概述:
- 算法主要由头文件<algorithm><functional><numeric>组成
- <algorithm>是所有STL头文件最大的一个,范围涉及到比较,交换,遍历操作,复制,修改等
- <numeric>体积很小,只包括几个在序列上面进行简单数学运算的模板函数
- <functional>定义了一些模板类,用以声明函数对象
一、常用遍历算法
算法简介:
- for_each //遍历容器
- transform //搬运容器到另一个容器中
(一)for_each
功能描述:
- 实现遍历容器
函数原型:
- for_each(iterator beg,iterator end ,_func);
//遍历算法 遍历容器元素
//beg 开始迭代器
//end 结束迭代器
//_func 函数或者函数对象
cpp
#include<iostream>
using namespace std;
#include<algorithm>
#include<vector>
//普通函数
void print01(int val){
cout<<val<<" ";
}
//仿函数
class print02{
public:
void operator()(const int& val)const{
cout<<val<<" ";
}
};
//常用遍历算法 for_each()
void test01(){
vector<int> v;
for(int i=0;i<10;i++){
v.push_back(i);
}
for_each(v.begin(),v.end(),print01);
cout<<endl;
for_each(v.begin(),v.end(),print02());
cout<<endl;
}
int main(){
test01();
system("pause");
return 0;
}
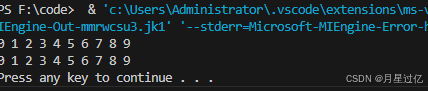
(二)transfrom
功能描述:
- 搬运容器到另一个容器中
函数原型:
- transfrom(iterator beg1,iterator end,iterator begin2,_func);
//beg1源容器开始迭代器
//end 源容器结束迭代器
/beg2 目标容器开始迭代器
//_func函数或者函数对象
cpp
#include<iostream>
#include<algorithm>
#include<vector>
using namespace std;
//transform()函数的使用
class Transform{
public:
int operator()(const int& a){
return a+100; //还可以在仿函数中加一些逻辑运算
}
};
class MyPrint{
public:
void operator()(const int& a){
cout<<a<<" ";
}
};
void test01(){
vector<int> v;
for(int i=0;i<10;i++){
v.push_back(i);
}
vector<int> v2;
v2.resize(v.size()); //transform前必须扩容
transform(v.begin(),v.end(),v2.begin(),Transform());
for_each(v2.begin(),v2.end(),MyPrint());
}
int main(){
test01();
system("pause");
return 0;
}
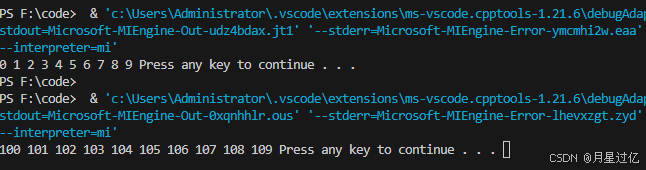
二、常用的查找算法
算法简介:
- find //查找元素
- find_if //按条件查找元素
- adjancent_find //查找相邻重复元素
- binary_serach //二分查找法
- count //统计元素个数
- count_if //按条件统计个数
(一)find
功能描述:
- 查找制定元素,找到返回指定元素的迭代器,找不到返回结束迭代器end()
函数原型:
- find(iterator beg,iterator end,value);
//按值查找元素,找到返回制定位置的迭代器,找不到返回结束迭代器位置
//beg 开始迭代器
//end 结束迭代器
//value 查找的元素
cpp
#include<iostream>
using namespace std;
#include<vector>
#include<algorithm>
//常用的查找算法
//find
//查找内置的数据类型
void test01(){
vector<int>v;
for(int i=0;i<10;i++){
v.push_back(i);
}
//查找容器中是否有5这个元素
vector<int>::iterator it=find(v.begin(),v.end(),5);
if(it == v.end()){
cout<<"没有找到"<<endl;
}else{
cout<<"找到了"<<endl;
}
}
class Person{
public:
Person(string name,int age){
this->m_name=name;
this->m_age=age;
}
//重载== 底层find知道如何对比Person数据类型
bool operator==(const Person& p){
if(this->m_name == p.m_name && this->m_age == p.m_age){
return true;
}else{
return false;
}
}
string m_name;
int m_age;
};
void test02(){
vector<Person> v;
//创建数据
Person p1("aaa",10);
Person p2("bbb",20);
Person p3("ccc",30);
v.push_back(p1);
v.push_back(p2);
v.push_back(p3);
vector<Person>::iterator it =find(v.begin(),v.end(),p3);
if(it == v.end()){
cout<<"没有找到"<<endl;
}else{
cout<<"找到了"<<endl;
cout<<"姓名:"<<it->m_name<<" 年龄:"<<it->m_age<<endl;
}
}
//查找自定义的数据类型
int main(){
test01();
test02();
system("pause");
return 0;
}
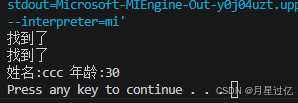
(二)find_if
功能描述:
- 按条件查找元素
函数原型:
- find_if(iterator beg,iterator end ,_Pred);
//按值查找元素,找到返回指定位置迭代器,找不到返回结束迭代器位置
//beg 开始迭代器
//end 结束迭代器
//_Pred 函数或者谓词(返回bool类型的仿函数)
cpp
#include<iostream>
#include<algorithm>
#include<vector>
using namespace std;
//常用的查找算法 find_if()
//1.查找内置数据类型
class GreaterFive
{
public:
bool operator()(const int& val) const{
return val>5;
}
};
void test01(){
vector<int> v;
for(int i=0;i<10;i++){
v.push_back(i);
}
vector<int>::iterator it = find_if(v.begin(),v.end(),GreaterFive());
if(it == v.end()){
cout<<"没有找到"<<endl;
}else{
cout<<"找到了"<<*it<<endl;
}
}
//2.查找自定义数类型
class Person{
public:
Person(string name,int age)
{
this->m_name = name;
this->m_age = age;
}
string m_name;
int m_age;
};
class MyFind{
public:
bool operator()(const Person& p)const{
return p.m_age >18;
}
};
void test02(){
vector<Person> v;
Person p1("Tom",19);
Person p2("Jerry",20);
Person p3("Mary",17);
v.push_back(p1);
v.push_back(p2);
v.push_back(p3);
vector<Person>::iterator it = find_if(v.begin(),v.end(),MyFind());
if(it == v.end()){
cout<<"没有找到"<<endl;
}else{
cout<<"找到了"<<(*it).m_name<<endl;
}
}
int main(){
test01();
test02();
system("pause");
return 0;
}
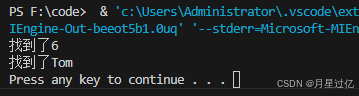
(三)adjacent_find
功能描述:
- 查找相邻重复元素
函数原型:
- adjacent_find(iterator beg,iterator end);
//查找相邻重复元素,返回相邻元素的第一个位置的迭代器
//beg开始迭代器
//end结束迭代器
cpp
#include <iostream>
#include <vector>
#include <algorithm>
using namespace std;
//常用查找算法 adjacent_find();
void test01(){
vector<int>v;
v.push_back(0);
v.push_back(2);
v.push_back(3);
v.push_back(1);
v.push_back(4);
v.push_back(3);
v.push_back(3);
vector<int>::iterator pos = adjacent_find(v.begin(),v.end());
if (pos !=v.end()){
cout<<"找到相邻重复元素" <<*pos <<endl;
}else{
cout<<"没有找到相邻重复元素"<<endl;
}
}
int main(){
test01();
system("pause");
return 0;
}
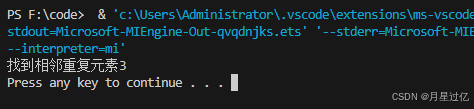
(四)binary_search
功能描述:
- 查找指定元素是否存在
函数原型:
- bool binary_search(iterator beg,iterator end ,value);
//查找指定的元素,查到返回true 否则返回false
//注意:在无序序列中不可用
//beg开始迭代器
//end 结束迭代器
//value 查找到元素
cpp
#include <iostream>
using namespace std;
#include<vector>
#include<algorithm>
//常用查找算法 binary_search
void test01(){
vector<int> v;
for(int i=0;i<10;i++){
v.push_back(i);
}
bool flag= binary_search(v.begin(),v.end(),9);
if(flag){
cout<<"9 is in the vector"<<endl;
}else{
cout<<"9 is not in the vector"<<endl;
}
}
int main(){
test01();
system("pause");
return 0;
}

(五)count
功能描述:
- 统计元素个数
函数原型:
- count (iterator beg,iterator end ,value);
//统计元素出现的次数
//beg开始迭代器
//end结束迭代器
//value统计的元素
cpp
#include<iostream>
#include<vector>
#include<algorithm>
using namespace std;
//常用查找算法count
//1.统计内置数据类型
void test01(){
vector<int> v;
v.push_back(10);
v.push_back(40);
v.push_back(30);
v.push_back(40);
v.push_back(20);
v.push_back(40);
v.push_back(40);
int num = count(v.begin(),v.end(),40);
cout<<"num of 40 is "<<num<<endl;
}
//2.统计自定义数据类型
class Person{
public:
Person(string name,int age){
this->m_name=name;
this->m_age=age;
}
bool operator == (const Person& p) const{
if(this->m_age==p.m_age){
return true;
}
return false;
}
string m_name;
int m_age;
};
void test02(){
vector<Person> v;
Person p1("刘备",20);
Person p2("关羽",20);
Person p3("张飞",20);
Person p4("Tom",40);
Person p5("Tom",60);
v.push_back(p1);
v.push_back(p2);
v.push_back(p3);
v.push_back(p4);
v.push_back(p5);
Person p("诸葛亮",20);
int num =count(v.begin(),v.end(),p);
cout<<"和诸葛亮同岁数的人数有"<<num<<"个"<<endl;
}
int main(){
test01();
test02();
system("pause");
return 0;
}
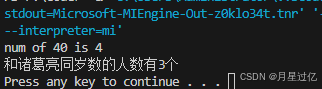
(六)count_if
功能描述:
- 按条件统计元素个数
函数原型:
- count_if(iterator beg,iterator end ,_Pred);
//按条件统计元素出现个数
//beg开始迭代器
//end 结束迭代器
//_Pred谓词
cpp
#include<iostream>
#include<vector>
#include<algorithm>
using namespace std;
//常用查找算法 count_if
//统计内置数据类型
class Greater20{
public:
bool operator()(const int& x)const{
return x>20;
}
};
void test01(){
vector<int> v;
v.push_back(10);
v.push_back(20);
v.push_back(30);
v.push_back(40);
v.push_back(50);
int num =count_if(v.begin(),v.end(),Greater20());
cout<<"大于20的数有"<<num<<"个"<<endl;
}
//统计自定义数据类型
class Person{
public:
Person(string name,int age){
this->m_name=name;
this->m_age=age;
}
string m_name;
int m_age;
};
class AgeGreater20{
public:
bool operator()(const Person& p)const{
return p.m_age>20;
}
};
void test02(){
vector<Person> v;
Person p1("Tom",20);
Person p2("Jerry",30);
Person p3("Mike",40);
Person p4("Lucy",50);
v.push_back(p1);
v.push_back(p2);
v.push_back(p3);
v.push_back(p4);
int num =count_if(v.begin(),v.end(),AgeGreater20());
cout<<"年龄大于20的有"<<num<<"个"<<endl;
}
int main(){
test01();
test02();
system("pause");
return 0;
}
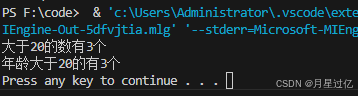
三、常用的排序算法
算法简介:
- sort //对容器内元素进行排序
- random_shuffle //洗牌 指定范围内的元素随机调整次序
- merge //容器元素合并,并存储到另一容器中
- reverse //反转指定范围的元素
(一)sort
功能描述:
- 对容器内元素进行排序
函数原型:
- sort(iterator beg,iterator end ,_Pred);
//beg 开始迭代器
//end 结束迭代器
//Pred 谓词
cpp
#include <iostream>
#include <algorithm>
#include <vector>
#include <functional>
using namespace std;
//常用排序算法 sort
void myPrint(int val){
cout<<val<<" ";
}
void test01(){
vector<int>v;
v.push_back(3);
v.push_back(1);
v.push_back(4);
v.push_back(2);
v.push_back(5);
//升序排序
sort(v.begin(),v.end());
for_each(v.begin(),v.end(),myPrint);
//改为降序
cout<<endl;
sort(v.begin(),v.end(),greater<int>());
for_each(v.begin(),v.end(),myPrint);
}
int main(){
test01();
system("pause");
return 0;
}
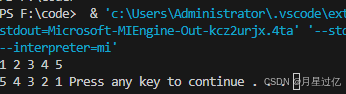
(二)random_shuffle
功能描述:
- 洗牌 指定范围内的元素随机调整次序
函数原型:
- random_shuffle(iterator beg,iterator end);
//指定范围内的元素随机调整次序
//beg 开始迭代器
//end 结束迭代器
cpp
#include <iostream>
#include <algorithm>
#include <vector>
#include <ctime>
using namespace std;
//常用排序算法 random_shuffle()
void myPrint(int val){
cout<<val<<" ";
}
void test01()
{
srand((unsigned)time(NULL));
vector<int>v;
for(int i=0;i<10;i++)
{
v.push_back(i);
}
random_shuffle(v.begin(),v.end());
for_each(v.begin(),v.end(),myPrint);
cout<<endl;
}
int main() {
test01();
system("pause");
return 0;
}
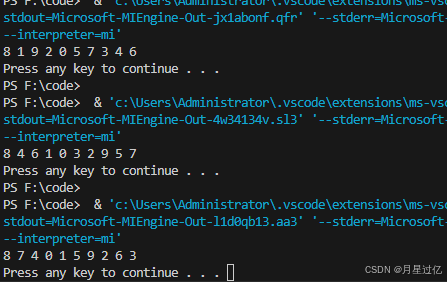
总结:random_shuffle洗牌算法比较实用,使用时记得加随机数种子
(三)merge
功能描述:
- 两个容器元素合并,并存储到另一容器中
函数原型:
- merge(iterator beg1,iterator end1,iterator beg2,iterator end2,iterator dest);
//容器元素合并,并存储到另一容器中
//注意:两个容器必须是有序的
//beg1 容器1开始迭代器
//end1 容器1结束迭代器
//beg2 容器2开始迭代器
//end2 容器2结束迭代器
//dest 目标容器开始迭代器
cpp
#include <iostream>
#include <vector>
#include <algorithm>
using namespace std;
//常用的排序算法 merge
void myPrint(int val){
cout<<val<<" ";
}
void test01(){
vector<int>v1;
vector<int>v2;
for(int i=0;i<10;i++){
v1.push_back(i);
v2.push_back(i+1);
}
//目标容器
vector<int>v3;
v3.resize(v1.size()+v2.size());//提前预留空间
//合并两个容器
merge(v1.begin(),v1.end(),v2.begin(),v2.end(),v3.begin());
for_each(v3.begin(),v3.end(),myPrint);
cout<<endl;
}
int main() {
test01();
system("pause");
return 0;
}
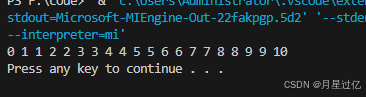
总结:merge合并的两个容器必须是有序序列,并且合并后的容器仍然是有序序列
(四)reverse
功能描述:
- 将容器中的元素进行反转
函数原型:
- reverse(iterator beg,iterator end);
//反转指定范围的元素
//beg开始迭代器
//end结束迭代器
cpp
#include <iostream>
#include <string>
#include <algorithm>
#include <vector>
//常用的排序算法 reverse
using namespace std;
void myPrint(int val){
cout<<val<<" ";
}
void test01(){
vector<int>v;
v.push_back(1);
v.push_back(3);
v.push_back(2);
v.push_back(5);
v.push_back(4);
cout<<"反转前:"<<" ";
for_each(v.begin(),v.end(),myPrint);
cout<<endl;
cout<<"反转后:"<<" ";
reverse(v.begin(),v.end());
for_each(v.begin(),v.end(),myPrint);
cout<<endl;
}
int main(){
test01();
system("pause");
return 0;
}
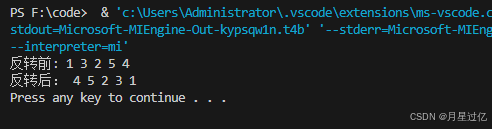
四、常用拷贝和替换算法
算法简介:
- copy //容器内指定范围的元素拷贝到另一容器中
- replace //将容器内指定范围的旧元素修改为新元素
- repace_if //容器内指定范围满足条件的元素替换为新元素
- swap //互换两个容器的元素
(一)copy
功能描述:
- 容器内指定范围的元素拷贝到另一容器中
函数原型:
- copy(iterator beg,iterator end,iterator dest);
//beg 开始迭代器
//end 结束迭代器
//dest 目标起始迭代器
cpp
#include <iostream>
#include <string>
#include <vector>
#include <algorithm>
using namespace std;
//常用拷贝和替换算法
void myPrint(int val){
cout<<val<<" ";
}
void test01(){
vector<int> v1;
for(int i=0;i<10;i++){
v1.push_back(i);
}
vector<int>v2;
v2.resize(v1.size());
copy(v1.begin(),v1.end(),v2.begin());
for_each(v2.begin(),v2.end(),myPrint);
}
int main(){
test01();
system("pause");
return 0;
}
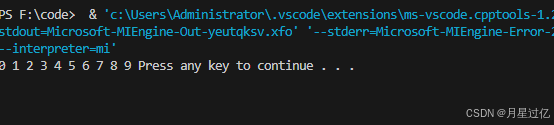
(二)replace
功能描述:
- replace(iterator beg,iterator end,oldvalue,newvalue);
//将区间内旧元素替换成新元素
//beg 开始迭代器
//end 结束迭代器
//oldvalue 就元素
//new value 新元素
cpp
#include <iostream>
#include <string>
#include <vector>
#include <algorithm>
using namespace std;
//常用拷贝和替换算法 replace
void myPrint(int val){
cout<<val<<" ";
}
void test01(){
vector<int>v1;
v1.push_back(10);
v1.push_back(20);
v1.push_back(30);
v1.push_back(40);
v1.push_back(30);
v1.push_back(30);
v1.push_back(40);
cout<<"替换前"<<endl;
for_each(v1.begin(),v1.end(),myPrint);
cout<<endl;
cout<<"替换后"<<endl;
replace(v1.begin(),v1.end(),30,300);
for_each(v1.begin(),v1.end(),myPrint);
}
int main(){
test01();
system("pause");
return 0;
}
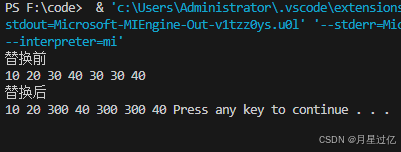
(三)replace_if
功能描述:
- 将区间内满足条件的元素,替换成指定元素
函数原型:
- replace_if(iterator beg,iterator end,_pred,newvalue);
//按照条件替换元素,满足条件的替换成指定元素
//beg开始迭代器
//end 结束迭代器
//-Pred谓词
//newvalue 替换成的新元素
cpp
#include <iostream>
#include <string>
#include <vector>
#include <algorithm>
using namespace std;
//常用拷贝和替换算法
void myPrint(int val){
cout<<val<<" ";
}
class MyReplace{
public:
bool operator()(const int &val)const{
return val>20;
}
};
void test01(){
vector<int>v;
v.push_back(20);
v.push_back(30);
v.push_back(40);
v.push_back(40);
v.push_back(60);
v.push_back(10);
v.push_back(80);
v.push_back(20);
v.push_back(90);
cout<<"替换前:"<<" ";
for_each(v.begin(),v.end(),myPrint);
cout<<endl;
cout<<"替换后:"<<" ";
replace_if(v.begin(),v.end(),MyReplace(),1000);
for_each(v.begin(),v.end(),myPrint);
}
int main(){
test01();
system("pause");
return 0;
}

(四)swap
功能描述:
- 互换两个容器的元素
函数原型:
- swap(container c1,container c2);
//互换两个容器的元素
//c1容器1
//c2容器2
cpp
#include <iostream>
#include <algorithm>
#include <vector>
using namespace std;
void myPrint(int val){
cout<<val<<" ";
}
void test01(){
vector<int> v1;
vector<int> v2;
for(int i=0;i<10;i++){
v1.push_back(i);
v2.push_back(i+100);
}
cout<<"交换前:"<<"v1"<<" ";
for_each(v1.begin(),v1.end(),myPrint);
cout<<endl;
cout<<"交换前:"<<"v2"<<" ";
for_each(v2.begin(),v2.end(),myPrint);
cout<<endl;
swap(v1,v2);
cout<<"交换后:"<<"v1"<<" ";
for_each(v1.begin(),v1.end(),myPrint);
cout<<endl;
cout<<"交换后:"<<"v2"<<" ";
for_each(v2.begin(),v2.end(),myPrint);
}
int main(){
test01();
system("pause");
return 0;
}
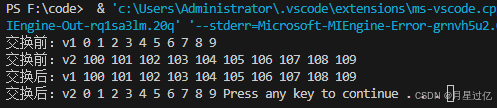
五、常用的算数生成算法
注意:
- 算数生成算法属于小型算法,使用时包含的头文件为#include <numeric>
算法简介:
- accumulate //计算容器元素累计总和
- fill //向容器中添加元素
(一)accumulate
功能描述:
- 计算区间内容器元素累计总和
函数原型:
- accumulate(iterator beg,iterator end,value);
//计算容器元素累计总和
//beg 开始迭代器
//end 结束迭代器
//value 起始值
cpp
#include<iostream>
#include<vector>
#include<numeric>
using namespace std;
//常用算数生成算法
void test01(){
vector<int> v;
for(int i=1;i<=100;i++){
v.push_back(i);
}
int sum =accumulate(v.begin(),v.end(),0);
cout<<"sum:"<<sum<<endl;
}
int main(){
test01();
system("pause");
return 0;
}
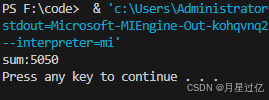
(二)fill
功能描述:
- 像容器中填充指定的元素
函数原型:
- fill(iterator beg,iterator end,value);
//向容器中填充元素
//beg 开始迭代器
//end 结束迭代器
//value 填充的值
cpp
#include <iostream>
#include <vector>
#include <numeric>
#include <algorithm>
using namespace std;
void myPrint(int x){
cout<<x<<" ";
}
//常用算数生成算法 fill
void test01(){
vector<int> v;
v.resize(10); //需要扩容
fill(v.begin(),v.end(),100);
for_each(v.begin(),v.end(),myPrint);
cout<<endl;
}
int main(){
test01();
system("pause");
return 0;
}
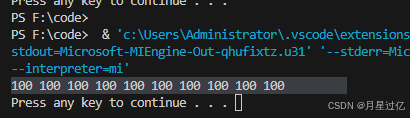
六、常用集合算法
算法简介:
- set_intersection //求两个容器的交集
- set_union //求两个容器的并集
- set_difference //求两个容器的差集
(一)set_intersection
功能描述:
- 求两个容器的交集
函数原型:
- set_intersection(iterator beg1,iterator end1,iterator beg2,iterator end2,iterator dest);
//求两个集合的交集
//注意:两个集合必须是有序序列
//beg1 容器1开始迭代器
//end1 容器1结束迭代器
//beg2 容器2开始迭代器
//end2 容器2结束迭代器
//dest 目标容器开始迭代器
cpp
#include <iostream>
#include <vector>
#include <algorithm>
using namespace std;
void myPrint(int val){
cout<<val<<" ";
}
void test01(){
vector<int>v1;
vector<int>v2;
vector<int>v3;
for(int i=0;i<10;i++){
v1.push_back(i);
v2.push_back(i+5);
}
//目标容器提前开辟空间
//最特殊情况 大容器包含了小容器 --》取小容器即可
v3.resize(min(v1.size(),v2.size()));
//获取交集
vector<int>::iterator itEnd = set_intersection(v1.begin(),v1.end(),v2.begin(),v2.end(),v3.begin());
for_each(v3.begin(),v3.end(),myPrint);
cout<<endl;
for_each(v3.begin(),itEnd,myPrint); //交集计算后的迭代器
cout<<endl;
}
int main() {
test01();
system("pause");
return 0;
}
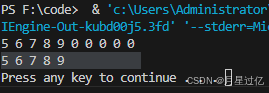
(二)set_union
功能描述:
- 求两个集合的并集
函数原型:
- set_union(iterator beg1,iterator end1,iterator beg2,iterator end2,iterator dest);
//求两个集合的并集
//注意:两个集合必须是有序序列
//beg1 容器1开始迭代器
//end1 容器1结束迭代器
//beg2 容器2开始迭代器
//end2 容器2结束迭代器
//dest 目标容器开始迭代器
cpp
#include <iostream>
#include <vector>
#include <algorithm>
using namespace std;
//常用集合算法Union
void MyPrint(int val){
cout<<val<<" ";
}
void test01(){
vector<int> v1;
vector<int> v2;
vector<int> v3;
for(int i=0;i<10;i++){
v1.push_back(i);
v2.push_back(i+5);
}
v3.resize(v1.size()+v2.size());
//合并两个集合
vector<int>::iterator itEnd = set_union(v1.begin(),v1.end(),v2.begin(),v2.end(),v3.begin());
for_each(v3.begin(),v3.end(),MyPrint);
cout<<endl;
for_each(v3.begin(),itEnd,MyPrint);
}
int main(){
test01();
system("pause");
return 0;
}

(三)set_difference
功能描述:
- 求两个集合的差集
函数原型:
- set_difference(iterator beg1,iterator end1,iterator beg2,iterator end2,iterator dest);
//求两个集合的并集
//注意:两个集合必须是有序序列
//beg1 容器1开始迭代器
//end1 容器1结束迭代器
//beg2 容器2开始迭代器
//end2 容器2结束迭代器
//dest 目标容器开始迭代器
cpp
#include <iostream>
#include <vector>
#include <algorithm>
using namespace std;
//求两个集合的差集
void MyPrint(int val){
cout<<val<<" ";
}
void test01(){
vector<int>v1;
vector<int>v2;
vector<int>v3;
vector<int>v4;
for(int i=0;i<10;i++){
v1.push_back(i);
v2.push_back(i+5);
}
v3.resize(max(v1.size(),v2.size()));
v4.resize(max(v1.size(),v2.size()));
//取差集
vector<int>::iterator itEnd=set_difference(v1.begin(),v1.end(),v2.begin(),v2.end(),v3.begin());
//输出差集
cout<<"v1-v2的交集"<<endl;
for_each(v3.begin(),v3.end(),MyPrint);
cout<<endl;
for_each(v3.begin(),itEnd,MyPrint);
cout<<endl;
cout<<"v2-v1的交集"<<endl;
vector<int>::iterator itEnd2=set_difference(v2.begin(),v2.end(),v1.begin(),v1.end(),v4.begin());
for_each(v4.begin(),v4.end(),MyPrint);
cout<<endl;
for_each(v4.begin(),itEnd2,MyPrint);
cout<<endl;
}
int main(){
test01();
system("pause");
return 0;
}
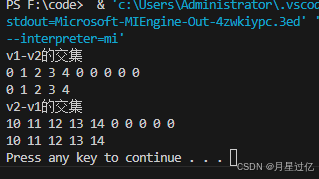