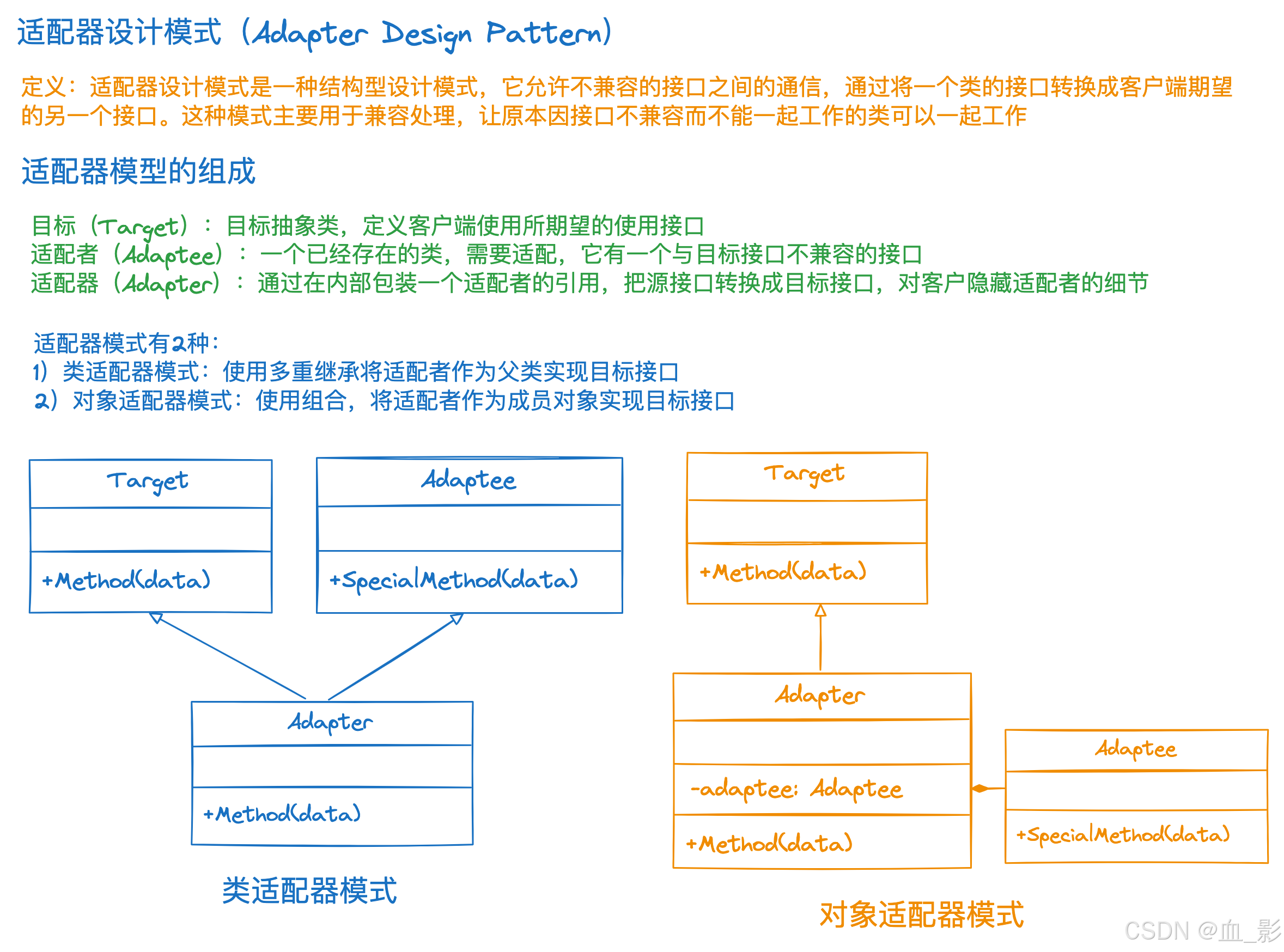
类适配器
c
#include <queue>
#include <iostream>
#include <algorithm>
#include <iterator>
using namespace std;
// 目标接口
class Target
{
public:
virtual ~Target() {}
virtual void method() = 0;
};
// 适配者类
class Adaptee
{
public:
void spec_method()
{
std::cout << "spec_method run" << std::endl;
}
};
// 类适配器
class Adapter : public Target, public Adaptee
{
public:
void method() override
{
std::cout << "call from Adapter: " << std::endl;
spec_method();
}
};
int main(int argc, char** argv)
{
Adaptee adaptee;
adaptee.spec_method();
std::cout << std::endl;
Adapter adapter;
adapter.method();
return 0;
}
对象适配器
c
#include <queue>
#include <iostream>
#include <algorithm>
#include <iterator>
using namespace std;
// 目标接口
class Target
{
public:
virtual ~Target() {}
virtual void method() = 0;
};
// 适配者类
class Adaptee
{
public:
void spec_method()
{
std::cout << "spec_method run" << std::endl;
}
};
// 类适配器
class Adapter : public Target
{
public:
Adapter(Adaptee* adaptee)
: adaptee_{adaptee}
{
}
void method() override
{
std::cout << "call from Adapter: " << std::endl;
adaptee_->spec_method();
}
private:
Adaptee* adaptee_;
};
int main(int argc, char** argv)
{
Adaptee adaptee;
adaptee.spec_method();
std::cout << std::endl;
Adapter adapter(&adaptee);
adapter.method();
return 0;
}