1、函数重载概述
作用:函数名可以相同,提高复用性。
函数重载满足条件:
(1)同一个作用域(我们现在写的函数都是全局函数,都在全局作用域中)
(2)函数名称相同
(3)函数参数类型不同或者参数个数不同或者顺序不同
注意:函数的返回值类型不可以作为函数重载的条件
示例:
参数个数不同
cpp
#include<iostream>
using namespace std;
void func()
{
cout << "func的调用" << endl;
}
void func(int a)
{
cout << "func(int a) 的调用" << endl;
}
int main()
{
func();
func(100);
system("pause");
return 0;
}
结果:
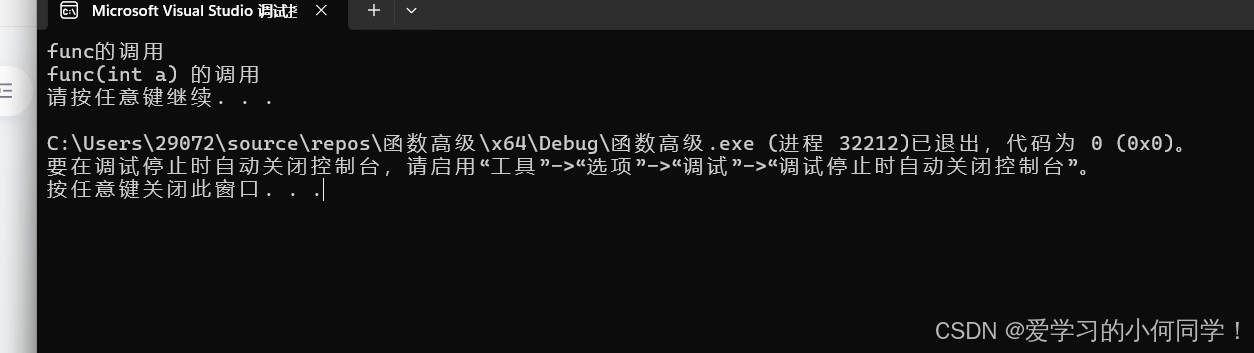
参数类型不同:
cpp
#include<iostream>
using namespace std;
void func(int a)
{
cout << "func(int a) 的调用" << endl;
}
void func(double a)
{
cout << "func(double a) 的调用" << endl;
}
int main()
{
func(10);
func(1.2);
system("pause");
return 0;
}
结果:
参数顺序不同:
cpp
#include<iostream>
using namespace std;
void func(int a, double b)
{
cout << "func(int a,double b)的调用" << endl;
}
void func(double a, int b)
{
cout << "func(double a,int b)的调用" << endl;
}
int main()
{
func(10, 2.1);
func(2.1, 10);
system("pause");
return 0;
}
结果:
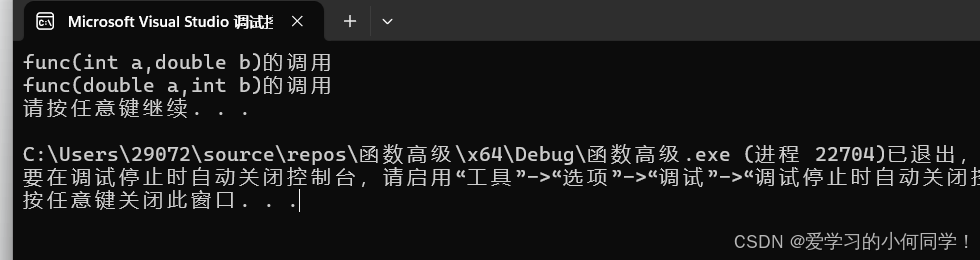
2、函数重载注意事项
(1)引用作为重载条件
示例:
cpp
#include<iostream>
using namespace std;
void func(int& a)
{
cout << "func(int &a)调用" << endl;
}
void func(const int& a)
{
cout << "func(const int & a)调用" << endl;
}
int main()
{
int a = 10;
func(a);//调用func(int &a),
func(10);//调用func(const int &a)
system("pause");
return 0;
}
结果:
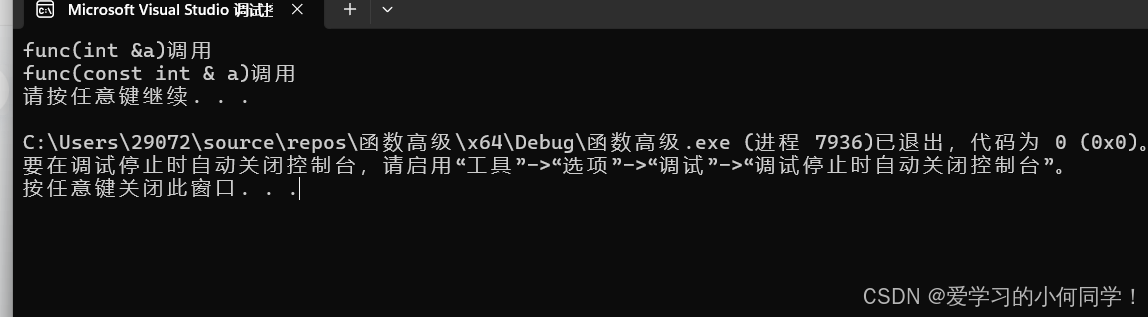
(2)函数重载碰到默认参数
示例:
cpp
#include<iostream>
using namespace std;
void func(int a, int b = 10)
{
cout << "func(int a,int b=10)的调用" << endl;
}
void func(int a)
{
cout << "func(int a)的调用" << endl;
}
int main()
{
func(10, 20);
system("pause");
return 0;
}
结果:
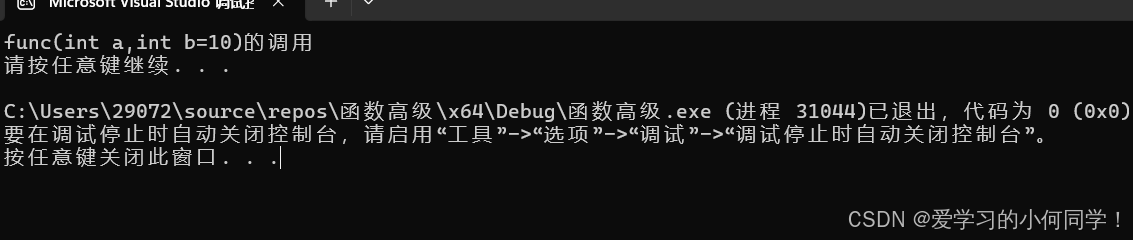