目录
[一、list 的简单介绍](#一、list 的简单介绍)
[二、list 的基本使用](#二、list 的基本使用)
[🎉list iterator 的使用](#🎉list iterator 的使用)
[🎉list capacity](#🎉list capacity)
[🎉list element access](#🎉list element access)
[🎉list modifiers](#🎉list modifiers)
[🎉list operator](#🎉list operator)
[三、list 的模拟实现](#三、list 的模拟实现)
[🎉 后置operator++(int) / operator--(int)](#🎉 后置operator++(int) / operator--(int))
[🌟List相关接口函数的模拟实现 :](#🌟List相关接口函数的模拟实现 :)
[🎉深拷贝List(const List& lt)](#🎉深拷贝List(const List& lt))
[🎉List& operator=()](#🎉List& operator=())
[四、list 和 vector 的对比](#四、list 和 vector 的对比)
一、list 的简单介绍
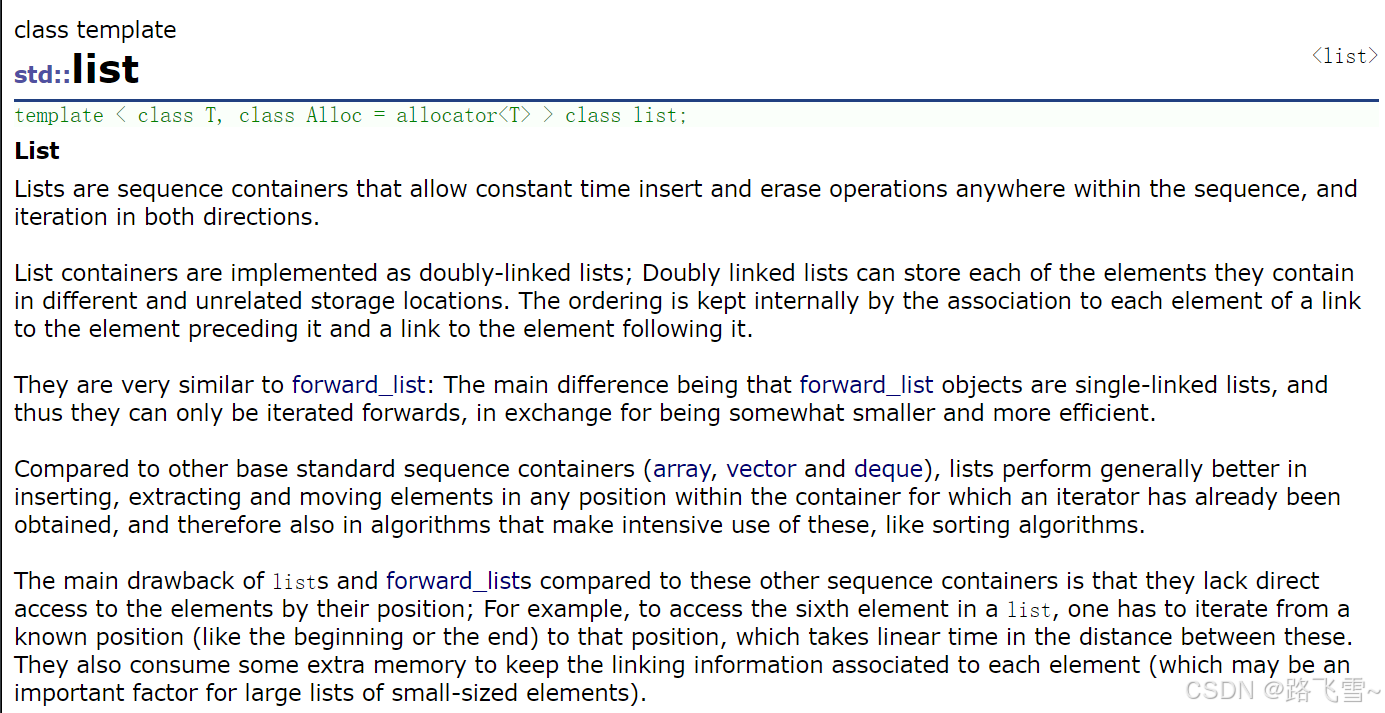
-
list是可以在常数范围内在任意位置进行插入和删除的序列式容器,并且该容器可以前后双向代。
-
list的底层是双向链表结构,双向链表中每个元素存储在互不相关的独立节点中,在节点中通过指针指向其前一个元素和后一个元素。
-
list与forward_list非常相似:最主要的不同在于forward_list是单链表,只能朝前迭代,已让其更简单高效。
-
与其他的序列式容器相比(array,vector,deque),list通常在任意位置进行插入、移除元素的执行效率更好。
-
与其他序列式容器相比,list和forward_list最大的缺陷是不支持任意位置的随机访问,比如:要访问list的第6个元素,必须从已知的位置(比如头部或者尾部)迭代到该位置,在这段位置上迭代需要线性的时间开销;list还需要一些额外的空间,以保存每个节点的相关联信息(对于存储类型较小元素的大list来说这可能是一个重要的因素)
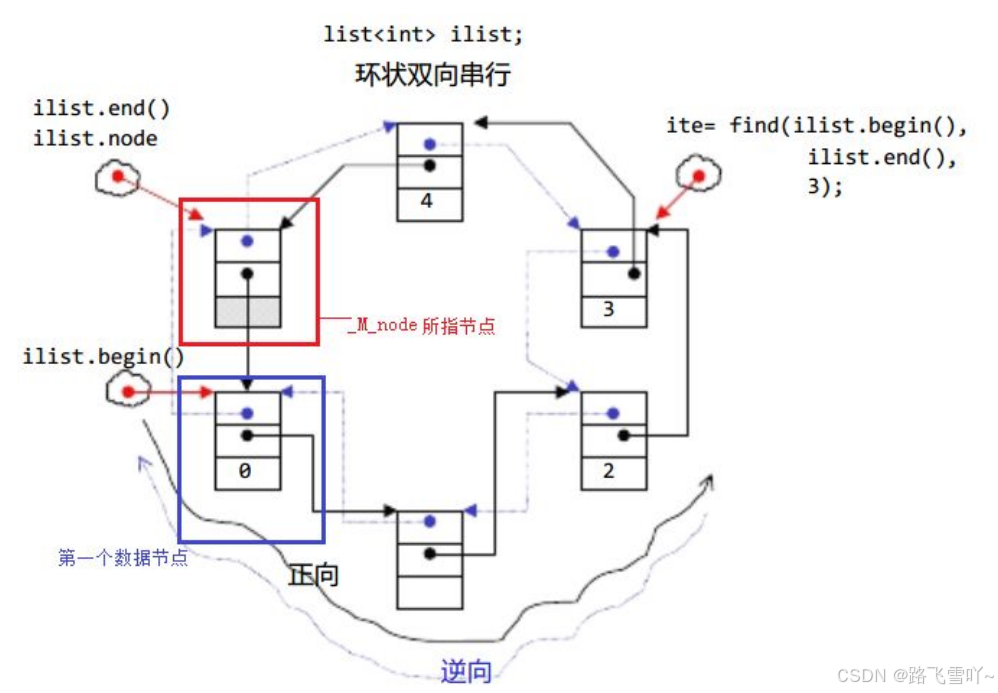
二、list 的基本使用
🎉list的构造
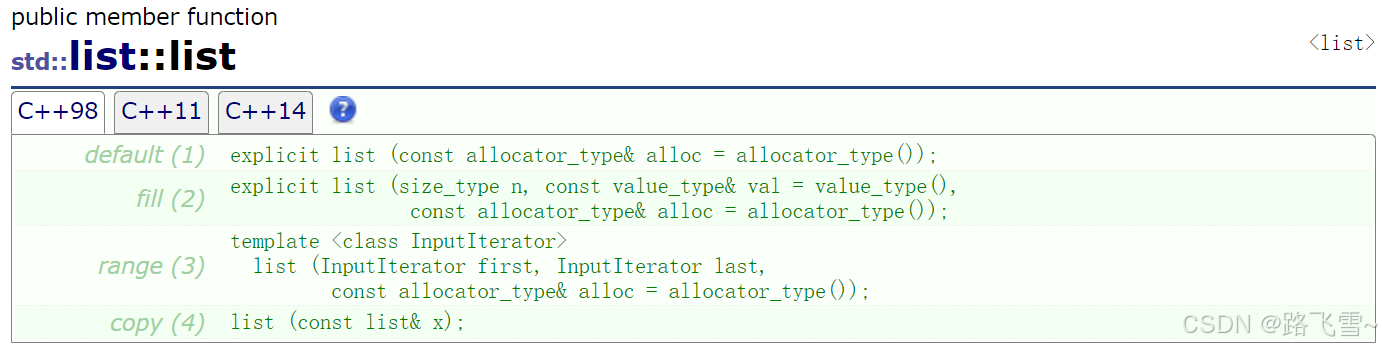
|---------------------------------------------------------|----------------------------------|
| 构造函数 | 接口说明 |
| list (size_type n, const value_type& val=value_type()) | 构造的list中包含n个值为val的元素 |
| list() | 构造空的list |
| list (const list& x) | 拷贝构造函数 |
| list ( InputIterator first, InputIterator last) | 用 [ first , last ) 区间中的元素构造list |
cpp
#include<iostream>
#include<list>
using namespace std;
int main()
{
list<int> l1; //构造空的l1
list<int> l2(4, 100); // 构造4个值为100的元素
list<int> l3(l2.begin(), l2.end()); //用l2的[begin(),end())左闭右开区间构造l3
list<int> l4(l3);
//以数组为迭代区间构造l5
int array[] = { 16,2,77,29 };
list<int> l5(array, array + sizeof(array) / sizeof(int));//array array+sizeof(4)
//列表格式初始化C++11
list<int> l6{ 1,2,3,4,5 };
//用迭代器方式打印l5中的元素
list<int>::iterator it = l5.begin();
while (it != l5.end())
{
cout << *it << " ";
++it;
}
cout << endl;
//C++范围for的方式遍历
for (auto& e : l5)
{
cout << e << " ";
}
cout << endl;
return 0;
}
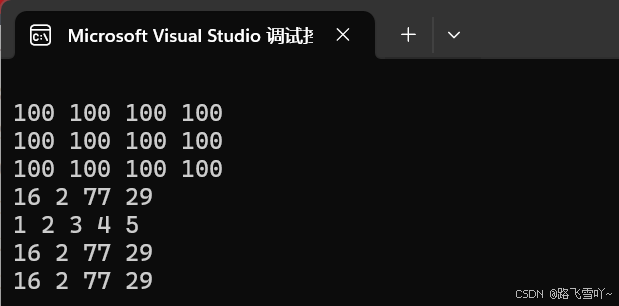
🎉list iterator 的使用


此处,可以暂时将迭代器理解成一个指针,该指针指向list中的某个结点。
|-------------------|----------------------------------------------------------------------------|
| 函数声明 | 接口说明 |
| begin() + end() | 返回第一个元素的迭代器+返回最后一个元素下一个位置的迭代器 |
| rbegin() + rend() | 返回第一个元素的 reverse_iterator, 即end位置,返回最后一个元素下一个位置的 reverse_iterator,即begin位置 |
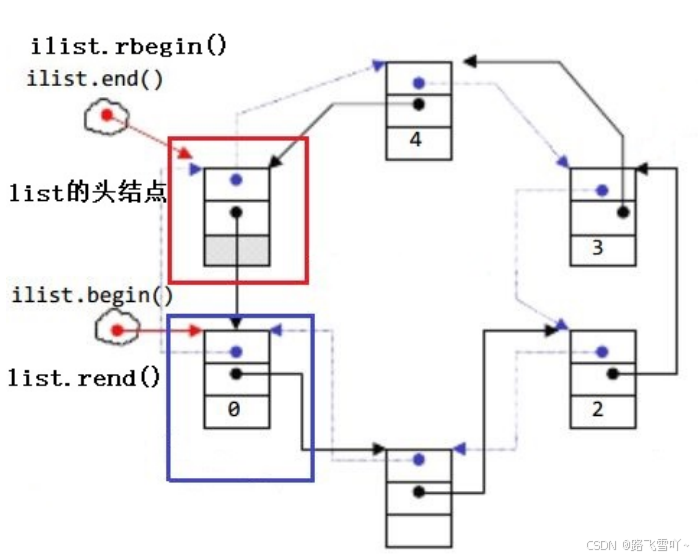
cpp
//list迭代器的使用
//注意:遍历链表只能用迭代器和范围for
void Printlist( const list<int>& l )
{
//注意这里调用的是list的 begin() const,返回list的 const_iterator对象
for (list<int>::const_iterator it = l.begin(); it != l.end(); ++it)
{
cout << *it << " ";
//*it = 10; 编译不通过
}
cout << endl;
}
cpp
#include<iostream>
#include<list>
using namespace std;
int main()
{
int array[] = { 1, 2, 3, 4, 5, 6, 7, 8, 9, 0 };
list<int> l(array, array + sizeof(array) / sizeof(array[0]));
//使用正向迭代器正向list中的元素
//list<int>::iterator it=l.begin(); //C++98中的语法
auto it = l.begin();//C++11之后的推荐写法
while (it != l.end())
{
cout << *it << " ";
++it;
}
cout << endl;
//使用反向迭代器逆向打印list中的元素
//list<int>::reverse_iterator rit = l.rbegin();
auto rit = l.rbegin();
while (rit != l.rend())
{
cout << *rit << " ";
++rit;
}
cout << endl;
return 0;
}
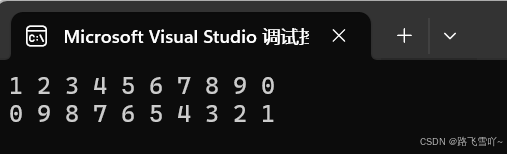
【注意】
1、begin与end为正向迭代器,对迭代器执行++操作,迭代器向后移动
2、rbegin(end) 与 rend(begin)为反向迭代器,对迭代器执行++操作,迭代器向前移动
🎉list capacity

|------------|------------------------------|
| 函数说明 | 接口说明 |
| empty() | 检测list是否为空,是返回ture,否则返回false |
| size() | 返回list中有效节点的个数 |
| max_size() | 返回列表容器可以容纳的最大元素数 |
cpp
#include<iostream>
#include<list>
using namespace std;
int main ()
{
std::list<int> mylist;
int sum (0);
for (int i=1;i<=10;++i) mylist.push_back(i);
while (!mylist.empty())
{
sum += mylist.front();
mylist.pop_front();
}
std::cout << "total: " << sum << '\n';
return 0;
}
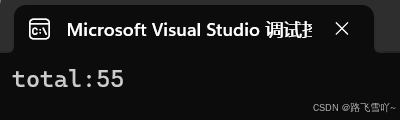
cpp
#include<iostream>
#include<list>
using namespace std;
int main()
{
std::list<int> myints;
std::cout << "0. size: " << myints.size() << '\n';
for (int i = 0; i < 10; i++)
{
myints.push_back(i);
}
std::cout << "1. size: " << myints.size() << '\n';
myints.insert(myints.begin(), 10, 100);
for (list<int>::const_iterator it = myints.begin(); it != myints.end(); ++it)
{
cout << *it << " ";
}
cout << endl;
std::cout << "2. size: " << myints.size() << '\n';
myints.pop_back();
std::cout << "3. size: " << myints.size() << '\n';
return 0;
}
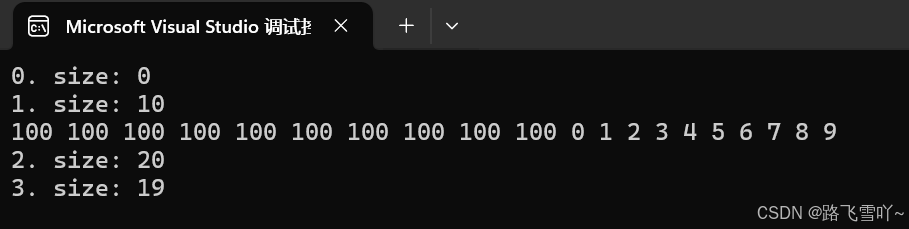
🎉list element access


|-------|------------------------|
| 函数声明 | 接口说明 |
| front | 返回list的第一个节点中值的引用 |
| back | 返回list的最后一个节点中值的引用 |
🎉list modifiers


|--------------|---------------------|
| 函数声明 | 接口说明 |
| push_front() | 在list首元素前插入值为val的元素 |
| pop_front() | 删除list中第一个元素 |
| push_back() | 在list的尾部插入值为val的元素 |
| pop_back() | 删除list中最后一个元素 |
cpp
#include<iostream>
#include<list>
using namespace std;
void Printlist(const list<int>& l)
{
//注意这里调用的是list的begin() const,返回list的 const_iterator对象
for (list<int>::const_iterator it = l.begin(); it != l.end(); ++it)
{
cout << *it << " ";
//*it = 10;编译不通过
}
cout << endl;
}
int main()
{
int array[] = { 1,2,3,4,5,6,7,8,9 };
list<int> L(array, array + sizeof(array) / sizeof(array[0]));
//尾插 头插
L.push_back(4);
L.push_front(0);
Printlist(L);
//尾删 头删
L.pop_back();
L.pop_front();
Printlist(L);
return 0;
}
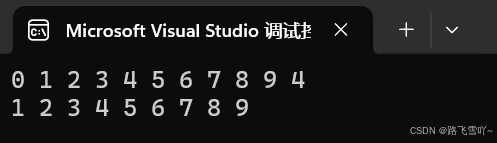


|--------|------------------------------|
| 函数声明 | 接口说明 |
| insert | 在list position 位置中插入值为val的元素 |
| erase | 删除list position 位置的元素 |
cpp
#include<iostream>
#include<list>
#include<vector>
using namespace std;
int main()
{
int array[] = { 1,2,3 };
list<int> L(array, array + sizeof(array) / sizeof(array[0]));
//获取链表中的第二个结点
//list<int>::iterator it = ++L.begin();
auto pos = ++L.begin();
cout << *pos << endl;
//在pos前插入值为4的元素
L.insert(pos, 4);
Printlist(L);
// 在pos前插入5个值为5的元素
L.insert(pos, 5, 5);
Printlist(L);
// 在pos前插入[v.begin(), v.end)区间中的元素
vector<int> v{ 7,8,9 };
L.insert(pos, v.begin(), v.end());
Printlist(L);
// 删除pos位置上的元素
L.erase(pos);
Printlist(L);
// 删除list中[begin, end)区间中的元素,即删除list中的所有元素
L.erase(L.begin(), L.end());
Printlist(L);
return 0;
}
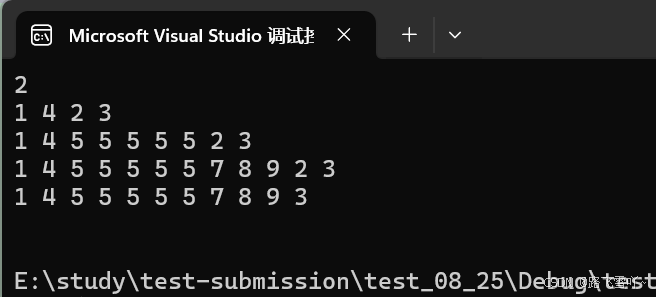

|--------|------------------|
| 函数声明 | 接口说明 |
| swap | 交换两个list中的元素 |
| resize | 调整容器的大小,使其包含n个元素 |
| clear | 清空list中的有效元素 |
cpp
#include<iostream>
#include<list>
using namespace std;
int main()
{
// 用数组来构造list
int array[] = { 1,2,3 };
list<int> L1(array, array + sizeof(array) / sizeof(array[0]));
Printlist(L1);
// 交换l1和l2中的元素
list<int> L2;
L1.swap(L2);
Printlist(L1);
Printlist(L2);
// 将l2中的元素清空
L2.clear();
cout << L2.size() << endl;
return 0;
}
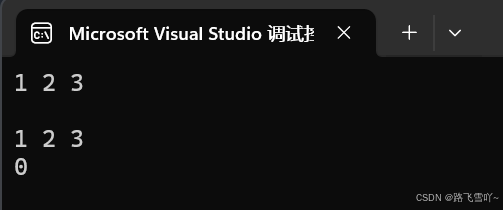
🎉list operator

(1)将元素从列表转移到列表
(2)将元素从x转移到容器中,并将其插入到指定位置
(3)有效地将这些元素插入容器中,并将其从x中删除,从而改变了两者的大小容器。该操作不涉及任何元素的构造或销毁,无论x是左值还是右值,或者值类型是否支持移动构造,它们都会被转移。
第一个版本(1)将x的所有元素转移到容器中。
第二个版本(2)只将i指向的元素从x转移到容器中
第三个版本(3)将范围[first,last)从x转移到容器中。

|--------|---------------------|
| 函数声明 | 接口函数 |
| splice | 将元素从列表转移到列表(剪切) |
| merge | 合并已排序列表 |
cpp
#include<iostream>
#include<list>
using namespace std;
int main()
{
std::list<int> mylist1, mylist2;
std::list<int>::iterator it;
for (int i = 1; i <= 4; ++i)
mylist1.push_back(i); //mylist1: 1 2 3 4
for (int i = 1; i <= 3; ++i)
mylist2.push_back(i * 10); //mylist2: 10 20 30
it = mylist1.begin();
++it; //position to 2
mylist1.splice(it, mylist2); //mylist1: 1 10 20 30 2 3 4
// mylist2(empty)
return 0;
}
三、list 的模拟实现
🌟模拟实现list
list的底层是双向链表结构,双向链表中每个元素存储在互不相关的独立节点中,在节点中通过指针指向其前一个元素和后一个元素,因此模拟实现时,要对双链表的节点进行封装,list对元素进行访问时,不能用普通的迭代器进行访问下一个元素,因为双链表在物理上是不连续的,因此又要对双链表的迭代器进行封装。
这里我们对list的模拟实现放在一个头文件进行实现,我们要写在一个命名空间里面,为了避免命名冲突或名字污染,对(带头双向循环链表)双链表的节点进行封装:
cpp
// List.h
namespace xlf
{
//双链表的定义
//类模板
template<class T>
struct ListNode
{
// ListNode<T>*是一个类型 例:int*
ListNode<T>* _prev; //指向前一个节点的指针
ListNode<T>* _next;//指向后一个节点的指针
T _data;//节点的数据
//构造
ListNode(const T& data)
:_prev(nullptr)
,_next(nullptr)
,_data(data)
{}
};
}
• 为什么用 struct 而不用 class 来创建类呢?
struct 不受访问限定符的限制,class 使用受限,如果使用class,则要把成员函数和成员变量全部设为公有,这里的ListNode节点要经常使用。
template 定义的模板参数,只能供当前类或当前函数使用
对List进行封装:
cpp
template<class T>
class List
{
typedef ListNode<T> Node; //为类型取别名
public:
//节点初始化
//双链表有哨兵位
List()
{
_head = new Node(T());//用匿名对象进行初始化
_head->_prev = _head;
_head->_next = _head;
}
//尾插
void push_back(const T& x)
{
//创建新节点
Node* newnode = new Node(x);
//找尾节点
Node* tail = _head->_prev;
//连接
newnode->_prev = tail;//1
tail->_next = newnode;//2
newnode->_next = _head;//3
_head->_prev = newnode;//4
}
private:
Node* _head;
};
哨兵位如何初始化?
此时,当我们对链表的数据进行访问/修改时,我们不能用普通的迭代器进行访问,链表在物理空间上是不连续的,因此我们需要对迭代器进行封装:
cpp
//链表迭代器
template<class T>
struct ListIterator
{
typedef ListNode<T> Node;//给节点取别名
typedef ListIterator<T> Self;//給迭代器取别名
Node* _node;//节点
public:
//构造函数
ListIterator(Node* node)
:_node(node)
{}
//对自定义进行运算符的重载,可控制迭代器的行为
};
现在我们来实现一般所需的重载运算符:
• 对自定义类型运算符的重载,可控制迭代器的行为。
🎉前置operator++/operator--
cpp
Self& operator++()//出了当前作用域不销毁,用传引用返回
{
_node = _node->_next;
return *this;
}
Self& operator--()//出了当前作用域不销毁,用传引用返回
{
_node = _node->_prev;
return *this;
}
• 传引用返回 ,是因为出了当前作用域,*this这个值不销毁
• _node=_node->_next 表示这个节点的下一个节点
• _node=_node->_prev 表示这个节点的前一个节点
🎉 后置operator++(int) / operator--(int)
cpp
Self operator++(int)//出了作用域这个值会销毁,用传值返回
{
Node* temp(*this); // d++ :返回的是++之前的值
// 所以要先保留++之前的值
_node = _node->_next;
return temp;
}
Self operator--(int)//出了作用域这个值会销毁,用传值返回
{
Node* temp(*this); // d-- :返回的是--之前的值
// 所以要先保留--之前的值
_node = _node->_prev;
return temp;
}
• 前置++和后置++的区别:(返回值不一样)
++d :返回++之后的值
d++:返回++之前的值
• Node* temp(*this) 表示先保留++/-- 之前的值,当做返回值
•用传值返回,是因为 temp 出了当前作用域后,就会被销毁
🎉operator!=/operator==
cpp
bool operator!=(const Self& it)
{
return _node != it._node;
}
bool operator==(const Self& it)
{
return _node == it._node;
}
🎉operator*()
cpp
T& operator*()
{
return _node->_data;
}
为什么重载 operator*() 呢?
• 重载operator*(),是为了可以获取到节点里面的数据。
测试例子:
cpp
List<int>::iterator it = L.begin();
while (it != L.end())
{
cout << *it << " ";
//*it 解引用要的是数值,不是节点,所以运算符重载了operator*()
++it;
}
cout << endl;
🎉operator->()
cpp
const T* operator->()
{
return &_node->_data;
}
为什么重载operator->()呢?
看这个例子:
cpp
struct Pos
{
int _row;
int _col;
Pos( int row = 0,int col = 0)
:_row(row)
,_col(col)
{}
};
void test_list3()
{
List<Pos> L;
L.push_back(Pos(100, 100));
L.push_back(Pos(200, 200));
L.push_back(Pos(300, 300));
List<Pos>::iterator it = L.begin();
while (it !=L. end())
{
cout << it->_row << ":" << it->_col << endl;
//cout << it.operator->()->_row << ":" << it.operator->()->_col << endl;
++it;
}
cout << endl;
}
• 我们访问 int类型,可以用int*,如果我们访问 Pos* 这个自定义类型数据该如何访问?重载运算符,在结构体、类(公有),想访问成员,模拟这个行为,可以用 -> 来进行访问。
• cout << it->_row << ":" << it->_col << endl 实际为:
cout << it.operator->()->_row << ":" << it.operator->()->_col << endl;
第一个->:运算符重载的调用;
第二个->:原生指针
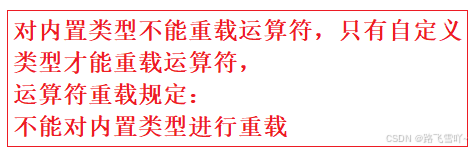
🎉迭代器iterator
此时就可以在List类里面实现部分迭代器:
cpp
template<class T>
class List
{
typedef ListNode<T> Node; //为类型取别名
public:
typedef ListIterator<T> iterator;
iterator begin()
{
iterator it(_head->_next);
return it;
}
iterator end()
{
iterator it(_head);
return it;
}
}
测试迭代器:
cpp
void test_list1()
{
List<int> L;
//实例化就报错
L.push_back(1);
L.push_back(2);
L.push_back(3);
L.push_back(4);
List<int>::iterator it = L.begin();
while (it != L.end())
{
*it += 10; // *it 解引用,返回的是这个节点的数据
// 此时可以修改这个节点数据的内容
cout << *it << " ";
//*it 解引用要的是数值,不是节点,所以运算符重载了operator*()
++it;
}
cout << endl;
for (auto& e : L)
{
cout << e << " ";
}
cout << endl;
}
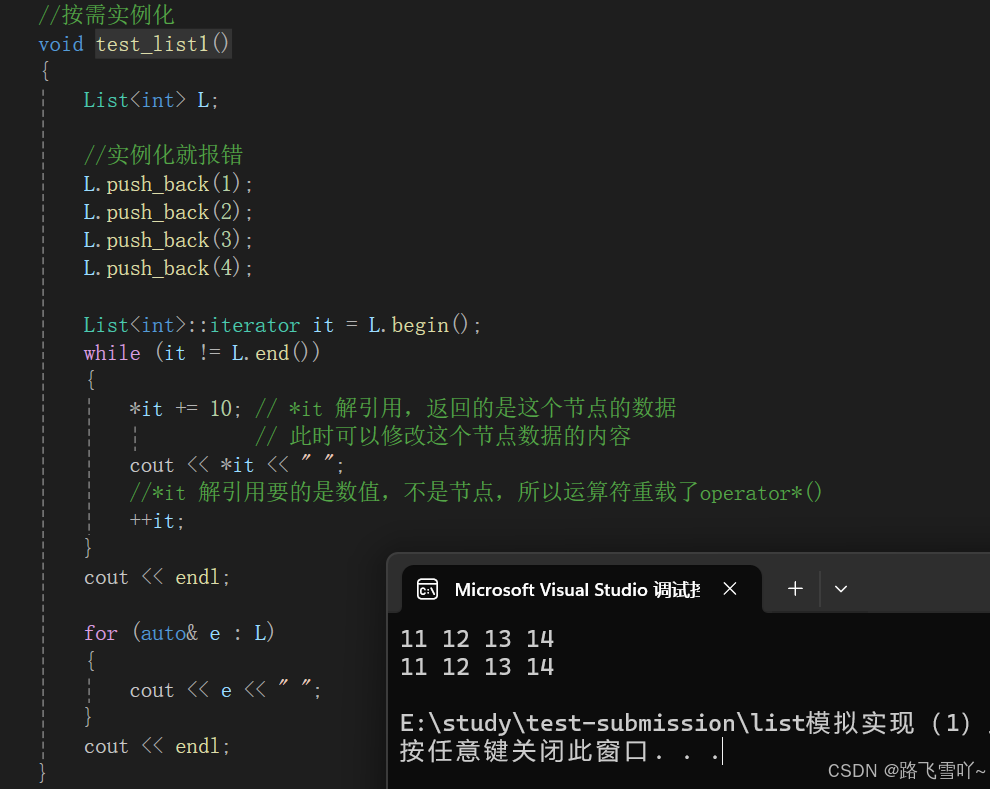
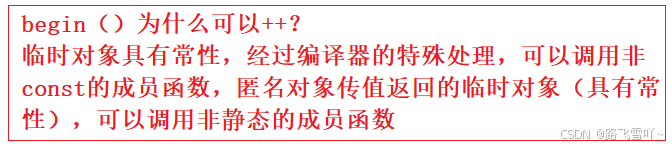
🎉迭代器const_iterator
如何做到自己能修改,指向的内容不能修改?
迭代器只能控制,不能修改的核心行为是 operator*()、operator->(),就是解引用时,要修改的东西。
cpp
// const_iterator
//链表迭代器
template<class T>
struct ListConstIterator
{
typedef ListNode<T> Node;//给节点取别名
typedef ListConstIterator<T> Self;//給迭代器取别名
Node* _node;//节点
public:
//构造函数
ListConstIterator(Node* node)
:_node(node)
{}
//对自定义进行运算符的重载,可控制迭代器的行为
Self& operator++()
{
_node = _node->_next;
return *this;
}
Self& operator--()
{
_node = _node->_prev;
return *this;
}
Self operator++(int)
{
Node* temp(*this);
_node = _node->_next;
return temp;
}
Self operator--(int)
{
Node* temp(*this);
_node = _node->_prev;
return temp;
}
bool operator!=(const Self& it)
{
return _node != it._node;
}
bool operator==(const Self& it)
{
return _node == it._node;
}
const T& operator*()
{
return _node->_data;
}
const T* operator->()
{
return &_node->_data;
}
};
• 实现与iterator()相似,区别就在于operator*() 和 operator->() 这两个运算符重载改为const,
即: const T& operator*(); const T* operator->()
• const iterator const 迭代器不能是普通迭代器前面加 const修饰
const迭代器目的:本身可以修改,指向的内容不能修改
例: const T* p ; p本身可以修改,*p不可以修改
代码测试:
cpp
void Func(const List<int>& lt)
{
List<int>::const_iterator it = lt.begin();
while (it!=lt.end())
{
//*it += 10; //const迭代器 指向的内容不能改变
cout << *it << " ";//可读
++it;//可遍历
}
cout << endl;
}
迭代器的另一种写法:
通过模板,给不同模板参数,让编译器帮我们写两个类(实例化)。
cpp
//迭代器的第二种写法:
//template 定义的模板参数,只能供当前类或当前函数使用
//链表迭代器
template<class T, class Ref, class Ptr>
struct ListIterator
{
typedef ListNode<T> Node;//给节点取别名
typedef ListIterator<T, Ref, Ptr> Self;//給迭代器取别名
Node* _node;//节点
public:
//构造函数
ListIterator(Node* node)
:_node(node)
{}
//对自定义进行运算符的重载,可控制迭代器的行为
Self& operator++()
{
_node = _node->_next;
return *this;
}
Self& operator--()
{
_node = _node->_prev;
return *this;
}
Self operator++(int)
{
Node* temp(*this);
_node = _node->_next;
return temp;
}
Self operator--(int)
{
Node* temp(*this);
_node = _node->_prev;
return temp;
}
bool operator!=(const Self& it)
{
return _node != it._node;
}
bool operator==(const Self& it)
{
return _node == it._node;
}
Ref operator*()
{
return _node->_data;
}
Ptr operator->()
{
return &_node->_data;
}
};
• template <class T , class Ref , class Ptr> 通过模板给不同模板参数;
• typedef ListIterator<T, Ref, Ptr> Self; typedef时也要做相应的修改,此时表示当传入的 Ref 和 Ptr 为常量时, Ref 和 Ptr就会替代为常量;当传入的 Ref 和 Ptr 为非常量时, Ref 和 Ptr就会替代为非常量;
• 改变 operator*() 的返回值类型从 T& 修改为 Ref ,
改变 operator->() 的返回值类型从 T* 修改为 Ptr ,
这时返回值的类型看传给 Ref / Ptr 是什么 ;
•此时 List类 中修改
typedef ListIterator<T> iterator ---> typedef ListIterator<T , T&, T*> iterator;
typedef ListConstIterator<T> const_iterator ---> typedef ListIterator<T, const T&, const T*> const_iterator;
🌟List相关接口函数的模拟实现 :
🎉insert()
cpp
//插入(在pos之前插入)
iterator insert(iterator pos, const T& x)
{
//找到Pos位置的节点
Node* cur = pos._node;
//开一个新节点
Node* newnode = new Node(x);
//找pos的前一个节点
Node* prev = cur->_prev;
//连接 prev newnode cur
prev->_next = newnode;
newnode->_prev = prev;
newnode->_next = cur;
cur->_prev = newnode;
return iterator(newnode);
}
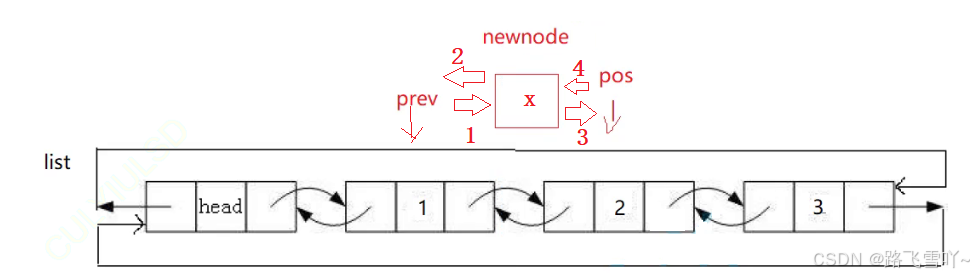
🎉erase()
cpp
iterator erase(iterator pos)
{
//防止删掉哨兵位
// pos end() 都是迭代器,_head是指针,所以不用
assert(pos != end());
//找到pos
Node* cur = pos._node;
//pos的前一个节点
Node* prev = cur->_prev;
//pos的后一个节点
Node* next = cur->_next;
//连接 prev next
prev->_next = next;
next->_prev = prev;
//删除节点
delete cur;
return iterator(next);
}
🌟迭代器失效问题
大家可将迭代器展示理解成类似于指针,迭代器失效及迭代器所指向的节点无效,即该节点被删除了。因为list的底层结构为带头结点的双向循环链表,因此在李斯特中进行插入时是不会导致list的迭代器失效的,只有删除时才会失效,并且时效的只是指向被删除节点的迭代器,其他迭代器不会受到影响。
cpp
void test_list()
{
int array[] = { 1,2,3,4,5,6,7,8,9 };
List<int> L(array, array + sizeof(array) / sizeof(array[0]));
auto it = L.begin();
while (it != L.end())
{
//erase()函数执行后,it所指向的节点已被删除,
// 因此it无效,在下一次使用it时,必须先给其重新赋值
it = L.erase(it);
++it;
}
}
🎉尾删/头删/头插
cpp
//尾删
void pop_back()
{
erase(--end());
}
//头删
void pop_front()
{
erase(begin());
}
//头插
void push_front(const T& x)
{
insert(begin(), x);
}
直接复用前面模拟实现过的函数。
🎉List()
cpp
//节点初始化
//双链表有哨兵位
List()
{
_head = new Node(T());//创建一个T()类型的节点
_head->_prev = _head;//节点前后都指向自己
_head->_next = _head;
}
因为下面的几个函数实现都需要初始化,所以把初始化写成一个函数。
cpp
//哨兵位头节点
void empty_init()
{
_head = new Node(T());
_head->_next = _head;
_head->_prev = _head;
}
//节点初始化
//双链表有哨兵位
List()
{
//_head = new Node(T());//创建一个T()类型的节点
//_head->_prev = _head;//节点前后都指向自己
//_head->_next = _head;
empty_init();
}
🎉深拷贝List(const List<T>& lt)
当不实现深拷贝时,系统会自动调用默认构造,此时就是浅拷贝,会出现一些问题:
此时,L1的更新会影响L2的数据,底层实际是L1和L2指向同一块空间,因此需要手动写拷贝构造。
cpp
//深拷贝
//lt2(lt1)
List(const List<T>& lt)
{
//先把原来list里面的数据初始化
empty_init();
//把lt1里面的数据一个一个尾插到lt2
for (const auto& e : lt)
{
push_back(e);
}
}
此时,L1数据的更新不会影响L2:
🎉~List()
在写析构时,我们先要把list里面的数据全部清除,然后再删除头节点的指针并置空。
cpp
~List()
{
clear();
delete _head;
_head = nullptr;
}
void clear()
{
auto it = begin();
while (it != end())
{
it = erase(it);
}
}
🎉List<T>& operator=()
cpp
//传值传参
//lt1=lt3
// 交换两个list的数据后,这两个list还在,所以用传引用返回
List<T>& operator=(List<T> lt)//lt3出了当前作用域不销毁,所以用传引用返回
{
swap(_head, lt._head);//直接用库里面的函数
return * this;
}
🎉初始化initializer_list
cpp
List(initializer_list<T> il)
{
empty_init();
for (const auto& e : il)
{
push_back(e);
}
}
四、list 和 vector 的对比
vector与list都是STL中非常重要的序列式容器,由于两个容器的底层结构不同,导致其特性以及应用场景不同,其主要不同如下:
|-------|--------------------------------------------------------------------|-------------------------------------------|
| | vector | list |
| 底层结构 | 动态顺序表,一段连续空间 | 带头结点的双向循环链表 |
| 随机访问 | 支持随机访问,访问某个元素效率O(1) | 不支持随机访问,访问某个元素效率O(N) |
| 插入和删除 | 任意位置插入和删除效率低,需要搬移元素,时间复杂度为O(N),插入时有空能增容,增容:开辟新空间,拷贝元素,释放旧空间,导致效率更低 | 任意位置插入和删除效率高,不需要搬移元素,时间复杂度为O(1) |
| 空间利用率 | 底层为连续空间,不容易造成内存碎片,空间利用率高,缓存利用率高 | 底层节点动态开辟,小节点容易造成内存碎片,空间利用率低,缓存利用率低 |
| 迭代器 | 原生态指针 | 对原生态指针(节点指针)进行封装 |
| 迭代器失效 | 在插入元素时,要给所有的迭代器进行赋值,因为插入元素可能会导致重新扩容,致使原来迭代器失效,删除时,当前迭代器需要重新赋值否则会失效 | 插入元素不会导致迭代器失效,删除元素时,只会导致当前迭代器失效,其他迭代器不受影响 |
| 使用场景 | 需要高效存储,支持随机访问,不关心插入删除效率 | 大量插入和删除操作,不关心随机访问 |
五、list模拟实现的完整代码
cpp
#pragma once
#include<iostream>
#include<assert.h>
using namespace std;
namespace xlf
{
//双链表的定义
//类模板
template<class T>
struct ListNode
{
// ListNode<T>*是一个类型 例:int*
ListNode<T>* _prev;
ListNode<T>* _next;
T _data;
//构造
ListNode(const T& data)
:_prev(nullptr)
,_next(nullptr)
,_data(data)
{}
};
//迭代器的第一种写法:
链表迭代器
//template<class T>
//struct ListIterator
//{
// typedef ListNode<T> Node;//给节点取别名
// typedef ListIterator<T> Self;//給迭代器取别名
// Node* _node;//节点
//public:
// //构造函数
// ListIterator(Node* node)
// :_node(node)
// {}
// //对自定义进行运算符的重载,可控制迭代器的行为
// Self& operator++()
// {
// _node = _node->_next;
// return *this;
// }
// Self& operator--()
// {
// _node = _node->_prev;
// return *this;
// }
// Self operator++(int)
// {
// Node* temp(*this);
// _node = _node->_next;
// return temp;
// }
// Self operator--(int)
// {
// Node* temp(*this);
// _node = _node->_prev;
// return temp;
// }
// bool operator!=(const Self& it)
// {
// return _node != it._node;
// }
// bool operator==(const Self& it)
// {
// return _node == it._node;
// }
// T& operator*()
// {
// return _node->_data;
// }
// T* operator->()
// {
// return &_node->_data;
// }
//};
const_iterator
链表迭代器
//template<class T>
//struct ListConstIterator
//{
// typedef ListNode<T> Node;//给节点取别名
// typedef ListConstIterator<T> Self;//給迭代器取别名
// Node* _node;//节点
//public:
// //构造函数
// ListConstIterator(Node* node)
// :_node(node)
// {}
// //对自定义进行运算符的重载,可控制迭代器的行为
// Self& operator++()
// {
// _node = _node->_next;
// return *this;
// }
// Self& operator--()
// {
// _node = _node->_prev;
// return *this;
// }
// Self operator++(int)
// {
// Node* temp(*this);
// _node = _node->_next;
// return temp;
// }
// Self operator--(int)
// {
// Node* temp(*this);
// _node = _node->_prev;
// return temp;
// }
// bool operator!=(const Self& it)
// {
// return _node != it._node;
// }
// bool operator==(const Self& it)
// {
// return _node == it._node;
// }
// const T& operator*()
// {
// return _node->_data;
// }
// const T* operator->()
// {
// return &_node->_data;
// }
//};
//迭代器的第二种写法:
//template 定义的模板参数,只能供当前类或当前函数使用
//链表迭代器
template<class T, class Ref, class Ptr>
struct ListIterator
{
typedef ListNode<T> Node;//给节点取别名
typedef ListIterator<T, Ref, Ptr> Self;//給迭代器取别名
Node* _node;//节点
public:
//构造函数
ListIterator(Node* node)
:_node(node)
{}
//对自定义进行运算符的重载,可控制迭代器的行为
Self& operator++()
{
_node = _node->_next;
return *this;
}
Self& operator--()
{
_node = _node->_prev;
return *this;
}
Self operator++(int)
{
Node* temp(*this);
_node = _node->_next;
return temp;
}
Self operator--(int)
{
Node* temp(*this);
_node = _node->_prev;
return temp;
}
bool operator!=(const Self& it)
{
return _node != it._node;
}
bool operator==(const Self& it)
{
return _node == it._node;
}
Ref operator*()
{
return _node->_data;
}
Ptr operator->()
{
return &_node->_data;
}
};
//链表实现
template<class T>
class List
{
typedef ListNode<T> Node; //为类型取别名
public:
迭代器的第一种写法:
//typedef ListIterator<T> iterator;
//typedef ListConstIterator<T> const_iterator;
//迭代器的第二种写法:
typedef ListIterator<T, T&, T*> iterator;
typedef ListIterator<T, const T&, const T*> const_iterator;
iterator begin()
{
/*iterator it(_head->_next);
return it;*/
return iterator(_head->_next);//匿名对象
}
iterator end()
{
/*iterator it(_head->_prev);
return it;*/
return iterator(_head);//匿名对象
}
const_iterator begin() const
{
/*const_iterator it(_head->_next);
return it;*/
return const_iterator(_head->_next);//匿名对象
}
const_iterator end() const
{
/*const_iterator it(_head);
return it;*/
return const_iterator(_head);
}
//尾插
void push_back(const T& x)
{
//创建新节点
Node* newnode = new Node(x);
//找尾节点
Node* tail = _head->_prev;
//连接 tail newnode _head
tail->_next = newnode;
newnode->_prev = tail;
newnode->_next = _head;
_head->_prev = newnode;
//insert(end(), x);
}
//插入(在pos之前插入)
iterator insert(iterator pos, const T& x)
{
//找到Pos位置的节点
Node* cur = pos._node;
//开一个新节点
Node* newnode = new Node(x);
//找pos的前一个节点
Node* prev = cur->_prev;
//连接 prev newnode cur
prev->_next = newnode;
newnode->_prev = prev;
newnode->_next = cur;
cur->_prev = newnode;
return iterator(newnode);
}
iterator erase(iterator pos)
{
//防止删掉哨兵位
// pos end() 都是迭代器,_head是指针,所以不用
assert(pos != end());
//找到pos
Node* cur = pos._node;
//pos的前一个节点
Node* prev = cur->_prev;
//pos的后一个节点
Node* next = cur->_next;
//连接 prev next
prev->_next = next;
next->_prev = prev;
//删除节点
delete cur;
return iterator(next);
}
//尾删
void pop_back()
{
erase(--end());
}
//头删
void pop_front()
{
erase(begin());
}
//头插
void push_front(const T& x)
{
insert(begin(), x);
}
//哨兵位头节点
void empty_init()
{
_head = new Node(T());
_head->_prev = _head;
_head->_next = _head;
}
//节点初始化
//双链表有哨兵位
List()
{
//_head = new Node(T());//创建一个T()类型的节点
//_head->_prev = _head;//节点前后都指向自己
//_head->_next = _head;
empty_init();
}
//深拷贝
//lt2(lt1)
List(const List<T>& lt)
{
//先把原来list里面的数据初始化
empty_init();
//把lt1里面的数据一个一个尾插到lt2
for (const auto& e : lt)
{
push_back(e);
}
}
~List()
{
clear();//清空list里面的数据
delete _head;//释放头节点
_head = nullptr;//头节点指针置为空,防止出现野指针
}
void clear()
{
auto it = begin();
while (it != end())
{
it = erase(it);
}
}
//传值传参
//lt1=lt3
// 交换两个list的数据后,这两个list还在,所以用传引用返回
List<T>& operator=(List<T> lt)//lt3出了当前作用域不销毁,所以用传引用返回
{
swap(_head, lt._head);//直接用库里面的函数
return * this;
}
List(initializer_list<T> il)
{
empty_init();
for (const auto& e : il)
{
push_back(e);
}
}
private:
Node* _head;
};
//按需实例化
void test_list1()
{
List<int> L;
//实例化就报错
L.push_back(1);
L.push_back(2);
L.push_back(3);
L.push_back(4);
List<int>::iterator it = L.begin();
while (it != L.end())
{
*it += 10; // *it 解引用,返回的是这个节点的数据
// 此时可以修改这个节点数据的内容
cout << *it << " ";
//*it 解引用要的是数值,不是节点,所以运算符重载了operator*()
++it;
}
cout << endl;
for (auto& e : L)
{
cout << e << " ";
}
cout << endl;
}
struct Pos
{
int _row;
int _col;
Pos( int row = 0,int col = 0)
:_row(row)
,_col(col)
{}
};
void test_list3()
{
List<Pos> L;
L.push_back(Pos(100, 100));
L.push_back(Pos(200, 200));
L.push_back(Pos(300, 300));
List<Pos>::iterator it = L.begin();
while (it !=L. end())
{
cout << it->_row << ":" << it->_col << endl;
++it;
}
cout << endl;
}
void Func(const List<int>& lt)
{
//List<int>::const_iterator it = lt.begin();
//while (it!=lt.end())
//{
// //*it += 10; //const迭代器 指向的内容不能改变
// cout << *it << " ";//可读
// ++it;//可遍历
//}
//cout << endl;
List<int>::const_iterator it = lt.begin();
while (it != lt.end())
{
//*it += 10;//const迭代器 指向的内容不能修改
cout << *it << " ";//可读
++it;//可遍历
}
cout << endl;
}
void test_List3()
{
List<int> L;
L.push_back(1);
L.push_back(2);
L.push_back(3);
L.push_back(4);
L.push_back(5);
Func(L);
L.push_front(10);
L.push_front(20);
L.push_front(30);
Func(L);
L.pop_front();
L.pop_front();
Func(L);
L.pop_back();
L.pop_back();
L.pop_back();
/*L.pop_back();
L.pop_back();
L.pop_back();
L.pop_back();
L.pop_back();
L.pop_back();*/
Func(L);
}
void test_List4()
{
List<int> L1;
L1.push_back(1);
L1.push_back(2);
L1.push_back(3);
L1.push_back(4);
L1.push_back(5);
Func(L1);
List<int> L2(L1);
L1.push_back(6);
Func(L2);
Func(L1);
List<int> L3;
L3.push_back(1);
L3.push_back(2);
L3.push_back(3);
L1 = L3;
Func(L1);
Func(L3);
}
void test_List5()
{
List<int> L1 = { 1,2,3,4,5,6 };
Func(L1);
}
}
如若对你有帮助,记得点赞、收藏、关注哦!
若有误,望各位,在评论区留言或者私信我 指点迷津!!!谢谢^ ^ ~