字符分类函数
头文件:
ctype.h
|----------|------------------------------------|
| 函数 | 功能 |
| iscntrl | 判断字符是否为控制字符 |
| isspace | 判断字符是否为空白字符(空格,换页、换行、回车、制表符或垂直制表符) |
| isdigit | 判断字符是否为十进制数字 |
| isxdigit | 判断字符是否为十六进制数字(0-9)(a-f)(A-F) |
| isupper | 判断字符是否为大写英文字母 |
| islower | 判断字符是否为小写英文字母 |
| isalpha | 判断字符是否为英文字母 |
| isalnum | 判断字符是否为字母或数字 |
| ispunct | 判断字符是否为标点符号 |
字符转换函数:
|---------|--------------|
| 函数 | 功能 |
| tolower | 把大写字母转换为小写字母 |
| toupper | 把小写字母转换为大写字母 |
cpp
#include <stdio.h>
#include <ctype.h>
int main() {
char ch1, ch2;
printf("小写转大写:\n");
printf("input a character:");
scanf("%c", &ch1);
ch2 = toupper(ch1);
printf("transform %c to %c.\n", ch1, ch2);
printf("大写转小写:\n");
char str[1024] = "Hello World!";
char* p_str = str;
char res[1024] = { 0 };
char* p_res = res;
while (*p_str) {
if (isupper(*p_str)) {
*p_res = tolower(*p_str);
}
else {
*p_res = *p_str;
}
p_str++;
p_res++;
}
printf("%s transform:%s\n", str, res);
return 0;
}
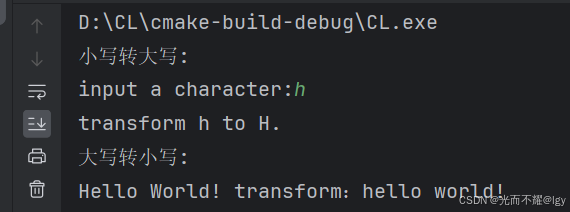
内存函数:
1.memcpy
原型:void * memcpy ( void * destination, const void * source, size_t num );
原理:从source开始位置向后复制num个字节的数据到destination的内存位置
cpp
#include <stdio.h>
#include <string.h>
typedef struct Stu{
char name[1024];
int age;
}S;
int main() {
int nums[] = { 1,2,3,4 };
int numsBak[10] = { 0 };
memcpy(numsBak, nums, sizeof(nums));
int length = sizeof(nums) / sizeof(nums[0]);
for (int i = 0; i < length; i++) {
printf("%d\n", numsBak[i]);
}
// 结构体的拷贝
S students[] = { {"xiaoming",10},{"xiaozhao",30} };
S studentsBak[3] = { 0 };
memcpy(studentsBak, students, sizeof(students));
for (int i = 0; i < 3; i++) {
S student = studentsBak[i];
printf("uname:%s,age:%d\n", student.name, student.age);
}
return 0;
}
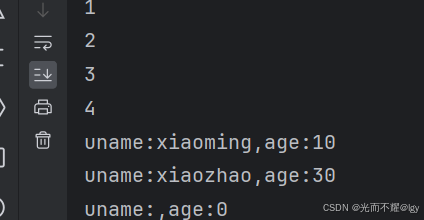
注意:该函数不检测源中的任何终止字符,它总是精确地复制num个字符
eg:
cpp
#include <stdio.h>
#include <string.h>
int main() {
char src[] = "He was an unusually\0 complex man";//这里的/0不被在意
char dist[1024] = { 0 }; memcpy(dist, src, 200);
printf("src:%s\ndist:%s\n", src, dist);
printf("dist[21]=%c\n",dist[21]);
return 0;
}
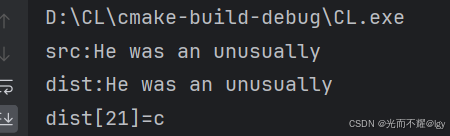
2.memmove
原型:void * memmove ( void * destination, const void * source, size_t num );
原理:将num个字节的值从源指向的位置复制到目标指向的内存块。复制就像使用了中间缓存,从而允许目标和源重叠, 该函数不检测源中的任何终止字符,它总是精确地复制num个字符。
section one :
destination的起始地址在src起始地址之后
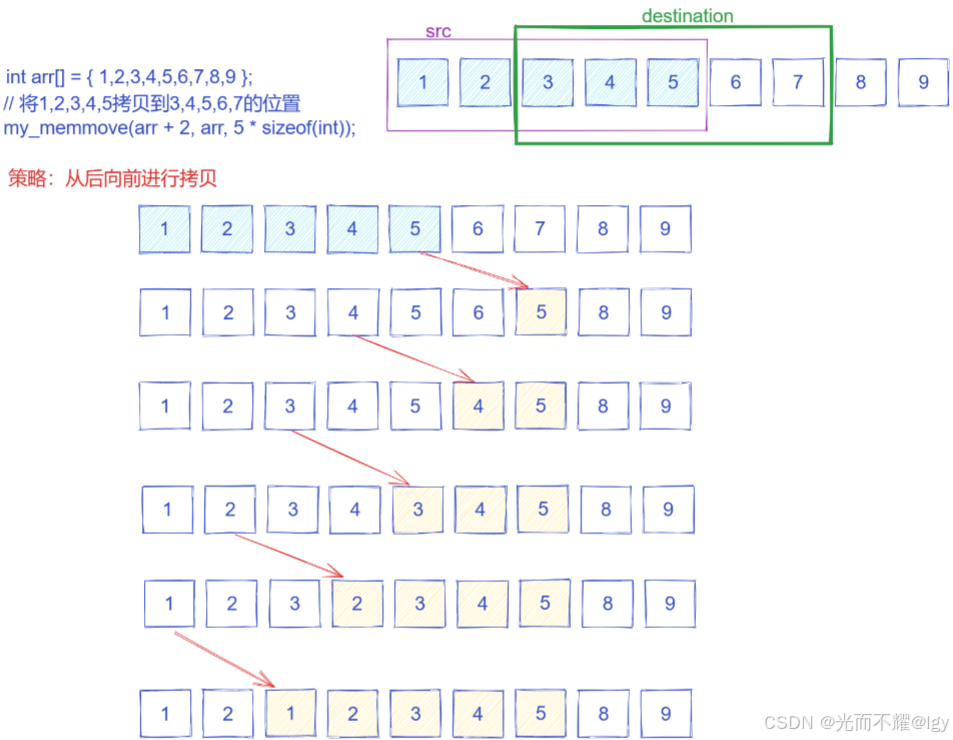
section two :
destination的结束地址在src结束地址之前
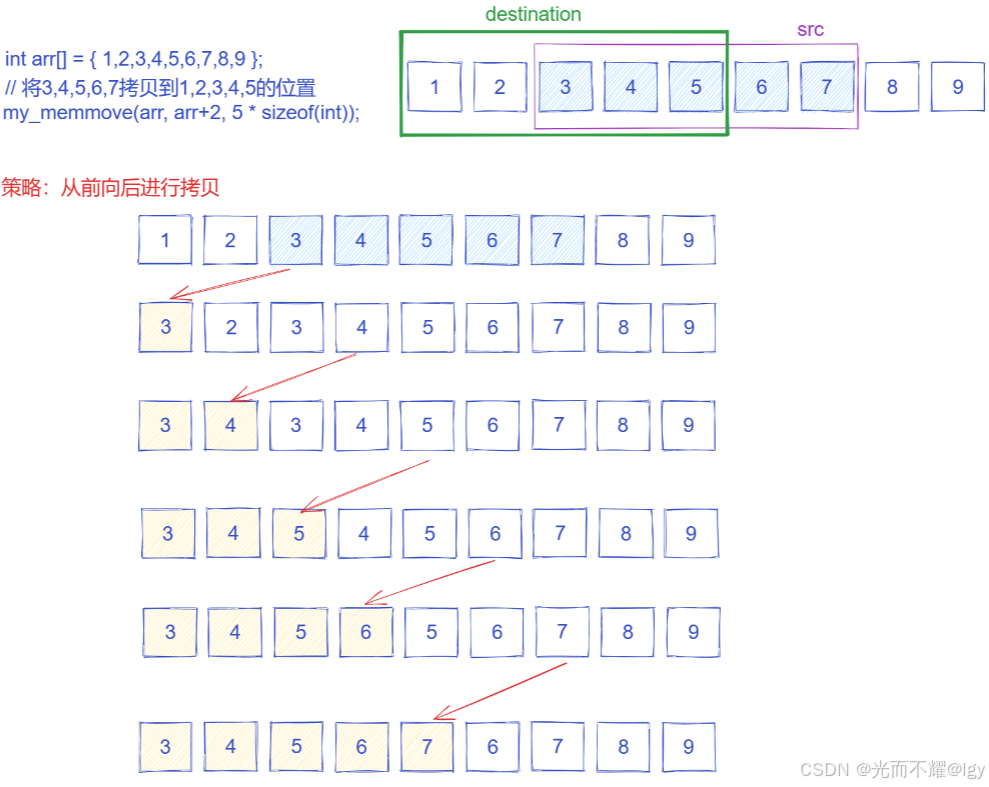
cpp
#include <stdio.h>
#include <string.h>
int main() {
char str[] = "abcdef";
// 重叠的区域复制:从 str + 1 开始的部分复制到 str 开头
memmove(str, str+1, 5);
printf("Result: %s\n", str); // 输出: "bcdef"
return 0;
}
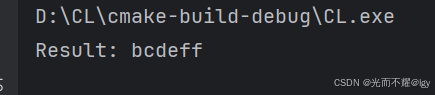
3.memcmp
原型:int memcmp ( const void * ptr1, const void * ptr2, size_t num );
原理:
- 将ptr1所指向的内存块的前num字节与ptr2所指向的前num字节进行比较,如果它们都匹配则返回0
- 在两个内存块中不匹配的第一个字节在ptr1中的值小于ptr2中的值,则返回<0的数(比较的是字母转换后的ASCII值)
- 在两个内存块中不匹配的第一个字节在ptr1中的值大于ptr2中的值,则返回>0的数
cpp
#include <stdio.h>
#include <string.h>
int main(){
char buffer1[] = "DWGaOtP12df0";
char buffer2[] = "DWgAOTP12DF0";
int n = memcmp(buffer1, buffer2, sizeof(buffer1));
if (n > 0) {
printf("'%s' is greater than '%s'.\n", buffer1, buffer2);
} else if (n < 0) {
printf("'%s' is less than '%s'.\n", buffer1, buffer2);
} else {
printf("'%s' is the same as '%s'.\n", buffer1, buffer2);
}
return 0;
}
4.memset
原型:void * memset ( void * ptr, int value, size_t num );
原理:将ptr指向的内存块的前num个字节设置为指定的值(解释为unsigned char)
cpp
#include <stdio.h>
#include <string.h>
int main() {
char name[] = "almost every programmer should know memset!";
// 将name指向的内存块的前6个字节设置为-
memset(name, '-', 6);
printf("%s\n", name);
return 0;
}
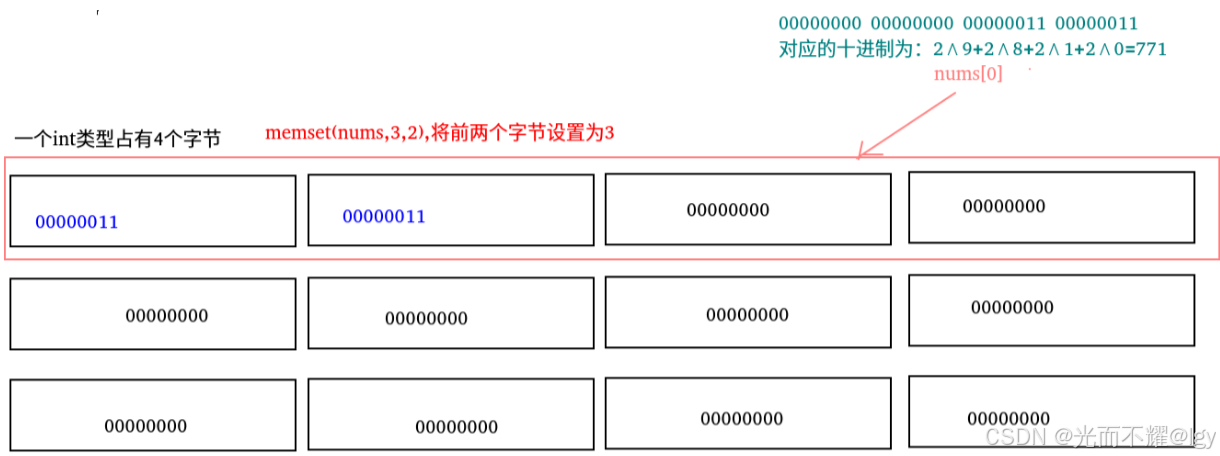
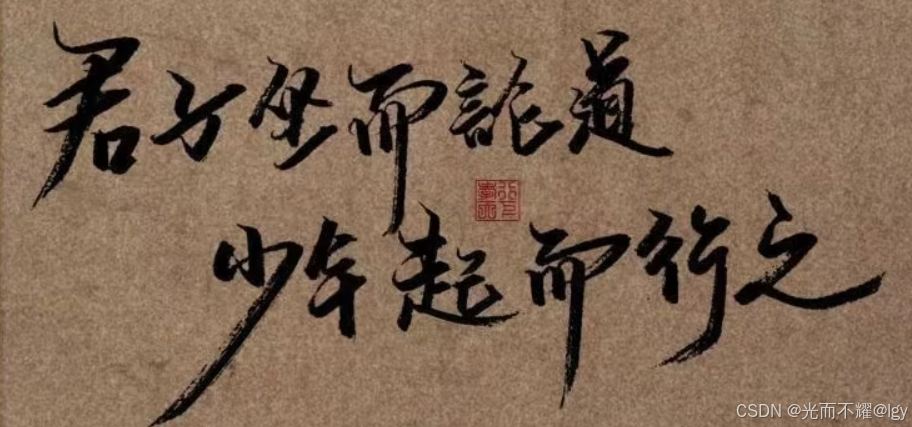
- 这是本人的学习笔记不是获利的工具,小作者会一直写下去,希望大家能多多监督我
- 文章会每攒够两篇进行更新发布(受平台原因,也是希望能让更多的人看见)
- 感谢各位的阅读希望我的文章会对诸君有所帮助