目录
[Dispose 方法](#Dispose 方法)
[InitializeComponents 方法](#InitializeComponents 方法)
[Button 控件 (button1)](#Button 控件 (button1))
[TextBox 控件 (textBox1)](#TextBox 控件 (textBox1))
[GroupBox 控件 (groupBox1)](#GroupBox 控件 (groupBox1))
[Label 控件 (label1 至 label5)](#Label 控件 (label1 至 label5))
[OpenFileDialog 控件 (openFileDialog1)](#OpenFileDialog 控件 (openFileDialog1))
[Button 控件 (button2)](#Button 控件 (button2))
[Label 控件 (label6)](#Label 控件 (label6))
[Button 控件 (button3)](#Button 控件 (button3))
项目概述
这是一个简单的C# Windows Forms应用程序,展示了如何通过用户界面与文件系统进行交互,并利用.NET框架提供的API来获取文件的元数据,用于浏览和获取程序文件的信息。具体功能包括:
- 浏览程序文件 :允许用户通过文件对话框选择一个
.exe
文件。 - 获取并显示程序文件信息:一旦选择了文件,应用程序会读取并显示该文件的详细信息,如公司名称、产品名称、语言标志、版本号和版权声明。
- 获取当前程序文件信息:还可以显示当前运行的应用程序本身的详细信息。
- 创建窗体和控件 :
- 定义窗体类
Form1
并添加各种控件(按钮、文本框、分组框、标签等)。 - 在
InitializeComponent
方法中配置每个控件的位置、大小、文本和其他属性。
- 定义窗体类
- 事件绑定 :
- 为每个按钮绑定点击事件处理方法。
button1_Click
: 打开文件对话框并显示选中的文件路径。button2_Click
: 获取并显示所选文件的详细信息。button3_Click
: 获取并显示所选的应用程序本身的详细信息。
主要组件及功能
类定义
cspublic class Form1 : System.Windows.Forms.Form
Form1
继承自System.Windows.Forms.Form
,是整个应用程序的主要窗体类。
控件声明
csprivate Button button1; private TextBox textBox1; private GroupBox groupBox1; private OpenFileDialog openFileDialog1; private Button button2; private Label label1; private Label label2; private Label label3; private Label label4; private Label label5; private Label label6; private Button button3;
- Button: 提供用户交互按钮。
- TextBox: 显示选定文件路径。
- GroupBox: 包含多个标签用于显示文件信息。
- OpenFileDialog: 文件打开对话框,用于让用户选择文件。
- Label: 标签控件,用于显示文本信息。
构造函数
cspublic Form1() { InitializeComponent(); }
- 初始化窗体及其所有子控件。
Dispose 方法
csprotected override void Dispose(bool disposing) { if (disposing && components != null) { components.Dispose(); } base.Dispose(disposing); }
- 清理所有正在使用的资源。
InitializeComponents 方法
此方法由Visual Studio设计器生成,用于初始化窗体上的各个控件及其属性。
csprivate void InitializeComponent() { this.button1 = new System.Windows.Forms.Button(); this.textBox1 = new System.Windows.Forms.TextBox(); this.groupBox1 = new System.Windows.Forms.GroupBox(); this.label5 = new System.Windows.Forms.Label(); this.label4 = new System.Windows.Forms.Label(); this.label3 = new System.Windows.Forms.Label(); this.label2 = new System.Windows.Forms.Label(); this.label1 = new System.Windows.Forms.Label(); this.openFileDialog1 = new System.Windows.Forms.OpenFileDialog(); this.button2 = new System.Windows.Forms.Button(); this.label6 = new System.Windows.Forms.Label(); this.button3 = new System.Windows.Forms.Button(); this.groupBox1.SuspendLayout(); this.SuspendLayout(); // // button1 // this.button1.Location = new System.Drawing.Point(41, 48); this.button1.Name = "button1"; this.button1.Size = new System.Drawing.Size(112, 23); this.button1.TabIndex = 1; this.button1.Text = "浏览程序文件"; this.button1.Click += new System.EventHandler(this.button1_Click); // // textBox1 // this.textBox1.BackColor = System.Drawing.SystemColors.Control; this.textBox1.Location = new System.Drawing.Point(276, 112); this.textBox1.Name = "textBox1"; this.textBox1.ReadOnly = true; this.textBox1.Size = new System.Drawing.Size(256, 21); this.textBox1.TabIndex = 2; // // groupBox1 // this.groupBox1.Controls.Add(this.label5); this.groupBox1.Controls.Add(this.label4); this.groupBox1.Controls.Add(this.label3); this.groupBox1.Controls.Add(this.label2); this.groupBox1.Controls.Add(this.label1); this.groupBox1.Location = new System.Drawing.Point(41, 187); this.groupBox1.Name = "groupBox1"; this.groupBox1.Size = new System.Drawing.Size(602, 236); this.groupBox1.TabIndex = 3; this.groupBox1.TabStop = false; this.groupBox1.Text = "程序文件信息"; // // label5 // this.label5.Location = new System.Drawing.Point(28, 197); this.label5.Name = "label5"; this.label5.Size = new System.Drawing.Size(320, 16); this.label5.TabIndex = 4; this.label5.Text = "版权声明:"; // // label4 // this.label4.Location = new System.Drawing.Point(28, 160); this.label4.Name = "label4"; this.label4.Size = new System.Drawing.Size(320, 16); this.label4.TabIndex = 3; this.label4.Text = "版本号:"; // // label3 // this.label3.Location = new System.Drawing.Point(28, 125); this.label3.Name = "label3"; this.label3.Size = new System.Drawing.Size(320, 16); this.label3.TabIndex = 2; this.label3.Text = "语言标志:"; // // label2 // this.label2.Location = new System.Drawing.Point(28, 81); this.label2.Name = "label2"; this.label2.Size = new System.Drawing.Size(320, 16); this.label2.TabIndex = 1; this.label2.Text = "产品名称:"; // // label1 // this.label1.Location = new System.Drawing.Point(28, 28); this.label1.Name = "label1"; this.label1.Size = new System.Drawing.Size(320, 16); this.label1.TabIndex = 0; this.label1.Text = "公司名称:"; // // openFileDialog1 // this.openFileDialog1.Filter = "程序文件(*.exe)|*.exe|All files (*.*)|*.*"; // // button2 // this.button2.Location = new System.Drawing.Point(277, 48); this.button2.Name = "button2"; this.button2.Size = new System.Drawing.Size(112, 23); this.button2.TabIndex = 11; this.button2.Text = "获取程序文件信息"; this.button2.Click += new System.EventHandler(this.button2_Click); // // label6 // this.label6.Location = new System.Drawing.Point(130, 117); this.label6.Name = "label6"; this.label6.Size = new System.Drawing.Size(96, 16); this.label6.TabIndex = 12; this.label6.Text = "程序文件名称:"; // // button3 // this.button3.Location = new System.Drawing.Point(515, 48); this.button3.Name = "button3"; this.button3.Size = new System.Drawing.Size(128, 23); this.button3.TabIndex = 13; this.button3.Text = "获取本程序文件信息"; this.button3.Click += new System.EventHandler(this.button3_Click); // // Form1 // this.AutoScaleBaseSize = new System.Drawing.Size(6, 14); this.ClientSize = new System.Drawing.Size(683, 450); this.Controls.Add(this.button3); this.Controls.Add(this.label6); this.Controls.Add(this.groupBox1); this.Controls.Add(this.textBox1); this.Controls.Add(this.button1); this.Controls.Add(this.button2); this.MaximizeBox = false; this.Name = "Form1"; this.StartPosition = System.Windows.Forms.FormStartPosition.CenterScreen; this.Text = "演示获取程序文件信息"; this.groupBox1.ResumeLayout(false); this.ResumeLayout(false); this.PerformLayout(); }
控件配置详解
Button 控件 (button1
)
csthis.button1.Location = new System.Drawing.Point(41, 48); this.button1.Name = "button1"; this.button1.Size = new System.Drawing.Size(112, 23); this.button1.TabIndex = 1; this.button1.Text = "浏览程序文件"; this.button1.Click += new System.EventHandler(this.button1_Click);
- Location : 设置按钮的位置为
(41, 48)
。 - Name : 按钮的名称为
button1
。 - Size : 按钮的大小为
112x23
像素。 - TabIndex : 按钮的Tab顺序索引为
1
。 - Text: 按钮上显示的文字为"浏览程序文件"。
- Click Event : 绑定点击事件到
button1_Click
方法。
TextBox 控件 (textBox1
)
csthis.textBox1.BackColor = System.Drawing.SystemColors.Control; this.textBox1.Location = new System.Drawing.Point(276, 112); this.textBox1.Name = "textBox1"; this.textBox1.ReadOnly = true; this.textBox1.Size = new System.Drawing.Size(256, 21); this.textBox1.TabIndex = 2;
- BackColor: 文本框背景颜色为系统控制颜色。
- Location : 设置文本框的位置为
(276, 112)
。 - Name : 文本框的名称为
textBox1
。 - ReadOnly: 文本框设置为只读模式。
- Size : 文本框的大小为
256x21
像素。 - TabIndex : 文本框的Tab顺序索引为
2
。
GroupBox 控件 (groupBox1
)
csthis.groupBox1.Controls.Add(this.label5); this.groupBox1.Controls.Add(this.label4); this.groupBox1.Controls.Add(this.label3); this.groupBox1.Controls.Add(this.label2); this.groupBox1.Controls.Add(this.label1); this.groupBox1.Location = new System.Drawing.Point(41, 187); this.groupBox1.Name = "groupBox1"; this.groupBox1.Size = new System.Drawing.Size(602, 236); this.groupBox1.TabIndex = 3; this.groupBox1.TabStop = false; this.groupBox1.Text = "程序文件信息";
- Controls : 将
label1
到label5
添加到groupBox1
中。 - Location : 设置
groupBox1
的位置为(41, 187)
。 - Name :
groupBox1
的名称为groupBox1
。 - Size :
groupBox1
的大小为602x236
像素。 - TabIndex :
groupBox1
的Tab顺序索引为3
。 - TabStop : 设置为
false
表示不作为Tab顺序的一部分。 - Text :
groupBox1
上显示的文字为"程序文件信息"。
Label 控件 (label1
至 label5
)
这些标签用于显示具体的文件信息。
cs// 示例:label1 this.label1.Location = new System.Drawing.Point(28, 28); this.label1.Name = "label1"; this.label1.Size = new System.Drawing.Size(320, 16); this.label1.TabIndex = 0; this.label1.Text = "公司名称:";
- Location: 设置标签的位置。
- Name: 标签的名称。
- Size: 标签的大小。
- TabIndex: 标签的Tab顺序索引。
- Text: 标签上显示的文字。
OpenFileDialog 控件 (openFileDialog1
)
csthis.openFileDialog1.Filter = "程序文件(*.exe)|*.exe|All files (*.*)|*.*";
- Filter : 设置文件过滤器,仅显示
.exe
文件和所有文件。
Button 控件 (button2
)
csthis.button2.Location = new System.Drawing.Point(277, 48); this.button2.Name = "button2"; this.button2.Size = new System.Drawing.Size(112, 23); this.button2.TabIndex = 11; this.button2.Text = "获取程序文件信息"; this.button2.Click += new System.EventHandler(this.button2_Click);
- 同样设置了位置、名称、大小、Tab顺序和文字,并绑定了点击事件到
button2_Click
方法。
Label 控件 (label6
)
csthis.label6.Location = new System.Drawing.Point(130, 117); this.label6.Name = "label6"; this.label6.Size = new System.Drawing.Size(96, 16); this.label6.TabIndex = 12; this.label6.Text = "程序文件名称:";
- 同样设置了位置、名称、大小、Tab顺序和文字。
Button 控件 (button3
)
csthis.button3.Location = new System.Drawing.Point(515, 48); this.button3.Name = "button3"; this.button3.Size = new System.Drawing.Size(128, 23); this.button3.TabIndex = 13; this.button3.Text = "获取本程序文件信息"; this.button3.Click += new System.EventHandler(this.button3_Click);
- 同样设置了位置、名称、大小、Tab顺序和文字,并绑定了点击事件到
button3_Click
方法。
窗体配置
csthis.AutoScaleBaseSize = new System.Drawing.Size(6, 14); this.ClientSize = new System.Drawing.Size(683, 450); this.Controls.Add(this.button3); this.Controls.Add(this.label6); this.Controls.Add(this.groupBox1); this.Controls.Add(this.textBox1); this.Controls.Add(this.button1); this.Controls.Add(this.button2); this.MaximizeBox = false; this.Name = "Form1"; this.StartPosition = System.Windows.Forms.FormStartPosition.CenterScreen; this.Text = "演示获取程序文件信息";
- AutoScaleBaseSize: 设置自动缩放基准大小。
- ClientSize: 设置客户端区域的大小。
- Controls: 添加所有控件到窗体中。
- MaximizeBox: 设置不允许最大化窗口。
- Name : 窗体的名称为
Form1
。 - StartPosition: 设置窗体启动时居中显示。
- Text: 窗体标题栏显示的文字为"演示获取程序文件信息"。
事件处理方法
浏览程序文件 (button1_Click
)
csprivate void button1_Click(object sender, EventArgs e) { if (openFileDialog1.ShowDialog() == DialogResult.OK) { textBox1.Text = openFileDialog1.FileName; } }
- 当点击"浏览程序文件"按钮时,调用
openFileDialog1.ShowDialog()
弹出文件对话框。 - 如果用户选择了一个文件并点击了"确定",则将选中的文件路径赋值给
textBox1.Text
。
获取程序文件信息 (button2_Click
)
csprivate void button2_Click(object sender, EventArgs e) { string myFileName = textBox1.Text; if (myFileName.Length < 1) return; string shortName = myFileName.Substring(myFileName.LastIndexOf("\\") + 1); groupBox1.Text = shortName + "程序文件信息"; FileVersionInfo myInfo = FileVersionInfo.GetVersionInfo(myFileName); label1.Text = "公司名称:" + myInfo.CompanyName; label2.Text = "产品名称:" + myInfo.ProductName; label3.Text = "语言标志:" + myInfo.Language; label4.Text = "版本号:" + myInfo.FileVersion; label5.Text = "版权声明:" + myInfo.LegalCopyright; }
- 从
textBox1
中获取文件路径存储在myFileName
变量中。 - 如果文件路径为空,则直接返回。
- 使用
Substring
和LastIndexOf
方法提取文件名,并设置groupBox1
的标题。 - 使用
FileVersionInfo.GetVersionInfo
方法获取文件的版本信息。 - 将版本信息分别赋值给
label1
到label5
的Text
属性以显示相关信息。
获取本程序文件信息 (button3_Click
)
csprivate void button3_Click(object sender, EventArgs e) { groupBox1.Text = "显示本程序文件信息"; label1.Text = "公司名称:" + Application.CompanyName; label2.Text = "区域信息:" + Application.CurrentCulture; label3.Text = "语言标志:" + Application.CurrentInputLanguage; label4.Text = "产品名称:" + Application.ProductName; label5.Text = "产品版本:" + Application.ProductVersion; }
- 直接使用
Application
类的静态属性获取当前应用程序的版本信息。 - 将这些信息分别赋值给
label1
到label5
的Text
属性以显示相关信息。
项目截图:
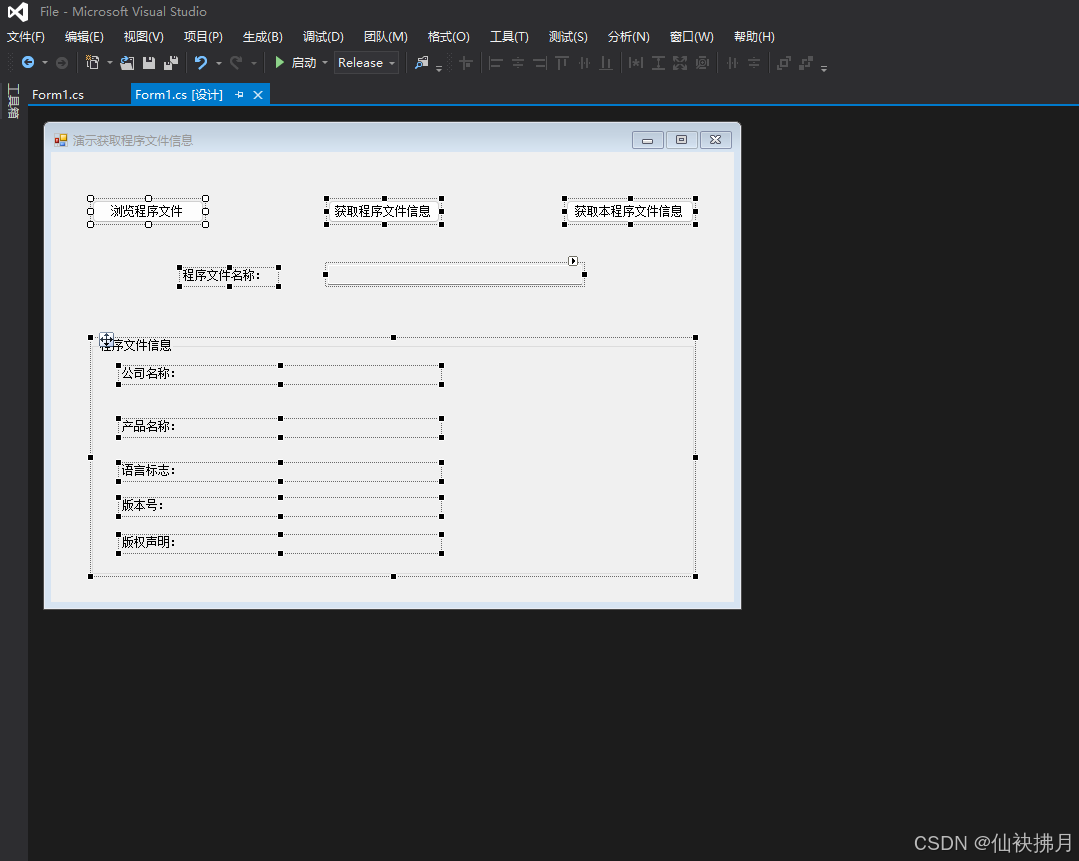
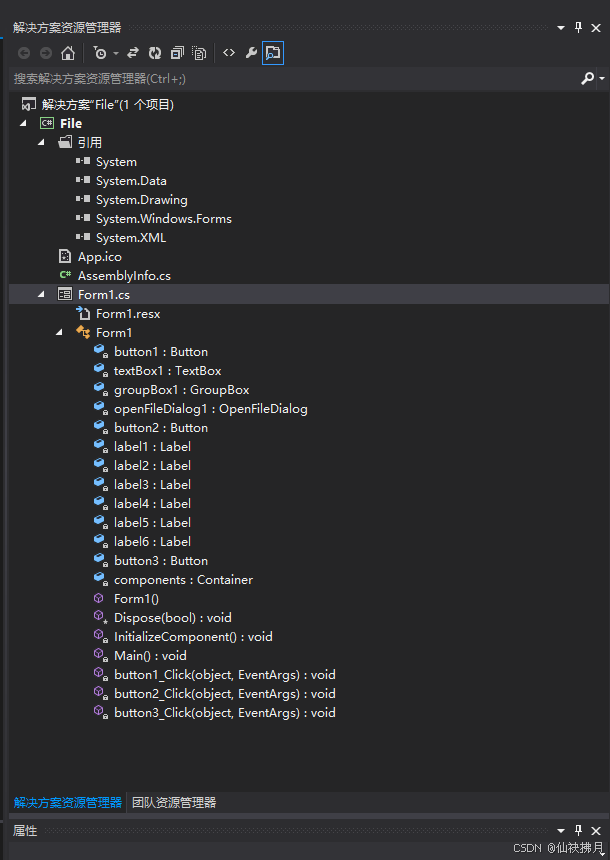
项目完整源码:
csusing System; using System.Drawing; using System.Collections; using System.ComponentModel; using System.Windows.Forms; using System.Data; using System.IO; using System.Diagnostics; namespace MyFile { /// <summary> /// Form1 的摘要说明。 /// </summary> public class Form1 : System.Windows.Forms.Form { private System.Windows.Forms.Button button1; private System.Windows.Forms.TextBox textBox1; private System.Windows.Forms.GroupBox groupBox1; private System.Windows.Forms.OpenFileDialog openFileDialog1; private System.Windows.Forms.Button button2; private System.Windows.Forms.Label label1; private System.Windows.Forms.Label label2; private System.Windows.Forms.Label label3; private System.Windows.Forms.Label label4; private System.Windows.Forms.Label label5; private System.Windows.Forms.Label label6; private System.Windows.Forms.Button button3; /// <summary> /// 必需的设计器变量。 /// </summary> private System.ComponentModel.Container components = null; public Form1() { // // Windows 窗体设计器支持所必需的 // InitializeComponent(); // // TODO: 在 InitializeComponent 调用后添加任何构造函数代码 // } /// <summary> /// 清理所有正在使用的资源。 /// </summary> protected override void Dispose( bool disposing ) { if( disposing ) { if (components != null) { components.Dispose(); } } base.Dispose( disposing ); } #region Windows 窗体设计器生成的代码 /// <summary> /// 设计器支持所需的方法 - 不要使用代码编辑器修改 /// 此方法的内容。 /// </summary> private void InitializeComponent() { this.button1 = new System.Windows.Forms.Button(); this.textBox1 = new System.Windows.Forms.TextBox(); this.groupBox1 = new System.Windows.Forms.GroupBox(); this.label5 = new System.Windows.Forms.Label(); this.label4 = new System.Windows.Forms.Label(); this.label3 = new System.Windows.Forms.Label(); this.label2 = new System.Windows.Forms.Label(); this.label1 = new System.Windows.Forms.Label(); this.openFileDialog1 = new System.Windows.Forms.OpenFileDialog(); this.button2 = new System.Windows.Forms.Button(); this.label6 = new System.Windows.Forms.Label(); this.button3 = new System.Windows.Forms.Button(); this.groupBox1.SuspendLayout(); this.SuspendLayout(); // // button1 // this.button1.Location = new System.Drawing.Point(41, 48); this.button1.Name = "button1"; this.button1.Size = new System.Drawing.Size(112, 23); this.button1.TabIndex = 1; this.button1.Text = "浏览程序文件"; this.button1.Click += new System.EventHandler(this.button1_Click); // // textBox1 // this.textBox1.BackColor = System.Drawing.SystemColors.Control; this.textBox1.Location = new System.Drawing.Point(276, 112); this.textBox1.Name = "textBox1"; this.textBox1.ReadOnly = true; this.textBox1.Size = new System.Drawing.Size(256, 21); this.textBox1.TabIndex = 2; // // groupBox1 // this.groupBox1.Controls.Add(this.label5); this.groupBox1.Controls.Add(this.label4); this.groupBox1.Controls.Add(this.label3); this.groupBox1.Controls.Add(this.label2); this.groupBox1.Controls.Add(this.label1); this.groupBox1.Location = new System.Drawing.Point(41, 187); this.groupBox1.Name = "groupBox1"; this.groupBox1.Size = new System.Drawing.Size(602, 236); this.groupBox1.TabIndex = 3; this.groupBox1.TabStop = false; this.groupBox1.Text = "程序文件信息"; // // label5 // this.label5.Location = new System.Drawing.Point(28, 197); this.label5.Name = "label5"; this.label5.Size = new System.Drawing.Size(320, 16); this.label5.TabIndex = 4; this.label5.Text = "版权声明:"; // // label4 // this.label4.Location = new System.Drawing.Point(28, 160); this.label4.Name = "label4"; this.label4.Size = new System.Drawing.Size(320, 16); this.label4.TabIndex = 3; this.label4.Text = "版本号:"; // // label3 // this.label3.Location = new System.Drawing.Point(28, 125); this.label3.Name = "label3"; this.label3.Size = new System.Drawing.Size(320, 16); this.label3.TabIndex = 2; this.label3.Text = "语言标志:"; // // label2 // this.label2.Location = new System.Drawing.Point(28, 81); this.label2.Name = "label2"; this.label2.Size = new System.Drawing.Size(320, 16); this.label2.TabIndex = 1; this.label2.Text = "产品名称:"; // // label1 // this.label1.Location = new System.Drawing.Point(28, 28); this.label1.Name = "label1"; this.label1.Size = new System.Drawing.Size(320, 16); this.label1.TabIndex = 0; this.label1.Text = "公司名称:"; // // openFileDialog1 // this.openFileDialog1.Filter = "程序文件(*.exe)|*.exe|All files (*.*)|*.*"; // // button2 // this.button2.Location = new System.Drawing.Point(277, 48); this.button2.Name = "button2"; this.button2.Size = new System.Drawing.Size(112, 23); this.button2.TabIndex = 11; this.button2.Text = "获取程序文件信息"; this.button2.Click += new System.EventHandler(this.button2_Click); // // label6 // this.label6.Location = new System.Drawing.Point(130, 117); this.label6.Name = "label6"; this.label6.Size = new System.Drawing.Size(96, 16); this.label6.TabIndex = 12; this.label6.Text = "程序文件名称:"; // // button3 // this.button3.Location = new System.Drawing.Point(515, 48); this.button3.Name = "button3"; this.button3.Size = new System.Drawing.Size(128, 23); this.button3.TabIndex = 13; this.button3.Text = "获取本程序文件信息"; this.button3.Click += new System.EventHandler(this.button3_Click); // // Form1 // this.AutoScaleBaseSize = new System.Drawing.Size(6, 14); this.ClientSize = new System.Drawing.Size(683, 450); this.Controls.Add(this.button3); this.Controls.Add(this.label6); this.Controls.Add(this.groupBox1); this.Controls.Add(this.textBox1); this.Controls.Add(this.button1); this.Controls.Add(this.button2); this.MaximizeBox = false; this.Name = "Form1"; this.StartPosition = System.Windows.Forms.FormStartPosition.CenterScreen; this.Text = "演示获取程序文件信息"; this.groupBox1.ResumeLayout(false); this.ResumeLayout(false); this.PerformLayout(); } #endregion /// <summary> /// 应用程序的主入口点。 /// </summary> [STAThread] static void Main() { Application.Run(new Form1()); } private void button1_Click(object sender, System.EventArgs e) {//浏览程序文件 if(this.openFileDialog1.ShowDialog()==DialogResult.OK) { this.textBox1.Text=this.openFileDialog1.FileName; } } private void button2_Click(object sender, System.EventArgs e) {//获取程序文件信息 string MyFileName=this.textBox1.Text; if(MyFileName.Length<1) return; string ShortName=MyFileName.Substring(MyFileName.LastIndexOf("\\")+1); this.groupBox1.Text=ShortName+"程序文件信息"; FileVersionInfo MyInfo=FileVersionInfo.GetVersionInfo(MyFileName); this.label1.Text="公司名称:"+MyInfo.CompanyName; this.label2.Text="产品名称:"+MyInfo.ProductName; this.label3.Text="语言标志:"+MyInfo.Language; this.label4.Text="版本号:"+MyInfo.FileVersion; this.label5.Text="版权声明:"+MyInfo.LegalCopyright; } private void button3_Click(object sender, System.EventArgs e) {//获取当前程序文件信息 this.groupBox1.Text="显示本程序文件信息"; this.label1.Text="公司名称:"+Application.CompanyName; this.label2.Text="区域信息:"+Application.CurrentCulture; this.label3.Text="语言标志:"+Application.CurrentInputLanguage; this.label4.Text="产品名称:"+Application.ProductName; this.label5.Text="产品版本:"+Application.ProductVersion; } } }