文章目录
- [1. 数据库约束](#1. 数据库约束)
-
- [1.1 约束类型](#1.1 约束类型)
- [1.2 NOT NULL约束](#1.2 NOT NULL约束)
- [1.3 UNIQUE唯一约束](#1.3 UNIQUE唯一约束)
- [1.4 DEFAULT默认值约束](#1.4 DEFAULT默认值约束)
- [1.5 PRIMARY KEY主键约束](#1.5 PRIMARY KEY主键约束)
- [1.6 FOREIGN KEY外键约束](#1.6 FOREIGN KEY外键约束)
- [1.7 CHECK约束](#1.7 CHECK约束)
- [2. 新增](#2. 新增)
- [3. 查询](#3. 查询)
-
- [3.1 聚合查询](#3.1 聚合查询)
-
- [3.1.1 聚合函数](#3.1.1 聚合函数)
- [3.1.2 GROUP BY子句](#3.1.2 GROUP BY子句)
- [3.1.3 HAVING](#3.1.3 HAVING)
- [3.2 联合查询](#3.2 联合查询)
-
- [3.2.1 内连接](#3.2.1 内连接)
- [3.2.2 外连接](#3.2.2 外连接)
- [3.2.3 自连接](#3.2.3 自连接)
1. 数据库约束
1.1 约束类型
约束类型 | 说明 |
---|---|
NOT NULL | 指定某列不能存储NULL值 |
UNIQUE | 保证某列的每行必须有唯一的值 |
DEFAULT | 规定没有给列赋值时的默认值 |
PRIMARY KEY | 主键约束,是NOT NULL和UNIQUE的结合,确保某列(或两个列多个列的结合)有唯一标识,有助于更容易更快速地找到表中的一个特定的记录。 |
FOREIGN KEY | 外键约束,外键用来保证一个表中的数据与另一个表的数据相关联,外键约束确保了表之间的引用完整性,即一个表中的外键列值必须在另一个表的主键列中存在。 |
CHECK | 保证列中的值符合指定的条件 |
1.2 NOT NULL约束
在创建表时,可以指定某列不为空
例如:指定序号不能为空,重新建立student表
sql
create table student(
serial_number int not null,
id int,
age int,
sex varchar(2),
name varchar(32),
qq_mail varchar(20)
);
1.3 UNIQUE唯一约束
在创建表时,指定某一列为唯一的
例如:指定学号为唯一的,重新重新创建学生表
sql
drop table if exists student;
create table student(
serial_number int not null,
id int unique,
age int,
sex varchar(2),
name varchar(32),
qq_mail varchar(20)
);
1.4 DEFAULT默认值约束
指定默认值
例如:如果年龄为空,默认值为15
sql
drop table if exists student;
create table student(
serial_number int not null,
id int unique,
age int default 15,
sex varchar(2),
name varchar(32),
qq_mail varchar(20)
);
1.5 PRIMARY KEY主键约束
指定学号列为主键
sql
drop table if exists student;
create table student(
serial_number int not null,
id int primary key,
age int default 15,
sex varchar(2),
name varchar(32),
qq_mail varchar(20)
);
1.6 FOREIGN KEY外键约束
外键用于关联其他表的主键 或唯一键.
语法:
sql
foreign key (字段名) references 主表(列)
- 创建班级表,学号为主键
sql
drop table if exists classes;
create table classes(
id int primary key,
name varchar(20)
);
- 创建学生表,学号为主键,班级号为外键,一个学生对应一个班级,一个班级对应多个学生。
sql
drop table if exists student;
create table student(
serial_number int not null,
id int primary key,
class_id int,
age int default 15,
sex varchar(2),
name varchar(32),
qq_mail varchar(20),
foreign key(class_id) references classes(id)
);
1.7 CHECK约束
在MySQL中无法使用check约束,但是使用不会报错。
sql
drop table if exists student;
create table student(
serial_number int not null,
id int primary key,
class_id int,
age int default 15,
sex varchar(2),
name varchar(32),
qq_mail varchar(20),
check(sex = '男' or sex = '女'),
foreign key(class_id) references classes(id)
);
2. 新增
语法:
sql
INSERT INTO table_name [(column [, column ...])] SELECT ...
示例:创建一张用户表,有姓名、性别、年龄、qq邮箱、手机号字段,将已有的学生数据复制进来
sql
drop table if exists user;
create table user(
id int primary key,
name varchar(32),
age int,
qq_mail varchar(20),
sex varchar(2),
mobile varchar(20)
);
insert into user(name,age,sex,qq_mail) select name,age,sex,qq_mail from student;
我真是不想再建表了
3. 查询
3.1 聚合查询
3.1.1 聚合函数
常见的聚合函数有:
函数 | 说明 |
---|---|
COUNT([DISTINCT] EXPR) | 返回查询到的数据的数量 |
SUM([DISTINCT] expr) | 返回查询到的数据的总和 |
AVG([DISTINCT] expr) | 返回查询到的数据的平均值 |
MAX([DISTINCT] expr) | 返回查询到的数据的最大值 |
MIN([DISTINCT] expr) | 返回查询到的数据的最小值 |
注意:后四个使用时,不是数字没有意义。
示例:
- COUNT
统计班级一共有多少同学
sql
select count(*) from student;
select count(0) from student;
统计已知qq_mail有多少同学(不为NULL)
sql
select count(qq_mail) from student;
- SUM
求班级数学成绩的总分
sql
select sum(math) from student;
求数学成绩在80以上的学生的数学总分
sql
select sum(math) from student where math > 80;
- AVG
求zhangsan同学的平均分
sql
select avg(math + chinese + english) from student where name = 'zhangsan';
求全班的平均分
sql
select avg(math + chinese + english) from student;
- MAX
返回数学最高分
sql
select max(math) from student;
- MIN
返回数学最低分
sql
select min(math) from student;
3.1.2 GROUP BY子句
对指定列进行分组查询,将具有相同属性值的记录分组在一起,并对每个组进行聚合操作。
满足条件 :使用 GROUP BY 进行分组查
询时,SELECT 指定的字段必须是"分组依据字段",其他字段若想出现在SELECT 中则必须包含在聚合函
数中。
3.1.3 HAVING
GROUP BY 子句进行分组以后,需要对分组结果再进行条件过滤时,不能使用 WHERE 语句,而需要用HAVING
例如:建立员工表
sql
create table employee(
id int primary key,
name varchar(32),
sex varchar(2),
depart varchar(50) comment '部门',
salary int comment '薪水'
);
insert into employee(id,name,sex,depart,salary) values(1,'zhangsan','男','销售部',8000),(2,'lisi','男','技术部',12000),(3,'wangwu','女','行政部','15000'),(4,'zhaoliu','男','技术部',12500),(5,'qianqi','女','销售部',9000),(6,'xiaoba','男','行政部',15000);
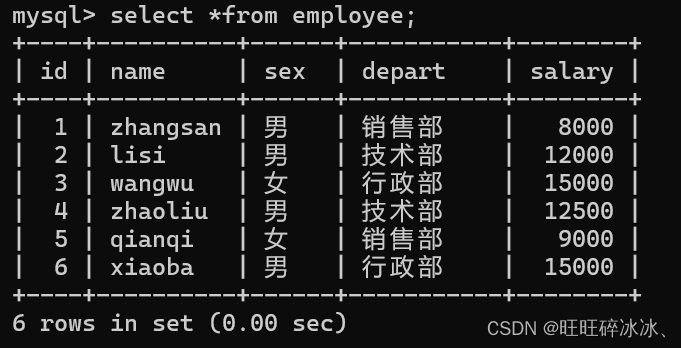
例题1:查询男女员工平均工资
sql
select sex,avg(salary) from employee group by sex;
例题2:查询各部门的总工资
sql
select depart,sum(salary) from employee group by depart;
例题3:查询姓名重复的员工信息
sql
select name from employee group by name having count(name) > 1;
3.2 联合查询
实际开发中往往数据来自不同的表,所以需要多表联合查询,需要对多张表取笛卡尔积。例如
3.2.1 内连接
语法
sql
select 字段 from 表1 别名1 [inner] join 表2 别名2 on 连接条件 and 其他条件;
select 字段 from 表1 别名1,表2 别名2 where 连接条件 and 其他条件;
例如:查询张三同学的成绩
sql
select sco.score from student stu inner join score sco on stu.id = sco.student_id and stu.name = '张三';
或者
sql
select sco.score from student stu,score sco where stu.id = sco.student_id and stu.name = '张三';
3.2.2 外连接
外连接分为左外连接 和右外连接。
如果联合查询,左侧的表完全显示就称为左外连接;右侧的表完全显示就称为右外连接。
语法:
sql
-- 左外连接,表1完全显示
select 字段名 from 表名1 left join 表名2 on 连接条件;
-- 右外连接,表2完全显示
select 字段 from 表名1 right join 表名2 on 连接条件;
例如:查询所有学生的成绩以及个人信息
左外连接
sql
select *from student stu left join score sco on stu.id = sco.student_id;
右外连接
sql
select *from student stu right join score sco on stu.id = sco.student_id;
3.2.3 自连接
即同一张表连接自身。
例如:查询成绩表中,数学成绩高于英语成绩的信息
sql
SELECT
s1.*
FROM
score s1
JOIN score s2 ON s1.student_id = s2.student_id
AND s1.score < s2.score
AND s1.course_id = 1
AND s2.course_id = 3;
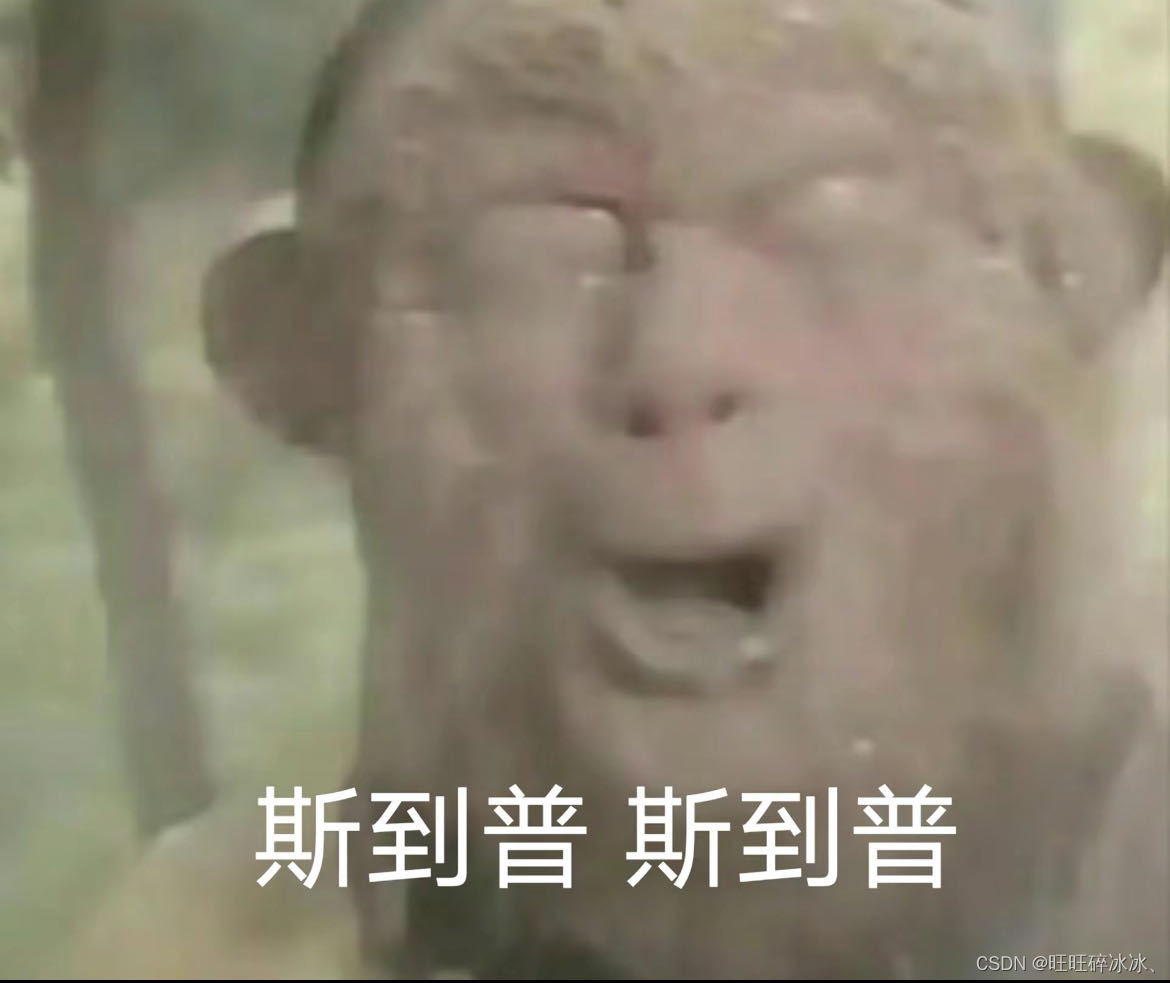
行了,连接这是不懵了,等我下篇会详细讲解,请点点关注期待我的下一篇文章
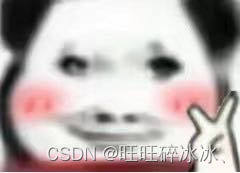