概念
本文在上一文章搭建完数据库,以及创建好项目之后,以及前端静态文件后,对项目的首页功能开发。
后端代码编写
在views.py文件中创建方法,连接数据库,并获取首页需要的数据
python
def getGoodsList(type):
# 获取所有横幅滚动商品id
res = Recommend.objects.filter(type=type)
goodsIds = []
for r in res:
goodsIds.append(r.goods_id)
# 根据推荐栏的商品信息
goods = Goods.objects.filter(id__in=tuple(goodsIds))
if type !=1:
# 将实体类对象转换成字典进行遍历
for g in goods.values():
t = Type.objects.get(id=g["type_id"]).name
g["typename"] = t
return goods
python
# 处理首页的请求
def index(request):
# 获取所有商品分类信息
global types
types=Type.objects.all()
# 根据推荐栏的商品
scrolls=getGoodsList(1)
# 获取新品
newList=getGoodsList(3)
# 获取热品
hotList=getGoodsList(2)
return render(request,"index.html",{"typeList":types,"scroll":scrolls,"newList":newList,"hotList":hotList})
前端页面搭建
在子项目中创建templates文件夹,在该文件夹下创建header.html,foot.html以及index.html作为首页的前端内容
header.html
html
<div class="header">
<div class="container">
<nav class="navbar navbar-default" role="navigation">
<div class="navbar-header">
<button type="button" class="navbar-toggle" data-toggle="collapse" data-target="#bs-example-navbar-collapse-1">
<span class="sr-only">Toggle navigation</span>
<span class="icon-bar"></span>
<span class="icon-bar"></span>
<span class="icon-bar"></span>
</button>
<h1 class="navbar-brand"><a href="/"></a></h1>
</div>
<!--navbar-header-->
<div class="collapse navbar-collapse" id="bs-example-navbar-collapse-1">
<ul class="nav navbar-nav">
<li><a href="/"
{% if flag == 1 %}
class="active"
{% endif %}
>首页</a></li>
<li class="dropdown">
<a href="#" class="dropdown-toggle
{% if flag == 2 %}
active
{% endif %}
" data-toggle="dropdown">商品分类<b class="caret"></b></a>
<ul class="dropdown-menu multi-column columns-2">
<li>
<div class="row">
<div class="col-sm-12">
<h4>商品分类</h4>
<ul class="multi-column-dropdown">
<li><a class="list" href="/goods_list">全部系列</a></li>
{% for t in typeList%}
<li><a class="list" href="/goods_list?typeid={{ t.id }}">{{t.name}}</a></li>
{% endfor %}
</ul>
</div>
</div>
</li>
</ul>
</li>
<li><a href="/goodsrecommend_list?type=2" {% if flag == 3 and t == 2 %} class="active" {% endif %}>热销</a></li>
<li><a href="/goodsrecommend_list?type=3" {% if flag == 3 and t == 3 %} class="active" {% endif %}>新品</a></li>
{% if user %}
<li><a href="/order_list" {% if flag == 5 %} class="active" {% endif %}>我的订单</a></li>
<li><a href="/user_center.jsp" {% if flag == 4 %} class="active" {% endif %}>个人中心</a></li>
<li><a href="/user_logout" >退出</a></li>
{% else %}
<li><a href="/user_register.jsp" {% if flag == 10 %} class="active" {% endif %}>注册</a></li>
<li><a href="/user_login.jsp" {% if flag == 9 %} class="active" {% endif %}>登录</a></li>
{% endif %}
{% if user and user.isadmin %}
<li><a href="/admin/index.jsp" target="_blank">后台管理</a></li>
{% endif %}
</ul>
<!--/.navbar-collapse-->
</div>
<!--//navbar-header-->
</nav>
<div class="header-info">
<div class="header-right search-box">
<a href="javascript:;"><span class="glyphicon glyphicon-search" aria-hidden="true"></span></a>
<div class="search">
<form class="navbar-form" action="/goods_search">
<input type="text" class="form-control" name="keyword">
<button type="submit" class="btn btn-default
{% if flag == 7 %}
active
{% endif %}
" aria-label="Left Align">搜索</button>
</form>
</div>
</div>
<div class="header-right cart">
<a href="goods_cart.jsp">
<span class="glyphicon glyphicon-shopping-cart
{% if flag == 8 %}
active
{% endif %}
" aria-hidden="true"><span class="card_num">
{% if order %}
{{ order.amount }}
{% else %}
0
{% endif %}
</span></span>
</a>
</div>
<div class="clearfix"> </div>
</div>
<div class="clearfix"> </div>
</div>
</div>
foot.html
html
<div class="footer">
<div class="container">
<div class="text-center">
</div>
</div>
</div>
index.html
python
<!DOCTYPE html>
<html>
<head>
{% load static %}
<title>首页</title>
<meta name="viewport" content="width=device-width, initial-scale=1">
<meta http-equiv="Content-Type" content="text/html; charset=utf-8">
<link type="text/css" rel="stylesheet" href="{% static 'css/bootstrap.css' %}">
<link type="text/css" rel="stylesheet" href="{% static 'css/style.css' %}">
<script type="text/javascript" src="{% static 'js/jquery.min.js' %}"></script>
<script type="text/javascript" src="{% static 'js/bootstrap.min.js' %}"></script>
<script type="text/javascript" src="{% static 'layer/layer.js' %}"></script>
<script type="text/javascript" src="{% static 'js/cart.js' %}"></script>
</head>
<body>
<!--header-->
{% include "header.html" with flag=1 typeList=typeList %}
<!--banner-->
<div class="banner">
<div class="container">
<div id="carousel-example-generic" class="carousel slide" data-ride="carousel">
<!-- Indicators -->
<ol class="carousel-indicators" id="olnum">
{% for g in scroll %}
{% if forloop.first %}
<li data-target="#carousel-example-generic" data-slide-to="0" class="active"></li>
{% else %}
<li data-target="#carousel-example-generic" data-slide-to="{{ forloop.counter0 }}"></li>
{% endif %}
{% endfor %}
</ol>
<!-- Wrapper for slides -->
<div class="carousel-inner" role="listbox" id="lunbotu" style="width: 1242px; height: 432px;">
{% for g in scroll %}
{% if forloop.first %}
<div class="item active">
<h2 class="hdng"><a href="/goods_detail?id={{ g.id }}">{{ g.name }}</a><span></span></h2>
<p>今日精选推荐</p>
<a class="banner_a" href="javascript:;" onclick="buy({{ g.id }})">立刻购买</a>
<div class="banner-text">
<a href="/goods_detail?id={{ g.id }}">
<img src="{% static g.cover %}" alt="{{ g.name }}" width="350" height="350">
</a>
</div>
</div>
{% else %}
<div class="item">
<h2 class="hdng"><a href="/goods_detail?id={{ g.id }}">{{ g.name }}</a><span></span></h2>
<p>今日精选推荐</p>
<a class="banner_a" href="javascript:;" onclick="buy({{ g.id }})">立刻购买</a>
<div class="banner-text">
<a href="/goods_detail?id={{ g.id }}">
<img src="{% static g.cover %}" alt="{{ g.name }}" width="350" height="350">
</a>
</div>
</div>
{% endif %}
{% endfor %}
</div>
</div>
</div>
</div>
<!--//banner-->
<div class="subscribe2"></div>
<!--gallery-->
<div class="gallery">
<div class="container">
<div class="alert alert-danger">热销推荐</div>
<div class="gallery-grids">
{% for g in hotList %}
<div class="col-md-4 gallery-grid glry-two">
<a href="/goods_detail?id={{ g.id }}">
<img src="{% static g.cover %}" class="img-responsive" alt="{{ g.name }}" width="350" height="350"/>
</a>
<div class="gallery-info galrr-info-two">
<p>
<span class="glyphicon glyphicon-eye-open" aria-hidden="true"></span>
<a href="/goods_detail?id={{ g.id }}">查看详情</a>
</p>
<a class="shop" href="javascript:;" onclick="buy({{ g.id }})">立刻购买</a>
<div class="clearfix"></div>
</div>
<div class="galy-info">
<p>{{ g.typeName }} > {{ g.name }}</p>
<div class="galry">
<div class="prices">
<h5 class="item_price">¥ {{ g.price }}</h5>
</div>
<div class="clearfix"></div>
</div>
</div>
</div>
{% endfor %}
</div>
<div class="clearfix"></div>
<div class="alert alert-info">新品推荐</div>
<div class="gallery-grids">
{% for g in newList %}
<div class="col-md-3 gallery-grid ">
<a href="/goods_detail?id={{ g.id }}">
<img src="{% static g.cover %}" class="img-responsive" alt="{{ g.name }}"/>
</a>
<div class="gallery-info">
<p>
<span class="glyphicon glyphicon-eye-open" aria-hidden="true"></span>
<a href="/goods_detail?id={{ g.id }}">查看详情</a>
</p>
<a class="shop" href="javascript:;" onclick="buy({{ g.id }})">立刻购买</a>
<div class="clearfix"></div>
</div>
<div class="galy-info">
<p>{{ g.typeName }} > {{ g.name }}</p>
<div class="galry">
<div class="prices">
<h5 class="item_price">¥ {{ g.price }}</h5>
</div>
<div class="clearfix"></div>
</div>
</div>
</div>
{% endfor %}
</div>
</div>
</div>
<!--//gallery-->
<!--subscribe-->
<div class="subscribe"></div>
<!--//subscribe-->
<!--footer-->
{% include "footer.html" %}
</body>
</html>
在主项目的urls.py文件中定义请求地址,用于浏览器访问views.py文件
python
path('',views.index),
在PyCharm的内置dos窗口中输入以下指令启动服务器
python
python manage.py runserver
浏览器中访问该地址
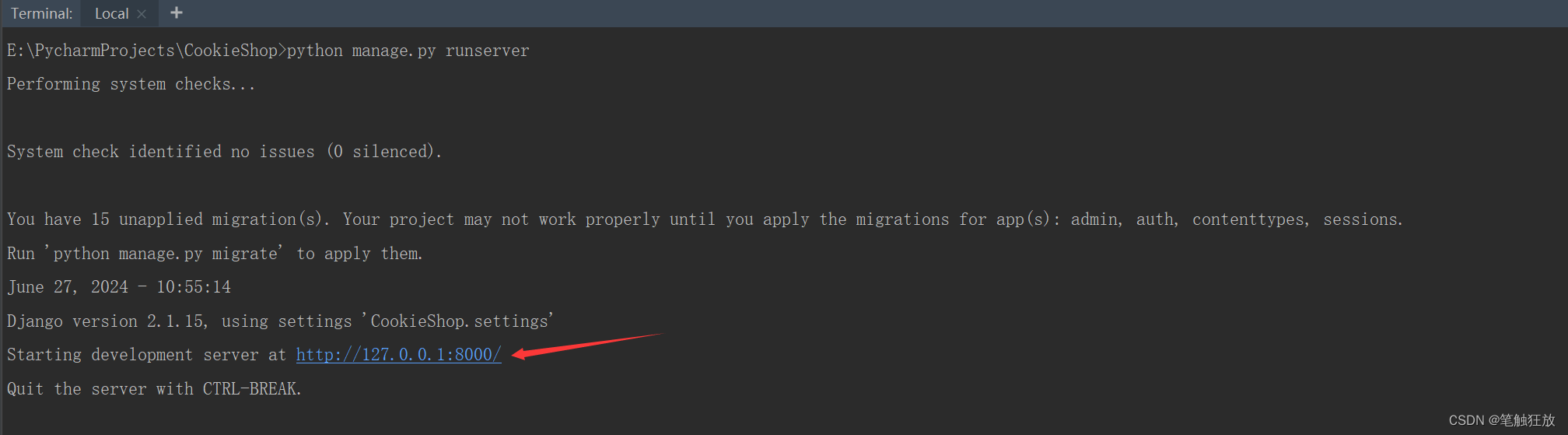
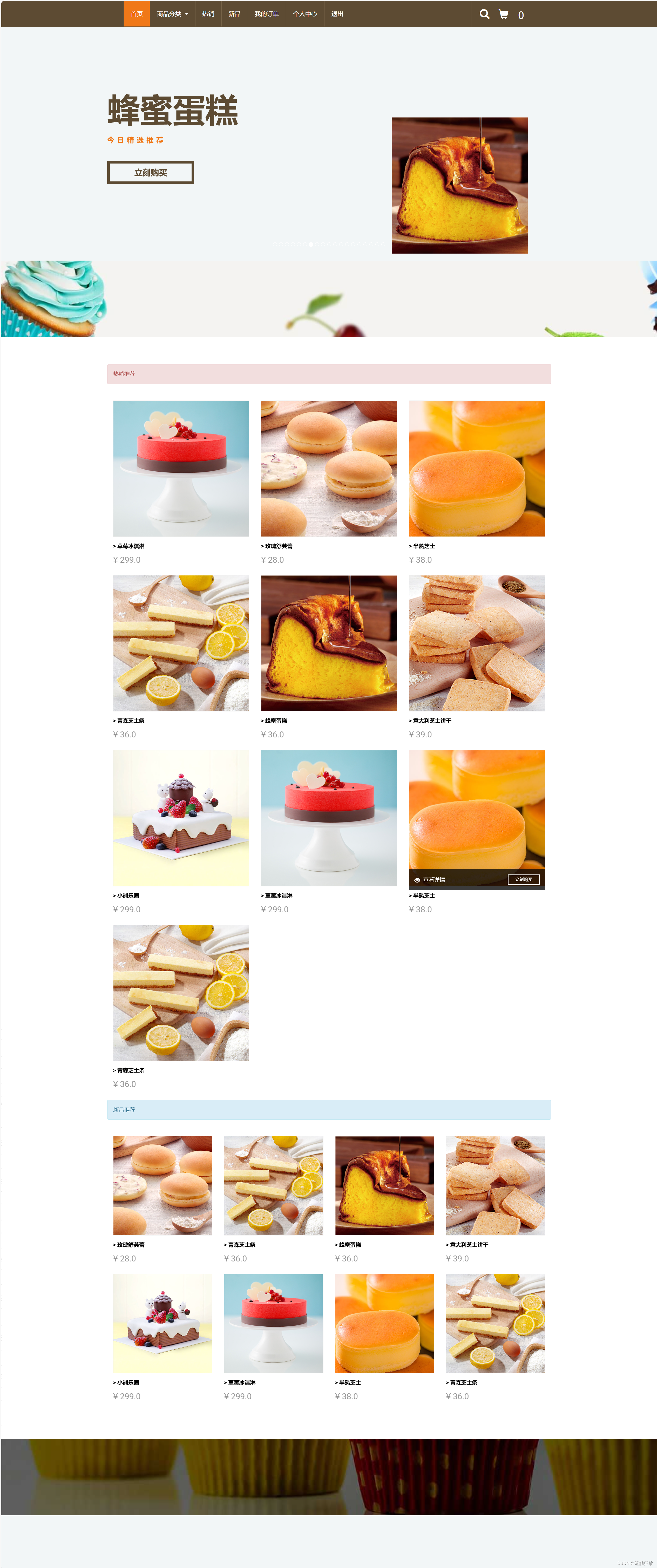