一、概述
1、java程序员在编写程序时提前编写好对异常的处理程序,在程序发生异常时就可以执行预先设定好的处理程序,处理程序执行完之后,可以继续向后执行后面的程序
2、异常处理程序是在程序执行出现异常时才执行的
二、5个关键字
1、try代码块,执行可能出现异常的代码
2、catch代码块,捕获类型匹配的异常对象并执行异常处理代码
3、finally代码块,里面包含的是必须要执行的代码
4、throws,定义一个方法时可以使用throws关键字声明,表示此方法不处理异常,而是就交给方法调用处进行处理
三、使用try和catch
try(异常类型 引用名){
异常处理代码
}
1、典例一
java
public static void main(String[] args) {
try {
// 可能会出现异常的代码
int a=1;
int b=0;
int c=a/b;
}catch (ArithmeticException e){// 异常类型 异常类型的引用变量名
System.out.println("除数不能为0");// 异常处理代码
}
System.out.println("后面的程序");
}

2、典例二:try代码块中出现异常的那一行程序后面的程序不会再被执行
java
public static void main(String[] args) {
try {
// 可能会出现异常的代码
int a=1;
int b=0;
int c=a/b;
String s="我爱吃西瓜";
System.out.println(s);
}catch (ArithmeticException e){// 异常类型 异常类型的引用变量名
System.out.println("除数不能为0");// 异常处理代码
}
System.out.println("后面的程序");
}
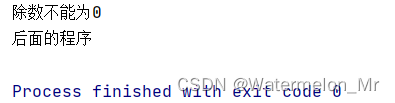
3、典例三: try代码块中如果可能出现多个异常则使用多个catch代码块去处理处理异常
java
public static void main(String[] args) {
try {
// 可能会出现异常的代码
int a=1;
int b=1;
int c=a/b;
String s=null;
System.out.println(s.length());
System.out.println("我爱吃西瓜");
}catch (ArithmeticException e){// 异常类型 异常类型的引用变量名
System.out.println("除数不能为0");// 异常处理代码
}catch (NullPointerException e){
System.out.println("字符串引用变量的值不能为null");
}
System.out.println("后面的程序");
}
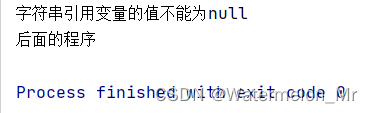
4、典例四: try代码块中如果不清楚会出现什么异常可以使用Exception作为异常类型去捕捉异常对象
java
public static void main(String[] args) {
try {
// 可能会出现异常的代码
int a=1;
int b=1;
int c=a/b;
String s=null;
System.out.println(s.length());
System.out.println("我爱吃西瓜");
}catch (ArithmeticException e){// 异常类型 异常类型的引用变量名
System.out.println("除数不能为0");// 异常处理代码
}catch (Exception e){
e.printStackTrace();// 输出异常类型和异常位置
System.out.println("程序异常:"+e.getMessage());// 打印异常原因
}
System.out.println("后面的程序");
}
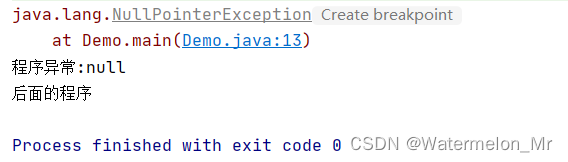
注意:捕捉父类异常的cath代码块必须放在捕捉子类异常的catch代码块之前
四、使用finally
finally代码块中都是一些必须要执行的代码
finally{
必须要执行的代码
}
1、典例一:应用场景(catch代码块中可能会出现异常)
java
try {
// 可能会出现异常的代码
int a=1;
int b=0;
int c=a/b;
System.out.println("我爱吃西瓜");
}catch (ArithmeticException e){// 异常类型 异常类型的引用变量名
String s=null;
System.out.println(s.length());
System.out.println("除数不能为0");// 异常处理代码
}finally {
System.out.println("最终必须要执行的代码");
}
System.out.println("后面的代码");

2、典例二:应用场景(必须要执行且只能放在finally代码块中的代码)
java
public int sum() throws IOException {
FileInputStream inputStream=null;
try {
inputStream=new FileInputStream("E:/a.txt");
}catch (FileNotFoundException f){
f.printStackTrace();
System.out.println("没找到文件");
}finally {
if (inputStream!=null){
inputStream.close();
System.out.println("关闭流通道");
}
return 100;
}
}

3、典例三:实际应用场景(IO读取文件时必须关闭流通道)
java
public static void main(String[] args) throws IOException {
FileInputStream inputStream=null;
try {
inputStream=new FileInputStream("E:/hhh.txt");// 创建流通道
inputStream.read();
}catch (FileNotFoundException f){
f.printStackTrace();
System.out.println("找不到文件");
}catch (IOException i){
i.printStackTrace();
System.out.println("读文件出现异常");
}finally {
if(inputStream!=null){
inputStream.close();// inputStream不为null,关闭流通道
}
}
}
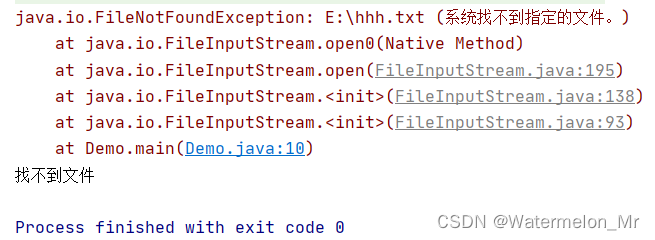
五、使用throws
public void test throws 异常1,异常2,异常3{
代码
}
1、任何方法都可以使用throws声明异常类型,包括抽象方法
2、一个方法可以声明多个异常类型
3、调用使用了throws的方法时必须处理声明的异常,要么使用try-catch,要么继续使用throws声明
4、如果抛出的是运行期异常,不会有提示,需要查看所调用的方法结构
5、典例
java
public class Demo {
public static void main(String[] args) {
try {
int res=divide(1,0);
System.out.println(res);
}catch (ArithmeticException a){
a.printStackTrace();
System.out.println("除数不能为0");
}
try {
byte[]bytes=transform("abc");
System.out.println(Arrays.toString(bytes));
} catch (UnsupportedEncodingException e) {
e.printStackTrace();
}
}
// 除法运算
public static int divide(int a,int b)throws ArithmeticException{// ArithmeticException 运行期异常 无提示 继承了RuntimeException
return a/b;
}
// 字符串转为字节数组返回
public static byte[] transform(String s) throws UnsupportedEncodingException {// UnsupportedEncodingException 编译期异常 有提示 不继承RuntimeException
byte[] bytes=s.getBytes("utf-8");// 指定编码格式
return bytes;
}
}
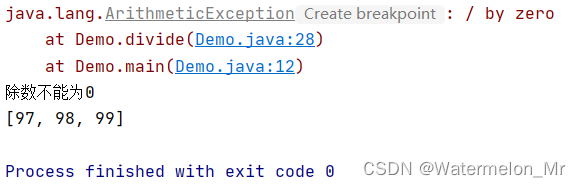
六、异常可以分为运行期异常和编译期异常两种
1、编译时期异常(即checked异常、受检异常):
(1)代码编译阶段,编译器就能明确当前代码可能发生XX异常
(2)并明确督促程序员提前编写处理它的的代码
(3)如果程序员没有编写对应的处理代码,编译期就会判定编译失败,从而不能生成字节码文件
(4)通常,这类异常的发生不是由程序员编写的代码引起的,例如:FileNotFoundException(文件找不到异常)
2、运行时期异常(即runtime异常、unchecked异常、非受检异常):
(1)代码编译阶段,编译器不做任何检查,无论该异常是否会发生,编译器都不会给出任何提示
(2)只有等代码运行起来并发生了XX异常,异常才能被发现
(3)通常,这类异常是由程序员的代码编写不当引起的,只要程序员编写代码稍加判断并且细心检查就可以避免
(4)java.lang.RuntimeException类及子类都是运行时异常,比如:ArrayIndexOutOfBoundsException数组下标越界异常,ClassCastException类型转换异常
七、使用throw
1、作用
在程序不满足某种条件时显示地抛出一个异常类的实例化对象,程序就不能继续向下执行
2、语法
throw new 异常类构造方法
如:throw new RunTimeException()
3、典例
java
public class Demo1 {
public static void main(String[] args) {
try {
char res=checkScore(690);
System.out.println(res);
} catch (Exception e) {
e.printStackTrace();
System.out.println("程序异常:"+e.getMessage());
}
}
/*
给分数划登记
100~90 A
89~80 B
79~0 C
*/
public static char checkScore(int score) throws Exception {
if (score < 0 || score > 100) {
throw new Exception("分数不合法");
}
if (score >= 90) {
return 'A';
} else if (score >= 80) {
return 'B';
} else {
return 'C';
}
}
}
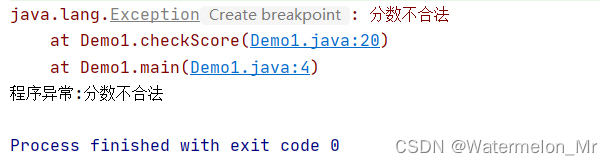
八、自定义异常
1、来源
(1)javaAPI中定义的标准异常类都是与语法相关的,比如索引越界异常、空指针异常
(2)但我们的程序有时候想要在不满足某种业务条件时以抛出异常的形式来做处理,此时就需要我们自定义一个与业务相关的异常类来表示,例如分数不合法异常(ScoreException)
java
public class ScoreException extends Exception{
public ScoreException() {
}
public ScoreException(String message) {
super(message);
}
}
java
public class Demo1 {
public static void main(String[] args) {
try {
char res=checkScore(690);
System.out.println(res);
} catch (ScoreException e) {
e.printStackTrace();
System.out.println("程序异常:"+e.getMessage());
}
}
/*
给分数划登记
100~90 A
89~80 B
79~0 C
*/
public static char checkScore(int score) throws ScoreException {
if (score < 0 || score > 100) {
throw new ScoreException("分数不合法");
}
if (score >= 90) {
return 'A';
} else if (score >= 80) {
return 'B';
} else {
return 'C';
}
}
}
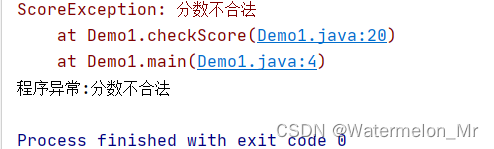