206.反转链表
给你单链表的头节点 head
,请你反转链表,并返回反转后的链表。
示例 1:
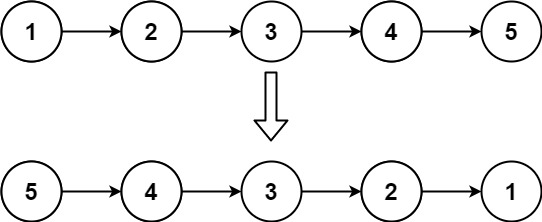
输入:head = [1,2,3,4,5]
输出:[5,4,3,2,1]
示例 2:
输入:head = [1,2]
输出:[2,1]
示例 3:
输入:head = []
输出:[]
提示:
- 链表中节点的数目范围是
[0, 5000]
-5000 <= Node.val <= 5000
python
# Definition for singly-linked list.
# class ListNode:
# def __init__(self, val=0, next=None):
# self.val = val
# self.next = next
class Solution:
def reverseList(self, head: Optional[ListNode]) -> Optional[ListNode]:
if head is None:
return None
elif head.next is None:
return head
else:
dummy = ListNode()
p = head
while p.next is not None:
p = p.next
dummy.next = p
while 1:
p = head
while p.next.next is not None:
p = p.next
p.next.next = p
p.next = None
if head.next is None:
return dummy.next
python
# Definition for singly-linked list.
# class ListNode:
# def __init__(self, val=0, next=None):
# self.val = val
# self.next = next
class Solution:
def reverseList(self, head: Optional[ListNode]) -> Optional[ListNode]:
if head is None:
return None
elif head.next is None:
return head
else:
q = None
p = head
while p is not None:
j = p.next
p.next = q
q = p
p = j
return q
总结
从后往前,不能遍历到最后一个,只能遍历到倒数第二个。
从前往后,需要三个指针,多一个存储下一个节点。