题目:
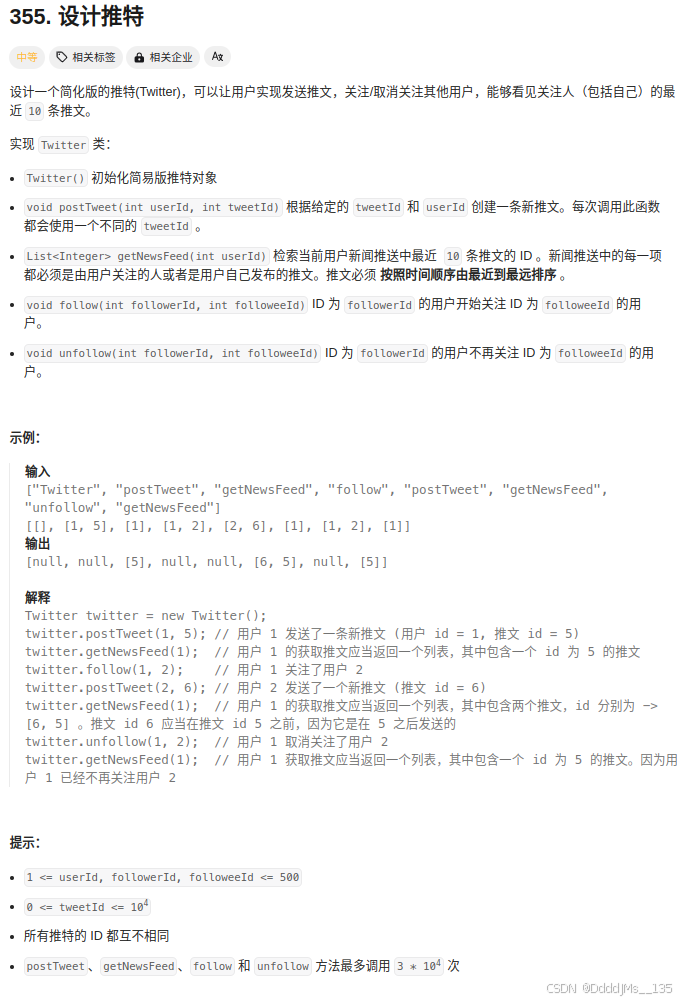
题解:
cpp
typedef struct {
int tweetId;
int userId;
} Tweet;
typedef struct {
int* dict[501];
Tweet* tweetList;
int tweetListLen;
} Twitter;
Twitter* twitterCreate() {
Twitter* obj = malloc(sizeof(Twitter));
for (int i = 0; i < 501; i++) {
obj->dict[i] = calloc(501, sizeof(int));
obj->dict[i][i] = 1;
}
obj->tweetList = malloc(30000 * sizeof(Tweet));
obj->tweetListLen = 0;
return obj;
}
void twitterPostTweet(Twitter* obj, int userId, int tweetId) {
obj->tweetList[obj->tweetListLen].tweetId = tweetId;
obj->tweetList[obj->tweetListLen].userId = userId;
obj->tweetListLen++;
return;
}
int* twitterGetNewsFeed(Twitter* obj, int userId, int* retSize) {
int* res = malloc(10 * sizeof(int));
*retSize = 0;
for (int i = obj->tweetListLen - 1; i >= 0; i--) {
if (obj->dict[userId][obj->tweetList[i].userId] == 1) {
res[*retSize] = obj->tweetList[i].tweetId;
(*retSize)++;
}
if (*retSize == 10) {
break;
}
}
return res;
}
void twitterFollow(Twitter* obj, int followerId, int followeeId) {
obj->dict[followerId][followeeId] = 1;
return;
}
void twitterUnfollow(Twitter* obj, int followerId, int followeeId) {
obj->dict[followerId][followeeId] = 0;
return;
}
void twitterFree(Twitter* obj) {
for (int i = 0; i < 501; i++) {
free(obj->dict[i]);
}
free(obj->tweetList);
free(obj);
return;
}