WebXR教学 09 项目7 使用python从0搭建一个简易个人博客(1)
前期设计规划
功能
呈现个人博客文章
技术选型
HTML+CSS+JS+Python+Flask
环境准备
VS Code
Python3.8
代码实现
包
bash
# 创建虚拟环境(-m 会先将模块所在路径加入 sys.path,更适合作为包的一部分执行。)
python -m venv venv
# 激活虚拟环境
venv\Scripts/activate.bat
# 设置清华源
pip config set global.index-url https://pypi.tuna.tsinghua.edu.cn/simple/
pip config set install.trusted-host pypi.tuna.tsinghua.edu.cn
# 设置阿里源
pip config set global.index-url https://mirrors.aliyun.com/pypi/simple
pip config set install.trusted-host mirrors.aliyun.com
# 安装要求的包
pip install -r requirements.txt
# 删除所有已安装包
for /f "delims=" %i in ('pip freeze') do pip uninstall -y %i
# 运行程序
python app.py
bash
Flask==2.0.1
markdown==3.3.4
Werkzeug==2.0.1
项目结构
markdown
blog/
│
├── app.py
├── templates/
│ ├── base.html
│ ├── index.html
│ └── post.html
├── static/
│ ├── style.css
│ └── script.js
└── posts/
└── sample_post.md
app.py
bash
from flask import Flask, render_template, send_from_directory # 导入Flask相关的必要模块
import markdown # 导入markdown模块,用于将markdown转换为HTML
import os # 导入os模块,用于文件系统操作
app = Flask(__name__) # 创建Flask应用实例
@app.route('/') # 设置根路由装饰器
def index(): # 定义首页视图函数
posts = [] # 创建空列表存储文章
for filename in os.listdir('posts'): # 遍历posts目录下的所有文件
if filename.endswith('.md'): # 检查是否是markdown文件
with open(os.path.join('posts', filename), 'r', encoding='utf-8') as file: # 以UTF-8编码打开文件
content = file.read() # 读取文件内容
html_content = markdown.markdown(content) # 将markdown内容转换为HTML
posts.append({'title': filename[:-3], 'content': html_content}) # 将文章标题和内容添加到列表中
return render_template('index.html', posts=posts) # 渲染index.html模板,传入文章列表
@app.route('/post/<string:filename>') # 设置文章详情页路由,接收文件名参数
def post(filename): # 定义文章详情页视图函数
with open(os.path.join('posts', filename + '.md'), 'r', encoding='utf-8') as file: # 打开对应的markdown文件
content = file.read() # 读取文件内容
html_content = markdown.markdown(content) # 将markdown内容转换为HTML
return render_template('post.html', title=filename, content=html_content) # 渲染post.html模板,传入标题和内容
@app.route('/static/<path:path>') # 设置静态文件路由
def serve_static(path): # 定义静态文件服务函数
return send_from_directory('static', path) # 从static目录提供静态文件
if __name__ == '__main__': # 判断是否直接运行此文件
app.run(debug=True) # 以调试模式运行Flask应用
templates
base.html
html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>90后小陈老师的个人网站</title>
<link rel="stylesheet" href="{{ url_for('static', filename='style.css') }}">
</head>
<body>
<header>
<h1>90后小陈老师的个人网站</h1>
<nav>
<a href="/">主页</a>
</nav>
</header>
<main>
{% block content %}{% endblock %}
</main>
<footer>
<p>© 2025 90后小陈老师的个人网站</p>
</footer>
<script src="{{ url_for('static', filename='script.js') }}"></script>
</body>
</html>
index.html
html
{% extends "base.html" %}
{% block content %}
<h2>博客文章</h2>
<ul>
{% for post in posts %}
<li><a href="/post/{{ post.title }}">{{ post.title }}</a></li>
{% endfor %}
</ul>
{% endblock %}
post.html
html
{% extends "base.html" %}
{% block content %}
<!-- <h2>{{ title }}</h2> -->
<div class="post-content">
{{ content|safe }}
</div>
{% endblock %}
static
script.js
javascript
// 当文档的 DOM 结构加载完成后执行这个事件监听器
document.addEventListener('DOMContentLoaded', function() { // 添加DOM内容加载完成事件的监听器,使用匿名函数作为回调
console.log('Page loaded'); // 在浏览器控制台输出"Page loaded"消息,用于调试和确认页面加载状态
}); // 结束事件监听器的回调函数
style.css
css
body {
font-family: Arial, sans-serif;
margin: 0;
padding: 0;
line-height: 1.6;
}
header {
background: #333;
color: #fff;
padding: 10px 0;
text-align: center;
}
nav a {
color: #fff;
margin: 0 10px;
text-decoration: none;
}
main {
max-width: 800px;
margin: 0 auto;
padding: 0 20px;
}
footer {
background: #333;
color: #fff;
text-align: center;
padding: 10px 0;
position: fixed;
width: 100%;
bottom: 0;
}
上线
https://blog.csdn.net/QQ727338622/article/details/140328195
- 宝塔中"网站"装Python环境
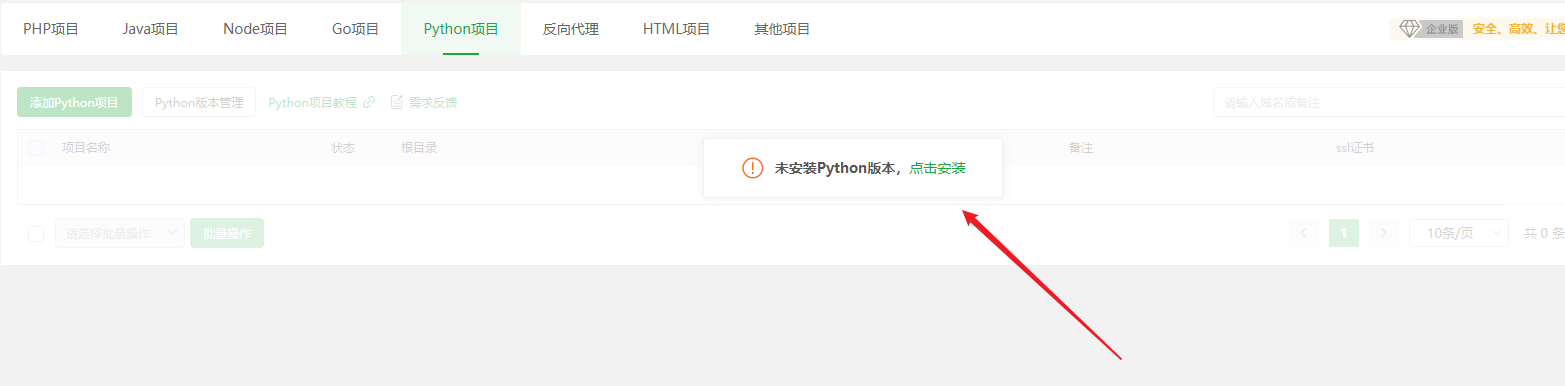
- 新建文件夹,上传项目文件
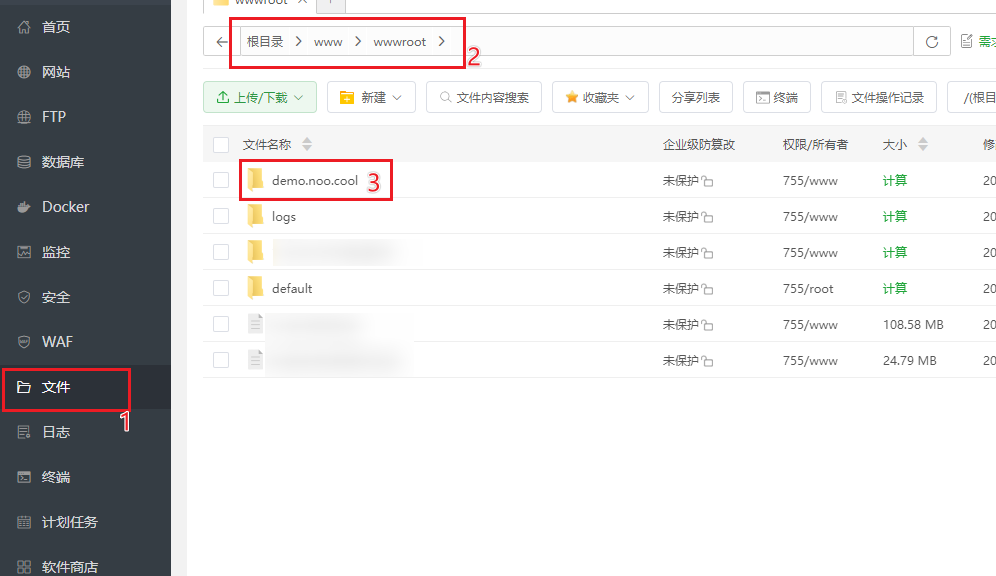
- 宝塔、服务器放行端口
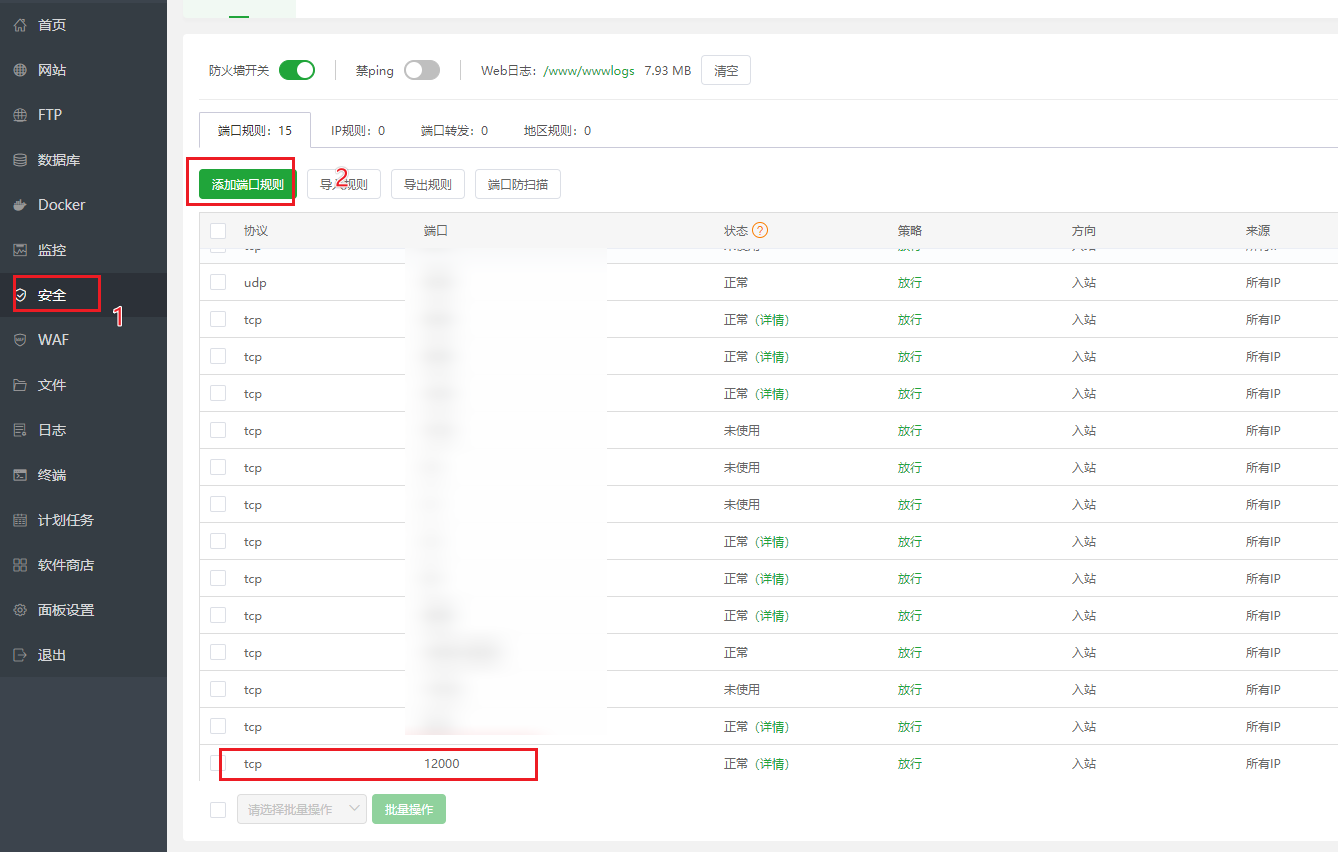
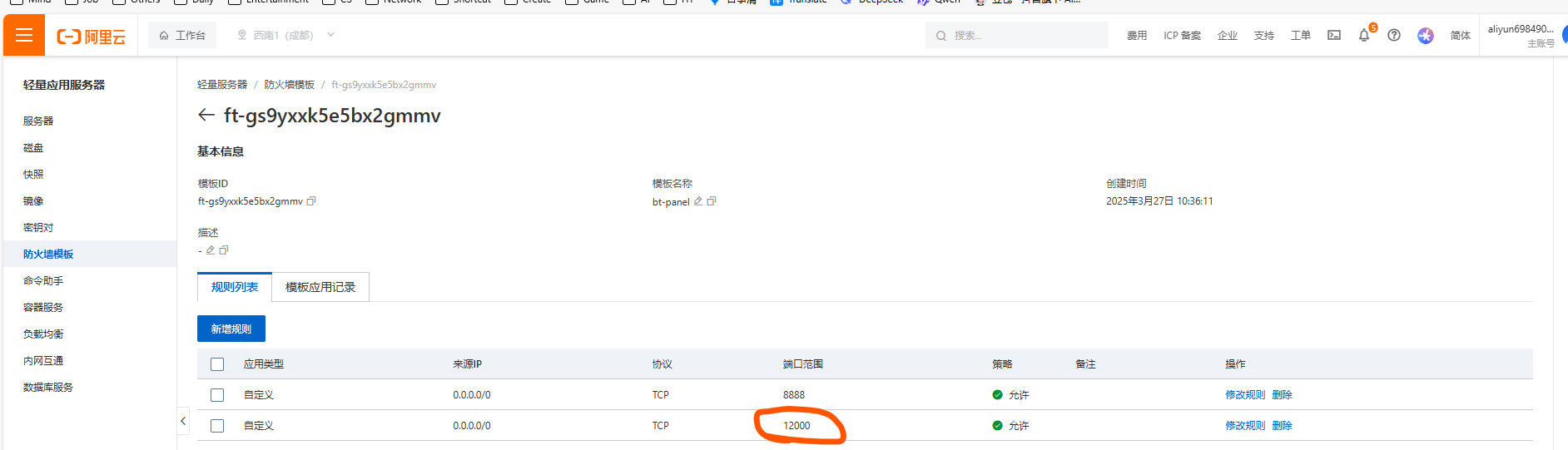
- "网站"添加Python项目
- uwsgi
- 安装依赖requirements.txt
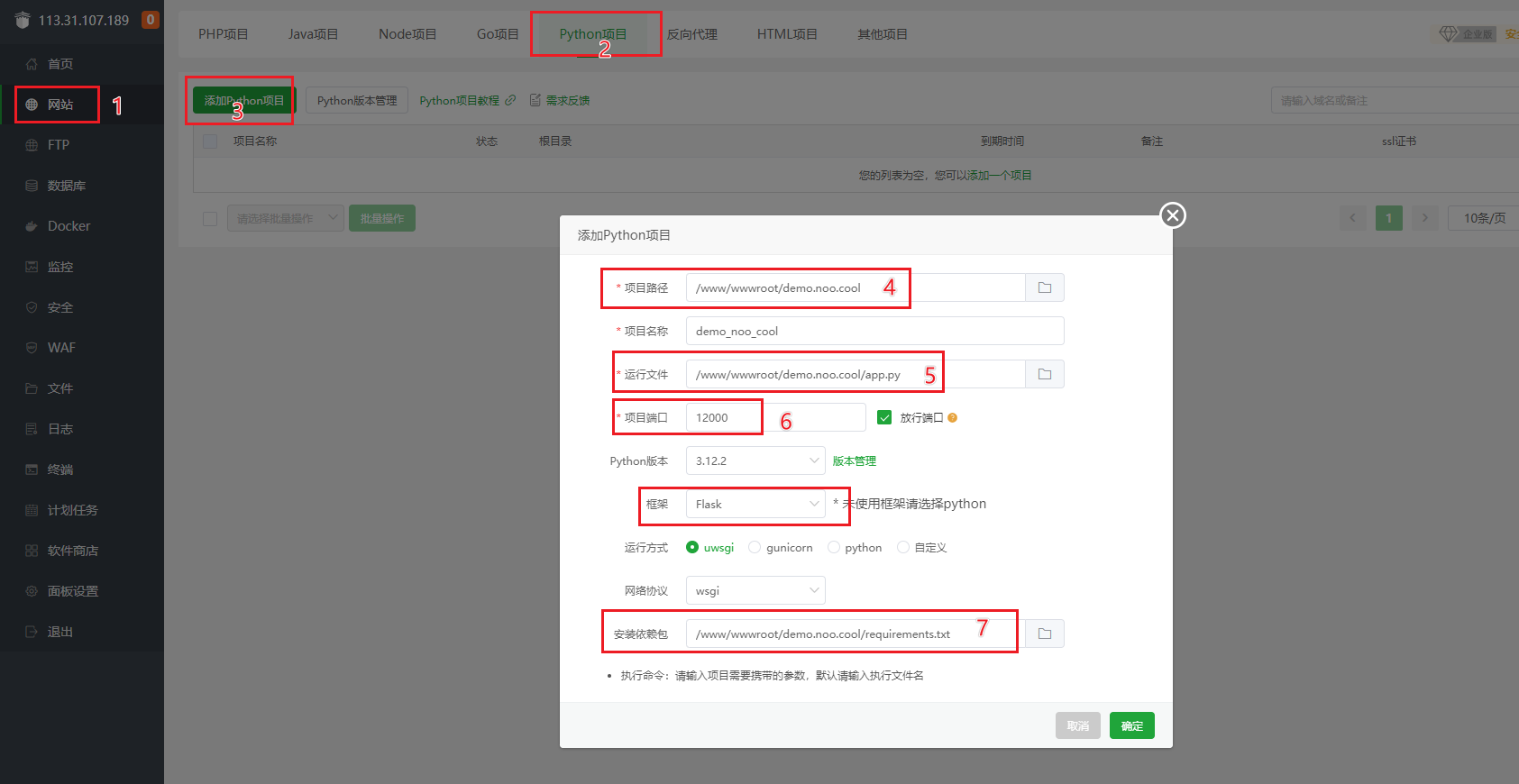