文章目录
封装
- 在private/protected 模块放置数据或者底层算法实现;
- 在public块提供对外接口,实现相应的功能调用;
- 类的封装案例
cpp
#include <iostream>
using namespace std;
// 类的定义 一般放在头文件
class Stu {
public: // 公有权限 接口
void setAge(const int& age) {
this->age = age;
}
const int& getAge() const {
return age;
}
private: // 数据封装
int age;
};
int main() {
// 创建对象
Stu s1;
s1.setAge(20);
cout << s1.getAge() << endl;
return 0;
}
继承
- 将一个类的成员变量、成员函数,应用到另一个类中,实现复用;
- 支持多继承,同python一样;而 java 仅支持单继承;
- 继承修饰符public、protected、private; 若不写修饰符,则默认private继承;
- public继承,基类中的public、protected、private 保持不变;
- protected继承,基类中的public、protected、private 变为protected、protected、private
- private继承, 基类中的public、protected、private全部变为private;
cpp
#include <iostream>
#include <string>
using namespace std;
// 基类
class People {
public:
string name;
People(const string& name, const int& age, const double& height) {
this->name = name;
this->age = age;
this->height = height;
cout << "父类完成初始化" << height << endl;
}
void printName() const {
cout << "name:" << name << endl;
}
void printAge() const {
cout << "age:" << this->age << endl;
}
void printHeight() const {
cout << "height:" << this->height << endl;
}
protected:
int age;
private:
double height;
};
// 类的定义 一般放在头文件
class Stu: public People { // 公有继承(经常使用)
public: // 可多个块
int num; // 若未放在public等修饰符下, 默认私有
Stu(const string& name, const int& age, const double&heigh): People(name, age, heigh){// 显式调用父类的有参构造,否则隐式调用父类的无参构造;
// 子类的构造 初始化
this->num = 102;
}
void printAge() const {// 子类方法中,只能访问继承的public/protected 成员,private成员无法访问,但可以通过父类的方法到父类代码中访问;
cout << "age:" << this->age << endl;
}
/*void printHeight() const {
cout << "height:" << this->height << endl;
} 不可访问继承的私有 */
};
int main() {
string name = "jack";
int age = 20;
double height = 23.5;
// 创建对象
Stu s1(name, age, height); // 父类的构造方法 完成对私有变量的初始化
s1.printName();
s1.printAge();
s1.printHeight(); // 在父类中访问私有
return 0;
}
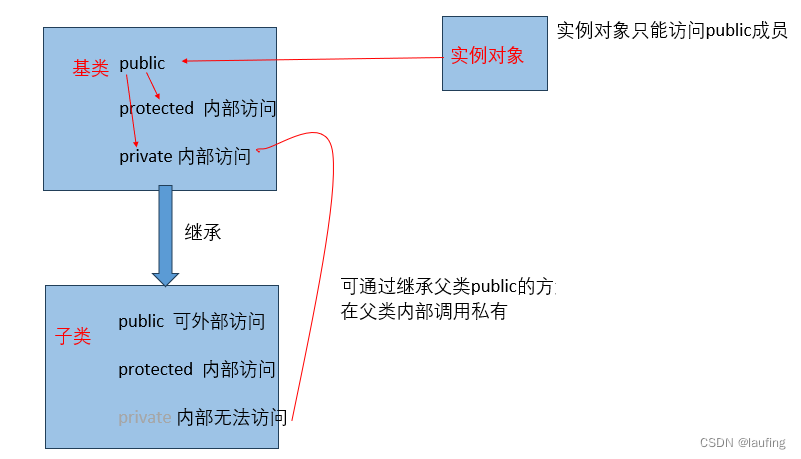
多态
- 多个子类继承父类中的方法,分别实现方法的重写;
- 父类中采用 virtual 声明为虚函数;
- 函数体赋值为=0,则为纯虚函数;
cpp
在这里插入代码片