一、项目背景
在本次IP Lab项目中,我们模拟了游戏"Lego's Life of George"的玩法。游戏要求玩家在屏幕短暂展示图像后,凭借记忆重建乐高结构。我们面临的任务是处理一系列存在各种缺陷的图像,像噪声干扰、旋转以及投影问题等,利用MATLAB开发程序对这些图像进行预处理,并准确生成代表16个方块颜色的字符串数组。项目选用了"images2"数据集开展图像处理工作。
二、方法与函数实现
(一)LoadImage函数
该函数用于处理图像输入,主要作用是将unit8
格式的图像转换为double
类型,这样做能提升后续MATLAB图像处理操作的精度。如果输入图像并非unit8
格式,函数会给出错误提示。示例代码如下:
matlab
function [ image] = LoadImage( filename )
%function to load the image and convert it to type double
image=imread(filename); %read the image file
if isa(image,'uint8')%check if the image is type unit8
image=double(image)/255; %change the image type to double
else
error('The image is of unknown type');%return error message if image is not type unit8
end
end
(二)findCircles函数
findCircles
函数以double
类型图像作为输入,其核心功能是定位图像中圆形的坐标(圆心和半径),并借助MATLAB的viscircles
命令在图像上直观标记出检测到的圆形。在实际测试中,该函数识别圆形的成功率高达100%。代码实现如下:
matlab
function [centers,radii] = findCircles(image)
[Centers,radii]= imfindcircles(image,[20 25],'ObjectPolarity','dark','Sensitivity',0.92,'Method','twostage'); %Detect and find the Circles with their centers and Radii.
circle= viscircles(Centers,radii) %Draw the Cricles boundaries on the image.
centers=[Centers] %centers of the circles
radii=[radii];%raddii of the circles
end
(三)correctImage函数
correctImage
函数旨在校正图像的旋转和投影问题。它以原始图像(org_1.png
)中四个圆形的质心作为参考点,通过fitgeotrans
函数依据这些参考点调整图像方向,同时保持图像大小不变。针对投影或旋转图像中findCircles
函数无法准确检测圆形的问题,采取了一系列手动干预步骤。示例代码如下:
matlab
function correctedImage= correctImage(filenmae)
%function that fix the projections/Rotations
orgImage=LoadImage(filenmae);
BandWimage=~im2bw(orgImage,0.5);%converting the image to black and white
BandWfill=imfill(BandWimage,'holes');%filling any holes in the image
medianFilt=medfilt2(BandWfill);%filtering using a median filter
connectedComps=bwconncomp(medianFilt);%finding the connceted components in the image
stats=regionprops(medianFilt,'Area','Centroid');%getting stats of the image(area,centroid)
Areas=[stats.Area];
Circles=zeros(connectedComps.ImageSize);%Isolating the circles
for p = 1:connectedComps.NumObjects
if stats(p).Area<max(Areas)
Circles(connectedComps.PixelIdxList{p}) = 1;
end
end
circlesLabeled=bwlabel(Circles, 8);%returning the label matrix L for 8 connceted objects (labeling the circles).
circlesProps=regionprops(circlesLabeled,'Area','Centroid');%Stats of the circles
circlesCentroids=[circlesProps.Centroid];%centroids of the circles
varyingPoints=reshape(circlesCentroids,2,[]);%seting centroids of the image as reference points for transformation.
MovingPointsT=transpose(varyingPoints);%transposing the matrix to get it in the correct form
staticPoints=flip(findCircles(LoadImage('org_1.png')));%Setting the fixed points as centroids of org_1.png
transformation=fitgeotrans(MovingPointsT,staticPoints,'Projective');%applying the transformation
Reference=imref2d(size(LoadImage('org_1.png')));%referencing the size of org_1.png
correctedImage=imwarp(orgImage,transformation,'OutputView',Reference);%determ ining pixel values for output image by mapping locations of the output to the input image
end
(四)findColours函数
findColours
函数负责处理double
类型图像,并生成代表16个方块颜色的字符串矩阵。由于部分图像存在噪声,为实现精确的颜色检测,进行了腐蚀、阈值处理、滤波和对比度调整等额外预处理操作。代码实现如下:
matlab
function colours=findColours(image)
%function to find the colours of the squares
image = imerode(image,ones(5));%erroding the image
image = medfilt3(image,[11 11 1]); % median filter to suppress noise
image = imadjust(image,stretchlim(image,0.05)); % increase contrast of the image
figure(),imshow(image)
imageMask= rgb2gray(image)>0.08; %converting the image into greyscale
imageMask = bwareaopen(imageMask,100); % removing positive specks
imageMask = ~bwareaopen(~imageMask,100); % removing negative specks
imageMask = imclearborder(imageMask); % removing outer white region
imageMask = imerode(imageMask,ones(10)); % eroding to exclude edge effects
% segmenting the image
[L N] = bwlabel(imageMask);
% getting average color in each image mask region
maskColors = zeros(N,3);
for p = 1:N % stepping throughout the patches
imgmask = L==p;
mask= image(imgmask(:,:,[1 1 1]));
maskColors(p,:) = mean(reshape(mask,[],3),1);
end
% trying to snap the centers to a grid
Stats = regionprops(imageMask,'centroid'); %obtaining the centroids
Centroids = vertcat(Stats.Centroid);%concatenatoin
centroidlimits = [min(Centroids,[],1); max(Centroids,[],1)];
Centroids= round((Centroids-centroidlimits(1,:))./range(centroidlimits,1)*3 + 1);
% reordering colour samples
index = sub2ind([4 4],Centroids(:,2),Centroids(:,1));
maskColors(index,:) = maskColors;
% specify colour names and their references
colorNames = {'white','red','green','blue','yellow'};
colorReferences = [1 1 1; 1 0 0; 0 1 0; 0 0 1; 1 1 0];
% finding color distances in RGB
distance = maskColors - permute(colorReferences,[3 2 1]);
distance = squeeze(sum(distance.^2,2));
% finding index of closest match for each patch
[~,index] = min(distance,[],2);
% looking up colour names and returning a matrix of colour names
colours = reshape(colorNames(index),4,4)
end
(五)colourMatrix函数
colourMatrix
函数作为主集成函数,对数据集中的每一幅图像调用上述三个函数(LoadImage
、findCircles
和findColours
) 。在处理的30幅图像中,该函数成功识别了28幅图像的颜色,但在rot_2
和proj_1
这两幅图像中,有3个方块颜色误分类,导致这两幅图像的错误率为18.75%(3/16个方块)。代码如下:
matlab
%Function which calls other functions
%reading the Image and converting it to type double, then fixing the image
%for any projections or rotations, also finding the circles
correctedImage=correctImage(filename);%fixing projections and rotations
figure(),imshow(correctedImage)
findCircles(correctedImage);%finding the circles
%Find The Colours of the Squares
colorRecognition=findColours(correctedImage);
finalImage=colorRecognition;
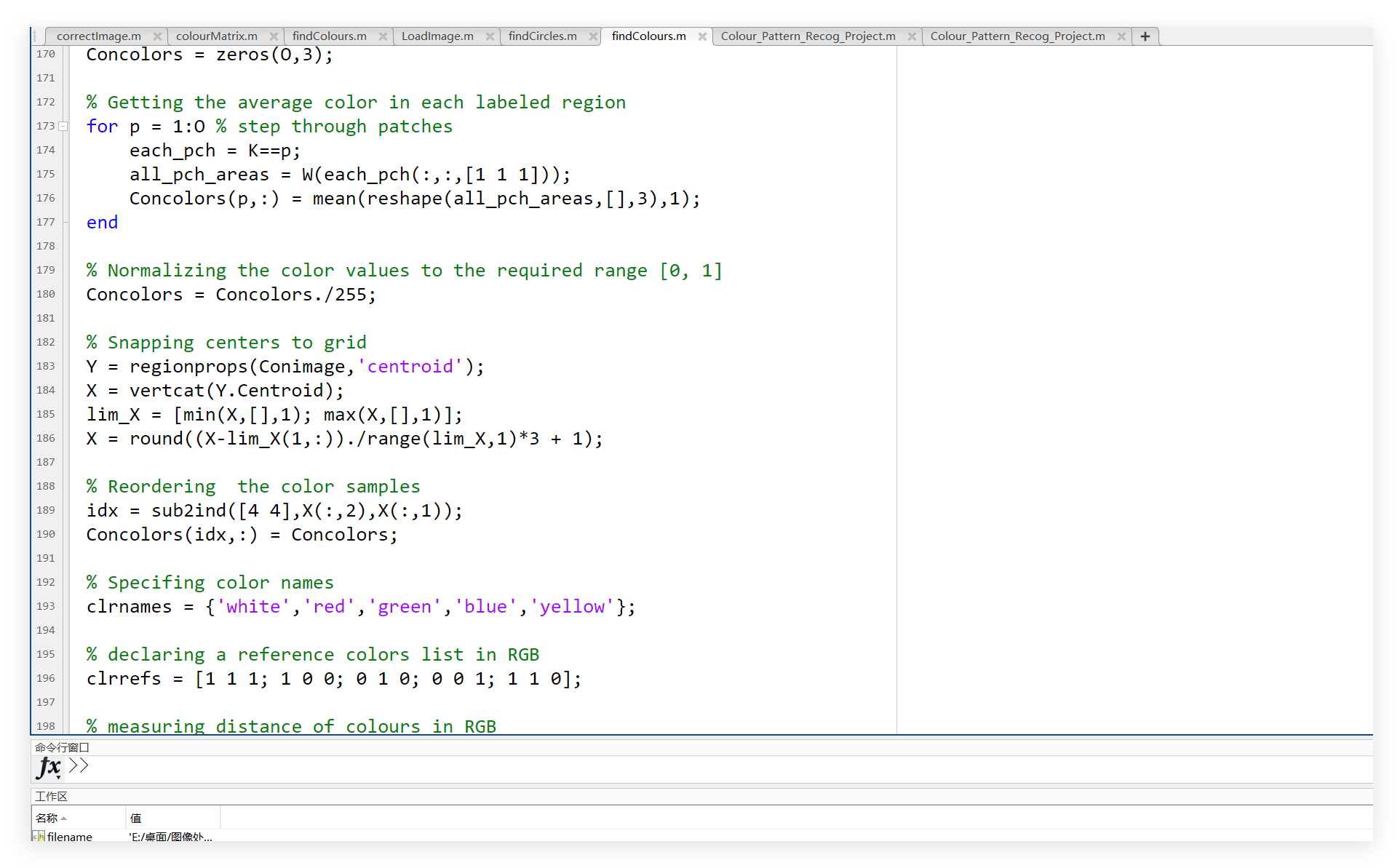
三、真实照片处理建议
项目中处理的"images_2"数据集是模拟图像,在查看真实照片后,为提升照片质量,提出以下建议:
- 固定拍摄角度:所有图像采用相同拍摄角度,避免因角度差异带来的图像变形问题。
- 统一光源:保证良好的光照条件,消除阴影影响,使图像色彩更真实、清晰。
- 更换优质相机:使用像素质量更高的相机,减少图像模糊,提高图像整体质量。
- 确保纸张平整:将图像放置在平坦表面,保证纸张笔直,防止图像出现扭曲。
四、项目总结
本项目运用MATLAB实现了识别图像中方块颜色的核心目标,且准确率较高。在处理的30幅图像中,28幅颜色识别准确率达100%,总体准确率为28/30 = 93.3%。在圆形检测以及校正旋转或投影图像方向方面,开发的函数成功率均为100%。通过此次项目,积累了丰富的MATLAB图像处理经验,同时也认识到在处理真实图像时还需进一步优化算法,以应对更复杂的图像场景。
需要代码可以联系