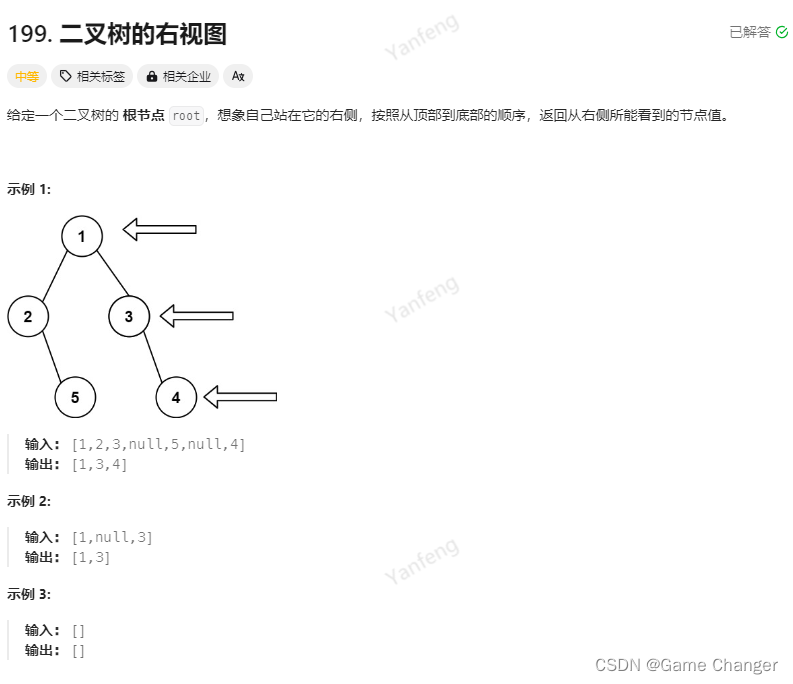
层序遍历,广度优先
queue先进后出,每层从左往右进树,最后一个就是最右边的数;pop掉这层的。push下一层;
cpp
class Solution {
public:
vector<int> rightSideView(TreeNode* root) {
if(root==nullptr)
return vector<int>();
vector<int> rtnum;
queue<TreeNode*> nodetree;
nodetree.push(root);
while(!nodetree.empty())
{
int size = nodetree.size();
TreeNode* tmp;
for(int i = 0; i < size; i++)
{
tmp = nodetree.front();
nodetree.pop();
if(tmp->left)
nodetree.push(tmp->left);
if(tmp->right){nodetree.push(tmp->right);}
}
rtnum.push_back(tmp->val);
}
return rtnum;
}
};
递归
遍历顺序改为根、右子树、左子树;
这样往下遍历,到达新一层的第一个节点就是右子树;
就是到达新的深度的第一个就是最右边的;
cpp
class Solution {
vector<int> ans;
void dfs(TreeNode* node, int depth) {
if (node == nullptr) {
return;
}
if (depth == ans.size()) { // 这个深度首次遇到
ans.push_back(node->val);
}
dfs(node->right, depth + 1); // 先递归右子树,保证首次遇到的一定是最右边的节点
dfs(node->left, depth + 1);
}
public:
vector<int> rightSideView(TreeNode* root) {
dfs(root, 0);
return ans;
}
};