匹配响应执行状态
我们创建了测试环境
而且发送了虚拟的请求
我们接下来要进行验证
验证请求和预期值是否匹配
MVC结果匹配器
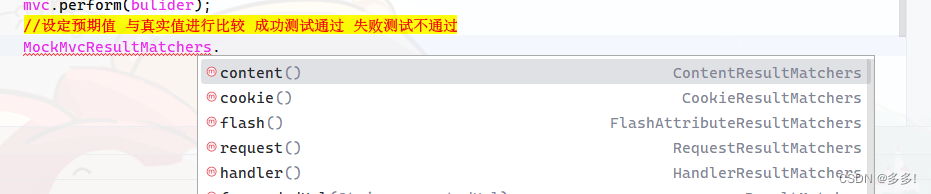
匹配上了
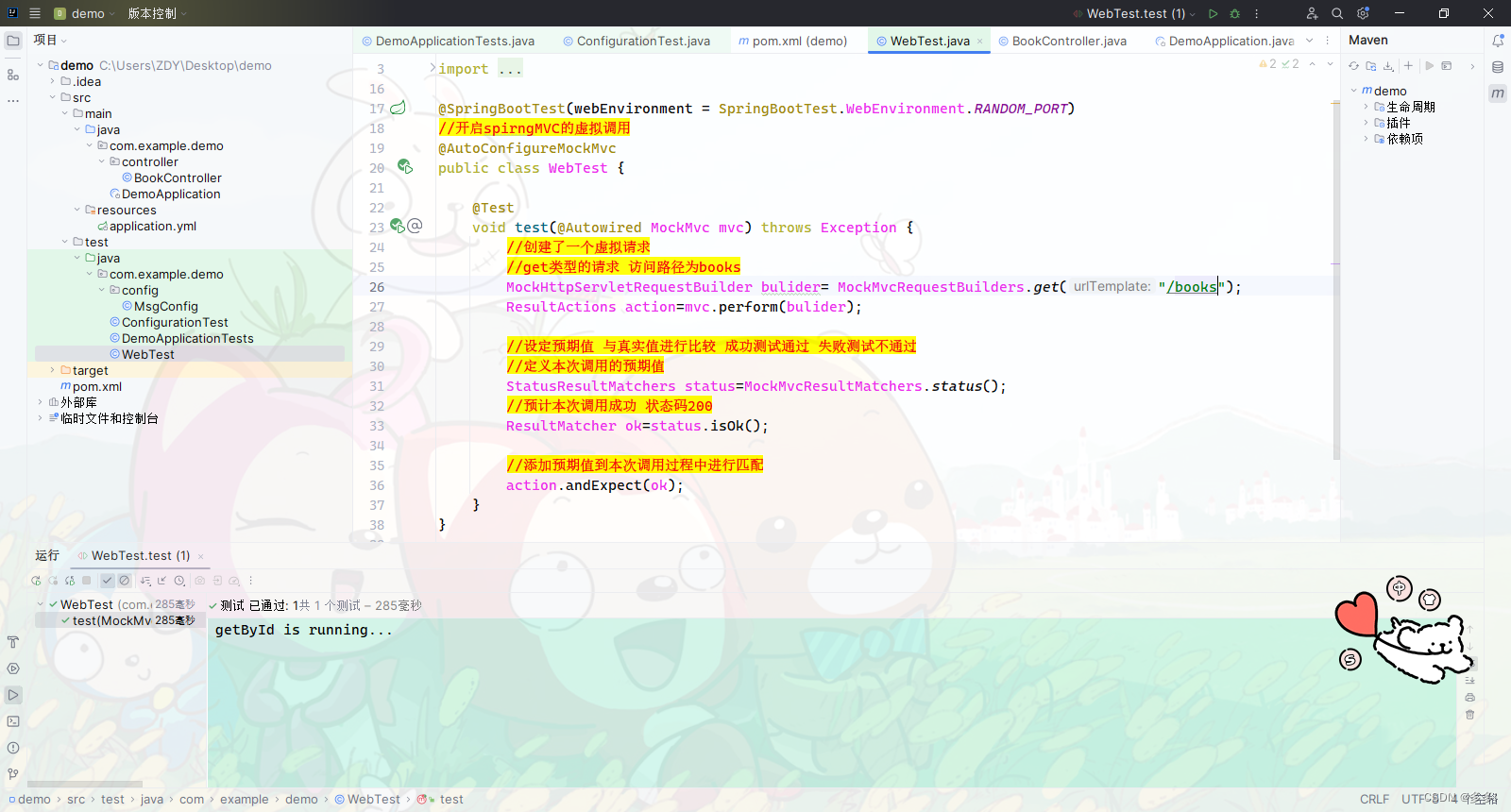
匹配失败
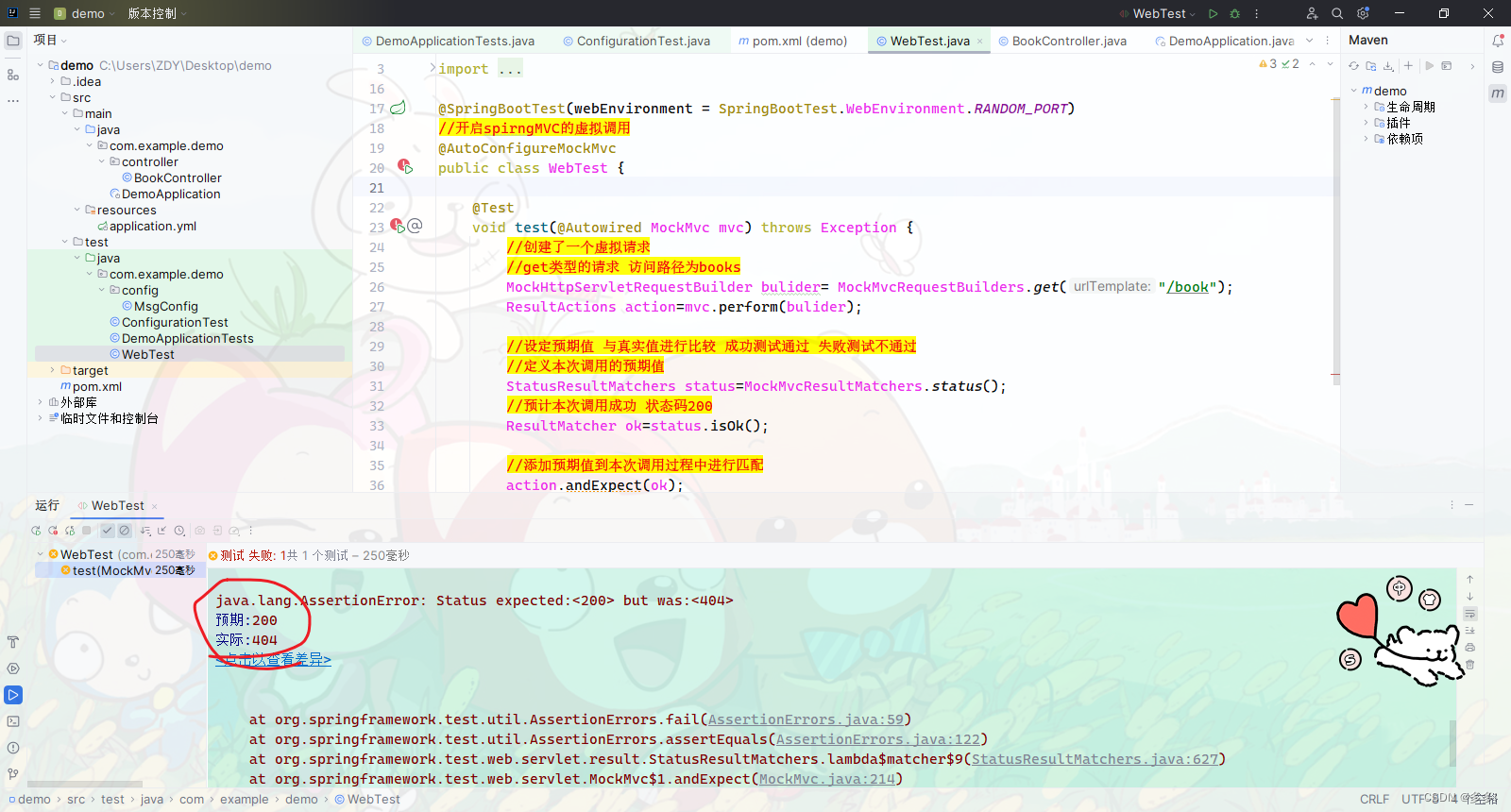
package com.example.demo;
import org.junit.jupiter.api.Test;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.test.autoconfigure.web.servlet.AutoConfigureMockMvc;
import org.springframework.boot.test.autoconfigure.web.servlet.MockMvcAutoConfiguration;
import org.springframework.boot.test.context.SpringBootTest;
import org.springframework.test.web.servlet.MockMvc;
import org.springframework.test.web.servlet.RequestBuilder;
import org.springframework.test.web.servlet.ResultActions;
import org.springframework.test.web.servlet.ResultMatcher;
import org.springframework.test.web.servlet.request.MockHttpServletRequestBuilder;
import org.springframework.test.web.servlet.request.MockMvcRequestBuilders;
import org.springframework.test.web.servlet.result.MockMvcResultMatchers;
import org.springframework.test.web.servlet.result.StatusResultMatchers;
@SpringBootTest(webEnvironment = SpringBootTest.WebEnvironment.RANDOM_PORT)
//开启spirngMVC的虚拟调用
@AutoConfigureMockMvc
public class WebTest {
@Test
void test(@Autowired MockMvc mvc) throws Exception {
//创建了一个虚拟请求
//get类型的请求 访问路径为books
MockHttpServletRequestBuilder bulider= MockMvcRequestBuilders.get("/books");
ResultActions action=mvc.perform(bulider);
//设定预期值 与真实值进行比较 成功测试通过 失败测试不通过
//定义本次调用的预期值
StatusResultMatchers status=MockMvcResultMatchers.status();
//预计本次调用成功 状态码200
ResultMatcher ok=status.isOk();
//添加预期值到本次调用过程中进行匹配
action.andExpect(ok);
}
}
小结
先去定义预期匹配结果
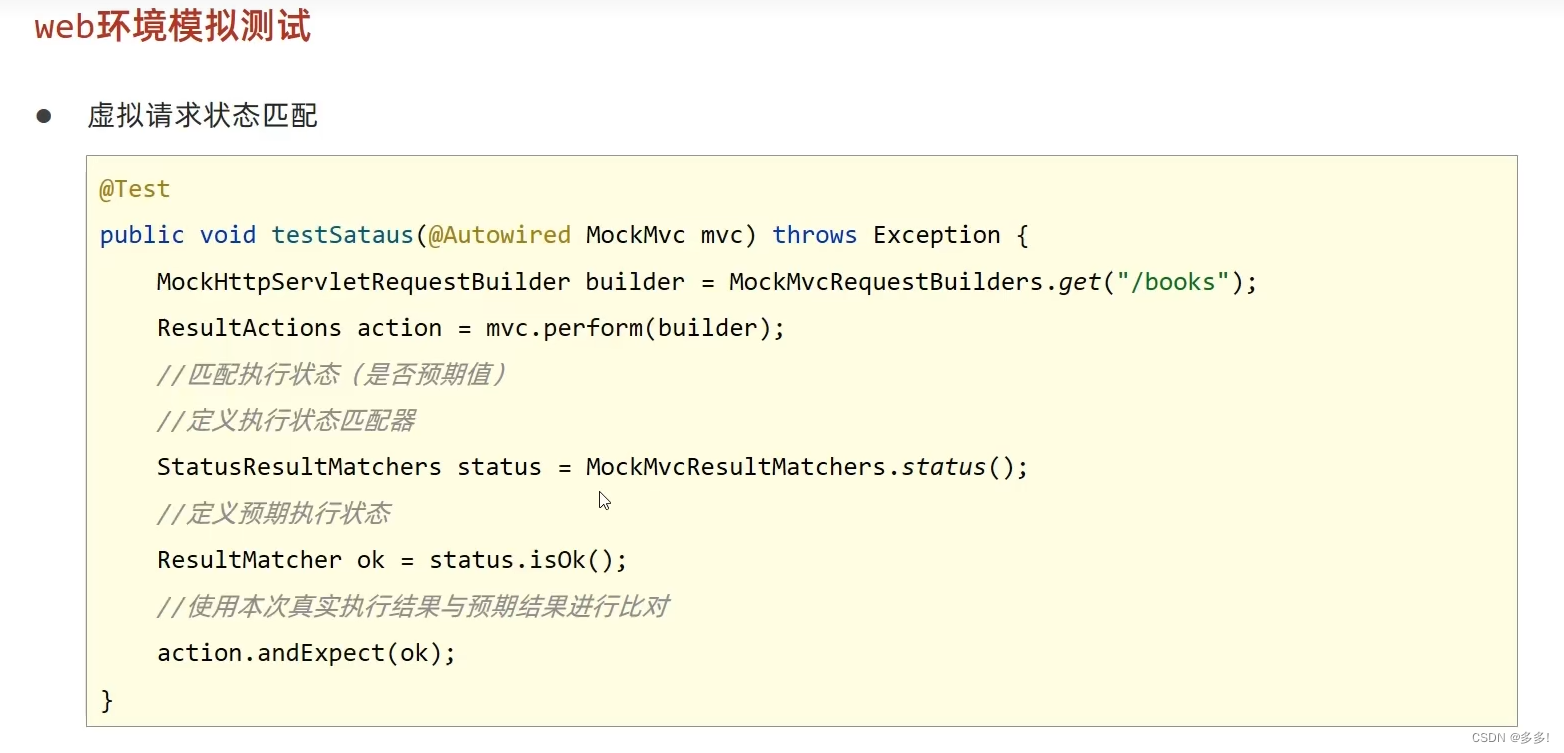
匹配响应体通解
匹配相应体是否和我们的预期结果一样
定义拿到的内容
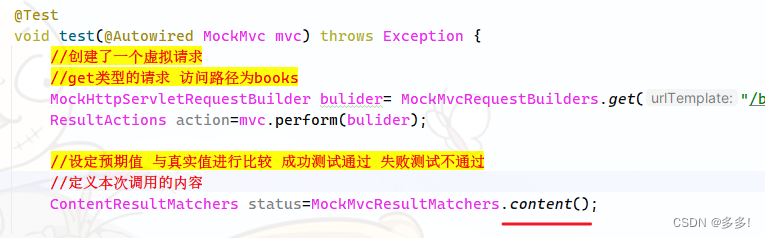
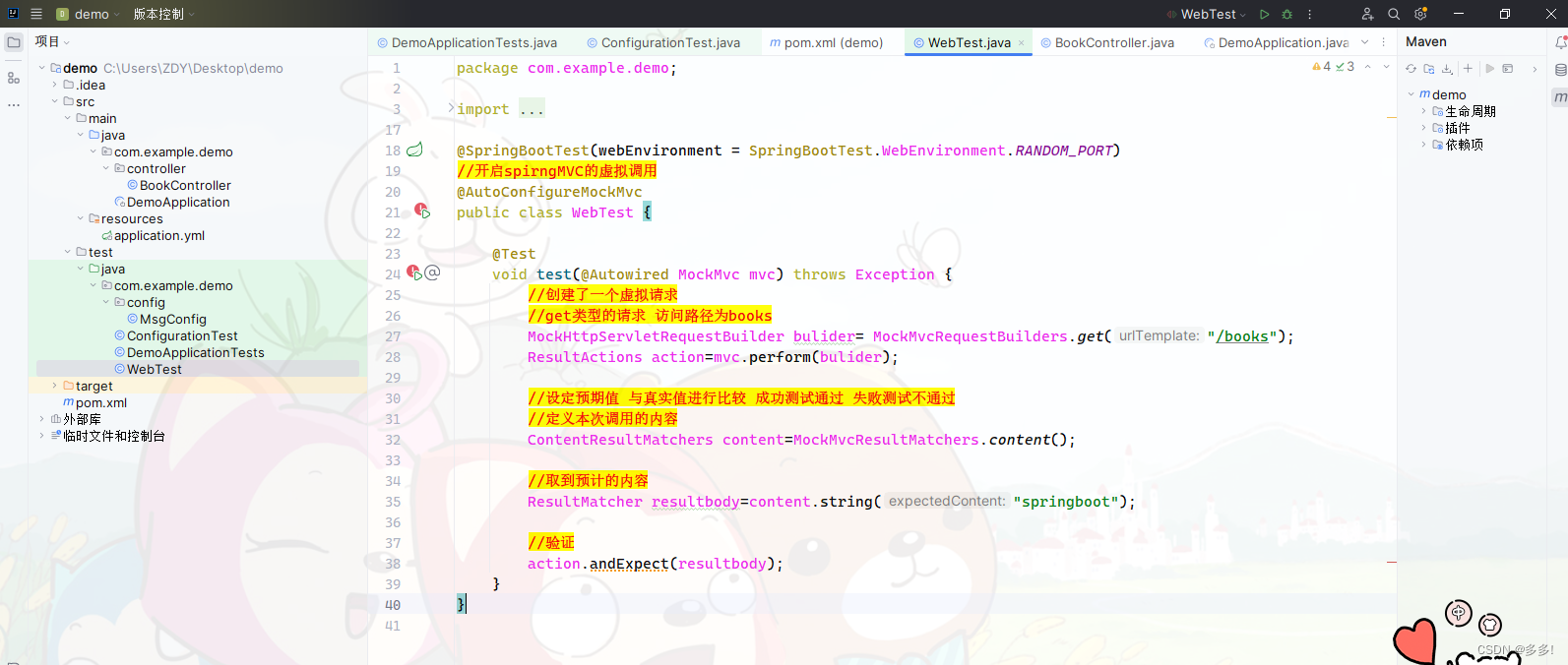
package com.example.demo;
import org.junit.jupiter.api.Test;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.test.autoconfigure.web.servlet.AutoConfigureMockMvc;
import org.springframework.boot.test.autoconfigure.web.servlet.MockMvcAutoConfiguration;
import org.springframework.boot.test.context.SpringBootTest;
import org.springframework.test.web.servlet.MockMvc;
import org.springframework.test.web.servlet.RequestBuilder;
import org.springframework.test.web.servlet.ResultActions;
import org.springframework.test.web.servlet.ResultMatcher;
import org.springframework.test.web.servlet.request.MockHttpServletRequestBuilder;
import org.springframework.test.web.servlet.request.MockMvcRequestBuilders;
import org.springframework.test.web.servlet.result.ContentResultMatchers;
import org.springframework.test.web.servlet.result.MockMvcResultMatchers;
import org.springframework.test.web.servlet.result.StatusResultMatchers;
@SpringBootTest(webEnvironment = SpringBootTest.WebEnvironment.RANDOM_PORT)
//开启spirngMVC的虚拟调用
@AutoConfigureMockMvc
public class WebTest {
@Test
void test(@Autowired MockMvc mvc) throws Exception {
//创建了一个虚拟请求
//get类型的请求 访问路径为books
MockHttpServletRequestBuilder bulider= MockMvcRequestBuilders.get("/books");
ResultActions action=mvc.perform(bulider);
//设定预期值 与真实值进行比较 成功测试通过 失败测试不通过
//定义本次调用的内容
ContentResultMatchers content=MockMvcResultMatchers.content();
//取到预计的内容
ResultMatcher resultbody=content.string("springboot");
//验证
action.andExpect(resultbody);
}
}
控制台输出打印信息
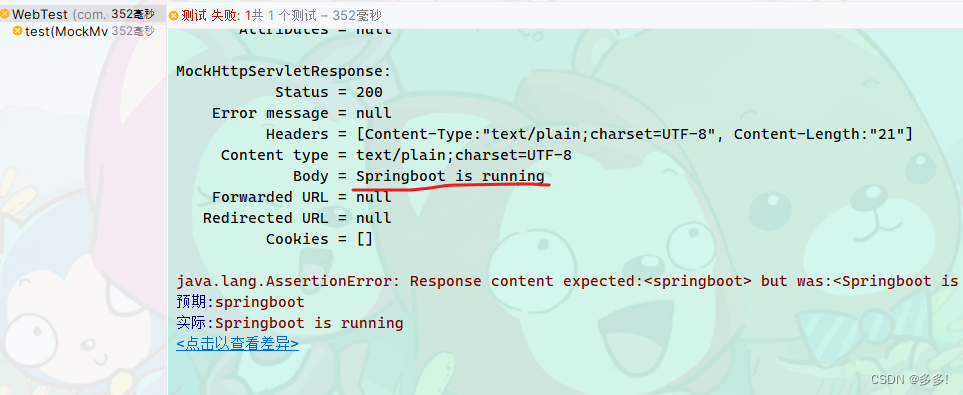
匹配JSON响应体
首先我们用lombok封装实体类
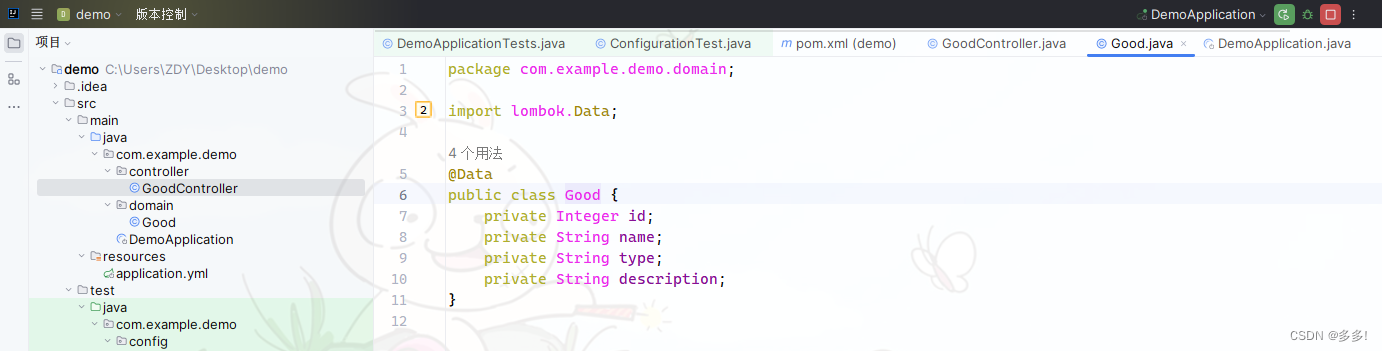
表现层把good数据发送到/goods路径上去
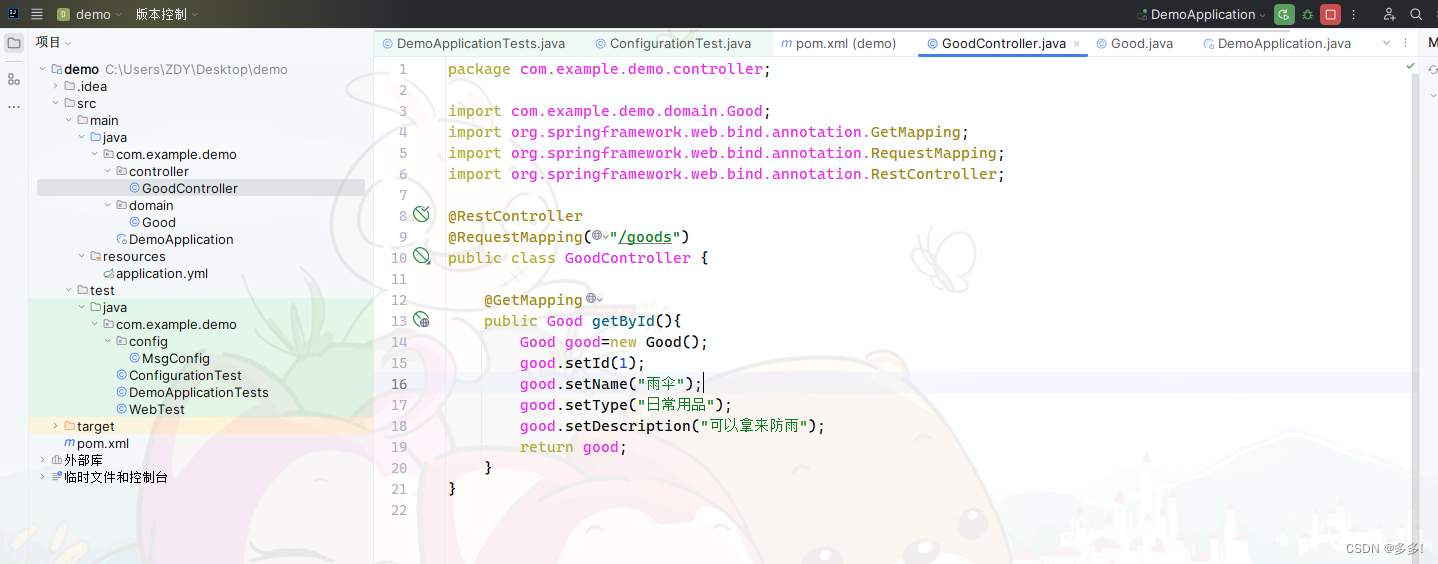
我们在页面中成功访问到
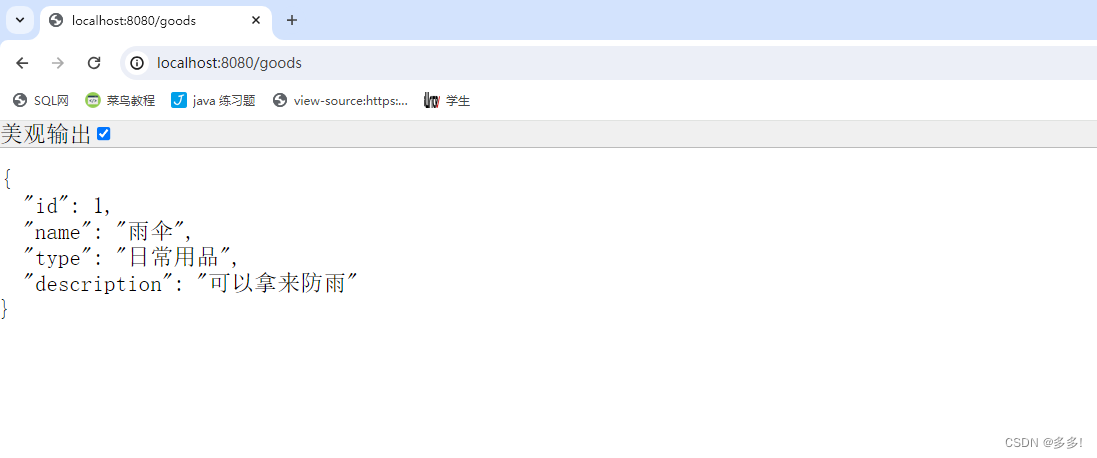
接下来我们要进行测试
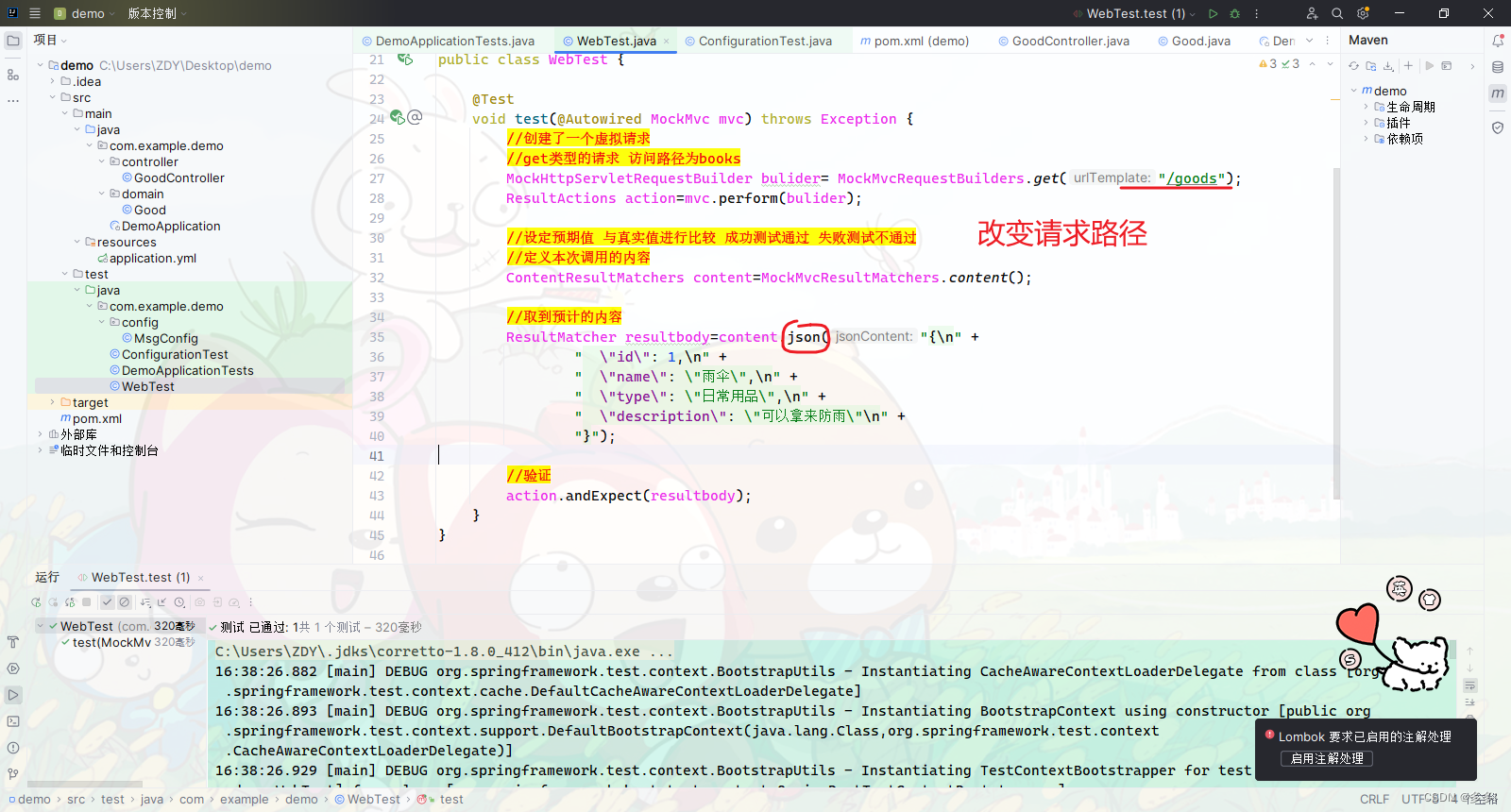
如果匹配失败
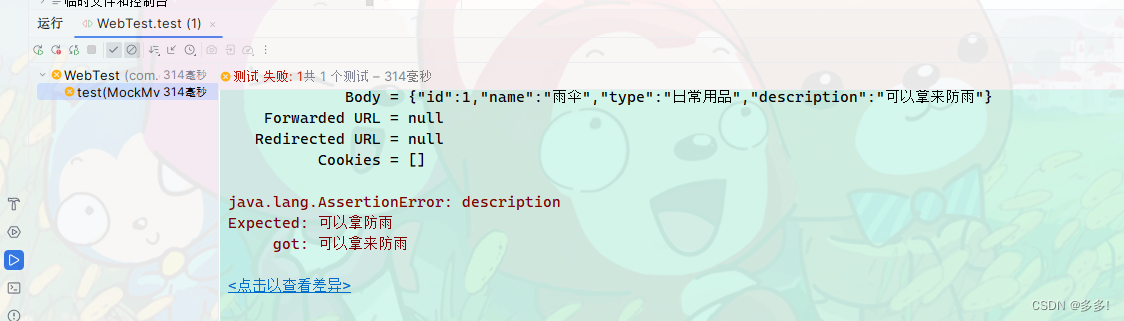
package com.example.demo;
import org.junit.jupiter.api.Test;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.test.autoconfigure.web.servlet.AutoConfigureMockMvc;
import org.springframework.boot.test.autoconfigure.web.servlet.MockMvcAutoConfiguration;
import org.springframework.boot.test.context.SpringBootTest;
import org.springframework.test.web.servlet.MockMvc;
import org.springframework.test.web.servlet.RequestBuilder;
import org.springframework.test.web.servlet.ResultActions;
import org.springframework.test.web.servlet.ResultMatcher;
import org.springframework.test.web.servlet.request.MockHttpServletRequestBuilder;
import org.springframework.test.web.servlet.request.MockMvcRequestBuilders;
import org.springframework.test.web.servlet.result.ContentResultMatchers;
import org.springframework.test.web.servlet.result.MockMvcResultMatchers;
import org.springframework.test.web.servlet.result.StatusResultMatchers;
@SpringBootTest(webEnvironment = SpringBootTest.WebEnvironment.RANDOM_PORT)
//开启spirngMVC的虚拟调用
@AutoConfigureMockMvc
public class WebTest {
@Test
void test(@Autowired MockMvc mvc) throws Exception {
//创建了一个虚拟请求
//get类型的请求 访问路径为books
MockHttpServletRequestBuilder bulider= MockMvcRequestBuilders.get("/goods");
ResultActions action=mvc.perform(bulider);
//设定预期值 与真实值进行比较 成功测试通过 失败测试不通过
//定义本次调用的内容
ContentResultMatchers content=MockMvcResultMatchers.content();
//取到预计的内容
ResultMatcher resultbody=content.json("{\n" +
" \"id\": 1,\n" +
" \"name\": \"雨伞\",\n" +
" \"type\": \"日常用品\",\n" +
" \"description\": \"可以拿来防雨\"\n" +
"}");
//验证
action.andExpect(resultbody);
}
}
匹配响应头
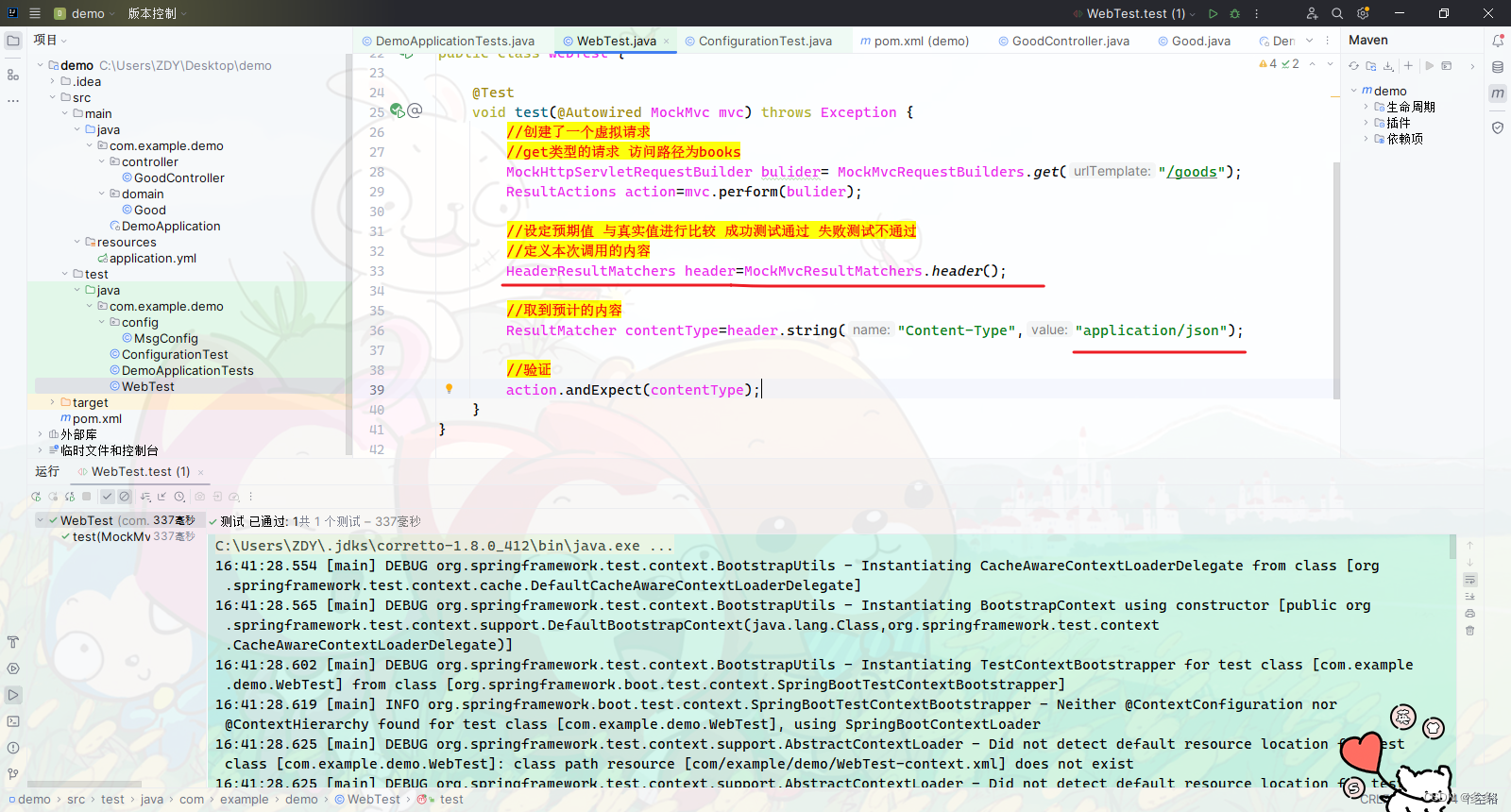
package com.example.demo;
import org.junit.jupiter.api.Test;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.test.autoconfigure.web.servlet.AutoConfigureMockMvc;
import org.springframework.boot.test.autoconfigure.web.servlet.MockMvcAutoConfiguration;
import org.springframework.boot.test.context.SpringBootTest;
import org.springframework.test.web.servlet.MockMvc;
import org.springframework.test.web.servlet.RequestBuilder;
import org.springframework.test.web.servlet.ResultActions;
import org.springframework.test.web.servlet.ResultMatcher;
import org.springframework.test.web.servlet.request.MockHttpServletRequestBuilder;
import org.springframework.test.web.servlet.request.MockMvcRequestBuilders;
import org.springframework.test.web.servlet.result.ContentResultMatchers;
import org.springframework.test.web.servlet.result.HeaderResultMatchers;
import org.springframework.test.web.servlet.result.MockMvcResultMatchers;
import org.springframework.test.web.servlet.result.StatusResultMatchers;
@SpringBootTest(webEnvironment = SpringBootTest.WebEnvironment.RANDOM_PORT)
//开启spirngMVC的虚拟调用
@AutoConfigureMockMvc
public class WebTest {
@Test
void test(@Autowired MockMvc mvc) throws Exception {
//创建了一个虚拟请求
//get类型的请求 访问路径为books
MockHttpServletRequestBuilder bulider= MockMvcRequestBuilders.get("/goods");
ResultActions action=mvc.perform(bulider);
//设定预期值 与真实值进行比较 成功测试通过 失败测试不通过
//定义本次调用的内容
HeaderResultMatchers header=MockMvcResultMatchers.header();
//取到预计的内容
ResultMatcher contentType=header.string("Content-Type","application/json");
//验证
action.andExpect(contentType);
}
}
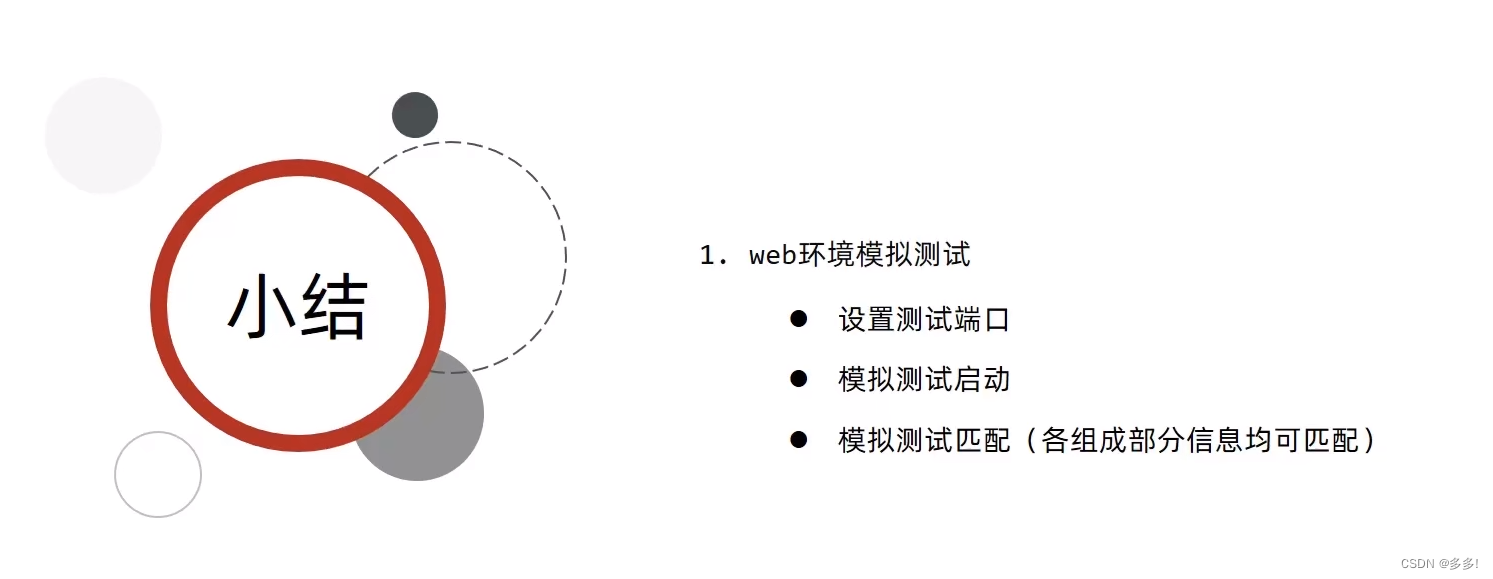