160. 相交链表
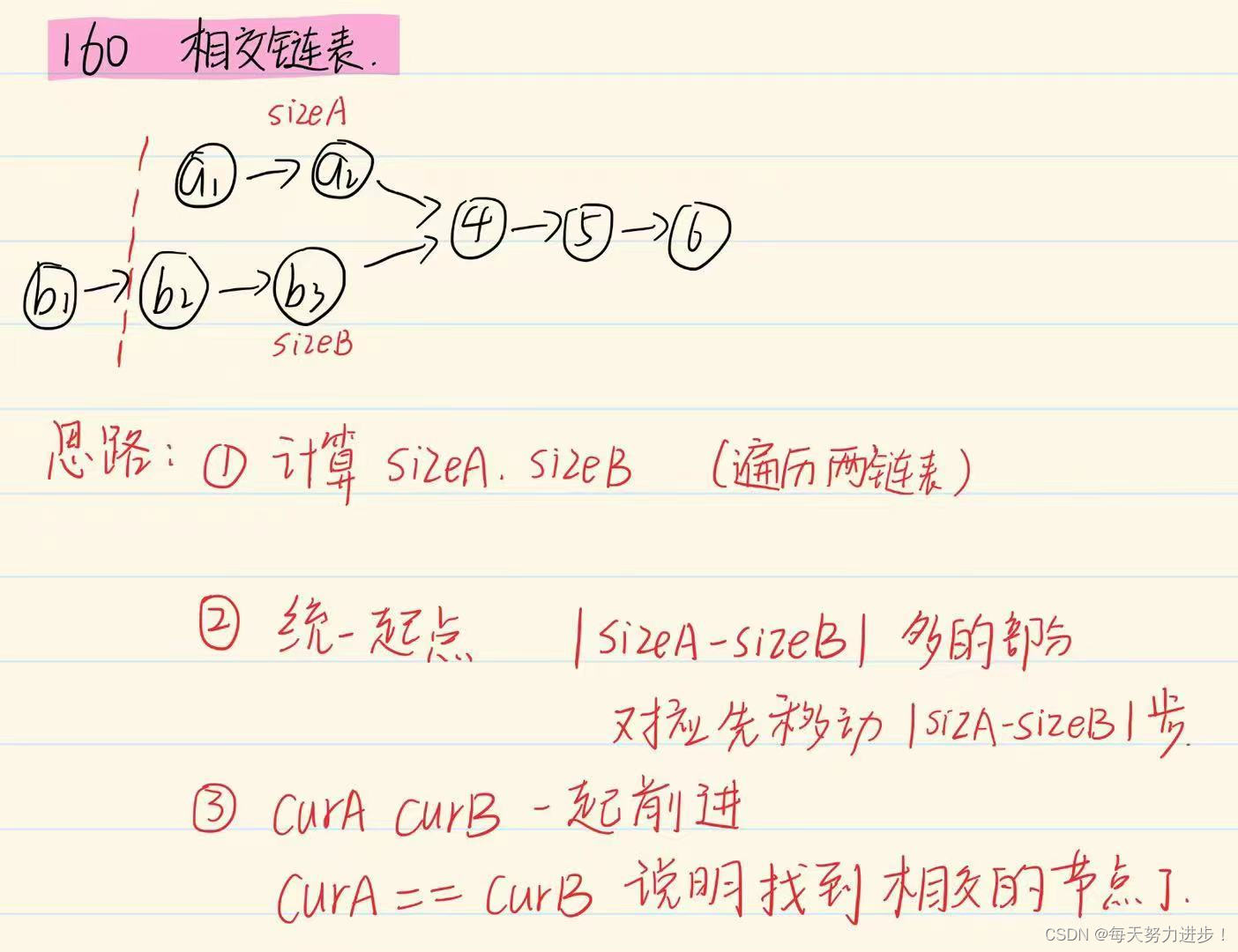
cpp
/**
* Definition for singly-linked list.
* struct ListNode {
* int val;
* ListNode *next;
* ListNode(int x) : val(x), next(NULL) {}
* };
*/
class Solution {
public:
ListNode *getIntersectionNode(ListNode *headA, ListNode *headB) {
ListNode* curA = headA;
ListNode* curB = headB;
int sizeA=0,sizeB=0;
while(curA != nullptr) {
sizeA++;
curA = curA->next;
}
while(curB != nullptr) {
sizeB++;
curB = curB->next;
}
curA = headA;
curB = headB;
if(sizeA > sizeB) {
int temp = sizeA - sizeB;
while(temp) {
curA = curA->next;
temp--;
}
}
else if(sizeA < sizeB) {
int temp = sizeB - sizeA;
while(temp) {
curB = curB->next;
temp--;
}
}
while(curA != curB) {
curA = curA->next;
curB = curB->next;
}
if(curA == NULL || curB == NULL)
return NULL;
else
return curA;
}
};
206. 反转链表
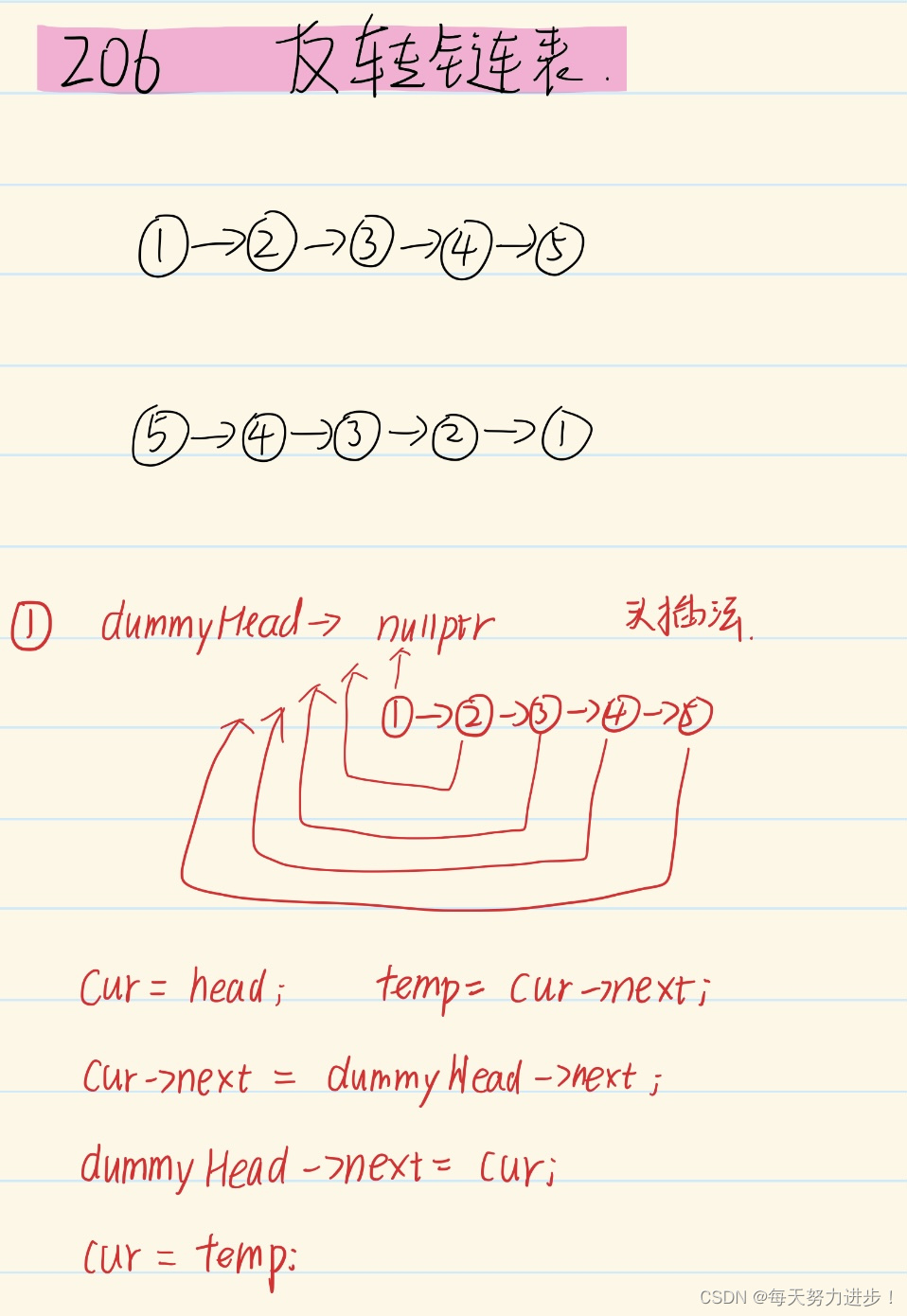
cpp
/**
* Definition for singly-linked list.
* struct ListNode {
* int val;
* ListNode *next;
* ListNode() : val(0), next(nullptr) {}
* ListNode(int x) : val(x), next(nullptr) {}
* ListNode(int x, ListNode *next) : val(x), next(next) {}
* };
*/
class Solution {
public:
ListNode* reverseList(ListNode* head) {
ListNode* dummyHead = new ListNode(0);
dummyHead->next = nullptr;
ListNode* cur = head;
while(cur != nullptr) {
ListNode* temp = cur->next;
cur->next = dummyHead->next;
dummyHead->next = cur;
cur = temp;
}
return dummyHead->next;
}
};
234. 回文链表
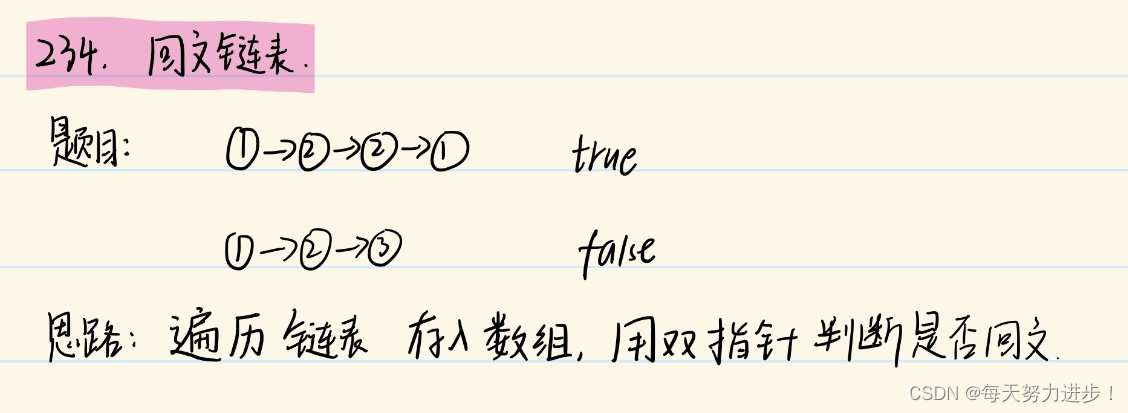
cpp
/**
* Definition for singly-linked list.
* struct ListNode {
* int val;
* ListNode *next;
* ListNode() : val(0), next(nullptr) {}
* ListNode(int x) : val(x), next(nullptr) {}
* ListNode(int x, ListNode *next) : val(x), next(next) {}
* };
*/
class Solution {
public:
bool isPalindrome(ListNode* head) {
if(head==nullptr || head->next==nullptr)
return true;
vector<int> nums;
while(head!=nullptr) {
nums.push_back(head->val);
head = head->next;
}
int size = nums.size();
int i=0,j=size-1;
while(i<j) {
if(nums[i] == nums[j]) {
i++;
j--;
continue;
}
else
return false;
}
return true;
}
};
141. 环形链表
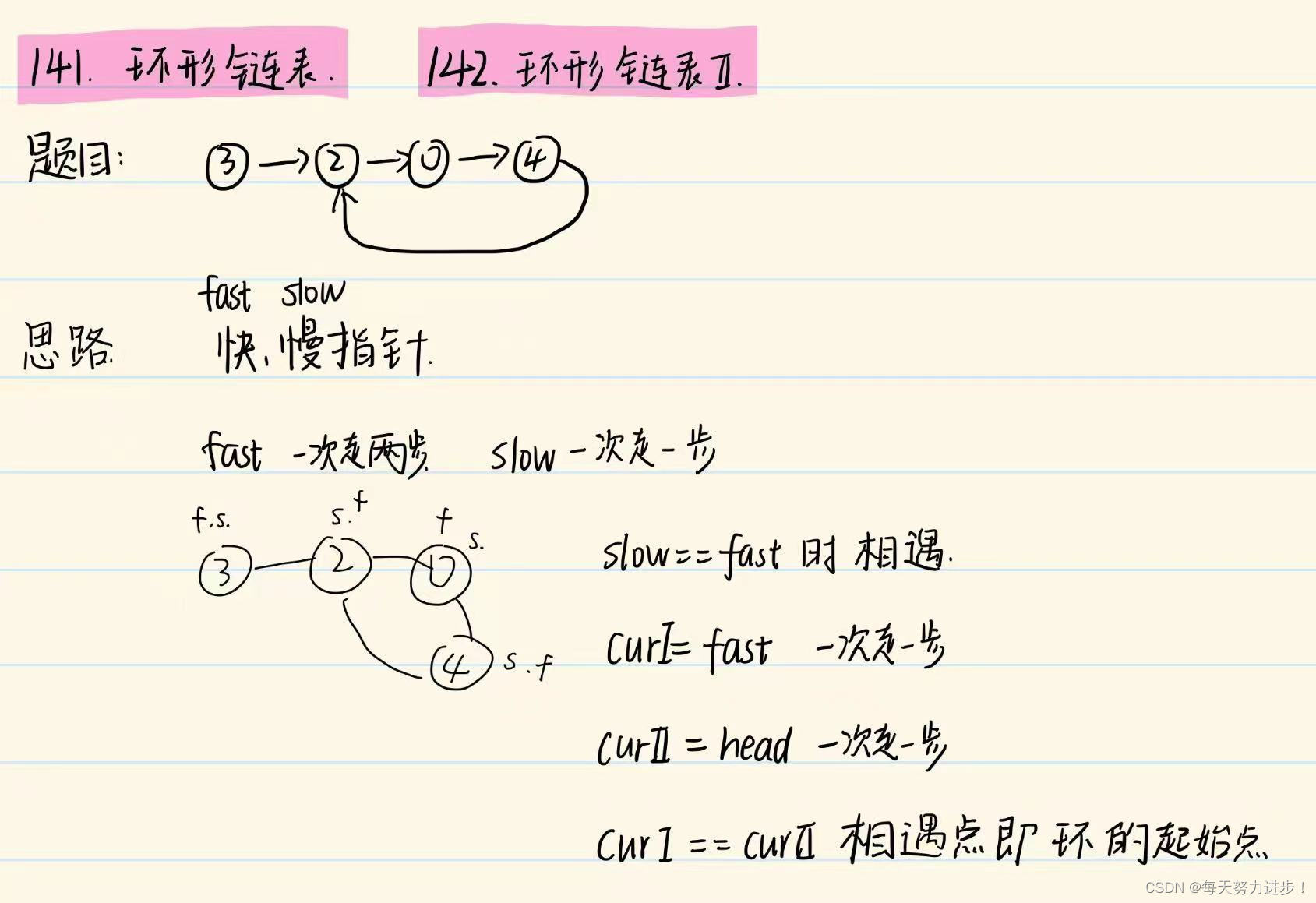
cpp
/**
* Definition for singly-linked list.
* struct ListNode {
* int val;
* ListNode *next;
* ListNode(int x) : val(x), next(NULL) {}
* };
*/
class Solution {
public:
bool hasCycle(ListNode *head) {
if(head==nullptr || head->next == nullptr)
return false;
ListNode* fast = head;
ListNode* slow = head;
while(fast && fast->next) { //这里保证fast->next也不为空是因为若为空 不存在->next了
fast = fast->next->next;
slow = slow->next;
if(slow == fast)
return true;
}
return false;
}
};
142. 环形链表 II
加粗样式
cpp
/**
* Definition for singly-linked list.
* struct ListNode {
* int val;
* ListNode *next;
* ListNode(int x) : val(x), next(NULL) {}
* };
*/
class Solution {
public:
bool hasCycle(ListNode *head) {
if(head==nullptr || head->next == nullptr)
return false;
ListNode* fast = head;
ListNode* slow = head;
while(fast && fast->next) { //这里保证fast->next也不为空是因为若为空 不存在->next了
fast = fast->next->next;
slow = slow->next;
if(slow == fast)
return true;
}
return false;
}
};