📁【开源解析】基于Python的智能文件备份工具开发实战:从定时备份到托盘监控

🌈 个人主页:创客白泽 - CSDN博客
🔥 系列专栏:🐍《Python开源项目实战》
💡 热爱不止于代码,热情源自每一个灵感闪现的夜晚。愿以开源之火,点亮前行之路。
👍 如果觉得这篇文章有帮助,欢迎您一键三连,分享给更多人哦
概述
在数字化时代,数据备份已成为个人和企业数据管理的重要环节。本文将详细介绍如何使用Python开发一款功能全面 的桌面级文件备份工具,该工具不仅支持即时备份 ,还能实现定时自动备份 、增量备份 等专业功能,并具备系统托盘驻留 能力。通过tkinter
+ttkbootstrap
构建现代化UI界面,结合pystray
实现后台运行,是Python GUI开发的经典案例。
功能亮点
- 双目录选择:可视化选择源目录和目标目录
- 三种备份模式 :
- 立即执行备份
- 每日/每周定时备份
- 精确到分钟的自定义时间备份
- 增量备份机制:仅复制新增或修改过的文件
- 实时日志系统:彩色分级日志输出
- 进度可视化:带条纹动画的进度条
- 托盘驻留:最小化到系统托盘持续运行
- 异常处理:完善的错误捕获和提示机制
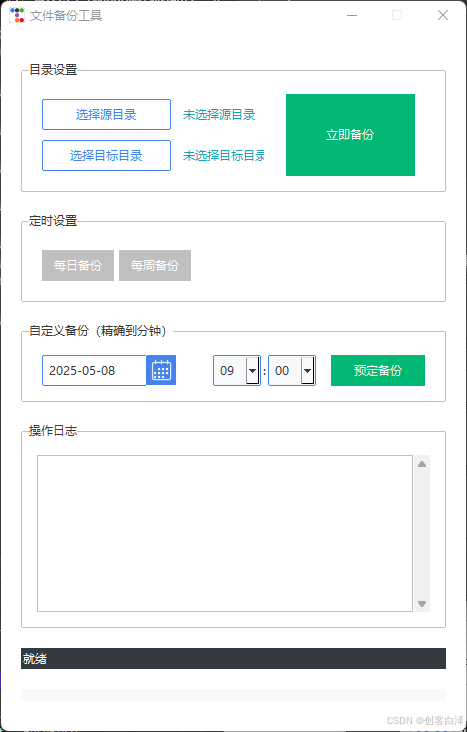
技术架构
主界面 目录选择模块 定时设置模块 日志显示模块 每日定时 每周定时 精确时间 备份引擎 文件遍历 增量判断 文件复制 托盘图标
核心代码解析
1. 增量备份实现
python
def execute_backup(self):
for root, dirs, files in os.walk(self.source_path):
rel_path = os.path.relpath(root, self.source_path)
dest_path = os.path.join(self.dest_path, rel_path)
os.makedirs(dest_path, exist_ok=True)
for file in files:
src_file = os.path.join(root, file)
dest_file = os.path.join(dest_path, file)
# 增量判断逻辑
if not os.path.exists(dest_file):
need_copy = True # 新文件
else:
src_mtime = os.path.getmtime(src_file)
dest_mtime = os.path.getmtime(dest_file)
need_copy = src_mtime > dest_mtime # 修改时间比对
这段代码实现了备份工具的核心功能------增量备份。通过对比源文件和目标文件的修改时间,仅当源文件较新时才执行复制操作,大幅提升备份效率。
2. 定时任务调度
python
def calculate_next_run(self, hour, minute, weekday=None):
now = datetime.now()
if weekday is not None: # 每周模式
days_ahead = (weekday - now.weekday()) % 7
next_date = now + timedelta(days=days_ahead)
next_run = next_date.replace(hour=hour, minute=minute, second=0)
else: # 每日模式
next_run = now.replace(hour=hour, minute=minute, second=0)
if next_run < now:
next_run += timedelta(days=1)
return next_run
该算法实现了智能的下一次运行时间计算,支持按日和按周两种循环模式,确保定时任务准确执行。
3. 托盘图标实现
python
def create_tray_icon(self):
image = Image.new('RGBA', (64, 64), (255, 255, 255, 0))
draw = ImageDraw.Draw(image)
draw.ellipse((16, 16, 48, 48), fill=(33, 150, 243))
menu = (
pystray.MenuItem("打开主界面", self.restore_window),
pystray.MenuItem("立即备份", self.start_backup_thread),
pystray.Menu.SEPARATOR,
pystray.MenuItem("退出", self.quit_app)
)
self.tray_icon = pystray.Icon("backup_tool", image, menu=menu)
threading.Thread(target=self.tray_icon.run).start()
通过Pillow
动态生成托盘图标,结合pystray
创建右键菜单,实现程序后台持续运行能力。
使用教程
基础备份操作
- 点击"选择源目录"按钮指定需要备份的文件夹
- 点击"选择目标目录"按钮设置备份存储位置
- 点击"立即备份"按钮开始执行备份
定时备份设置
- 选择定时类型(每日/每周)
- 设置具体执行时间
- 每日模式:只需设置时分
- 每周模式:需额外选择星期
- 点击"确认定时"按钮保存设置
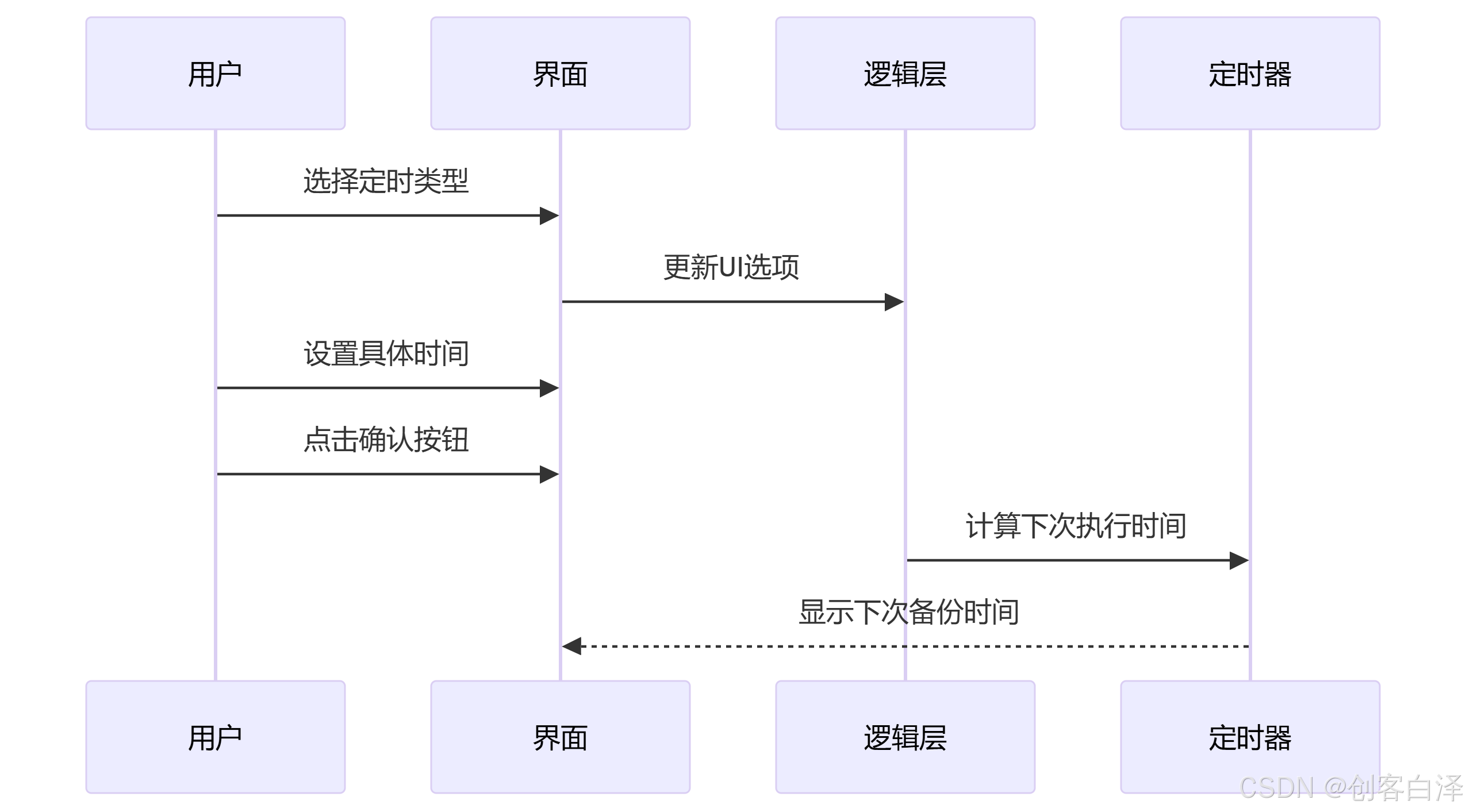
定时器逻辑层界面用户定时器逻辑层界面用户选择定时类型更新UI选项设置具体时间点击确认按钮计算下次执行时间显示下次备份时间
进阶功能解析
线程安全设计
python
def start_backup_thread(self):
if self.validate_paths():
threading.Thread(target=self.execute_backup, daemon=True).start()
采用多线程技术将耗时的备份操作放在后台执行,避免界面卡顿,同时设置为守护线程确保程序能正常退出。
日志系统实现
python
def log_message(self, message, level="INFO"):
color_map = {
"INFO": "#17a2b8",
"SUCCESS": "#28a745",
"WARNING": "#ffc107",
"ERROR": "#dc3545"
}
self.window.after(0, self._append_log, message, level, color_map.get(level))
通过after
方法实现线程安全的日志更新,不同级别日志显示不同颜色,方便问题排查。
完整源码下载
python
import tkinter as tk
import ttkbootstrap as ttk
from ttkbootstrap.constants import *
from tkinter import filedialog, messagebox, scrolledtext
import shutil
import os
import sys
from datetime import datetime, timedelta
import threading
from PIL import Image, ImageDraw
import pystray
class BackupApp:
def __init__(self):
self.window = ttk.Window(themename="litera")
self.window.title("文件备份工具")
self.window.geometry("465x700")
self.window.resizable(False, False)
self.window.minsize(465, 700)
self.window.maxsize(465, 700)
self.window.protocol("WM_DELETE_WINDOW", self.on_close)
self.font = ("微软雅黑", 10)
self.source_path = ""
self.dest_path = ""
self.schedule_type = tk.StringVar(value='')
self.scheduled_job = None
self.current_schedule = {}
main_frame = ttk.Frame(self.window, padding=20)
main_frame.pack(fill=tk.BOTH, expand=True)
dir_frame = ttk.Labelframe(main_frame, text="目录设置", padding=15)
dir_frame.pack(fill=tk.X, pady=10)
dir_frame.grid_columnconfigure(0, weight=0)
dir_frame.grid_columnconfigure(1, weight=1)
dir_frame.grid_columnconfigure(2, weight=0)
ttk.Button(
dir_frame,
text="选择源目录",
command=self.select_source,
bootstyle="primary-outline",
width=15
).grid(row=0, column=0, padx=5, pady=5, sticky="w")
self.source_label = ttk.Label(dir_frame, text="未选择源目录", bootstyle="info")
self.source_label.grid(row=0, column=1, padx=5, sticky="w")
ttk.Button(
dir_frame,
text="选择目标目录",
command=self.select_destination,
bootstyle="primary-outline",
width=15
).grid(row=1, column=0, padx=5, pady=5, sticky="w")
self.dest_label = ttk.Label(dir_frame, text="未选择目标目录", bootstyle="info")
self.dest_label.grid(row=1, column=1, padx=5, sticky="w")
ttk.Button(
dir_frame,
text="立即备份",
command=self.start_backup_thread,
bootstyle="success",
width=15
).grid(row=0, column=2, rowspan=2, padx=15, sticky="ns")
schedule_frame = ttk.Labelframe(main_frame, text="定时设置", padding=15)
schedule_frame.pack(fill=tk.X, pady=10)
type_frame = ttk.Frame(schedule_frame)
type_frame.pack(fill=tk.X, pady=5)
ttk.Radiobutton(
type_frame,
text="每日备份",
variable=self.schedule_type,
value='daily',
bootstyle="info-toolbutton",
command=self.update_schedule_ui
).pack(side=tk.LEFT, padx=5)
ttk.Radiobutton(
type_frame,
text="每周备份",
variable=self.schedule_type,
value='weekly',
bootstyle="info-toolbutton",
command=self.update_schedule_ui
).pack(side=tk.LEFT)
self.settings_container = ttk.Frame(schedule_frame)
custom_frame = ttk.Labelframe(main_frame, text="自定义备份(精确到分钟)", padding=15)
custom_frame.pack(fill=tk.X, pady=10)
custom_frame.grid_columnconfigure(0, weight=1)
custom_frame.grid_columnconfigure(1, weight=0)
custom_frame.grid_columnconfigure(2, weight=0)
self.date_entry = ttk.DateEntry(
custom_frame,
bootstyle="primary",
dateformat="%Y-%m-%d",
startdate=datetime.now().date(),
width=13
)
self.date_entry.grid(row=0, column=0, padx=5, sticky="w")
time_selector = ttk.Frame(custom_frame)
time_selector.grid(row=0, column=1, padx=(5,10), sticky="w")
self.custom_hour = ttk.Combobox(
time_selector,
width=3,
values=[f"{i:02d}" for i in range(24)],
bootstyle="primary",
state="readonly"
)
self.custom_hour.set("09")
self.custom_hour.pack(side=tk.LEFT)
ttk.Label(time_selector, text=":").pack(side=tk.LEFT)
self.custom_minute = ttk.Combobox(
time_selector,
width=3,
values=[f"{i:02d}" for i in range(60)],
bootstyle="primary",
state="readonly"
)
self.custom_minute.set("00")
self.custom_minute.pack(side=tk.LEFT)
ttk.Button(
custom_frame,
text="预定备份",
command=self.custom_backup,
bootstyle="success",
width=10
).grid(row=0, column=2, padx=5, sticky="e")
log_frame = ttk.Labelframe(main_frame, text="操作日志", padding=15)
log_frame.pack(fill=tk.BOTH, expand=True, pady=10)
self.log_area = scrolledtext.ScrolledText(
log_frame,
wrap=tk.WORD,
font=("Consolas", 9),
height=8,
bg="#f8f9fa",
fg="#495057"
)
self.log_area.pack(fill=tk.BOTH, expand=True)
self.log_area.configure(state="disabled")
self.status_label = ttk.Label(
main_frame,
text="就绪",
bootstyle="inverse-default",
font=("微软雅黑", 9)
)
self.status_label.pack(fill=tk.X, pady=10)
self.progress = ttk.Progressbar(
main_frame,
orient='horizontal',
mode='determinate',
bootstyle="success-striped",
length=500
)
self.progress.pack(pady=10)
self.window.bind("<Unmap>", self.check_minimized)
self.create_tray_icon()
def update_schedule_ui(self):
for widget in self.settings_container.winfo_children():
widget.destroy()
time_frame = ttk.Frame(self.settings_container)
time_frame.pack(side=tk.LEFT, fill=tk.X, expand=True)
ttk.Label(time_frame, text="执行时间:").pack(side=tk.LEFT)
self.hour_var = ttk.Combobox(
time_frame,
width=3,
values=[f"{i:02d}" for i in range(24)],
bootstyle="primary",
state="readonly"
)
self.hour_var.set("09")
self.hour_var.pack(side=tk.LEFT, padx=5)
ttk.Label(time_frame, text=":").pack(side=tk.LEFT)
self.minute_var = ttk.Combobox(
time_frame,
width=3,
values=[f"{i:02d}" for i in range(60)],
bootstyle="primary",
state="readonly"
)
self.minute_var.set("00")
self.minute_var.pack(side=tk.LEFT)
if self.schedule_type.get() == 'weekly':
weekday_frame = ttk.Frame(self.settings_container)
weekday_frame.pack(side=tk.LEFT, padx=20)
self.weekday_selector = ttk.Combobox(
weekday_frame,
values=["星期一","星期二","星期三","星期四","星期五","星期六","星期日"],
state="readonly",
width=8,
bootstyle="primary"
)
self.weekday_selector.pack()
self.weekday_selector.set("星期一")
ttk.Button(
self.settings_container,
text="确认定时",
command=self.set_schedule,
bootstyle="success",
width=10
).pack(side=tk.RIGHT, padx=10)
self.settings_container.pack(fill=tk.X, pady=10)
def on_close(self):
if messagebox.askokcancel("退出", "确定要退出程序吗?"):
self.quit_app()
else:
self.minimize_to_tray()
def check_minimized(self, event):
if self.window.state() == 'iconic':
self.minimize_to_tray()
def minimize_to_tray(self):
self.window.withdraw()
self.log_message("程序已最小化到托盘", "INFO")
def restore_window(self, icon=None, item=None):
self.window.deiconify()
self.window.attributes('-topmost', 1)
self.window.after(100, lambda: self.window.attributes('-topmost', 0))
self.log_message("已恢复主窗口", "SUCCESS")
def quit_app(self, icon=None, item=None):
self.tray_icon.stop()
self.window.destroy()
sys.exit()
def log_message(self, message, level="INFO"):
color_map = {"INFO": "#17a2b8", "SUCCESS": "#28a745", "WARNING": "#ffc107", "ERROR": "#dc3545"}
self.window.after(0, self._append_log, message, level, color_map.get(level, "#000000"))
def _append_log(self, message, level, color):
timestamp = datetime.now().strftime("%Y-%m-%d %H:%M:%S")
formatted_msg = f"[{timestamp}] {level}: {message}\n"
self.log_area.configure(state="normal")
self.log_area.insert(tk.END, formatted_msg)
self.log_area.tag_add(level, "end-2l linestart", "end-2l lineend")
self.log_area.tag_config(level, foreground=color)
self.log_area.see(tk.END)
self.log_area.configure(state="disabled")
def validate_paths(self):
errors = []
if not os.path.isdir(self.source_path):
errors.append("源目录无效或不存在")
if not os.path.isdir(self.dest_path):
errors.append("目标目录无效或不存在")
if errors:
messagebox.showerror("路径错误", "\n".join(errors))
return False
return True
def start_backup_thread(self):
if self.validate_paths():
self.progress["value"] = 0
threading.Thread(target=self.execute_backup, daemon=True).start()
def execute_backup(self):
try:
self.log_message("开始备份准备...", "INFO")
total_files = sum(len(files) for _, _, files in os.walk(self.source_path))
self.log_message(f"发现待处理文件总数: {total_files}", "INFO")
copied_files = 0
processed_files = 0
for root, dirs, files in os.walk(self.source_path):
rel_path = os.path.relpath(root, self.source_path)
self.log_message(f"正在处理目录: {rel_path}", "INFO")
dest_path = os.path.join(self.dest_path, rel_path)
os.makedirs(dest_path, exist_ok=True)
for file in files:
src_file = os.path.join(root, file)
dest_file = os.path.join(dest_path, file)
need_copy = False
if not os.path.exists(dest_file):
need_copy = True
self.log_message(f"新增文件: {file}", "INFO")
else:
src_mtime = os.path.getmtime(src_file)
dest_mtime = os.path.getmtime(dest_file)
if src_mtime > dest_mtime:
need_copy = True
self.log_message(f"检测到更新: {file}", "INFO")
if need_copy:
try:
shutil.copy2(src_file, dest_file)
copied_files += 1
except Exception as e:
self.log_message(f"文件 {file} 备份失败: {str(e)}", "ERROR")
processed_files += 1
self.progress["value"] = (processed_files / total_files) * 100
self.window.update()
self.progress["value"] = 100
self.window.after(0, self.show_completion_popup, copied_files)
self.window.after(0, self.reschedule_backup)
except Exception as e:
self.log_message(f"备份失败:{str(e)}", "ERROR")
messagebox.showerror("备份失败", str(e))
self.progress["value"] = 0
finally:
self.window.update_idletasks()
def show_completion_popup(self, copied_files):
popup = tk.Toplevel(self.window)
popup.title("备份完成")
popup.geometry("300x150+%d+%d" % (
self.window.winfo_x() + 100,
self.window.winfo_y() + 100
))
ttk.Label(popup, text=f"成功备份 {copied_files} 个文件", font=("微软雅黑", 12)).pack(pady=20)
popup.after(5000, popup.destroy)
close_btn = ttk.Button(
popup,
text="立即关闭",
command=popup.destroy,
bootstyle="success-outline"
)
close_btn.pack(pady=10)
def reschedule_backup(self):
if self.current_schedule:
try:
next_run = self.calculate_next_run(
self.current_schedule['hour'],
self.current_schedule['minute'],
self.current_schedule.get('weekday')
)
delay = (next_run - datetime.now()).total_seconds()
if delay > 0:
if self.scheduled_job:
self.window.after_cancel(self.scheduled_job)
self.scheduled_job = self.window.after(
int(delay * 1000),
self.start_backup_thread
)
self.status_label.configure(
text=f"下次备份时间: {next_run.strftime('%Y-%m-%d %H:%M')}",
bootstyle="inverse-info"
)
self.log_message(f"已更新下次备份时间: {next_run.strftime('%Y-%m-%d %H:%M')}", "SUCCESS")
else:
self.log_message("无效的时间间隔,定时任务未设置", "ERROR")
except Exception as e:
self.log_message(f"重新安排定时失败: {str(e)}", "ERROR")
def set_schedule(self):
try:
if self.schedule_type.get() == 'weekly' and not hasattr(self, 'weekday_selector'):
messagebox.showwarning("提示", "请选择具体星期")
return
self.current_schedule = {
'hour': int(self.hour_var.get()),
'minute': int(self.minute_var.get()),
'weekday': ["星期一","星期二","星期三","星期四","星期五","星期六","星期日"].index(
self.weekday_selector.get()) if self.schedule_type.get() == 'weekly' else None
}
self.reschedule_backup()
except Exception as e:
self.log_message(f"定时设置失败:{str(e)}", "ERROR")
messagebox.showerror("设置错误", str(e))
def calculate_next_run(self, hour, minute, weekday=None):
now = datetime.now()
if weekday is not None:
days_ahead = (weekday - now.weekday()) % 7
next_date = now + timedelta(days=days_ahead)
next_run = next_date.replace(hour=hour, minute=minute, second=0, microsecond=0)
if next_run < now:
next_run += timedelta(weeks=1)
else:
next_run = now.replace(hour=hour, minute=minute, second=0, microsecond=0)
if next_run < now:
next_run += timedelta(days=1)
return next_run
def custom_backup(self):
try:
selected_date = self.date_entry.entry.get()
hour = int(self.custom_hour.get())
minute = int(self.custom_minute.get())
target_time = datetime.strptime(selected_date, "%Y-%m-%d").replace(
hour=hour, minute=minute, second=0
)
if target_time < datetime.now():
raise ValueError("时间不能早于当前时刻")
delay = (target_time - datetime.now()).total_seconds()
if delay <= 0:
raise ValueError("定时时间必须晚于当前时间")
self.window.after(int(delay * 1000), self.start_backup_thread)
self.log_message(f"已预定备份时间:{target_time.strftime('%Y-%m-%d %H:%M')}", "SUCCESS")
self.status_label.configure(
text=f"预定备份时间: {target_time.strftime('%Y-%m-%d %H:%M')}",
bootstyle="inverse-warning"
)
except Exception as e:
self.log_message(f"预定失败:{str(e)}", "ERROR")
messagebox.showerror("设置错误", str(e))
def create_tray_icon(self):
try:
image = Image.new('RGBA', (64, 64), (255, 255, 255, 0))
draw = ImageDraw.Draw(image)
draw.ellipse((16, 16, 48, 48), fill=(33, 150, 243))
menu = (
pystray.MenuItem("打开主界面", self.restore_window),
pystray.MenuItem("立即备份", self.start_backup_thread),
pystray.Menu.SEPARATOR,
pystray.MenuItem("退出", self.quit_app)
)
self.tray_icon = pystray.Icon(
"backup_tool",
image,
"数据备份工具",
menu=menu
)
threading.Thread(target=self.tray_icon.run, daemon=True).start()
except Exception as e:
messagebox.showerror("托盘错误", f"初始化失败: {str(e)}")
sys.exit(1)
def select_source(self):
path = filedialog.askdirectory(title="选择备份源文件夹")
if path:
self.source_path = path
self.source_label.config(text=path)
self.status_label.config(text="源目录已更新", bootstyle="inverse-success")
self.log_message(f"源目录设置为: {path}", "INFO")
def select_destination(self):
path = filedialog.askdirectory(title="选择备份存储位置")
if path:
self.dest_path = path
self.dest_label.config(text=path)
self.status_label.config(text="目标目录已更新", bootstyle="inverse-success")
self.log_message(f"目标目录设置为: {path}", "INFO")
if __name__ == "__main__":
app = BackupApp()
app.window.mainloop()
文件结构:
backup_tool/
├── main.py # 主程序入口
├── requirements.txt # 依赖库清单
└── README.md # 使用说明
安装依赖:
bash
pip install -r requirements.txt
总结与扩展
本文开发的备份工具具有以下技术亮点:
- 采用现代化GUI框架ttkbootstrap,界面美观
- 完善的异常处理机制,健壮性强
- 支持后台运行,实用性强
- 增量备份算法节省时间和存储空间
扩展建议:
- 增加云存储支持(如阿里云OSS、七牛云等)
- 添加压缩备份功能
- 实现多版本备份管理
- 增加邮件通知功能
通过本项目的学习,读者可以掌握:
- Python GUI开发进阶技巧
- 定时任务调度实现
- 系统托盘程序开发
- 文件操作最佳实践
"数据备份不是可选项,而是必选项。这个工具用200行代码实现了商业软件的核心功能,值得每个开发者学习。"
相关技术栈:
- Python 3.8+
- tkinter/ttkbootstrap
- pystray
- Pillow
- shutil/os/sys
适用场景:
- 个人文档备份
- 开发项目版本存档
- 服务器重要文件备份
- 自动化运维任务