员工信息管理系统
成品展示
system-analysis-and-design: 系统分析与设计成品展示
需求
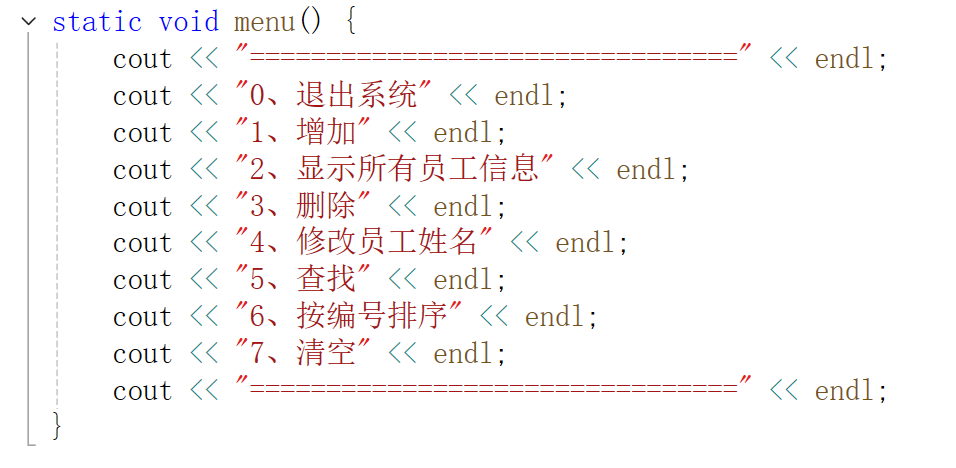
Employee.h
cpp
#pragma once
#include<iostream>
#include<string>
using namespace std;
class Employee {
public:
int id; // 编号
string name; // 姓名
string position; // 岗位
int deptId; // 部门编号
Employee();
Employee(int id, string name, string position, int deptId);
~Employee();
virtual string responsibilities() const { return "员工职责!"; };
void setName(string name);
};
class PuTong : public Employee {
public:
PuTong(int id, string name, string position, int deptId);
virtual string responsibilities() const;
};
class JingLi : public Employee {
public:
JingLi(int id, string name, string position, int deptId);
virtual string responsibilities() const;
};
class LaoBan : public Employee {
public:
LaoBan(int id, string name, string position, int deptId);
virtual string responsibilities() const;
};
Employee.cpp
cpp
#include"Employee.h"
void Employee::setName(string name) {
this->name = name;
}
Employee::Employee() :id(0), name(""), position(""), deptId(0) {
}
Employee::Employee(int id, string name, string position, int deptId) {
this->id = id;
this->name = name;
this->position = position;
this->deptId = deptId;
}
Employee::~Employee() {
}
PuTong::PuTong(int id, string name, string position, int deptId) {
this->id = id;
this->name = name;
this->position = position;
this->deptId = deptId;
}
string PuTong::responsibilities() const {
return "完成经理交给的任务.";
}
JingLi::JingLi(int id, string name, string position, int deptId) {
this->id = id;
this->name = name;
this->position = position;
this->deptId = deptId;
}
string JingLi::responsibilities() const {
return "完成老板交给的任务,并下发任务给员工.";
}
LaoBan::LaoBan(int id, string name, string position, int deptId) {
this->id = id;
this->name = name;
this->position = position;
this->deptId = deptId;
}
string LaoBan::responsibilities() const {
return "管理公司所有事务.";
}
EmployeeManager.cpp
cpp
#pragma once
#include<iostream>
#include<string>
#include<fstream>
#include "outer.h"
using namespace std;
static const int MAX_EMP_COUNT = 10;
//Employee emp[MAX_EMP_COUNT]; // 数组 类似结构体数组
Employee* emp = new Employee[MAX_EMP_COUNT]; // 动态分配的数组
Employee** p_emp = new Employee* [MAX_EMP_COUNT]; // Employee指针数组 Employee为抽象类时可以实现多态
//auto emp = make_unique<Employee[]>(MAX_EMP_COUNT);
static int emp_size = 0; // 员工数 限制emp访问区间
static const string DATA = "data.dat";
static void add_emp(Employee& e) {
if (emp_size == MAX_EMP_COUNT) { // 扩容
cout << "已经存满了!" << endl;
}
else {
emp[emp_size] = e;
p_emp[emp_size] = &e;
emp_size++;
}
}
static void print_emp(const Employee& e) {
cout << "员工[" << e.id << "] ->"
<< " 姓名: " << e.name
<< " 岗位: " << e.position
<< " 部门编号: " << e.deptId
<< " 职责:" << e.responsibilities()
<< endl;
}
static void print_emp(const Employee* const e) {
cout << "员工[" << e->id << "] ->"
<< " 姓名: " << e->name
<< " 岗位: " << e->position
<< " 部门编号: " << e->deptId
<< " 职责:" << e->responsibilities()
<< endl;
}
static void show_all_emp() {
if (emp_size <= 0) {
return;
}
cout << "所有员工信息如下:" << endl;
for (int i = 0; i < emp_size; i++) {
Employee e = emp[i];
print_emp(&e);
}
}
static Employee& search_emp(int id) {
for (int i = 0; i < emp_size; i++) {
if (emp[i].id == id) {
return emp[i];
}
}
cout << "没有找到" << endl;
exit(-1);
}
static Employee& search_emp(string name) {
for (int i = 0; i < emp_size; i++) {
if (emp[i].name == name) {
return emp[i];
}
}
cout << "没有找到" << endl;
exit(-1);
}
static bool del_emp(int id) {
for (int i = 0; i < emp_size; i++) {
if (emp[i].id == id) {
while (i < emp_size - 1) {
emp[i] = emp[i + 1];
i++;
}
emp_size--;
return true;
}
}
return false;
}
static void modify_emp(int id, string name) {
Employee& e = search_emp(id);
e.name = name;
}
static void add() {
int id; // 编号
string name; // 姓名
string position; // 岗位
int deptId; // 部门编号
while (true) {
cout << "输入-1随时退出!" << endl;
cout << "请输入编号:";
cin >> id;
if (id == -1) {
break;
}
//Employee &x = search_emp(id);
cout << "请输入姓名:";
cin >> name;
if (name == "-1") {
break;
}
pos: cout << "请输入岗位[1:普通 2:经理 3:老板]:";
cin >> position;
if (position == "-1") {
break;
}
Employee* e;
if (position == "1") {
e = new PuTong(id, name, position, 0);
}
else if (position == "2") {
e = new JingLi(id, name, position, 0);
}
else if (position == "3") {
e = new LaoBan(id, name, position, 0);
}
else {
cout << "无效输入!" << endl;
goto pos;
}
cout << "请输入部门编号:";
cin >> deptId;
if (deptId == -1) {
break;
}
//Employee* e = new Employee(id, name, position, deptId);
//Employee e(id, name, position, deptId);
e->deptId = deptId;
add_emp(*e);
show_all_emp();
cout << "选择继续添加还是退出:0 表示退出,1 表示继续添加. ";
int select;
cin >> select;
if (!select) {
break;
}
}
}
static void del() {
cout << "选择要删除的员工的编号:";
int id;
cin >> id;
bool d = del_emp(id);
if (d) {
cout << "删除成功!" << endl;
}
else {
cout << "删除失败!" << endl;
}
show_all_emp();
}
static void modify() {
cout << "选择要修改的员工的编号:";
int id;
cin >> id;
Employee &e = search_emp(id);
cout << "修改姓名:";
string name;
cin >> name;
modify_emp(id, name);
show_all_emp();
}
static void search() {
cout << "输入1按编号查找,输入2按姓名查找.";
int select;
cin >> select;
if (select == 1) {
int id;
cout << "输入编号:";
cin >> id;
Employee &e = search_emp(id);
cout << "查找到的员工信息如下:" << endl;
print_emp(&e);
} else if(select == 2) {
string name;
cout << "输入姓名:";
cin >> name;
Employee& e = search_emp(name);
cout << "查找到的员工信息如下:" << endl;
print_emp(&e);
}
}
static int comp(const void *e1, const void *e2) {
const Employee s1 = *static_cast<const Employee*>(e1);
const Employee s2 = *static_cast<const Employee*>(e2);
return s1.id - s2.id;
//return ((Employee*)e1)->id - ((Employee*)e2)->id;
}
// 泛型比较方法,比较id
template<typename T>
static int comp_id(const void* e1, const void* e2) {
const T t1 = *static_cast<const T*>(e1);
const T t2 = *static_cast<const T*>(e2);
return t1.id - t2.id;
}
static void sort() {
qsort(emp, emp_size, sizeof(Employee), comp_id<Employee>);
}
static void init() {
fstream in(DATA, ios::in);
Employee* e = new Employee;
while (in.read((char*)e, sizeof(Employee))) {
emp[emp_size++] = *e;
}
//p_emp = new Employee* [emp_size];
for (int i = 0; i < emp_size; i++) {
p_emp[i] = &emp[i];
}
//int i = 0;
//while (getline(in)) {
// int id, deptId;
// string name, position;
// in >> id >> name >> position >> deptId;
// Employee e(id, name, position, deptId);
// emp[i] = e;
//}
}
static void store_file() {
cout << "文件同步..." << endl;
fstream out(DATA, ios::out);
for (int i = 0; i < emp_size; i++) {
Employee e = emp[i];
out.write((const char*)&e, sizeof(Employee));
//out << e.id << " "
// << e.name << " "
// << e.position << " "
// << e.deptId
// << endl;
}
}
static void clear_file(const string name) {
fstream out(DATA, ios::out|ios::trunc);
out.close();
}
static void clear() {
cout << "操作不可恢复!确定清空数据吗?" << endl;
cout << "输入数字0取消!" << endl;
int n;
cin >> n;
if (!n) {
return;
}
emp_size = 0;
delete[] emp; // 删除动态分配的数组
emp = new Employee[MAX_EMP_COUNT];
//emp = NULL;
//emp = make_unique<Employee[]>(MAX_EMP_COUNT);
clear_file(DATA);
}
static void menu() {
cout << "================================" << endl;
cout << "0、退出系统" << endl;
cout << "1、增加" << endl;
cout << "2、显示所有员工信息" << endl;
cout << "3、删除" << endl;
cout << "4、修改员工姓名" << endl;
cout << "5、查找" << endl;
cout << "6、按编号排序" << endl;
cout << "7、清空" << endl;
cout << "================================" << endl;
}
void start() {
init();
int flag = 1;
int cls = 0;
label: while (flag) {
show_all_emp();
menu();
cout << "输入要执行的操作 : ";
int select;
cin >> select;
switch(select) {
case 0:
flag = 0;
goto label;
case 1:
add();
store_file();
break;
case 2:
show_all_emp();
break;
case 3:
del();
store_file();
break;
case 4:
modify();
store_file();
break;
case 5:
search();
break;
case 6:
sort();
store_file();
system("cls");
goto label;
case 7:
clear();
break;
default:
cout << "无效选项" << endl;
}
system("pause");
system("cls");
}
cout << "退出系统,欢迎使用!" << endl;
}
outer.h
cpp
#pragma once
#include "Employee.h"
// 员工信息管理系统
void start();
// 测试多态
void test_polymorphism();
测试多态
Worker.h
cpp
#pragma once
#include<string>
using namespace std;
class Worker {
public:
int id; // 编号
string name; // 姓名
string position; // 岗位
//Worker();
//Worker(int id, string name, string position);
virtual string showInfo() const = 0;
};
class Worker1 : public Worker {
public:
Worker1(int id, string name, string position);
virtual string showInfo() const;
};
class Worker2 : public Worker {
public:
Worker2(int id, string name, string position);
virtual string showInfo() const;
};
Worker.cpp
cpp
#include<string>
#include"Worker.h"
using namespace std;
Worker1::Worker1(int id, string name, string position) {
this->id = id;
this->name = name;
this->position = position;
}
string Worker1::showInfo() const {
return "完成经理交给的任务.";
}
Worker2::Worker2(int id, string name, string position) {
this->id = id;
this->name = name;
this->position = position;
}
string Worker2::showInfo() const {
return "完成老板交给的任务,并下发任务给员工.";
}
WorkerManager.cpp
cpp
#include<iostream>
#include<string>
#include"Worker.h"
using namespace std;
static int init_size = 1;
static Worker** worker_arry = new Worker*[init_size];
static int worker_size = 0;
static void add(Worker* w) {
if (worker_size == init_size) {
Worker** w_arr = new Worker * [2 * init_size];
for (int i = 0; i < worker_size; i++) {
w_arr[i] = worker_arry[i];
}
delete[] worker_arry;
worker_arry = w_arr;
}
worker_arry[worker_size++] = w;
}
static void add() {
cout << "分别输入id、name、position[1:普通、2:经理]." << endl;
Worker* w = NULL;
int id;
cout << "输入id: ";
cin >> id;
string name;
cout << "输入name: ";
cin >> name;
string position;
pos: cout << "输入position: ";
cin >> position;
if (position == "1") {
w = new Worker1(id, name, position);
}
else if (position == "2") {
w = new Worker2(id, name, position);
}
else {
cout << "position输入有误!" << endl;
goto pos;
}
add(w);
}
static void print_worker(const Worker* const w) {
cout << "id: " << w->id
<< " name: " << w->name
<< " position: " << w->position
<< " 职责: " << w->showInfo()
<< endl;
}
static void print_worker_array() {
for (int i = 0; i < worker_size; i++) {
Worker* w = worker_arry[i];
print_worker(w);
}
}
void test_polymorphism() {
bool flag = true;
while (flag) {
int s;
cout << "输入0退出. " << "输入1添加." << endl;
cin >> s;
switch (s)
{
case 0:
system("pause");
exit(1);
//flag = false;
//break;
case 1:
add();
print_worker_array();
default:
break;
}
}
}
测试类
cpp
int main(int argc, char *argv[])
{
// 面向对象设计
start();
test_polymorphism();
return 1;
}
下一步:C++标准库