实现my_string中可能实现的功能
cpp
#include <iostream>
#include<cstring>
using namespace std;
class my_string
{
char *str; //记录c风格的字符串
int size; //记录字符串的实际长度
int capacit;//记录最大容量
public:
//无参的构造函数
my_string():size(0),capacit(15){
str = new char[capacit];
}
// 带参数的构造函数
my_string(const char *s):capacit(15) {
size = strlen(s) ;
if(size>capacit){
extend();
}
str = new char[capacit];
strcpy(str, s);
}
//拷贝构造函数
my_string(const my_string &other):str(new char[other.capacit]),size(other.size),capacit(other.capacit){
strcpy(str,other.str);
cout<<"拷贝构造函数"<<endl;
}
//移动构造函数
my_string(const my_string &&other):str(new char[other.capacit]),size(other.size){
strcpy(str,other.str);
cout<<"拷贝构造函数"<<endl;
}
//拷贝赋值函数
my_string &operator=(const my_string&other){
if(this!=&other){
size=other.size;
capacit=other.capacit;
str=new char[capacit];
strcpy(str,other.str);
}
cout<<"拷贝赋值函数"<<endl;
return *this;
}
//移动赋值函数
my_string &operator=(const my_string &&other){
if(this!=&other){
size=other.size;
capacit=other.capacit;
str=new char[capacit];
strcpy(str,other.str);
}
cout<<"拷贝赋值函数"<<endl;
return *this;
}
~my_string(){
delete []str;
}
friend bool operator<=(const my_string &L,const my_string &R);
friend bool operator>=(const my_string &L,const my_string &R);
friend istream &operator>>(istream &L,my_string &R);
friend ostream &operator<<(ostream &L,const my_string &R);
friend bool operator>(const my_string &L,const my_string &R);
friend bool operator<(const my_string &L,const my_string &R);
friend bool operator!=(const my_string &L,const my_string &R);
friend bool operator==(const my_string &L,const my_string &R);
friend const my_string operator+(const my_string &L,const my_string &R);
bool my_string_empty();//判空函数
int str_size(); //字符串长度
char * my_c_ctl(){//将c++的字符串转化为c风格的字符串
return str;
}
char &at(int pos){//仿at功能
return str[pos];
}
void extend();//二倍扩容
void show();
int capacity(){
return this->capacit;
}
//清空字符串
void clear(){
str[0]='\0';
}
//push_back
void push_back(char a){
if(size>=capacit){
extend();
}
str[this->size]=a;
size++;
}
//pop_back
bool pop_back(){
if(size==0){ return false;}
str[size-1]='\0';
size--;
return true;
}
//运算符+=重载函数
void operator+=(char a);
};
void my_string::operator+=(char a){
if(size>=capacit){
extend();
}
str[this->size]=a;
size++;
}
void my_string::extend(){
char *arg;
arg=new char[capacit*2+1];//在堆区申请一个两倍capacity大小的空间
strcpy(arg,str);//将原先str字符串复制到arg中;
delete []str;//删除原先的在堆区的str
str=arg;
}
void my_string::show(){
cout<<str<<endl;
}
bool my_string::my_string_empty(){//判空函数
if(strlen(str)==0){
return true;
}
else
return false;
}
int my_string::str_size(){ //字符串长度
return strlen(str);
}
//运算符+重载函数
const my_string operator+(const my_string &L,const my_string &R){
my_string temp;
temp.size = L.size + R.size;
temp.capacit=L.capacit;
if(temp.size>=temp.capacit){
temp.extend();
}
temp.str = new char[temp.capacit];
strcpy(temp.str, L.str);
strcat(temp.str, R.str);
return temp;
}
//运算符==重载函数
bool operator==(const my_string &L,const my_string &R){
return strcmp(L.str,R.str)==0;
}
//运算符!=重载函数
bool operator!=(const my_string &L,const my_string &R){
return strcmp(L.str,R.str)!=0;
}
//运算符>重载函数
bool operator>(const my_string &L,const my_string &R){
return strcmp(L.str,R.str)>0;
}
//运算符>=重载函数
bool operator>=(const my_string &L,const my_string &R){
return strcmp(L.str,R.str)>=0;
}
//运算符<=重载函数
bool operator<=(const my_string &L,const my_string &R){
return strcmp(L.str,R.str)<=0;
}
//运算符<重载函数
bool operator<(const my_string &L,const my_string &R){
return strcmp(L.str,R.str)<0;
}
//<<重载函数
ostream &operator<<(ostream &L,const my_string &R){
L<<R.str<<endl;
return L;
}
//>>重载函数
istream &operator>>(istream &L,my_string &R){
L>>R.str;
R.size=strlen(R.str);
return L;
}
int main()
{
my_string s1("aaa");
my_string s2(s1);
s2.show();
cout<<"********************************"<<endl;
my_string s3;
s3=s2;
s3.show();
cout<<"********************************"<<endl;
my_string s4=s1+s2;
s4.show();
cout<<"********************************"<<endl;
if(s4!=s1){
cout<<"yes"<<endl;
}
else{
cout<<"no"<<endl;
}
cout<<"********************************"<<endl;
my_string s5="abcd";
cout<<s5;
cout<<"********************************"<<endl;
my_string s6;
cin>>s6;
cout<<s6;
cout<<"********************************"<<endl;
s6.push_back('a');
cout<<s6;
cout<<"********************************"<<endl;
s6.clear();
cout<<s6;
cout<<"********************************"<<endl;
cout<<s5.capacity();
return 0;
}
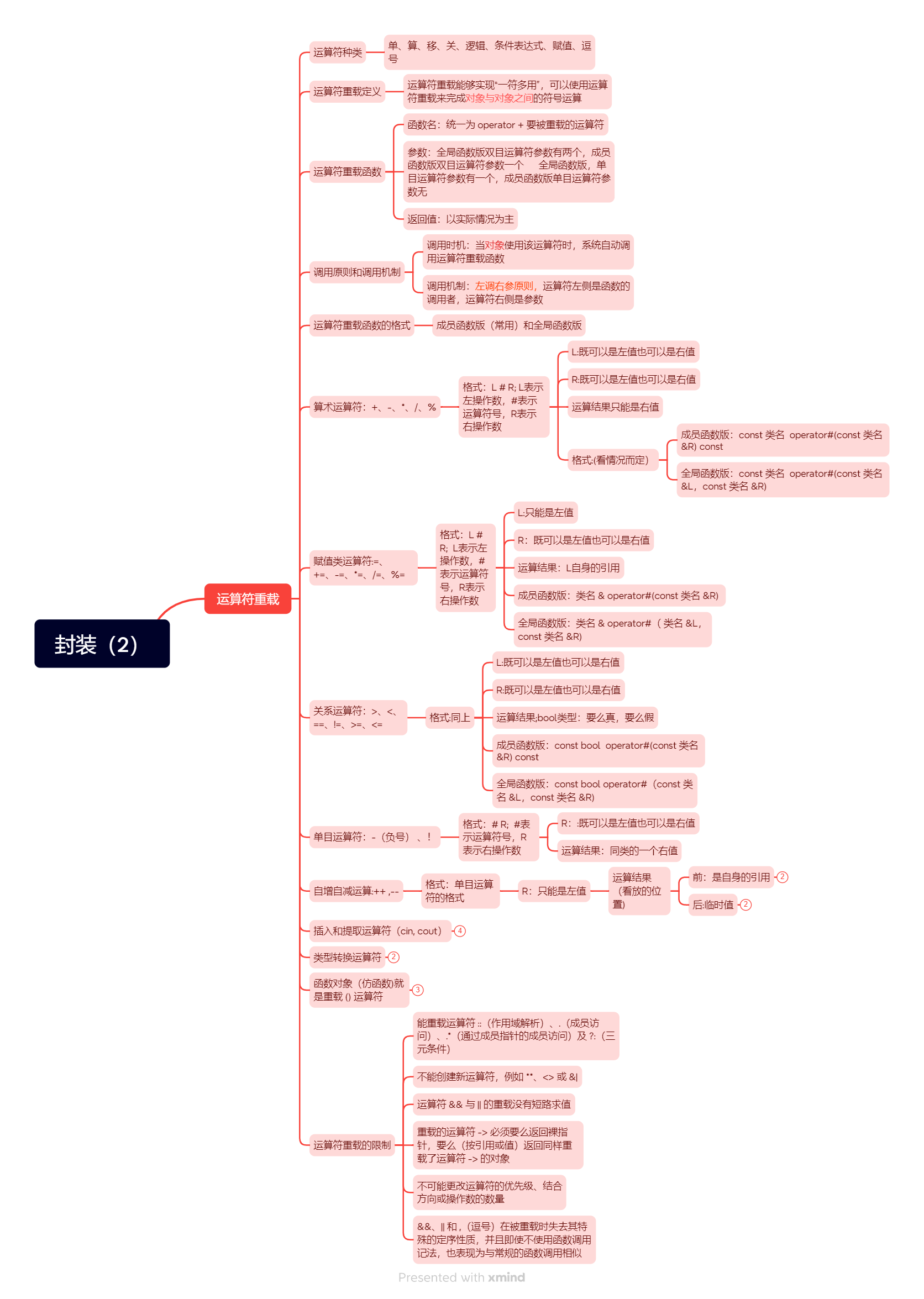
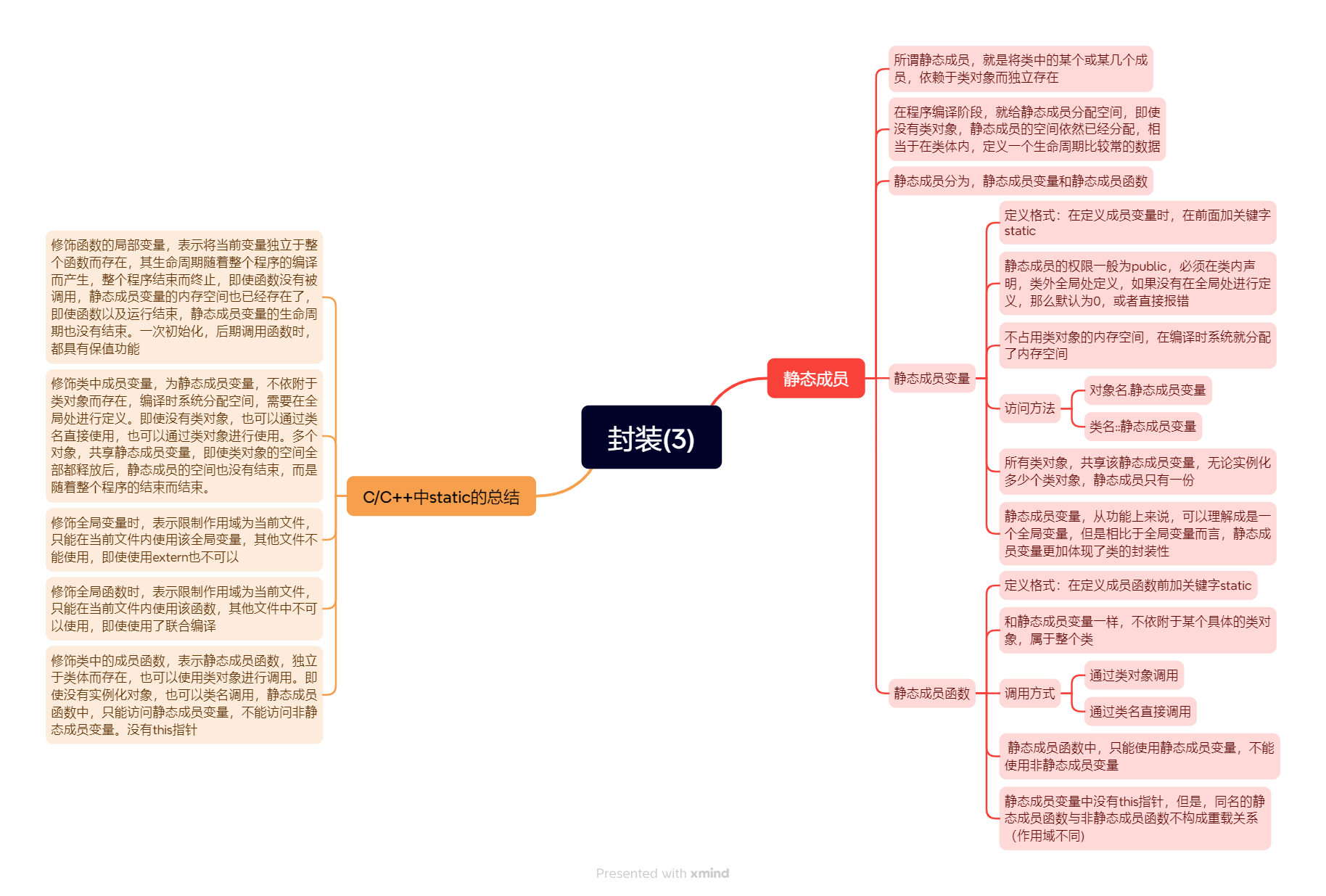
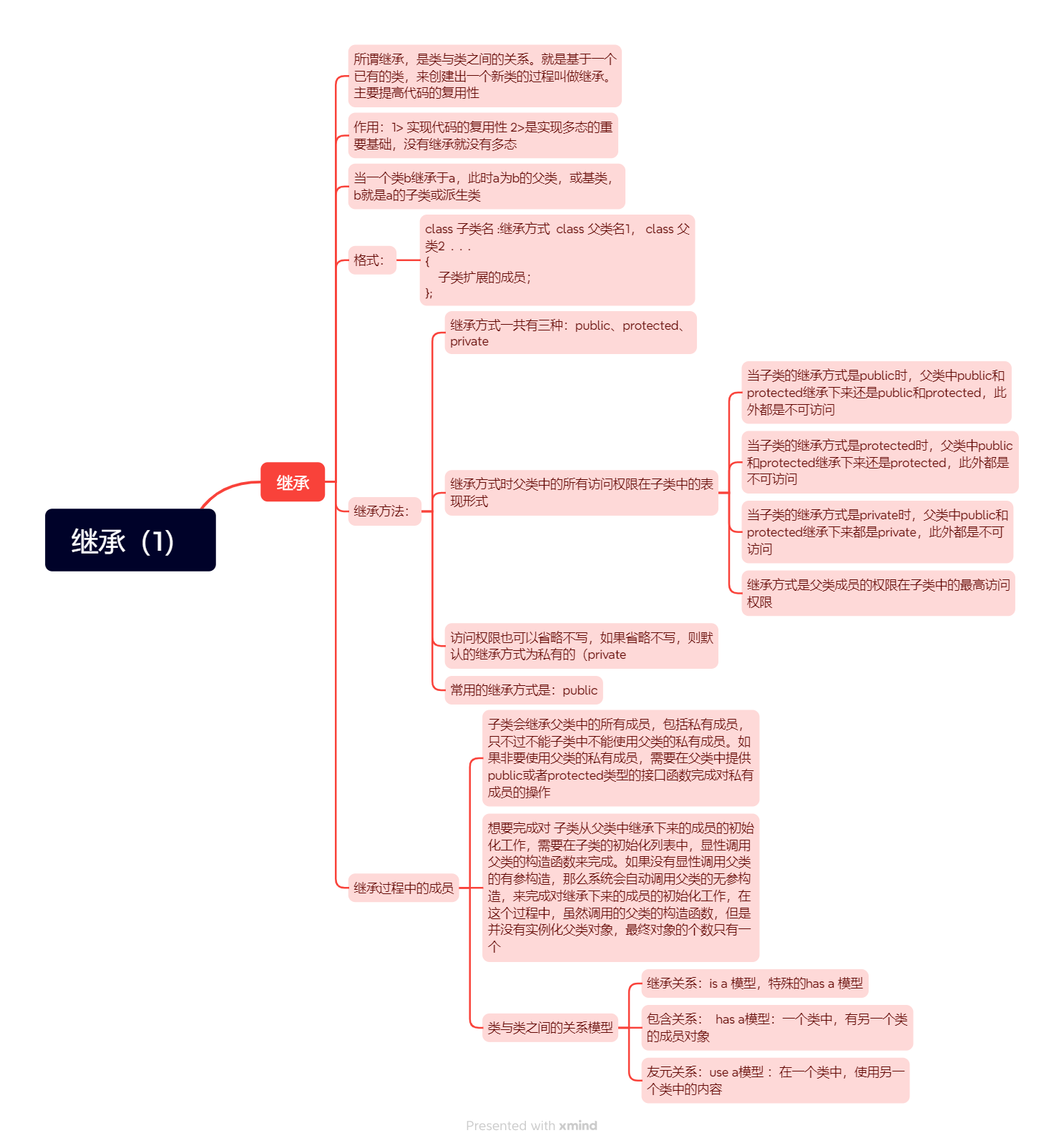