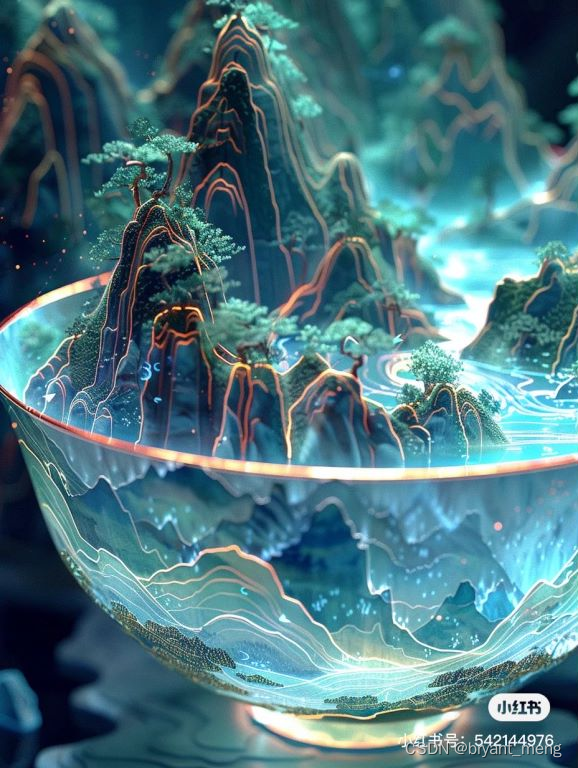
文章目录
- [Detect Aruco](#Detect Aruco)
- [Guess Aruco Type](#Guess Aruco Type)
Detect Aruco
学习参考来自:OpenCV基础(19)使用 OpenCV 和 Python 检测 ArUco 标记
更多使用细节可以参考:【python】OpenCV---Color Correction
源码:
链接:https://pan.baidu.com/s/1bEPuiix0MrtL7Fu3paoRug
提取码:123a
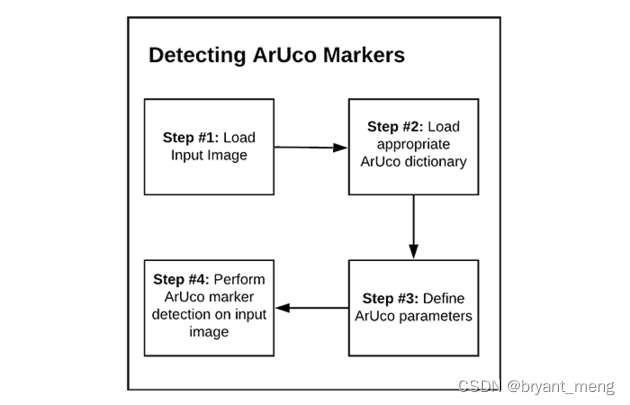
python
# -----------------------------
# USAGE
# -----------------------------
# python detect_aruco_image.py --image images/example_01.png --type DICT_5X5_100
# python detect_aruco_image.py --image images/example_02.png --type DICT_ARUCO_ORIGINAL
# -----------------------------
# IMPORTS
# -----------------------------
# Import the necessary packages
import argparse
import imutils
import cv2
import sys
# Construct the argument parser and parse the arguments
ap = argparse.ArgumentParser()
ap.add_argument("-i", "--image", required=True, help="Path to the input image containing the ArUCo tag")
ap.add_argument("-t", "--type", type=str, default="DICT_ARUCO_ORIGINAL", help="Tpe of ArUCo tag to detect")
args = vars(ap.parse_args())
# Define the names of each possible ArUco tag that OpenCV supports
ARUCO_DICT = {"DICT_4X4_50": cv2.aruco.DICT_4X4_50, "DICT_4X4_100": cv2.aruco.DICT_4X4_100,
"DICT_4X4_250": cv2.aruco.DICT_4X4_250, "DICT_4X4_1000": cv2.aruco.DICT_4X4_1000,
"DICT_5X5_50": cv2.aruco.DICT_5X5_50, "DICT_5X5_100": cv2.aruco.DICT_5X5_100,
"DICT_5X5_250": cv2.aruco.DICT_5X5_250, "DICT_5X5_1000": cv2.aruco.DICT_5X5_1000,
"DICT_6X6_50": cv2.aruco.DICT_6X6_50, "DICT_6X6_100": cv2.aruco.DICT_6X6_100,
"DICT_6X6_250": cv2.aruco.DICT_6X6_250, "DICT_6X6_1000": cv2.aruco.DICT_6X6_1000,
"DICT_7X7_50": cv2.aruco.DICT_7X7_50, "DICT_7X7_100": cv2.aruco.DICT_7X7_100,
"DICT_7X7_250": cv2.aruco.DICT_7X7_250, "DICT_7X7_1000": cv2.aruco.DICT_7X7_1000,
"DICT_ARUCO_ORIGINAL": cv2.aruco.DICT_ARUCO_ORIGINAL,
"DICT_APRILTAG_16h5": cv2.aruco.DICT_APRILTAG_16h5,
"DICT_APRILTAG_25h9": cv2.aruco.DICT_APRILTAG_25h9,
"DICT_APRILTAG_36h10": cv2.aruco.DICT_APRILTAG_36h10,
"DICT_APRILTAG_36h11": cv2.aruco.DICT_APRILTAG_36h11}
# Load the input image from disk and resize it
print("[INFO] Loading image...")
image = cv2.imread(args["image"])
image = imutils.resize(image, width=600)
# Verify that the supplied ArUCo tag exists is supported by OpenCV
if ARUCO_DICT.get(args["type"], None) is None:
print("[INFO] ArUCo tag of '{}' is not supported!".format(args["type"]))
sys.exit(0)
# Load the ArUCo dictionary, grab the ArUCo parameters and detect the markers
print("[INFO] Detecting '{}' tags...".format(args["type"]))
arucoDict = cv2.aruco.Dictionary_get(ARUCO_DICT[args["type"]])
arucoParams = cv2.aruco.DetectorParameters_create()
(corners, ids, rejected) = cv2.aruco.detectMarkers(image, arucoDict, parameters=arucoParams)
# Verify *at least* one ArUCo marker was detected
if len(corners) > 0:
# Flatten the ArUCo IDs list
ids = ids.flatten()
# Loop over the detected ArUCo corners
for (markerCorner, markerID) in zip(corners, ids):
# Extract the markers corners which are always returned in the following order:
# TOP-LEFT, TOP-RIGHT, BOTTOM-RIGHT, BOTTOM-LEFT
corners = markerCorner.reshape((4, 2))
(topLeft, topRight, bottomRight, bottomLeft) = corners
# Convert each of the (x, y)-coordinate pairs to integers
topRight = (int(topRight[0]), int(topRight[1]))
bottomRight = (int(bottomRight[0]), int(bottomRight[1]))
bottomLeft = (int(bottomLeft[0]), int(bottomLeft[1]))
topLeft = (int(topLeft[0]), int(topLeft[1]))
# Draw the bounding box of the ArUCo detection
cv2.line(image, topLeft, topRight, (0, 255, 0), 2)
cv2.line(image, topRight, bottomRight, (0, 255, 0), 2)
cv2.line(image, bottomRight, bottomLeft, (0, 255, 0), 2)
cv2.line(image, bottomLeft, topLeft, (0, 255, 0), 2)
# Compute and draw the center (x, y) coordinates of the ArUCo marker
cX = int((topLeft[0] + bottomRight[0]) / 2.0)
cY = int((topLeft[1] + bottomRight[1]) / 2.0)
cv2.circle(image, (cX, cY), 4, (0, 0, 255), -1)
# Draw the ArUco marker ID on the image
cv2.putText(image, str(markerID), (topLeft[0], topLeft[1] - 15), cv2.FONT_HERSHEY_SIMPLEX,
0.5, (0, 255, 0), 2)
print("[INFO] ArUco marker ID: {}".format(markerID))
# write the output image
cv2.imwrite("{}_{}.jpg".format(args["type"], markerID), image)
# Show the output image
cv2.imshow("Image", image)
cv2.waitKey(0)
输入图像
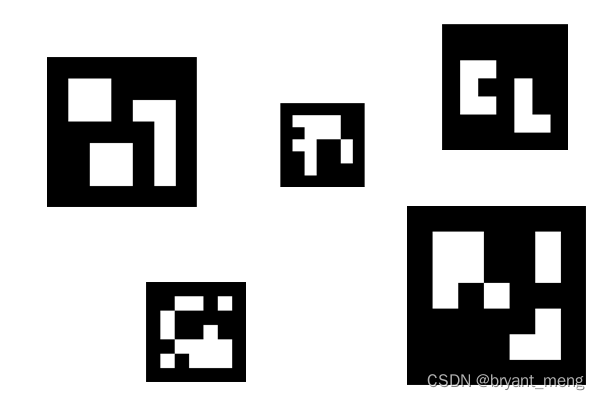
依次输出 DICT_5X5_100_42
DICT_5X5_100_24
DICT_5X5_100_70
DICT_5X5_100_66
DICT_5X5_100_87
再来一组
输入图片
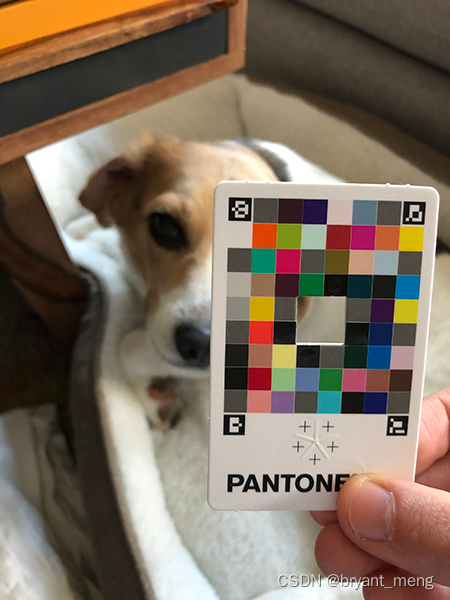
依次输出
DICT_ARUCO_ORIGINAL_241
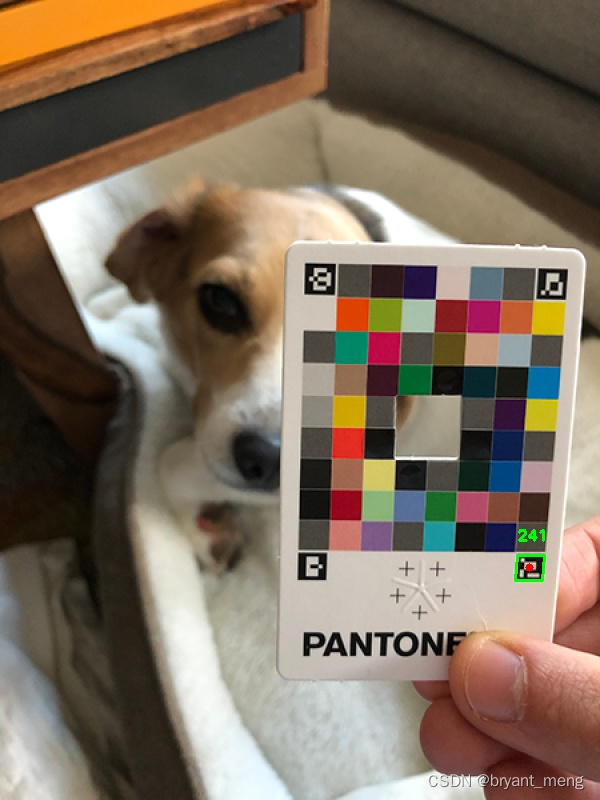
DICT_ARUCO_ORIGINAL_1007
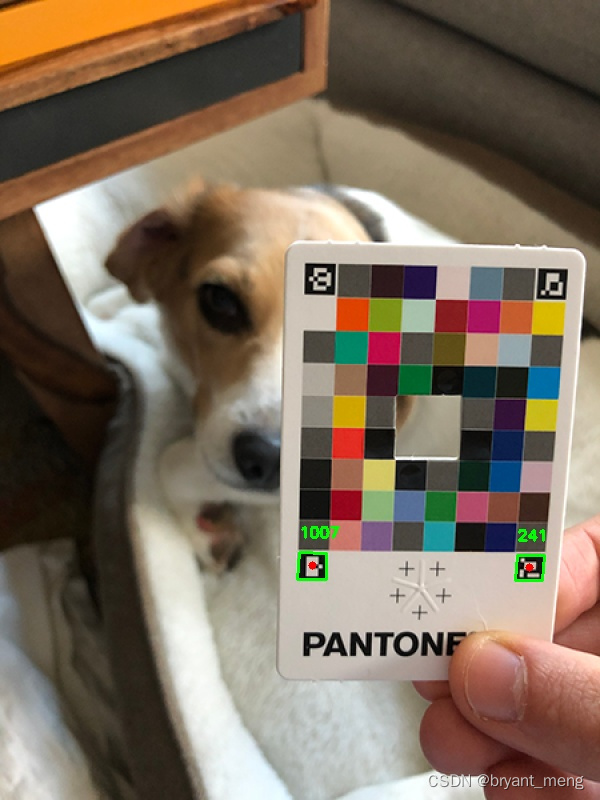
DICT_ARUCO_ORIGINAL_1001
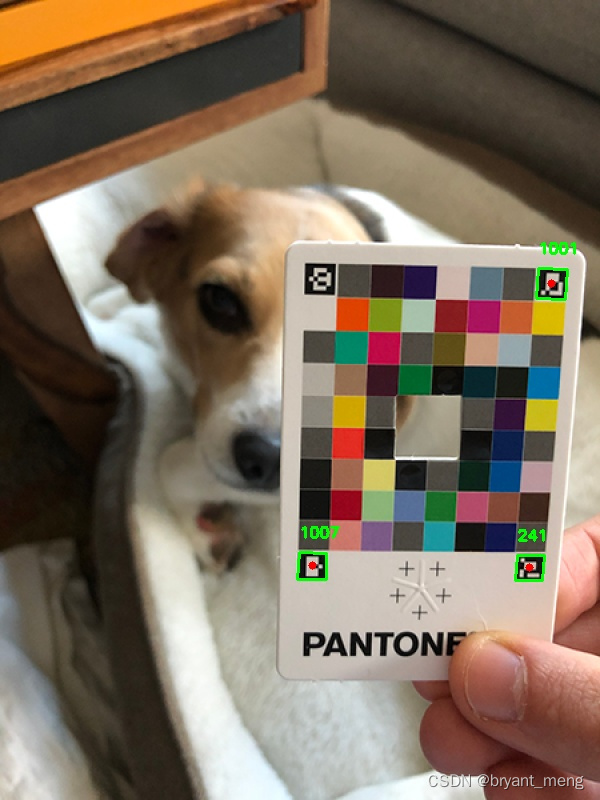
DICT_ARUCO_ORIGINAL_923
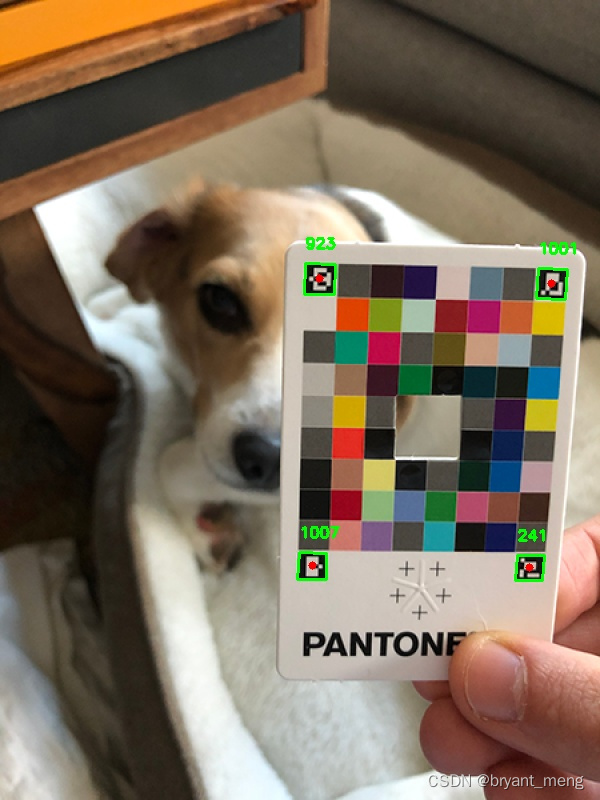
演示了如何检测图片,下面是检测视频的代码
python
# -----------------------------
# USAGE
# -----------------------------
# python detect_aruco_video.py
# -----------------------------
# IMPORTS
# -----------------------------
# Import the necessary packages
from imutils.video import VideoStream
import argparse
import imutils
import time
import cv2
import sys
# Construct the argument parser and parse the arguments
ap = argparse.ArgumentParser()
ap.add_argument("-t", "--type", type=str, default="DICT_ARUCO_ORIGINAL", help="Type of ArUCo tag to detect")
args = vars(ap.parse_args())
# Define the names of each possible ArUCo tag that OpenCV supports
ARUCO_DICT = {"DICT_4X4_50": cv2.aruco.DICT_4X4_50, "DICT_4X4_100": cv2.aruco.DICT_4X4_100,
"DICT_4X4_250": cv2.aruco.DICT_4X4_250, "DICT_4X4_1000": cv2.aruco.DICT_4X4_1000,
"DICT_5X5_50": cv2.aruco.DICT_5X5_50, "DICT_5X5_100": cv2.aruco.DICT_5X5_100,
"DICT_5X5_250": cv2.aruco.DICT_5X5_250, "DICT_5X5_1000": cv2.aruco.DICT_5X5_1000,
"DICT_6X6_50": cv2.aruco.DICT_6X6_50, "DICT_6X6_100": cv2.aruco.DICT_6X6_100,
"DICT_6X6_250": cv2.aruco.DICT_6X6_250, "DICT_6X6_1000": cv2.aruco.DICT_6X6_1000,
"DICT_7X7_50": cv2.aruco.DICT_7X7_50, "DICT_7X7_100": cv2.aruco.DICT_7X7_100,
"DICT_7X7_250": cv2.aruco.DICT_7X7_250, "DICT_7X7_1000": cv2.aruco.DICT_7X7_1000,
"DICT_ARUCO_ORIGINAL": cv2.aruco.DICT_ARUCO_ORIGINAL,
"DICT_APRILTAG_16h5": cv2.aruco.DICT_APRILTAG_16h5,
"DICT_APRILTAG_25h9": cv2.aruco.DICT_APRILTAG_25h9,
"DICT_APRILTAG_36h10": cv2.aruco.DICT_APRILTAG_36h10,
"DICT_APRILTAG_36h11": cv2.aruco.DICT_APRILTAG_36h11}
# Verify that the supplied ArUCo tag exists and is supported by OpenCV
if ARUCO_DICT.get(args["type"], None) is None:
print("[INFO] ArUCo tag of '{}' is not supported".format(args["type"]))
sys.exit(0)
# Load the ArUCo dictionary and grab the ArUCo parameters
print("[INFO] Detecting '{}' tags...".format(args["type"]))
arucoDict = cv2.aruco.Dictionary_get(ARUCO_DICT[args["type"]])
arucoParams = cv2.aruco.DetectorParameters_create()
# Initialize the video stream and allow the camera sensor to warm up
print("[INFO] Starting video stream...")
vs = VideoStream(src=0).start()
time.sleep(2.0)
# Loop over the frames from the video stream
while True:
# Grab the frame from the threaded video stream and resize it to have a maximum width of 600 pixels
frame = vs.read()
frame = imutils.resize(frame, width=1000)
# Detect ArUco markers in the input frame
(corners, ids, rejected) = cv2.aruco.detectMarkers(frame, arucoDict, parameters=arucoParams)
# Verify *at least* one ArUco marker was detected
if len(corners) > 0:
# Flatten the ArUco IDs list
ids = ids.flatten()
# Loop over the detected ArUCo corners
for (markerCorner, markerID) in zip(corners, ids):
# Extract the marker corners (which are always returned
# in top-left, top-right, bottom-right, and bottom-left order)
corners = markerCorner.reshape((4, 2))
(topLeft, topRight, bottomRight, bottomLeft) = corners
# Convert each of the (x, y)-coordinate pairs to integers
topRight = (int(topRight[0]), int(topRight[1]))
bottomRight = (int(bottomRight[0]), int(bottomRight[1]))
bottomLeft = (int(bottomLeft[0]), int(bottomLeft[1]))
topLeft = (int(topLeft[0]), int(topLeft[1]))
# Draw the bounding box of the ArUCo detection
cv2.line(frame, topLeft, topRight, (0, 255, 0), 2)
cv2.line(frame, topRight, bottomRight, (0, 255, 0), 2)
cv2.line(frame, bottomRight, bottomLeft, (0, 255, 0), 2)
cv2.line(frame, bottomLeft, topLeft, (0, 255, 0), 2)
# Compute and draw the center (x, y)-coordinates of the ArUco marker
cX = int((topLeft[0] + bottomRight[0]) / 2.0)
cY = int((topLeft[1] + bottomRight[1]) / 2.0)
cv2.circle(frame, (cX, cY), 4, (0, 0, 255), -1)
# Draw the ArUco marker ID on the frame
cv2.putText(frame, str(markerID), (topLeft[0], topLeft[1] - 15),
cv2.FONT_HERSHEY_SIMPLEX, 0.5, (0, 255, 0), 2)
# Show the output frame
cv2.imshow("Frame", frame)
key = cv2.waitKey(1) & 0xFF
# If the `q` key was pressed, break from the loop
if key == ord("q"):
break
# Do a bit of cleanup
cv2.destroyAllWindows()
vs.stop()
Guess Aruco Type
学习参考来自:OpenCV基础(20)使用 OpenCV 和 Python 确定 ArUco 标记类型
源码:
链接:https://pan.baidu.com/s/1DmjKL1tVbQX0YkDUzki2Jw
提取码:123a
python
# ------------------------
# USAGE
# ------------------------
# python guess_aruco_type.py --image images/example_01.png
# python guess_aruco_type.py --image images/example_02.png
# python guess_aruco_type.py --image images/example_03.png
# -----------------------------
# IMPORTS
# -----------------------------
# Import the necessary packages
import argparse
import imutils
import cv2
# Construct the argument parser and parse the arguments
ap = argparse.ArgumentParser()
ap.add_argument("-i", "--image", required=True, help="Path to the input image containing ArUCo tag")
args = vars(ap.parse_args())
# Define the names of each possible ArUCo tag that the OpenCV supports
ARUCO_DICT = {"DICT_4X4_50": cv2.aruco.DICT_4X4_50, "DICT_4X4_100": cv2.aruco.DICT_4X4_100,
"DICT_4X4_250": cv2.aruco.DICT_4X4_250, "DICT_4X4_1000": cv2.aruco.DICT_4X4_1000,
"DICT_5X5_50": cv2.aruco.DICT_5X5_50, "DICT_5X5_100": cv2.aruco.DICT_5X5_100,
"DICT_5X5_250": cv2.aruco.DICT_5X5_250, "DICT_5X5_1000": cv2.aruco.DICT_5X5_1000,
"DICT_6X6_50": cv2.aruco.DICT_6X6_50, "DICT_6X6_100": cv2.aruco.DICT_6X6_100,
"DICT_6X6_250": cv2.aruco.DICT_6X6_250, "DICT_6X6_1000": cv2.aruco.DICT_6X6_1000,
"DICT_7X7_50": cv2.aruco.DICT_7X7_50, "DICT_7X7_100": cv2.aruco.DICT_7X7_100,
"DICT_7X7_250": cv2.aruco.DICT_7X7_250, "DICT_7X7_1000": cv2.aruco.DICT_7X7_1000,
"DICT_ARUCO_ORIGINAL": cv2.aruco.DICT_ARUCO_ORIGINAL,
"DICT_APRILTAG_16h5": cv2.aruco.DICT_APRILTAG_16h5,
"DICT_APRILTAG_25h9": cv2.aruco.DICT_APRILTAG_25h9,
"DICT_APRILTAG_36h10": cv2.aruco.DICT_APRILTAG_36h10,
"DICT_APRILTAG_36h11": cv2.aruco.DICT_APRILTAG_36h11}
# Load the input image from disk and resize it
print("[INFO] Loading image...")
image = cv2.imread(args["image"])
image = imutils.resize(image, width=800)
# Loop over the types of ArUCo dictionaries
for (arucoName, arucoDictionary) in ARUCO_DICT.items():
# Load the ArUCo dictionary, grab the ArUCo parameters and attempt to detect the markers for the current dictionary
arucoDict = cv2.aruco.Dictionary_get(arucoDictionary)
arucoParams = cv2.aruco.DetectorParameters_create()
(corners, ids, rejected) = cv2.aruco.detectMarkers(image, arucoDict, parameters=arucoParams)
# If at least one ArUCo marker was detected display the ArUCo marker and its type name in the terminal
if len(corners) > 0:
print("[INFO] Detected {} markers for '{}'".format(len(corners), arucoName))
输入
输出
python
[INFO] Loading image...
[INFO] Detected 2 markers for 'DICT_5X5_50'
[INFO] Detected 5 markers for 'DICT_5X5_100'
[INFO] Detected 5 markers for 'DICT_5X5_250'
[INFO] Detected 5 markers for 'DICT_5X5_1000'
输入
输出
python
[INFO] Loading image...
[INFO] Detected 1 markers for 'DICT_4X4_50'
[INFO] Detected 1 markers for 'DICT_4X4_100'
[INFO] Detected 1 markers for 'DICT_4X4_250'
[INFO] Detected 1 markers for 'DICT_4X4_1000'
[INFO] Detected 4 markers for 'DICT_ARUCO_ORIGINAL'
输入
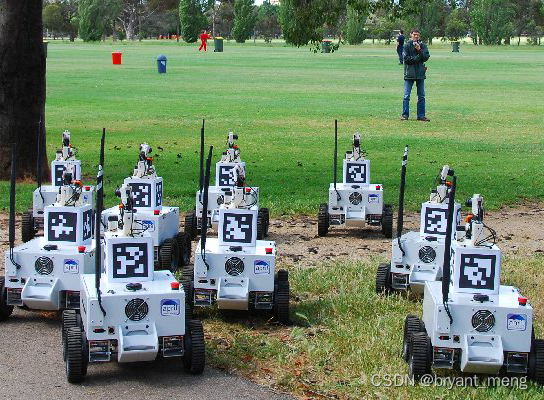
输出
python
[INFO] Loading image...
[INFO] Detected 5 markers for 'DICT_APRILTAG_36h11'
猜出来了 Aruco 的类型,我们就可以设定检测了
python
# ------------------------
# USAGE
# ------------------------
# python detect_aruco_image_type.py --image images/example_03.png
# -----------------------------
# IMPORTS
# -----------------------------
# Import the necessary packages
import argparse
import imutils
import cv2
import sys
# Construct the argument parser and parse the arguments
ap = argparse.ArgumentParser()
ap.add_argument("-i", "--image", required=True, help="Path to the input image containing ArUCo tag")
args = vars(ap.parse_args())
# Define the names of each possible ArUCo tag that the OpenCV supports
ARUCO_DICT = {"DICT_4X4_50": cv2.aruco.DICT_4X4_50, "DICT_4X4_100": cv2.aruco.DICT_4X4_100,
"DICT_4X4_250": cv2.aruco.DICT_4X4_250, "DICT_4X4_1000": cv2.aruco.DICT_4X4_1000,
"DICT_5X5_50": cv2.aruco.DICT_5X5_50, "DICT_5X5_100": cv2.aruco.DICT_5X5_100,
"DICT_5X5_250": cv2.aruco.DICT_5X5_250, "DICT_5X5_1000": cv2.aruco.DICT_5X5_1000,
"DICT_6X6_50": cv2.aruco.DICT_6X6_50, "DICT_6X6_100": cv2.aruco.DICT_6X6_100,
"DICT_6X6_250": cv2.aruco.DICT_6X6_250, "DICT_6X6_1000": cv2.aruco.DICT_6X6_1000,
"DICT_7X7_50": cv2.aruco.DICT_7X7_50, "DICT_7X7_100": cv2.aruco.DICT_7X7_100,
"DICT_7X7_250": cv2.aruco.DICT_7X7_250, "DICT_7X7_1000": cv2.aruco.DICT_7X7_1000,
"DICT_ARUCO_ORIGINAL": cv2.aruco.DICT_ARUCO_ORIGINAL,
"DICT_APRILTAG_16h5": cv2.aruco.DICT_APRILTAG_16h5,
"DICT_APRILTAG_25h9": cv2.aruco.DICT_APRILTAG_25h9,
"DICT_APRILTAG_36h10": cv2.aruco.DICT_APRILTAG_36h10,
"DICT_APRILTAG_36h11": cv2.aruco.DICT_APRILTAG_36h11}
# Load the input image from disk and resize it
print("[INFO] Loading image...")
image = cv2.imread(args["image"])
image = imutils.resize(image, width=800)
# Verify that the supplied ArUCo tag exists is supported by OpenCV
# if ARUCO_DICT.get(args["type"], None) is None:
# print("[INFO] ArUCo tag of '{}' is not supported!".format(args["type"]))
# sys.exit(0)
# Loop over the types of ArUCo dictionaries
for (arucoName, arucoDictionary) in ARUCO_DICT.items():
# Load the ArUCo dictionary, grab the ArUCo parameters and attempt to detect the markers for the current dictionary
arucoDict = cv2.aruco.Dictionary_get(arucoDictionary)
arucoParams = cv2.aruco.DetectorParameters_create()
(corners, ids, rejected) = cv2.aruco.detectMarkers(image, arucoDict, parameters=arucoParams)
# If at least one ArUCo marker was detected display the ArUCo marker and its type name in the terminal
if len(corners) > 0:
print("[INFO] Detected {} markers for '{}'".format(len(corners), arucoName))
# Flatten the ArUCo IDs list
IDS = ids.flatten()
# Loop over the detected ArUCo corners
for (markerCorner, markerID) in zip(corners, IDS):
# Extract the markers corners which are always returned in the following order:
# TOP-LEFT, TOP-RIGHT, BOTTOM-RIGHT, BOTTOM-LEFT
corners = markerCorner.reshape((4, 2))
(topLeft, topRight, bottomRight, bottomLeft) = corners
# Convert each of the (x, y)-coordinate pairs to integers
topRight = (int(topRight[0]), int(topRight[1]))
bottomRight = (int(bottomRight[0]), int(bottomRight[1]))
bottomLeft = (int(bottomLeft[0]), int(bottomLeft[1]))
topLeft = (int(topLeft[0]), int(topLeft[1]))
# Draw the bounding box of the ArUCo detection
cv2.line(image, topLeft, topRight, (0, 255, 0), 2)
cv2.line(image, topRight, bottomRight, (0, 255, 0), 2)
cv2.line(image, bottomRight, bottomLeft, (0, 255, 0), 2)
cv2.line(image, bottomLeft, topLeft, (0, 255, 0), 2)
# Compute and draw the center (x, y) coordinates of the ArUCo marker
cX = int((topLeft[0] + bottomRight[0]) / 2.0)
cY = int((topLeft[1] + bottomRight[1]) / 2.0)
cv2.circle(image, (cX, cY), 4, (0, 0, 255), -1)
# Get marker type name
markerType = "{} -> {}".format(markerID, arucoName)
# Draw the ArUco marker ID on the image
cv2.putText(image, str(markerType), (topLeft[0], topLeft[1] - 15), cv2.FONT_HERSHEY_SIMPLEX, 0.5,
(0, 255, 0), 2)
print("[INFO] ArUco marker ID: {}".format(markerID))
# Write the output image
cv2.imwrite(f"{markerID}_{arucoName}.jpg", image)
# Show the output image
cv2.imshow("Image", image)
cv2.waitKey(0)
输入
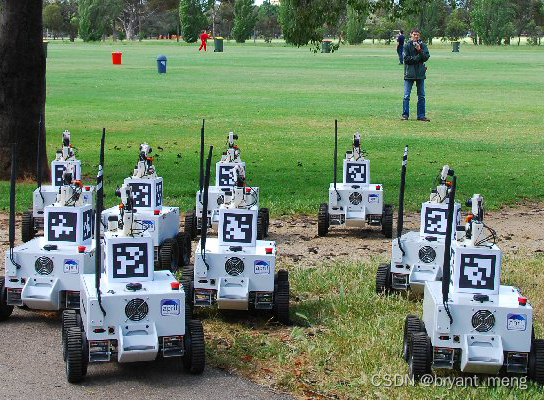
依次输出
7_DICT_APRILTAG_36h11
3_DICT_APRILTAG_36h11
5_DICT_APRILTAG_36h11
14_DICT_APRILTAG_36h11
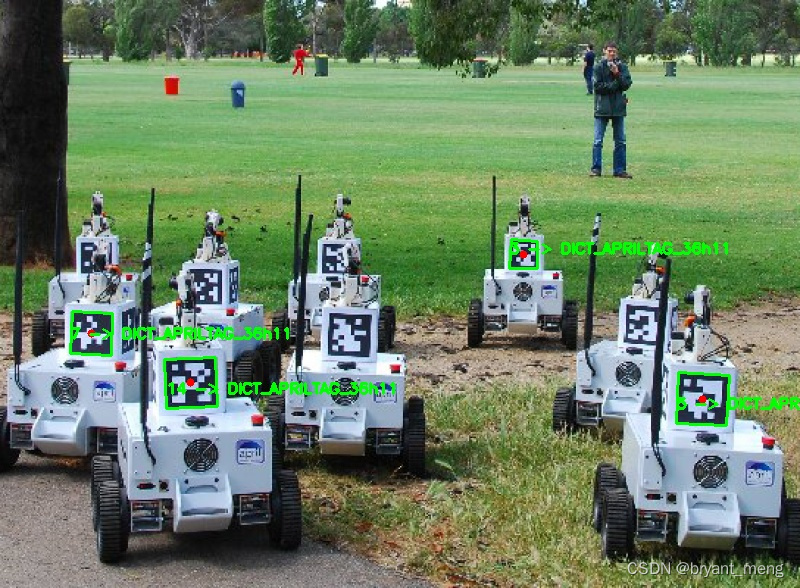
8_DICT_APRILTAG_36h11
再看看另外一个的案例
DICT_5X5_100
87_DICT_5X5_250
87_DICT_5X5_1000
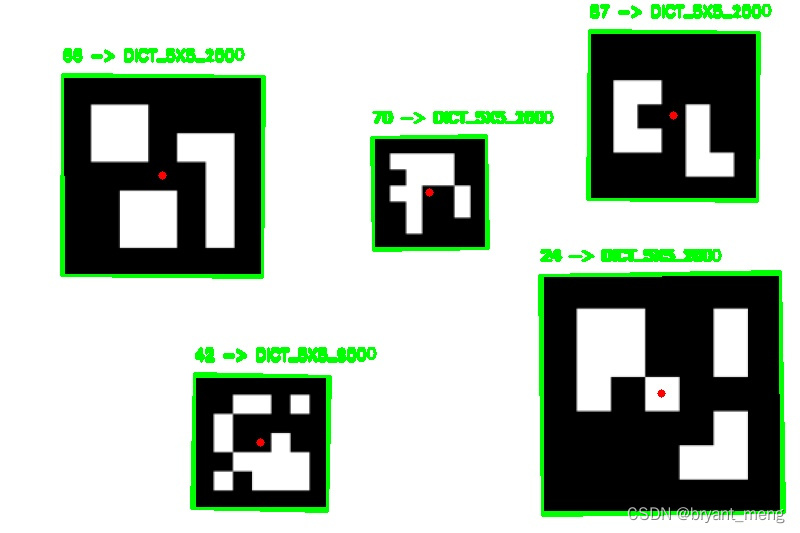