说明
wv(webview2) 是Go语言基于LCL和WebView2基础上封装的框架,用于开发Windows GUI软件。
介绍
LCL(Lazarus Component Library) :跨平台原生UI组件库.
wv(WebView2): Microsoft Edge WebView2 控件允许在本机应用中嵌入 web 技术(HTML、CSS 以及 JavaScript)。 WebView2 控件使用 Microsoft Edge 作为绘制引擎,以在本机应用中显示 web 内容
WebView2使用 [WebView4Delphi] 得益于封装(https://github.com/salvadordf/WebView4Delphi) ,并做为LCL中的一个控件使用.
限制
- 仅支持 Windows
- Windows 7 需要安装 WebView2 运行时环境
- Windows 10, 11 不需要安装运行时环境.
依赖
- Golang >= 1.20
- Webview2 运行时环境, Windows10/11一搬不需要安装
- liblcl.dll LCL控件库动态链接库, 绑定库.
环境准备
创建目录并初始化Golang为工作空间
mkdir demoworkspace
cd demoworkspace
go work init
获取&克隆 lcl 和 wv 仓库
- https://gitee.com/energye/lcl
- https://gitee.com/energye/wv
- 将lcl和wv添加到工作空间
go
go work use lcl
go work use wv
获取 WebView2 和 liblcl.dll
说明
- 创建任意目录并设置到 ENERGY_HOME 环境变量, 并将两个动态链接库放置到该环境变量目录
- 或把两个动态链接库放置到Go编译的二进制.exe同一目录
环境总结
- 将lcl和wv仓库克隆到本地的Golang工作空间目录
- 下载依赖的动态链接库 WebView2 和 liblcl.dll
- Golang >= 1.20
创建自己应用
在工作空间目录 demoworkspace
创建自己的Go项目做为GUI应用, 并添加到工作空间
go
mkdir demo
cd demo
go mod init demo
cd ../
go work use demo
示例代码
go
package main
import (
"fmt"
"github.com/energye/lcl/api/libname"
"github.com/energye/lcl/lcl"
"github.com/energye/lcl/tools/exec"
"github.com/energye/lcl/types"
"github.com/energye/wv/wv"
"path/filepath"
)
var (
mainForm TMainForm // 主窗口
load wv.IWVLoader // webview2 loader
)
func main() {
wv.Init(nil, nil)
load = wv.GlobalWebView2Loader()
liblcl := libname.LibName
webView2Loader, _ := filepath.Split(liblcl)
webView2Loader = filepath.Join(webView2Loader, "WebView2Loader.dll")
load.SetUserDataFolder(filepath.Join(exec.CurrentDir, "EnergyCache"))
load.SetLoaderDllPath(webView2Loader)
if load.StartWebView2() {
// 底层库全局异常
lcl.Application.SetOnException(func(sender lcl.IObject, e lcl.IException) {
fmt.Println("全局-底层库异常:", e.ToString())
})
lcl.Application.Initialize()
lcl.Application.SetMainFormOnTaskBar(true)
lcl.Application.CreateForm(&mainForm)
lcl.Application.Run()
}
}
type TMainForm struct {
lcl.TForm
windowParent wv.IWVWindowParent
browser wv.IWVBrowser
}
func (m *TMainForm) FormCreate(sender lcl.IObject) {
m.SetCaption("Go wv(Webview2) - demo") // 窗口标题
m.SetBounds(0, 0, 800, 600) // 窗口大小
m.WorkAreaCenter() // 屏幕居中
// webview2 window组件
m.windowParent = wv.NewWVWindowParent(m)
m.windowParent.SetParent(m)
m.windowParent.SetAlign(types.AlClient)
// webview2 browser组件
m.browser = wv.NewWVBrowser(m)
m.browser.SetDefaultURL("https://www.baidu.com") // 指定一个要加载的地址
m.browser.SetOnAfterCreated(func(sender lcl.IObject) {
// browser创建完成后刷新 window组件大小
m.windowParent.UpdateSize()
})
// 设置browser到window parent
m.windowParent.SetBrowser(m.browser)
// 窗口显示事件, 创建browser
m.SetOnShow(func(sender lcl.IObject) {
if load.InitializationError() {
} else {
if load.Initialized() {
m.browser.CreateBrowser(m.windowParent.Handle(), true)
}
}
})
}
Go WebView2效果
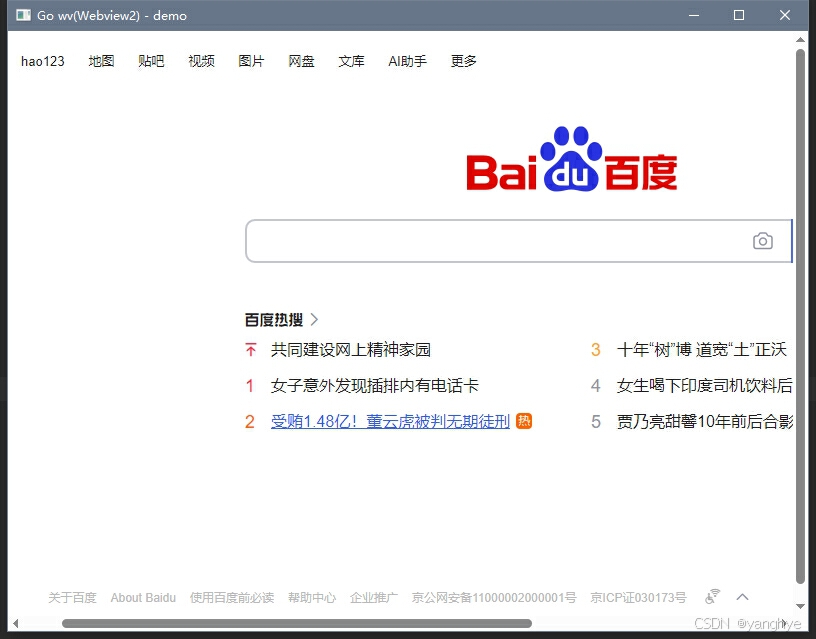
Go WebView2 架构
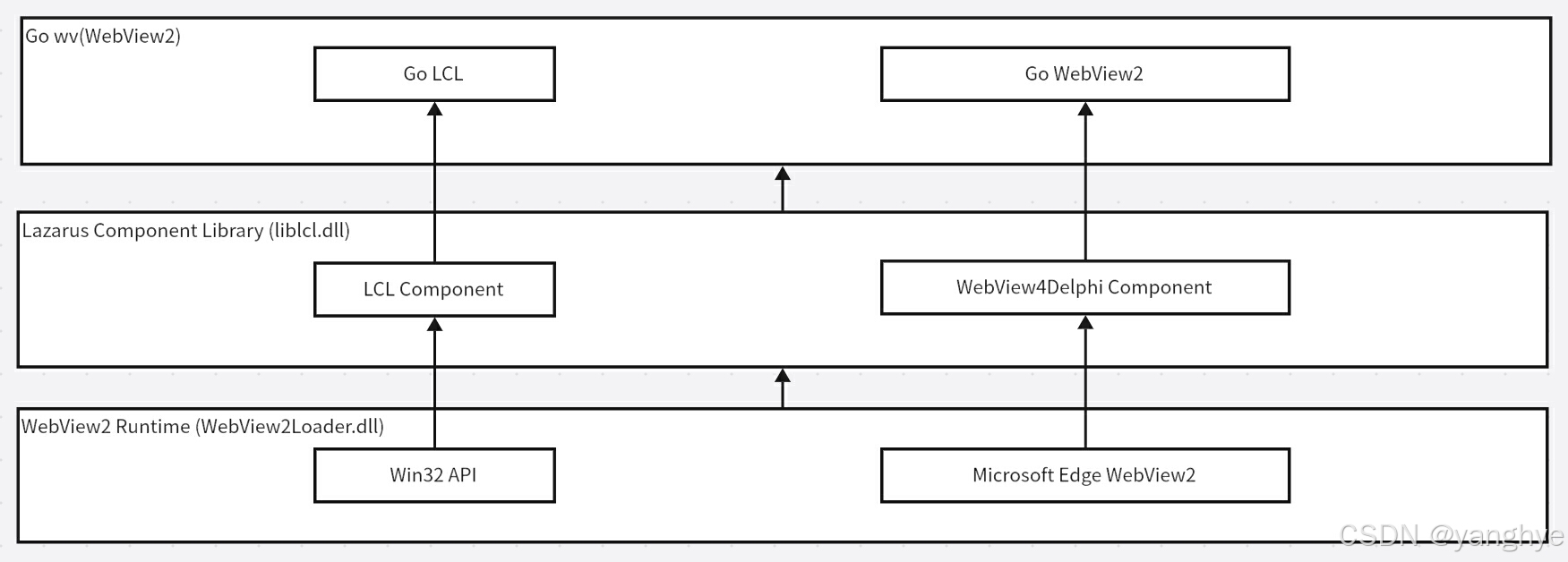