前言
询问DeepSeek根据自己所学到的知识来生成多个可执行的代码,为高考学子加油。最开始生成的都会有点小问题,还是需要自己调试一遍,下面就是完整的代码,当然了最后几天也不会有多少人看,都在专心的备考。
Python励志桌面程序
bash
pip install pyqt5
通过按下ESC键进行退出。
python
# 文件名:inspire_screensaver.py
import sys
import random
import time
from PyQt5.QtWidgets import QApplication, QWidget, QLabel
from PyQt5.QtCore import Qt, QTimer, QPoint
from PyQt5.QtGui import QFont, QColor, QPainter
class Screensaver(QWidget):
def __init__(self):
super().__init__()
self.texts = [
"你考的不是试,是前途和暮年的欢喜",
"关关难过关关过,前路漫漫亦灿灿",
"愿你合上笔盖的刹那,有侠客收剑入鞘的骄傲",
"笔锋所指处,皆是心之所向,愿合笔时如收刀入鞘般骄傲!",
"十二载星月为伴,今朝试锋,定当光芒万丈",
"你的考卷终将化作通向理想大学的云梯,拾级而上终见星辰",
"此刻奋笔疾书的每个字,都是未来人生的精彩伏笔",
"乾坤未定,你我皆是奔腾向前的黑马",
"愿提笔时惊风落雨,收卷日笑看云起",
"寒窗墨香终成剑,一朝出鞘动四方",
"且将新火试新茶,少年仗剑趁年华",
"鹏北海,凤朝阳,今携书剑路茫茫",
"春风得意马蹄疾,一日看尽长安花",
"不必追求完美答卷,只需写出青春无悔",
"错的每道题都是为了遇见对的人,对的每道题都是为了遇见更好的自己",
"高考只是人生车站,从容下车后还有万里山河待你丈量",
"备好2B铅笔,也请带上百分百的勇气",
"早餐要吃好,准考证别忘带,你平稳发挥就是最棒状态",
"当交卷铃声响起,整个世界都会为你的坚持鼓掌",
"把三年青春浓缩成笔尖锋芒,刺破迷茫照亮远方",
"此刻你不仅是考生,更是手握命运改写权的英雄",
"那些熬过的夜终将化作星光,铺就你的状元之路",
"少年应有鸿鹄志,当骑骏马踏平川",
"愿九月踏入的校园,正是你此刻心驰神往的方向",
"今日考场方寸地,明日天地任尔行",
"这场考试过后,你选择的世界正在向你奔来",
"现在写下的每个答案,都在勾勒未来人生的轮廓",
"金榜题名时,勿忘与恩师共赏这漫天彩霞",
"请相信:你的long类型努力终将转化为double型成功",
"人生不是单选题,但这次请坚定选择自己的最优解",
"用三年的函数积累,求导出最灿烂的极值人生",
"当交卷铃声如约而至,便是你开启新副本的入场音效",
"这场考试的隐藏奖励是:解锁无限可能的人生DLC"
]
self.initUI()
# 初始化存储数组
self.positions = [] # 存储(坐标, 颜色, 文本)
self.time_stamps = [] # 对应生成时间戳
self.directions = [] # 移动方向向量
# 运动参数配置
self.timer = QTimer(self)
self.timer.timeout.connect(self.update)
self.timer.start(40) # 刷新间隔(ms)
self.fade_speed = 1.5 # 淡出速度
self.max_trails = 15 # 最大点数
self.move_speed = 2 # 移动速度(像素/帧)
def initUI(self):
self.setWindowTitle("高考加油屏保")
self.showFullScreen()
self.setCursor(Qt.BlankCursor)
self.setStyleSheet("background: black;")
# 退出提示标签(动态适应分辨率)
self.status_label = QLabel("按 ESC 退出", self)
self.status_label.setStyleSheet("color: white; font-size: 16px;")
self.status_label.adjustSize()
self.status_label.move(10, self.height() - self.status_label.height() - 10)
def paintEvent(self, event):
painter = QPainter(self)
painter.setRenderHint(QPainter.Antialiasing)
current_time = time.time()
text_metrics = painter.fontMetrics()
text_font = QFont("微软雅黑", 20) # 字号从默认调整为20
painter.setFont(text_font)
# === 运动计算 ===
survived_indices = []
for idx in range(min(len(self.positions), len(self.time_stamps), len(self.directions))):
age = current_time - self.time_stamps[idx]
alpha = 255 - int(self.fade_speed * age * 30)
if alpha > 0: # 保留未消失的点
survived_indices.append(idx)
# 更新存活点数据(严格同步截断)
self.positions = [self.positions[i] for i in survived_indices][:self.max_trails]
self.directions = [self.directions[i] for i in survived_indices][:self.max_trails]
self.time_stamps = [self.time_stamps[i] for i in survived_indices][:self.max_trails]
# === 移动计算 ===
new_positions = []
new_directions = []
for idx in range(len(self.positions)):
(pos, color, text) = self.positions[idx]
dx, dy = self.directions[idx]
# 计算新坐标(带边界约束)
text_width = text_metrics.width(text)
text_height = text_metrics.height()
new_x = pos.x() + dx
new_y = pos.y() + dy
# 边界反弹处理
if new_x < 0 or new_x > (self.width() - text_width):
dx = -dx * 0.8
new_x = max(0, min(new_x, self.width() - text_width))
if new_y < text_height or new_y > (self.height() - text_height):
dy = -dy * 0.8
new_y = max(text_height, min(new_y, self.height() - text_height))
new_positions.append((QPoint(int(new_x), int(new_y)), color, text))
new_directions.append((dx, dy))
# 更新数据
self.positions = new_positions
self.directions = new_directions
# === 生成新点 ===
while len(self.positions) < self.max_trails:
new_text = random.choice(self.texts)
text_width = text_metrics.width(new_text)
text_height = text_metrics.height()
# 安全坐标生成(带异常处理)
try:
safe_x = random.randint(0, self.width() - text_width)
safe_y = random.randint(text_height, self.height() - text_height)
except ValueError:
safe_x = 0
safe_y = text_height
self.positions.append((
QPoint(safe_x, safe_y),
QColor(
random.randint(150, 255),
random.randint(150, 255),
random.randint(150, 255)
),
new_text
))
self.directions.append((
random.uniform(-self.move_speed, self.move_speed),
random.uniform(-self.move_speed, self.move_speed)
))
self.time_stamps.append(time.time())
# === 绘制所有点 ===
for (pos, color, text), ts in zip(self.positions, self.time_stamps):
age = current_time - ts
alpha = max(0, 255 - int(self.fade_speed * age * 50))
painter.setPen(QColor(
color.red(),
color.green(),
color.blue(),
alpha
))
painter.drawText(pos, text)
def keyPressEvent(self, event):
if event.key() == Qt.Key_Escape:
self.close()
if __name__ == "__main__":
# Windows系统内存优化
if sys.platform == 'win32':
import ctypes
ctypes.windll.kernel32.SetProcessWorkingSetSize(-1, 0x100000, 0x200000)
app = QApplication(sys.argv)
ex = Screensaver()
sys.exit(app.exec_())
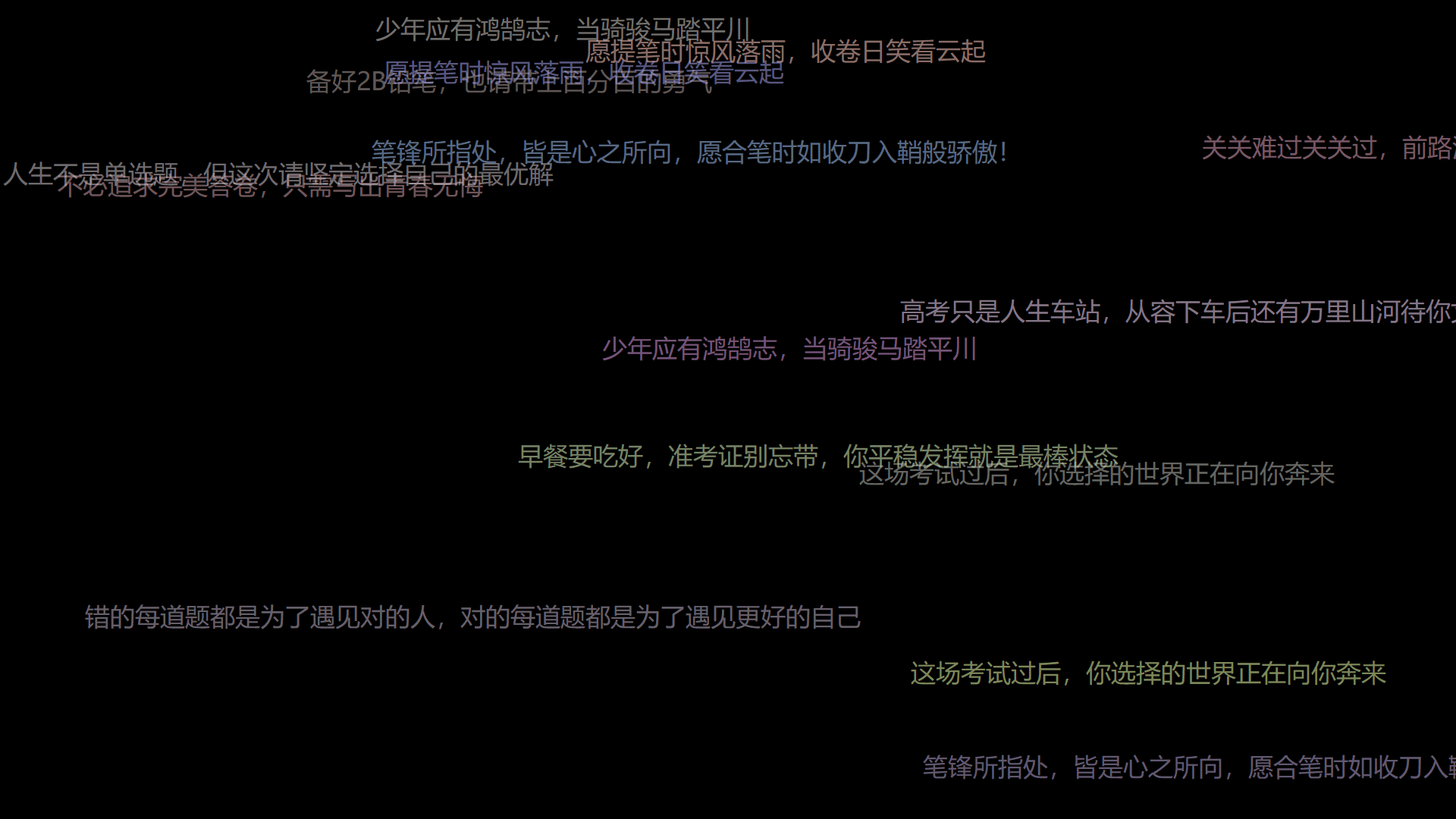
Vue3互动网页
时间设置的是const target = new Date('2025-06-07T09:00:00')
html
<!-- 文件结构 -->
<!-- index.html -->
<!DOCTYPE html>
<html>
<head>
<title>高考能量站</title>
<script src="https://unpkg.com/vue@3"></script>
<style>
body {
margin: 0;
background: linear-gradient(45deg, #1a237e, #4a148c);
height: 100vh;
overflow: hidden;
}
#app {
display: flex;
flex-direction: column;
align-items: center;
color: white;
}
.countdown {
font-size: 3em;
text-shadow: 0 0 10px rgba(255, 255, 255, 0.5);
}
.btn {
padding: 15px 30px;
background: #00e676;
border: none;
border-radius: 25px;
font-size: 1.2em;
cursor: pointer;
transition: transform 0.3s;
}
canvas {
position: fixed;
top: 0;
left: 0;
pointer-events: none;
}
</style>
</head>
<body>
<div id="app">
<h1>高考能量补给站</h1>
<div class="countdown">{{ days }}天{{ hours }}时{{ minutes }}分{{ second }}秒</div>
<button class="btn" @click="sendConfetti">获取好运</button>
<canvas ref="canvas"></canvas>
</div>
<script>
const { createApp, ref, onMounted } = Vue;
createApp({
setup() {
const canvas = ref(null)
const days = ref(0)
const hours = ref(0)
const minutes = ref(0)
const second = ref(0)
let ctx = null
// 倒计时计算
const updateTime = () => {
// 高考时间(今年是25年了)
const target = new Date('2025-06-07T09:00:00')
const now = new Date()
const diff = target - now
days.value = Math.floor(diff / (1000 * 60 * 60 * 24))
hours.value = Math.floor((diff % (1000 * 60 * 60 * 24)) / (1000 * 60 * 60))
minutes.value = Math.floor((diff % (1000 * 60 * 60)) / (1000 * 60))
second.value = Math.floor((diff % (1000 * 60)) / 1000)
}
// 彩纸特效
const sendConfetti = () => {
for (let i = 0; i < 50; i++) {
const x = Math.random() * canvas.value.width
const y = Math.random() * canvas.value.height
ctx.fillStyle = `hsl(${Math.random() * 360}, 70%, 60%)`
ctx.beginPath()
ctx.arc(x, y, 3, 0, Math.PI * 2)
ctx.fill()
}
console.log('🎉')
}
onMounted(() => {
// 初始化画布
ctx = canvas.value.getContext('2d')
canvas.value.width = window.innerWidth
canvas.value.height = window.innerHeight
// 启动计时器
setInterval(updateTime, 1000)
updateTime()
})
// 需要返回
return { days, hours, minutes, second, canvas, sendConfetti }
}
}).mount('#app')
</script>
</body>
</html>
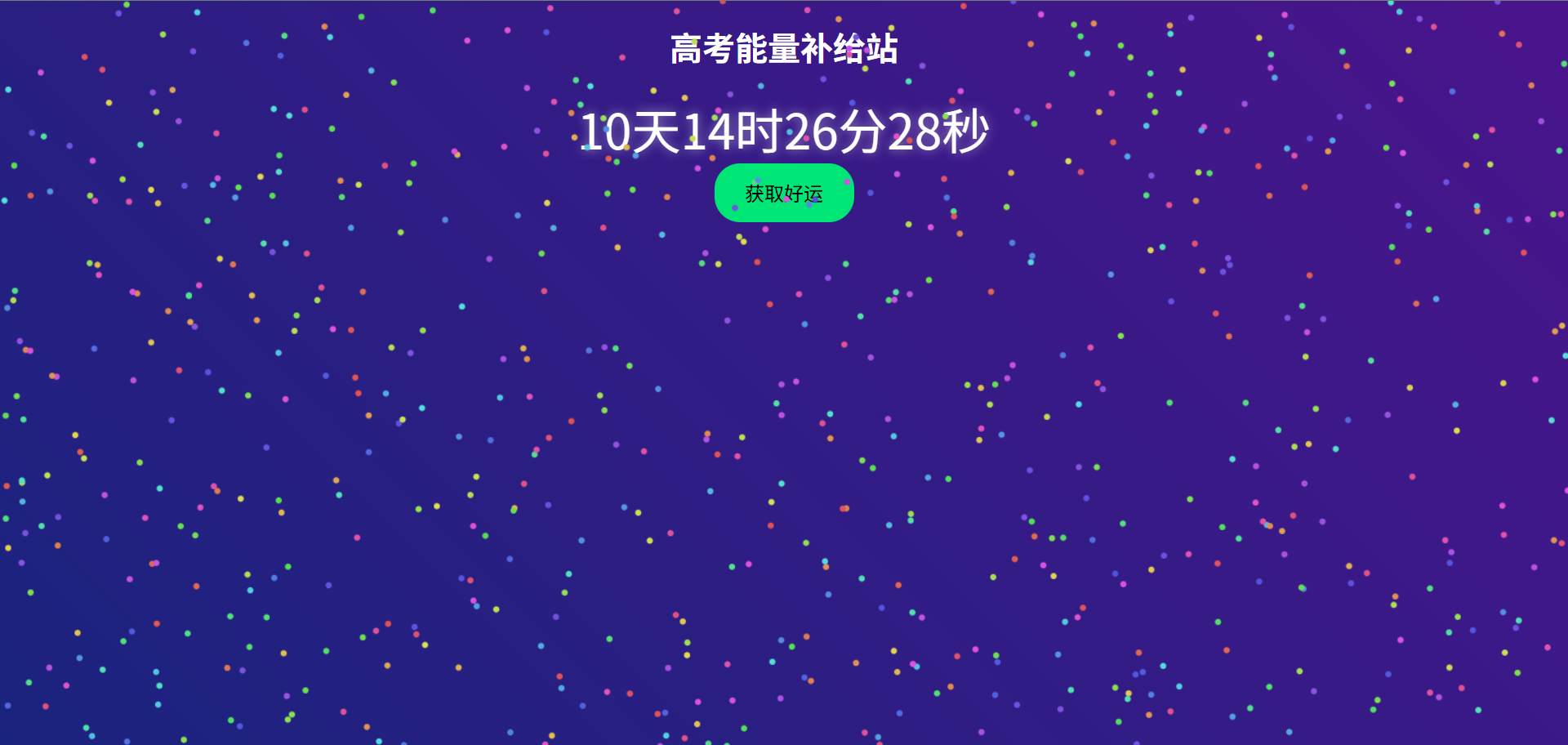
总结
暂时先这样吧,有时间再来完善。