流式输出:实时输出响应片段,提升交互体验
注:两种模式都提供了对话历史管理机制,确保多轮对话上下文连贯性。
3.1 会话代码封装
csharp
/// <summary>
/// 统一对话管理(非流式)
/// </summary>
/// <param name="kernel"></param>
/// <returns></returns>
private async Task StartChatSession(Kernel kernel)
{
var chatService = kernel.GetRequiredService<IChatCompletionService>();
var history = new ChatHistory();
while (true)
{
Console.Write("用户 > ");
var input = Console.ReadLine();
history.AddUserMessage(input);
var response = await chatService.GetChatMessageContentAsync(history);
Console.WriteLine($"助手 > {response.Content}");
history.AddAssistantMessage(response.Content);
}
}
/// <summary>
/// 统一对话管理(流式输出)
/// </summary>
/// <param name="kernel"></param>
/// <returns></returns>
private async Task StartStreamingChatSession(Kernel kernel)
{
var chatService = kernel.GetRequiredService<IChatCompletionService>();
var history = new ChatHistory();
while (true)
{
///获取用户输入
Console.Write("用户 > ");
var input = Console.ReadLine();
//将用户输入添加到历史记录
history.AddUserMessage(input);
//获取流式响应
var response = chatService.GetStreamingChatMessageContentsAsync(
chatHistory: history,
kernel: kernel
);
Console.WriteLine($"助手 > ");
string resStr = "";
//输出流式响应
await foreach (var chunk in response)
{
resStr += chunk;//拼接聊天记录
Console.Write(chunk);
}
//将完整的响应添加到历史记录
history.AddAssistantMessage(resStr);
//输出换行
Console.WriteLine();
}
}
3.2 CurModelContext封装
将要获取的模型,按如下方式封装,也可直接写死在代码中,其中"sk-xx"和"Model"按需替换实际使用的key和模型。
csharp
/// <summary>
/// 全局参数
/// </summary>
public class Global
{
/// <summary>
/// 获取模型配置
/// </summary>
public static ModelConfig CurModelContext(string model)
{
switch (model)
{
case "glm-4-flash":
return new ModelConfig
{
Model = "glm-4-flash",
EndpointKey = "https://open.bigmodel.cn/api/paas/v4",
ApiKey = "sk-xxx"
};
case "glm-z1-flash":
return new ModelConfig
{
Model = "glm-z1-flash",
EndpointKey = "https://open.bigmodel.cn/api/paas/v4",
ApiKey = "sk-xxx"
};
case "Qwen/Qwen2.5-72B-Instruct":
return new ModelConfig
{
Model = "Qwen/Qwen2.5-72B-Instruct",
EndpointKey = "https://api.siliconflow.cn",
ApiKey = "sk-xxx"
};
case "deepseek-chat":
return new ModelConfig
{
Model = "deepseek-chat",
EndpointKey = "https://api.deepseek.com/v1",
ApiKey = "sk-xxx"
};
case "deepseek-reasoner":
return new ModelConfig
{
Model = "deepseek-reasoner",
EndpointKey = "https://api.deepseek.com/v1",
ApiKey = "sk-xxx"
};
default:
break;
}
return null;
}
}
3.3 DeepSeek对接示例
csharp
var modelConfig = Global.CurModelContext("deepseek-chat");
// 1. 填充OpenAI格式LLM调用参数值
var modelId = modelConfig.Model;
var endpoint = modelConfig.EndpointKey;
var apiKey = modelConfig.ApiKey;
// 2. 创建一个OpenAI聊天完成的内核
var builder = Kernel.CreateBuilder()
.AddOpenAIChatCompletion(modelId,
new Uri(endpoint),
apiKey);
// 4.构建内核
Kernel kernel = builder.Build();
//5. 对话功能(流式)
await StartStreamingChatSession(kernel);
//6. 对话功能(非流式)
//await StartChatSession(kernel);
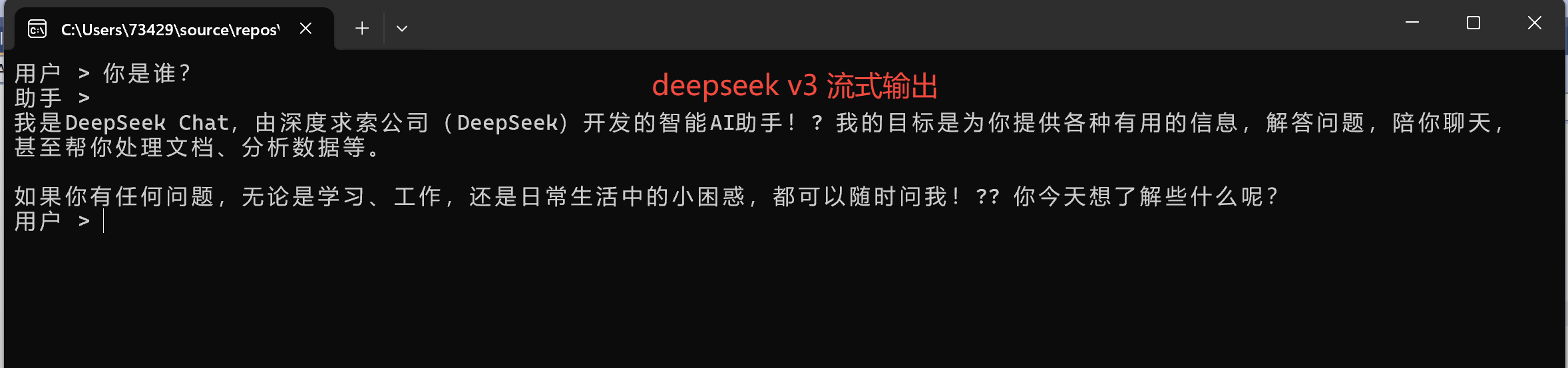
3.4 Qwen对接示例
csharp
var modelConfig = Global.CurModelContext("Qwen/Qwen2.5-72B-Instruct");
// 1. 填充OpenAI格式LLM调用参数值
var modelId = modelConfig.Model;
var endpoint = modelConfig.EndpointKey;
var apiKey = modelConfig.ApiKey;
// 2. 创建一个OpenAI聊天完成的内核
var builder = Kernel.CreateBuilder()
.AddOpenAIChatCompletion(modelId,
new Uri(endpoint),
apiKey);
// 4.构建内核
Kernel kernel = builder.Build();
//5. 对话功能(流式)
await StartStreamingChatSession(kernel);
//6. 对话功能(非流式)
//await StartChatSession(kernel);
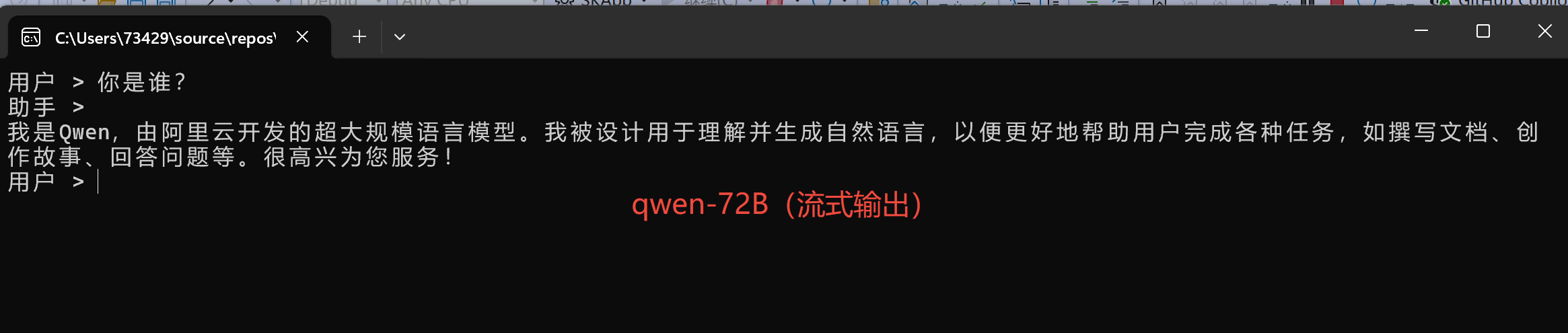
3.5 GLM对接示例
csharp
var modelConfig = Global.CurModelContext("glm-4-flash");
// 1. 填充OpenAI格式LLM调用参数值
var modelId = modelConfig.Model;
var endpoint = modelConfig.EndpointKey;
var apiKey = modelConfig.ApiKey;
// 2. 创建一个OpenAI聊天完成的内核
var builder = Kernel.CreateBuilder()
.AddOpenAIChatCompletion(modelId,
new Uri(endpoint),
apiKey);
// 4.构建内核
Kernel kernel = builder.Build();
//5. 对话功能(流式)
await StartStreamingChatSession(kernel);
//6. 对话功能(非流式)
//await StartChatSession(kernel);
四、常见问题排查
-
401 鉴权失败
- 检查 API Key 有效性
- 确认密钥传递方式符合平台要求
-
模型响应超时
-
检查网络连通性
-
确认 endpoint 配置正确
-
-
输出格式异常
-
调整 temperature 参数
-
检查 max_tokens 限制
-
五、总结
通过 Semantic Kernel 的统一接口,开发者可以快速实现国内主流大模型的集成。建议根据实际需求选择模型,并充分利用SK的插件系统、记忆机制和工具调用特性构建企业级AI应用。
- 优先选用兼容 OpenAI 格式的模型
- 对于特殊接口的模型需实现自定义OpenAI 格式封装