文章目录
Map(java.util)
- Collection集合与Map集合的区别
- Collection 中的集合,元素是孤立存在的,向集合中存储元素采用一个个元素的方式存储。 Map 中的集合,元素是成对存在的。
- Collection 中的集合称为单列集合, Map 中的集合称为双列集合。
- Map接口中的常用方法
public V put(K key, V value)
: 把指定的键与指定的值添加到Map集合中。
public V remove(Object key)
: 把指定的键 所对应的键值对元素 在Map集合中删除,返回被删除元素的
值。
public V get(Object key)
根据指定的键,在Map集合中获取对应的值。
public Set<K> keySet()
: 获取Map集合中所有的键,存储到Set集合中。
public Set<Map.Entry<K,V>> entrySet()
: 获取到Map集合中所有的键值对对象的集合(Set集合)。
代码示例:
java
public class MapDemo {
public static void main(String[] args) {
Map<String, Integer> map = new HashMap<>();
map.put("xiaohu",18);
map.put("xiaoming",19);
map.put("xiaofang",21);
map.put("xiaohua",45);
System.out.println(map.get("xiaohu"));
Set<String> set = map.keySet();
for (String key : set) {
System.out.println(key + ":" + map.get(key));
}
System.out.println("-------------------------------------");
map.remove("xiaohua");
Set<Map.Entry<String, Integer>> set1 = map.entrySet();
for (Map.Entry<String, Integer> entry : set1) {
System.out.println(entry.getKey() + ":" + entry.getValue());
}
}
}
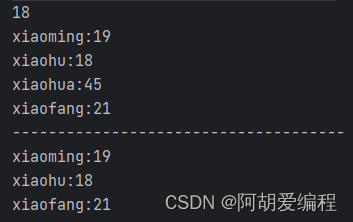
这里我们通过 **put(K key, V value)**方法存入了四个数值(HashMap),通过 **map.get("xiaohu")**来获取到键为"xiaohu"的值(18),通过 Set keySet() 来获取到所有的键的Set集合。通过增强for循环进行循环遍历, 这里我们发现遍历的结果和我们存储元素的顺序并不一致(可以通过LinkedHashMap来保证存取顺序一致) ,然后我们使用了 remove(Object key) 删除了一个键为"xiaohua"的值。最后我们通过 entrySet() 来获取一个Set集合,最后通过该集合进行遍历,通过 getKey()方法来获取键,通过 entry.getValue()方法来获取值。
注意:
当我们存储自定义类型键值时,我们必须要注意的一个点就是我们自定义类型必须要重写equals()方法以及hashCode()方法
- 当没有重写这俩个方法时:
java
public class Student {
private String name;
private int age;
public Student() {
}
public Student(String name, int age) {
this.name = name;
this.age = age;
}
@Override
public String toString() {
return "Student{" +
"name='" + name + '\'' +
", age=" + age +
'}';
}
public static void main(String[] args) {
Map<Student, String> map = new HashMap<>();
map.put(new Student("xiaohu",18),"001");
map.put(new Student("xiaoming",19),"002");
map.put(new Student("xiaofang",20),"003");
map.put(new Student("xiaohua",21) ,"004");
map.put(new Student("xiaohua",21) ,"005");
Set<Student> set = map.keySet();
for (Student key : set) {
System.out.println(key + ":" + map.get(key));
}
}
}
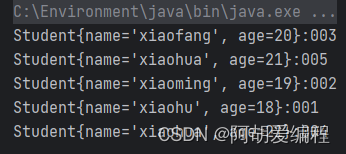
这里我们存入了俩个姓名为xiaohua,年龄为21的学生数据。
- 当我们重写了这俩个方法时:
java
import java.util.HashMap;
import java.util.Map;
import java.util.Objects;
import java.util.Set;
public class Student {
private String name;
private int age;
public Student() {
}
public Student(String name, int age) {
this.name = name;
this.age = age;
}
@Override
public String toString() {
return "Student{" +
"name='" + name + '\'' +
", age=" + age +
'}';
}
@Override
public boolean equals(Object o) {
if (this == o)
return true;
if (o == null || getClass() != o.getClass())
return false;
Student student = (Student) o;
return age == student.age && Objects.equals(name, student.name);
}
@Override
public int hashCode() {
return Objects.hash(name, age);
}
public static void main(String[] args) {
Map<Student, String> map = new HashMap<>();
map.put(new Student("xiaohu",18),"001");
map.put(new Student("xiaoming",19),"002");
map.put(new Student("xiaofang",20),"003");
map.put(new Student("xiaohua",21) ,"004");
map.put(new Student("xiaohua",21) ,"005");
Set<Student> set = map.keySet();
for (Student key : set) {
System.out.println(key + ":" + map.get(key));
}
}
}
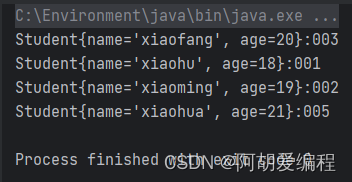
此时我们的map集合中不存在相同的学生数据了。
使用LinkedHashMap集合来存储这些数据,保证我们存和取的顺序是一致的。
java
import java.util.*;
public class Student {
private String name;
private int age;
public Student() {
}
public Student(String name, int age) {
this.name = name;
this.age = age;
}
@Override
public String toString() {
return "Student{" +
"name='" + name + '\'' +
", age=" + age +
'}';
}
@Override
public boolean equals(Object o) {
if (this == o)
return true;
if (o == null || getClass() != o.getClass())
return false;
Student student = (Student) o;
return age == student.age && Objects.equals(name, student.name);
}
@Override
public int hashCode() {
return Objects.hash(name, age);
}
public static void main(String[] args) {
Map<Student, String> map = new LinkedHashMap<>();
map.put(new Student("xiaohu",18),"001");
map.put(new Student("xiaoming",19),"002");
map.put(new Student("xiaofang",20),"003");
map.put(new Student("xiaohua",21) ,"004");
map.put(new Student("xiaohua",21) ,"005");
Set<Student> set = map.keySet();
for (Student key : set) {
System.out.println(key + ":" + map.get(key));
}
}
}
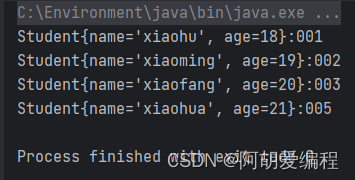
JDK9优化集合
新的List、Set、Map的静态工厂方法可以更方便地创建集合的
不可变实例
java
public class HelloJDK9 {
public static void main(String[] args) {
Set<String> str1=Set.of("a","b","c");
//str1.add("c");这里编译的时候不会错,但是执行的时候会报错,因为是不可变的集合
System.out.println(str1);
Map<String,Integer> str2=Map.of("a",1,"b",2);
System.out.println(str2);
List<String> str3=List.of("a","b");
System.out.println(str3);
}
}
- of()方法只是Map,List,Set这三个接口的静态方法,其父类接口和子类实现并没有这类方法,比如
HashSet,ArrayList等; - 返回的集合是不可变的;
欢迎java热爱者了解文章,作者将会持续更新中,期待各位友友的关注和收藏。。。