文章目录
- Github
- 文档
- 推荐文章
- 简介
- 安装
- 官方示例
- 中文情感分析模型
-
- [分词器 Tokenizer](#分词器 Tokenizer)
- [填充 Padding](#填充 Padding)
- [截断 Truncation](#截断 Truncation)
- google-t5/t5-small
- 使用脚本进行训练
- 机器翻译
Github
文档
- https://huggingface.co/docs/transformers/index
- https://github.com/huggingface/transformers/blob/main/i18n/README_zh-hans.md
推荐文章
简介
Transformers是一种基于注意力机制(Attention Mechanism)的神经网络模型,广泛应用于自然语言处理(Natural Language Processing)任务中,如机器翻译、文本生成和文本分类等。
传统的序列模型(如循环神经网络)在处理长距离依赖时可能遇到困难,而Transformers通过引入注意力机制来解决这个问题。注意力机制使得模型能够在序列中对不同位置的信息进行加权关注,从而捕捉到全局的上下文信息。
在Transformers中,输入序列首先被分别编码为查询(Query)、键(Key)和值(Value)向量。通过计算查询与键的相似度,得到注意力分数,再将注意力分数与值相乘并加权求和,即可得到最终的上下文表示。这种自注意力机制允许模型在编码器和解码器中自由交换信息,从而更好地处理长距离依赖关系。
Transformer模型的核心组件是多层的自注意力机制和前馈神经网络。它的架构被广泛应用于许多重要的NLP任务,其中最著名的是BERT(Bidirectional Encoder Representations from Transformers),它在多项NLP任务上取得了突破性的性能。
除了NLP领域,Transformers模型也被应用于计算机视觉和其他领域,用于处理序列建模和生成任务。它已经成为深度学习中非常重要和有影响力的模型架构之一。
安装
bash
pip install transformers
# PyTorch(推荐)
pip install 'transformers[torch]'
# TensorFlow 2.0
pip install 'transformers[tf-cpu]'
- M1 / ARM 用户在安装 TensorFLow 2.0 之前,需要安装以下内容
bash
brew install cmake
brew install pkg-config
- 验证是否安装成功
bash
python -c "from transformers import pipeline; print(pipeline('sentiment-analysis')('we love you'))"
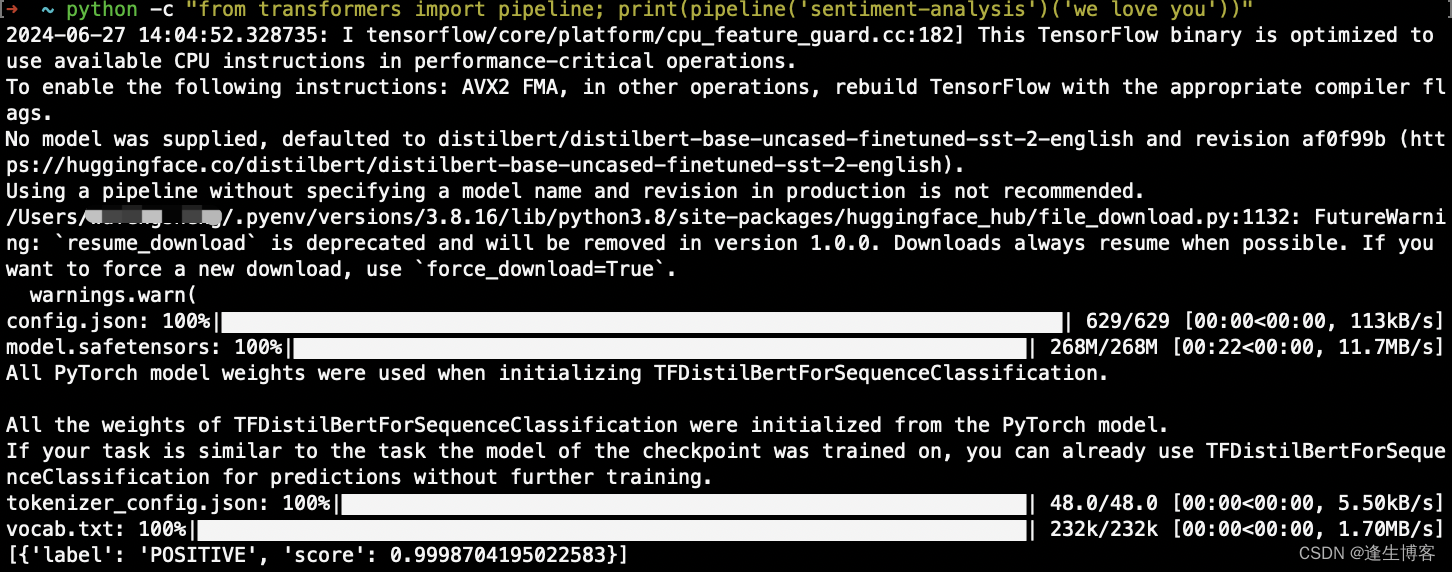
注意: 以上验证操作需要"连网",否则因无法下载文件而出现报错。
官方示例
python
from transformers import pipeline
# 使用情绪分析流水线
classifier = pipeline('sentiment-analysis')
classifier('We are very happy to introduce pipeline to the transformers repository.')
- 输出结果
bash
[{'label': 'POSITIVE', 'score': 0.9996980428695679}]
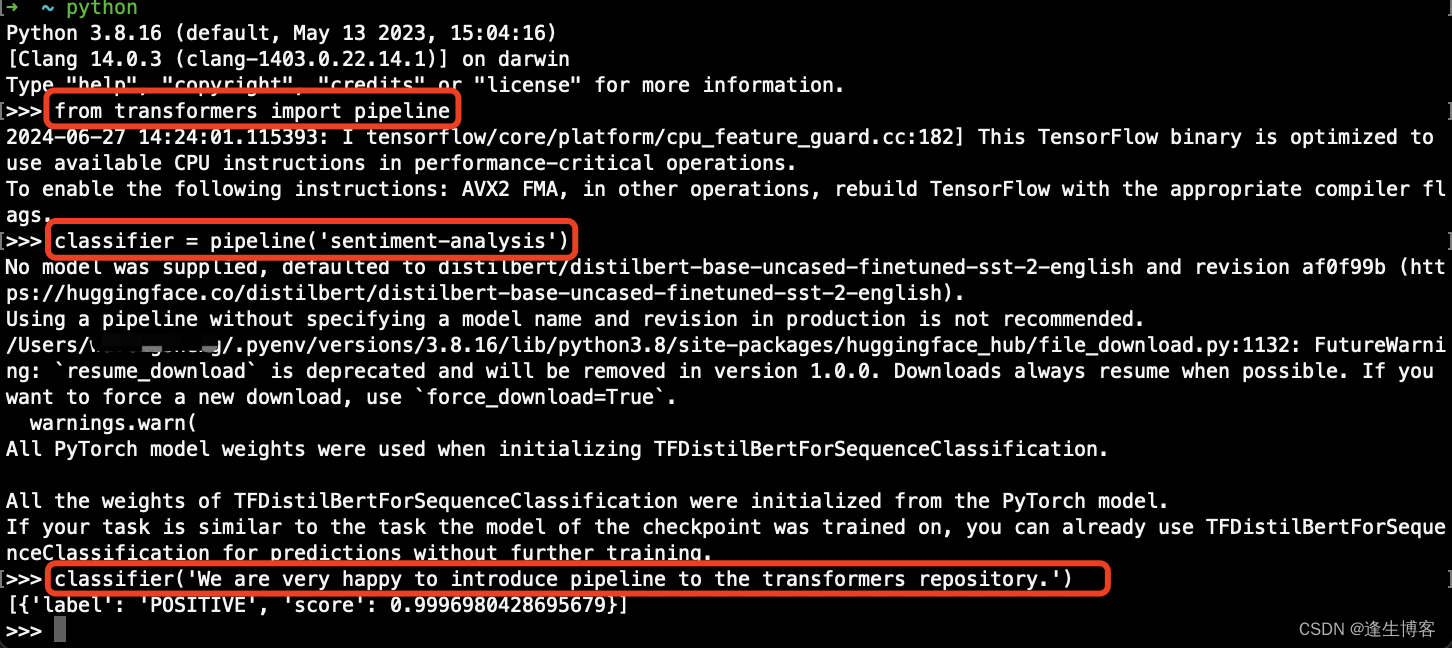
中文情感分析模型
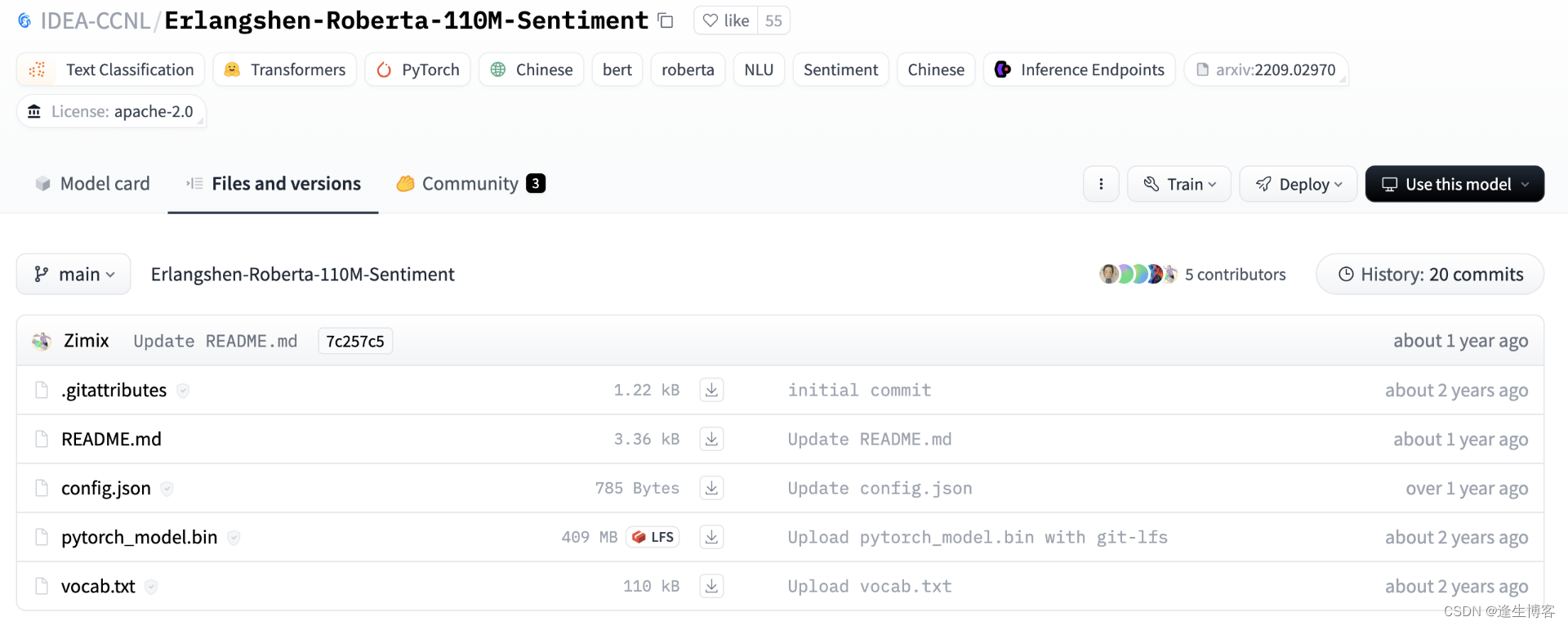
中文的RoBERTa-wwm-ext-base在数个情感分析任务微调后的版本
bash
git clone https://huggingface.co/IDEA-CCNL/Erlangshen-Roberta-110M-Sentiment
python
from transformers import BertForSequenceClassification, BertTokenizer
import torch
# 加载预训练模型和分词器
tokenizer = BertTokenizer.from_pretrained('Erlangshen-Roberta-110M-Sentiment')
model = BertForSequenceClassification.from_pretrained('Erlangshen-Roberta-110M-Sentiment')
# 待分类的文本
text = '今天心情不好'
# 对文本进行编码并转换为张量,然后输入模型中
input_ids = torch.tensor([tokenizer.encode(text)])
output = model(input_ids)
# 对输出的logits进行softmax处理,得到分类概率
probabilities = torch.nn.functional.softmax(output.logits, dim=-1)
# 打印输出分类概率
print(probabilities)
- 输出
bash
tensor([[0.9551, 0.0449]], grad_fn=<SoftmaxBackward0>)
bash
from transformers import pipeline
# 使用pipeline函数加载预训练的情感分析模型,并进行情感分析
classifier = pipeline("sentiment-analysis", model="Erlangshen-Roberta-110M-Sentiment")
# 对输入文本进行情感分析
result = classifier("今天心情很好")
# 打印输出结果
print(result)
- 输出
bash
[{'label': 'Positive', 'score': 0.9374911785125732}]
python
from transformers import AutoModelForSequenceClassification, AutoTokenizer, pipeline
# 加载预训练模型和分词器
model_path = "Erlangshen-Roberta-110M-Sentiment"
model = AutoModelForSequenceClassification.from_pretrained(model_path)
tokenizer = AutoTokenizer.from_pretrained(model_path)
# 创建情感分析的pipeline
classifier = pipeline("sentiment-analysis", model=model, tokenizer=tokenizer)
# 对文本进行情感分析
result = classifier("今天心情很好")
print(result)
- 输出
bash
[{'label': 'Positive', 'score': 0.9374911785125732}]
分词器 Tokenizer
python
from transformers import AutoTokenizer
# 加载预训练模型的分词器
tokenizer = AutoTokenizer.from_pretrained("Erlangshen-Roberta-110M-Sentiment")
# 对文本进行编码
encoded_input = tokenizer("今天心情很好")
print(encoded_input)
# 解码已编码的输入,还原原始文本
decoded_input = tokenizer.decode(encoded_input["input_ids"])
print(decoded_input)
- 输出
bash
{'input_ids': [101, 791, 1921, 2552, 2658, 2523, 1962, 102],
'token_type_ids': [0, 0, 0, 0, 0, 0, 0, 0],
'attention_mask': [1, 1, 1, 1, 1, 1, 1, 1]}
[CLS] 今 天 心 情 很 好 [SEP]
填充 Padding
模型的输入需要具有统一的形状(shape)。
python
from transformers import AutoTokenizer
tokenizer = AutoTokenizer.from_pretrained("Erlangshen-Roberta-110M-Sentiment")
batch_sentences = ["今天天气真好", "今天天气真好,适合出游"]
encoded_inputs = tokenizer(batch_sentences, padding=True)
print(encoded_inputs)
- 输出
bash
{'input_ids': [
[101, 791, 1921, 1921, 3698, 4696, 1962, 102, 0, 0, 0, 0, 0],
[101, 791, 1921, 1921, 3698, 4696, 1962, 8024, 6844, 1394, 1139, 3952, 102]],
'token_type_ids': [
[0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0],
[0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0]
],
'attention_mask': [
[1, 1, 1, 1, 1, 1, 1, 1, 0, 0, 0, 0, 0],
[1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1]
]}
截断 Truncation
句子模型无法处理,可以将句子进行截断。
python
from transformers import AutoTokenizer
tokenizer = AutoTokenizer.from_pretrained("Erlangshen-Roberta-110M-Sentiment")
batch_sentences = ["今天天气真好", "今天天气真好,适合出游"]
# return_tensors pt(PyTorch模型) tf(TensorFlow模型)
encoded_inputs = tokenizer(batch_sentences, padding=True, truncation=True, return_tensors="pt")
print(encoded_inputs)
- 输出
bash
{'input_ids': tensor([
[ 101, 791, 1921, 1921, 3698, 4696, 1962, 102, 0, 0, 0, 0, 0],
[ 101, 791, 1921, 1921, 3698, 4696, 1962, 8024, 6844, 1394, 1139, 3952, 102]
]),
'token_type_ids': tensor([
[0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0],
[0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0]
]),
'attention_mask': tensor([
[1, 1, 1, 1, 1, 1, 1, 1, 0, 0, 0, 0, 0],
[1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1]
])
}
google-t5/t5-small
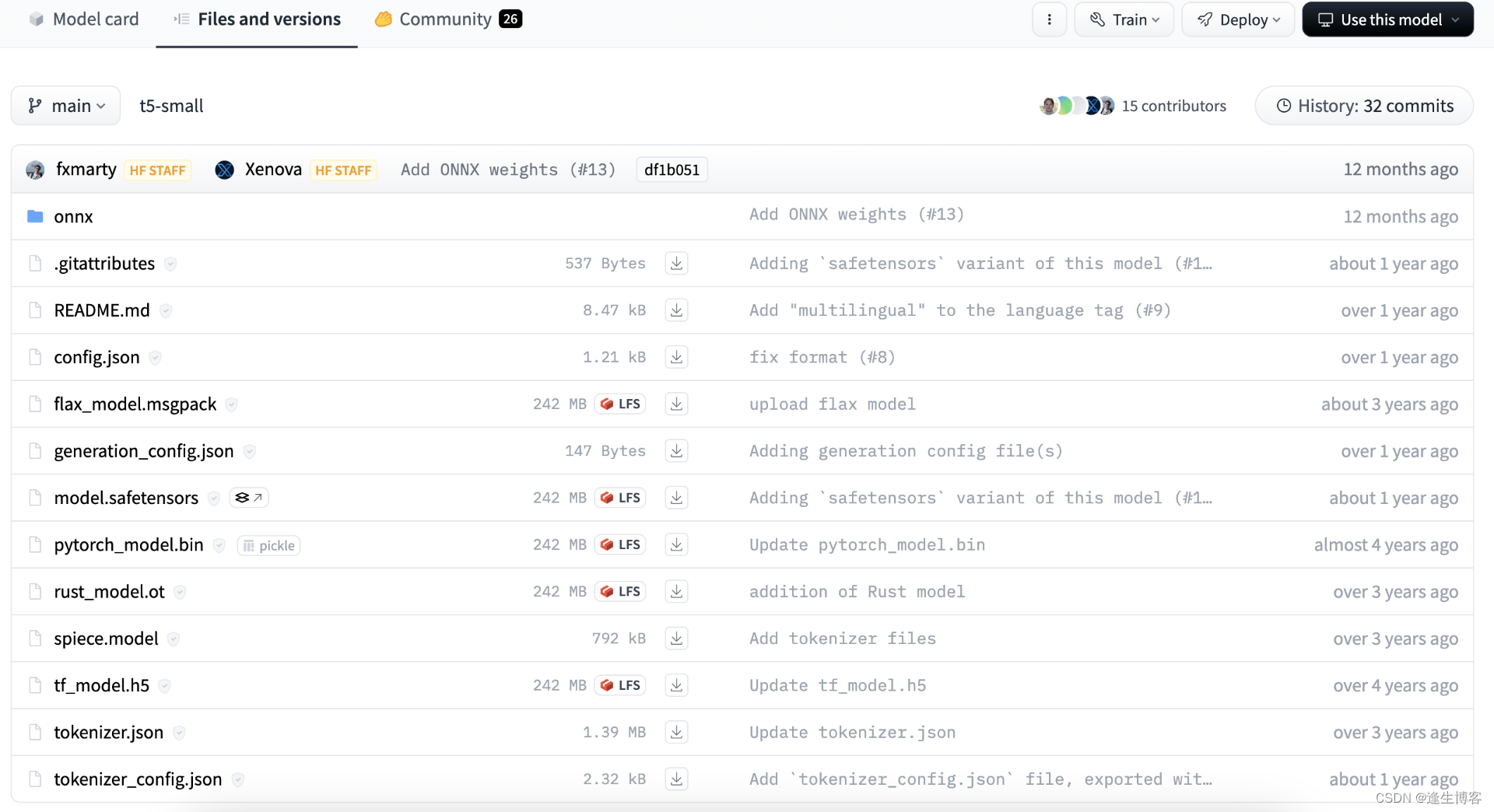
Google的T5(Text-To-Text Transfer Transformer)是由Google Research开发的一种多功能的基于Transformer的模型。T5-small是T5模型的一个较小的变体,专为涉及自然语言理解和生成任务而设计。
-
Transformer架构:与其它模型类似,T5-small采用了Transformer架构,该架构在各种自然语言处理(NLP)任务中表现出色。
-
多功能性:T5-small的设计理念是将所有的NLP任务都看作文本到文本的转换问题,使得模型可以通过简单地调整输入和输出来适应不同的任务。
-
预训练和微调:T5-small通常通过大规模的无监督预训练来学习通用的语言表示,然后通过有监督的微调来适应特定任务,如问答、摘要生成等。
-
应用广泛:由于其灵活性和性能,在各种NLP应用中都有广泛的应用,包括机器翻译、文本生成、情感分析等。
- 下载 google-t5/t5-small 模型
bash
# 模型大小 4.49G
git clone https://huggingface.co/google-t5/t5-small
- 安装依赖库
bash
pip install 'transformers[torch]'
pip install sentencepiece
- 文本生成示例
python
from transformers import T5Tokenizer, T5ForConditionalGeneration
# Step 1: 加载预训练的T5 tokenizer和模型
tokenizer = T5Tokenizer.from_pretrained("t5-small")
model = T5ForConditionalGeneration.from_pretrained("t5-small")
while True:
# Step 2: 接收用户输入
input_text = input("请输入要生成摘要的文本 (输入 'exit' 结束): ")
if input_text.lower() == 'exit':
print("程序结束。")
break
# 使用tokenizer对输入文本进行编码
input_ids = tokenizer(input_text, return_tensors="pt").input_ids
# Step 3: 进行生成
# 使用model.generate来生成文本
output = model.generate(input_ids, max_length=50, num_beams=4, early_stopping=True)
# Step 4: 解码输出
output_text = tokenizer.decode(output[0], skip_special_tokens=True)
# 打印输入和输出结果
print("输入:", input_text)
print("输出:", output_text)
print("=" * 50) # 分隔符,用来区分不同输入的输出结果
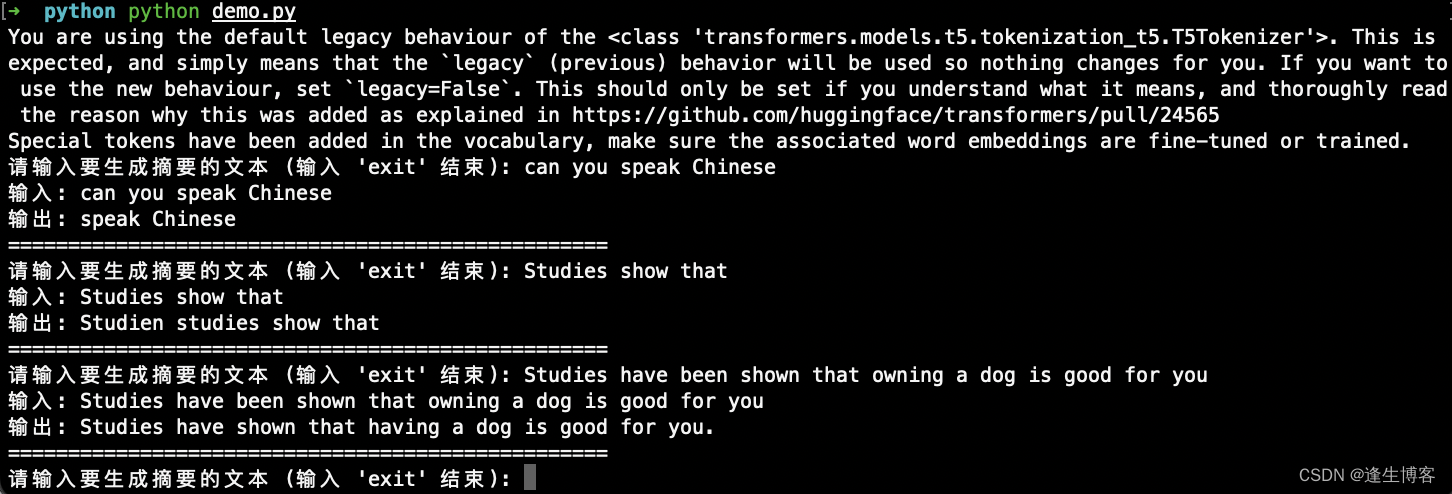
使用脚本进行训练
-
从源代码安装 Transformers
bash
git clone https://github.com/huggingface/transformers
cd transformers
pip install .
- 将当前的 Transformers 克隆切换到特定版本
bash
# 本地分支
git branch
# 远程分支
git branch -a
# 切换分支 v4.41.2,因为当前安装的版本是 v4.41.2
git checkout tags/v4.41.2
- 安装依赖库
bash
# 安装用于处理人类语言数据的工具集库
pip install nltk
# 安装用于计算ROUGE评估指标库
pip install rouge_score
Pytorch
示例脚本从 🤗 Datasets库下载并预处理数据集。然后,该脚本使用Trainer在支持摘要的架构上微调数据集。以下示例展示了如何在CNN/DailyMail数据集上微调T5-small。由于训练方式的原因,T5 模型需要额外的参数。此提示让 T5 知道这是一项摘要任务。
bash
cd transformers/examples/pytorch/summarization
pip install -r requirements.txt
bash
python run_summarization.py \
--model_name_or_path google-t5/t5-small \
--do_train \
--do_eval \
--dataset_name cnn_dailymail \
--dataset_config "3.0.0" \
--source_prefix "summarize: " \
--output_dir /tmp/tst-summarization \
--per_device_train_batch_size=4 \
--per_device_eval_batch_size=4 \
--overwrite_output_dir \
--predict_with_generate
注意: 家用机上训练非常耗时,建议租用GPU服务器进行测试。
- 数据缓存目录
bash
# Linux/macOS
cd ~/.cache/huggingface
# Windows
C:\Users\{your_username}\.cache\huggingface
- datasets
bash
2.6G cnn_dailymail
798M downloads
机器翻译
数据集下载
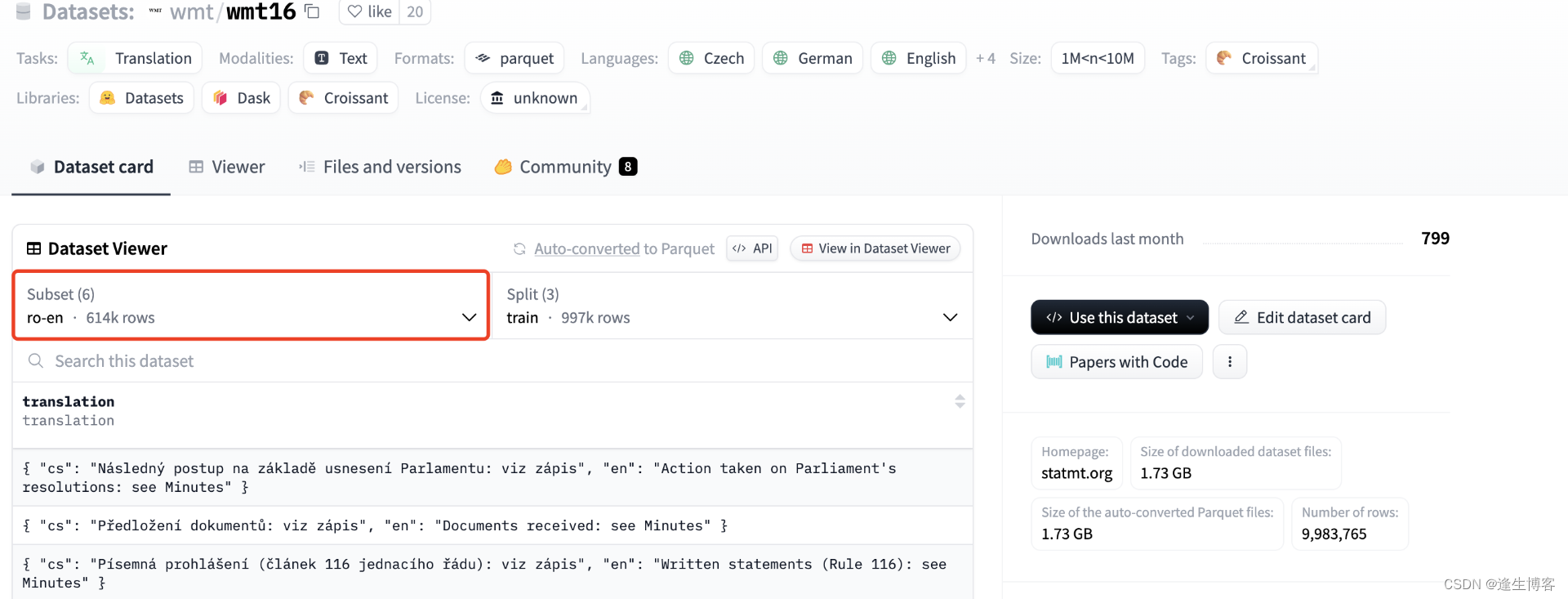
数据集格式转换
bash
pip install pandas
python
import pandas as pd
import jsonlines
# 输入和输出文件路径
input_parquet_file = './input_file.parquet'
output_jsonl_file = './output_file.jsonl'
# 加载 Parquet 文件
df = pd.read_parquet(input_parquet_file)
# 将数据写入 JSONLines 文件
with jsonlines.open(output_jsonl_file, 'w') as writer:
for index, row in df.iterrows():
json_record = {
"source_text": row['source_column'], # 替换成实际的源语言列名
"target_text": row['target_column'] # 替换成实际的目标语言列名
}
writer.write(json_record)
- train.jsonl
json
{ "cs": "Následný postup na základě usnesení Parlamentu: viz zápis", "en": "Action taken on Parliament's resolutions: see Minutes" }
- validation.jsonl
json
{ "en": "UN Chief Says There Is No Military Solution in Syria", "ro": "Șeful ONU declară că nu există soluții militare în Siria" }
bash
cd examples/pytorch/translation
pip install -r requirements.txt
bash
python run_translation.py \
--model_name_or_path google-t5/t5-small \
--do_train \
--do_eval \
--source_lang en \
--target_lang ro \
--source_prefix "translate English to Romanian: " \
--dataset_name wmt16 \
--dataset_config_name ro-en \
--train_file ./train.jsonl \
--validation_file ./validation.jsonl \
--output_dir /tmp/tst-translation \
--per_device_train_batch_size=4 \
--per_device_eval_batch_size=4 \
--overwrite_output_dir \
--predict_with_generate
注意: 家用机上训练非常耗时,建议租用GPU服务器进行测试。