目录
[61. Rotate List](#61. Rotate List)
[86. Partition List](#86. Partition List)
61. Rotate List
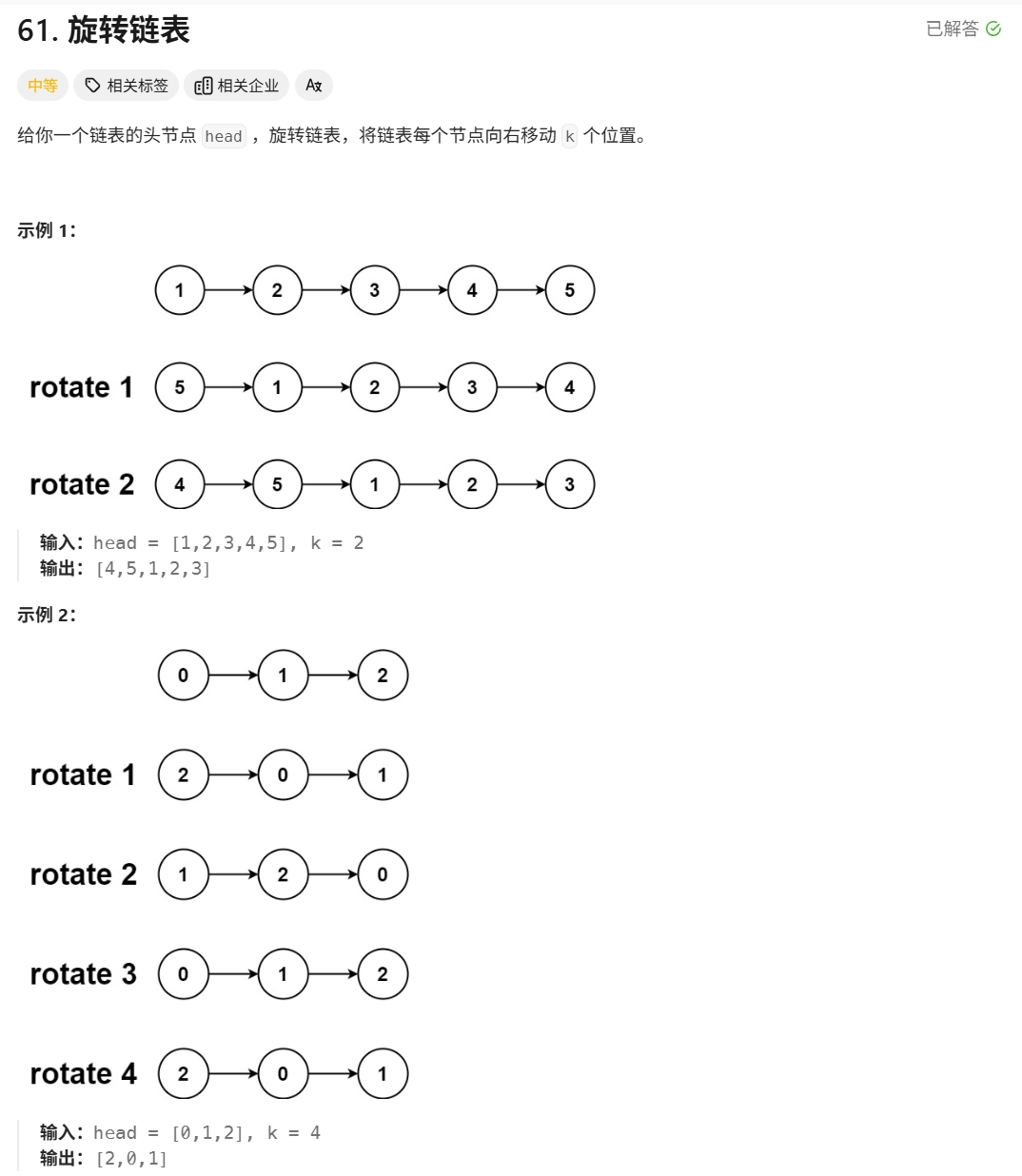
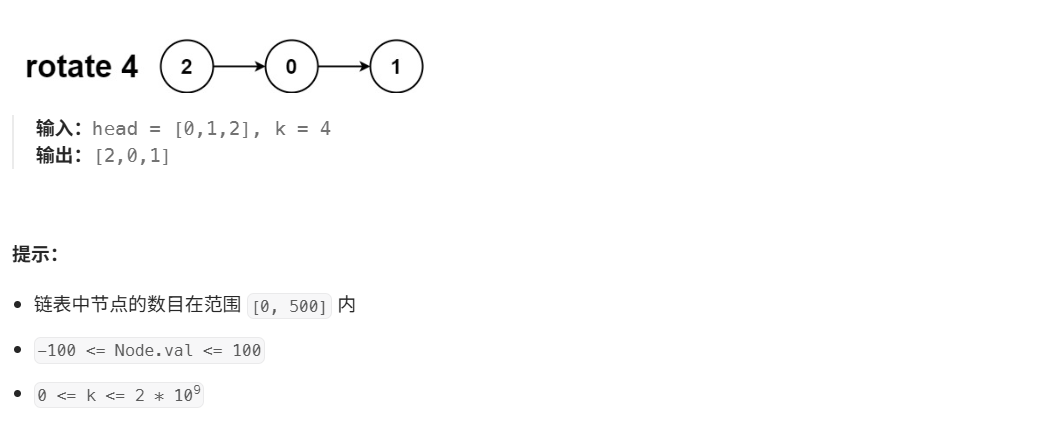
代码:
cpp
/**
* Definition for singly-linked list.
* struct ListNode {
* int val;
* ListNode *next;
* ListNode() : val(0), next(nullptr) {}
* ListNode(int x) : val(x), next(nullptr) {}
* ListNode(int x, ListNode *next) : val(x), next(next) {}
* };
*/
class Solution {
public:
ListNode* rotateRight(ListNode* head, int k) {
if(head == nullptr)
return head;
if(k == 0)
return head;
ListNode* cur = head;
int count = 0;
while(cur){
count++;
cur = cur->next;
}
k = k%count;
if(k == 0)
return head;
ListNode *pre = nullptr;
cur = head;
for(int i = 0;i < count -k;i++){
pre = cur;
cur = cur->next;
}
if(pre ==nullptr)
return head;
pre->next = nullptr;
ListNode* newhead = cur;
while(cur->next){
cur = cur->next;
}
cur->next = head;
return newhead;
}
};
86. Partition List
代码:
cpp
/**
* Definition for singly-linked list.
* struct ListNode {
* int val;
* ListNode *next;
* ListNode() : val(0), next(nullptr) {}
* ListNode(int x) : val(x), next(nullptr) {}
* ListNode(int x, ListNode *next) : val(x), next(next) {}
* };
*/
class Solution {
public:
ListNode* partition(ListNode* head, int x) {
if(head == nullptr)
return nullptr;
ListNode* left_dummy = new ListNode(-1,nullptr);
ListNode* right_dummy =new ListNode(-1,nullptr);
ListNode* leftpre = left_dummy;
ListNode* rightpre = right_dummy;
ListNode* cur = head;
while(cur){
if(cur->val < x){
leftpre->next = cur;
leftpre = cur;
}else{
rightpre->next = cur;
rightpre = cur;
}
cur = cur->next;
}
leftpre->next = right_dummy->next;
rightpre->next = nullptr;
ListNode* ans = left_dummy->next;
delete left_dummy;
delete right_dummy;
return ans;
}
};