一、思维导图
二、myString
cpp
#ifndef MYSTRING_H
#define MYSTRING_H
#include <iostream>
#include<cstring>
using namespace std;
class myString
{
public:
//无参构造
myString();
//有参构造
myString(const char *str);
//析构函数
~myString();
//判空函数
bool is_empty();
//strlen函数
int strlen();
//get_size
int get_capacity();
//c_str函数
const char* c_str();
//at函数
char& at(int index);
//clear函数
void clear();
//二倍扩容
void expend();
//data函数
char* data();
//operator =
void operator=(const myString &other);
//operator +
myString& operator+(const myString &other);
//operator []
char& operator[](int index);
//operator +=
myString& operator+=(const myString &other);
//operator ==字符内容相等即可
bool operator==(const myString &other);
//operator != 字符内容不相等即可
bool operator!=(const myString &other);
//operator > 字符内容大于即可
bool operator>(const myString &other);
//operator >= 字符内容大于等于即可
bool operator>=(const myString &other);
//operator < 字符内容小于即可
bool operator<(const myString &other);
//operator <= 字符内容小于等于即可
bool operator<=(const myString &other);
//push_back,末尾添加字符
void push_back(const char &other);
//push_back,末尾删除字符
void pop_back();
private:
char *str; //记录c风格的字符串
int len; //记录字符串的实际长度
int capacity; //记录String在堆区申请的空间大小
//将赋值运算符重载函数,设置成友元函数
friend ostream & operator<<(ostream &mycout, const myString &value);
friend istream & operator>>(istream &mycin,myString &value);
};
#endif // MYSTRING_H
cpp
#include"myString.h"
//无参构造
myString::myString():capacity(10),len(0)
{
str = new char[capacity]; //构造出一个默认长度为10的字符串
str[0] = '\0';
}
//有参构造
myString::myString(const char *str)
{
capacity =10;
this->str = new char[capacity];
int count = 0;
char* ptr =(char *)str;
while(*ptr != '\0')
{
ptr++;
count++;
if(count >= capacity)
expend();
}
strcpy(this->str,str);
len = count;
}
//析构函数
myString::~myString()
{
delete[] str;
cout<<"空间释放成功\n"<<endl;
}
//判空函数
bool myString::is_empty()
{
return len<=0?1:0;
}
//strlen函数
int myString::strlen()
{
return len;
}
//get_size
int myString::get_capacity()
{
return capacity;
}
//c_str函数
const char* myString::c_str()
{
return str;
}
//at函数
char& myString::at(int index)
{
if(index<0 || index>= len)
{
cout <<"输入错误"<<endl;
}
char &str = this->str[index];
return str;
}
//data函数,返回字符串首字符地址
char* myString::data()
{
return this->str;
}
//二倍扩容
void myString::expend()
{
char* temp = new char [capacity*2];
strcpy(temp, str); // 将旧字符串复制到新内存空间
delete[] str;
str = temp;
capacity *= 2;
}
//clear函数
void myString::clear()
{
this->len = 0;
}
//push_back,末尾添加字符
void myString::push_back(const char &other)
{
//判断大小
if((len+1) == this->capacity)
{
//二倍扩容
this->expend();
}
str[len] = other;
this->len++;
}
//push_back,末尾删除字符
void myString::pop_back()
{
str[len-1] = '\0';
this->len--;
}
//operator +=
myString& myString::operator+=(const myString &other)
{
while (this->capacity< (this->len+other.len)) {
//二倍扩容
this->expend();
}
strcat(this->str,other.str);
this->len = this->len+other.len;
return *this;
}
//operator =
void myString::operator=(const myString &other)
{
this->capacity = other.capacity;
this->len = other.len;
strcpy(this->str,other.str);
this->len = other.len;
}
//operator +
myString& myString::operator+(const myString &other)
{
static myString Temp;
while (Temp.capacity< (this->len+other.len)) {
//二倍扩容
Temp.expend();
}
strcpy(Temp.str,this->str);
strcat(Temp.str,other.str);
Temp.len = this->len+other.len;
return Temp;
}
//operator == 字符内容相等即可
bool myString::operator==(const myString &other)
{
if(strcmp(this->str,other.str) == 0)
{
return true;
}else
{
return false;
}
}
//operator != 字符内容不相等即可
bool myString::operator!=(const myString &other)
{
if(strcmp(this->str,other.str) != 0)
{
return true;
}else
{
return false;
}
}
//operator > 字符内容大于即可
bool myString::operator>(const myString &other)
{
if(strcmp(this->str,other.str) > 0)
{
return true;
}else
{
return false;
}
}
//operator >= 字符内容大于等于即可
bool myString::operator>=(const myString &other)
{
if(strcmp(this->str,other.str) >= 0)
{
return true;
}else
{
return false;
}
}
//operator < 字符内容小于即可
bool myString::operator<(const myString &other)
{
if(strcmp(this->str,other.str) < 0)
{
return true;
}else
{
return false;
}
}
//operator <= 字符内容小于等于即可
bool myString::operator<=(const myString &other)
{
if(strcmp(this->str,other.str) <= 0)
{
return true;
}else
{
return false;
}
}
//自定义输出运算符重载函数:只能实现全局函数版
ostream & operator<<(ostream &mycout, const myString &value)
{
//输出内容
if(value.len > 0)
{
mycout<<value.str;
}
//返回左操作数自身
return mycout;
}
//自定义插入运算符重载函数:只能实现全局函数版
istream& operator>>(istream &mycin, myString &value)
{
//输入内容
char temp[1024] = {0};
mycin>>temp;
while(value.len < strlen(temp))
{
//二倍扩容
value.expend();
}
strcpy(value.str,temp);
value.len = strlen(temp);
//返回左操作数自身
return mycin;
}
//operator []
char& myString::operator[](int index)
{
if(index<0 || index>= this->len)
{
cout <<"输入错误"<<endl;
}
char &str = this->str[index];
return str;
}
cpp
#include"myString.h"
int main()
{
//实例化一个myString的str,调用了myString的无参构造
myString str1;
cout<<"str1_size ="<<str1.get_capacity()<<endl; //输出默认size
cout<<"str1_len ="<<str1.strlen()<<endl; //输出默认len
//有参构造
myString str2("hello world");
cout<<"str2_size ="<<str2.get_capacity()<<endl; //输出size
cout<<"str2_len ="<<str2.strlen()<<endl; //输出len
//c_str函数
const char *str = str2.c_str();
cout<<"str ="<<str<<endl;
//at函数
cout<<"str[8] ="<<str[8]<<endl;
//operator =
str1 = str2;
//cout<<"str1 ="<<str1<<endl;
//operator +
myString str3 = str1 + str2;
cout<<"str1 ="<<str1<<endl;
cout<<"str2 ="<<str2<<endl;
cout<<"str3 ="<<str3<<endl;
//push_back,末尾添加字符
str1.push_back('H');
cout<<"str1 ="<<str1<<endl;
//push_back,末尾删除字符
str1.pop_back();
cout<<"str1 ="<<str1<<endl;
//operator == 字符内容相等即可
if(str1 == str2)
{
cout<<"str1 == str2"<<endl;
}
//operator +=
str3 += str1;
cout<<"str3 ="<<str3<<endl;
//operator >>
cout<<"请输入str1"<<endl;
cin>>str1;
cout<<"str1 ="<<str1<<endl;
cout<<"str1.len ="<<str1.strlen()<<endl;
//operator []
cout<<"str1[2] ="<<str1[2]<<endl;
return 0;
}
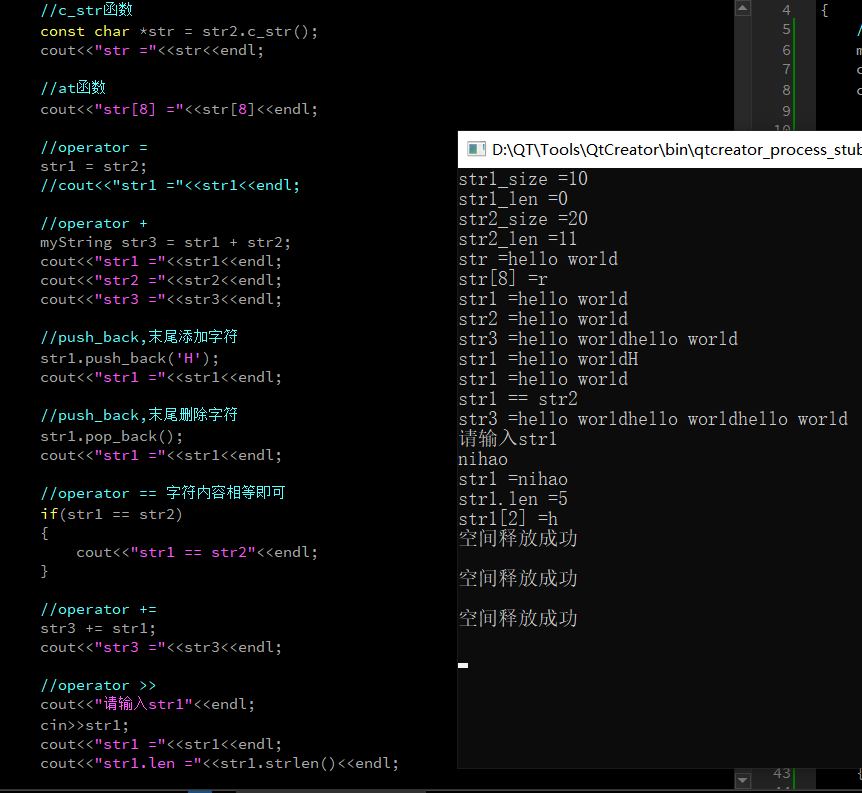