题意:"使用 PHP 从 OpenAI GPT-3 API 流式传输数据"
问题背景:
I'm having trouble with the OpenAI API, Basically what I'm trying to do is stream each data node that is streamed back from the openai API response and output each data node one at a time as it streams in from the API call, but I have no clue how this is done, I researched for hours and can not find any information on how this can be achieved with PHP.
"我在使用 OpenAI API 时遇到了问题。基本上,我想要做的是流式处理从 OpenAI API 响应中返回的每个数据节点,并在从 API 调用中接收到时逐个输出这些数据节点,但我不知道如何实现这一点。我研究了好几个小时,却找不到任何关于如何使用 PHP 实现这一点的信息。"
How can I get my code to output each data node in real time as the API streams in the data?
The Following is the best I can come up with, it outputs all the data at once after the call is complete, but It does not stream in the data.
"如何让我的代码在 API 流式传输数据时实时输出每个数据节点?
以下是我能想到的最好办法,但它在调用完成后一次性输出所有数据,而不是实时流式传输数据。"
php
function openAI(){
$OPENAI_API_KEY="API_KEY_GOES_HERE";
$user_id="1"; // users id optional
$prompt="tell me what you can do for me.";
$temperature=0.5; // 1 adds complete randomness 0 no randomness 0.0
$max_tokens=30;
$data = array('model'=>'text-davinci-002',
'prompt'=>$prompt,
'temperature'=>$temperature,
'max_tokens'=>$max_tokens,
'top_p'=>1.0,
'stream'=>TRUE,// stream back response
'frequency_penalty'=>0.0,
'presence_penalty'=>0.0,
'user' => $user_id);
$post_json= json_encode($data);
$ch = curl_init();
curl_setopt($ch, CURLOPT_URL, 'https://api.openai.com/v1/completions');
curl_setopt($ch, CURLOPT_RETURNTRANSFER, 1);
curl_setopt($ch, CURLOPT_POST, 1);
curl_setopt($ch, CURLOPT_SSL_VERIFYHOST, false);
curl_setopt($ch, CURLOPT_SSL_VERIFYPEER, false);
curl_setopt($ch, CURLOPT_POSTFIELDS, $post_json);
$headers = array();
$headers[] = 'Content-Type: application/json';
// $headers[] = 'Content-Type: text/event-stream';
$headers[] = "Authorization: Bearer $OPENAI_API_KEY";
curl_setopt($ch, CURLOPT_HTTPHEADER, $headers);
$result = curl_exec($ch);
return $result;
curl_close($ch);
}
echo openAI();
问题解决:
I ended up solving my problem. Hopefully my answer will help someone in the future.
I made the following additions to my code. This simple logic makes what I inquired about work.
"我最终解决了我的问题。希望我的答案能在未来帮助到其他人。
我在代码中做了以下添加。这种简单的逻辑实现了我所询问的功能。"
php
// This should be at the very top, alternatively can be set in you php.ini file
@ini_set('zlib.output_compression',0);
@ini_set('implicit_flush',1);
// This function discards the contents of the topmost output buffer and turns off this output buffering.
@ob_end_clean();
The following curl_setopt should also be added. I personally added it on the line after CURLOPT_POSTFIELDS
"还应添加以下 curl_setopt
。我个人是在 CURLOPT_POSTFIELDS
之后的一行添加的。"
php
curl_setopt($ch, CURLOPT_WRITEFUNCTION, function($curl, $data) {
# str_repeat(' ',1024*8) is needed to fill the buffer and will make streaming the data possible
echo $data.str_repeat(' ',1024*8);
return strlen($data);
});
Alternatively instead of adding str_repeat(' ',1024*8) you can shut off buffering in your web servers config file, e.g.(nginx.conf)
"或者,可以不添加 str_repeat(' ',1024*8)
,而是在你的 Web 服务器配置文件(如 nginx.conf)中关闭缓冲。"
gzip off;
proxy_buffering off;
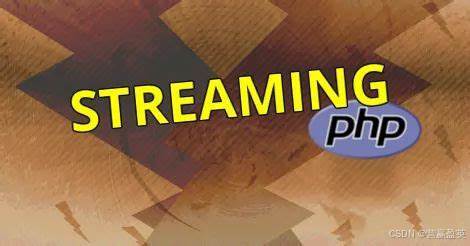