房贷计算程序,主要实现以下功能:
- 用户友好的界面:使用文本菜单来引导用户选择功能。
- 支持不同还款频率:例如每季度还款、每半年还款等。
- 支持贷款提前还款:计算提前还款对总支付利息的影响。
- 详细的还款计划表:输出每月的本金、利息和总还款额。
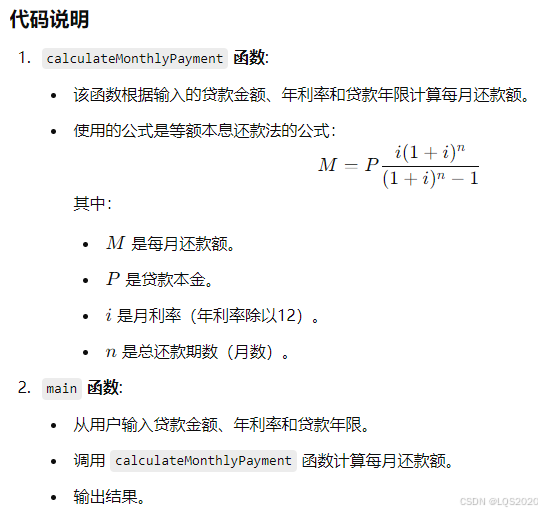
下面是一个复杂的C++程序示例,涵盖了以上功能:
C++ 程序代码
cpp
#include <iostream>
#include <iomanip>
#include <cmath>
#include <vector>
using namespace std;
// Function to validate user input
bool validateInput(double value) {
return value > 0;
}
// Function to calculate monthly payment for equal principal repayment
double calculateEqualPrincipalPayment(double principal, double annualInterestRate, int years) {
double monthlyInterestRate = annualInterestRate / 12 / 100;
int totalMonths = years * 12;
double totalPayment = 0;
for (int month = 1; month <= totalMonths; ++month) {
double remainingPrincipal = principal * (totalMonths - month + 1) / totalMonths;
double monthlyPayment = (remainingPrincipal * monthlyInterestRate) + (principal / totalMonths);
totalPayment += monthlyPayment;
}
return totalPayment / totalMonths; // Average monthly payment
}
// Function to calculate monthly payment for equal monthly installments
double calculateEqualInstallmentsPayment(double principal, double annualInterestRate, int years) {
double monthlyInterestRate = annualInterestRate / 12 / 100;
int totalMonths = years * 12;
double numerator = monthlyInterestRate * std::pow(1 + monthlyInterestRate, totalMonths);
double denominator = std::pow(1 + monthlyInterestRate, totalMonths) - 1;
return principal * (numerator / denominator);
}
// Function to generate a repayment schedule
void generateRepaymentSchedule(double principal, double annualInterestRate, int years, int frequency) {
double rate = annualInterestRate / frequency / 100;
int totalPeriods = years * frequency;
double principalPayment = principal / totalPeriods;
cout << fixed << setprecision(2);
cout << "Repayment Schedule:\n";
cout << setw(10) << "Period" << setw(20) << "Principal Payment" << setw(20) << "Interest Payment" << setw(20) << "Total Payment\n";
double remainingPrincipal = principal;
for (int period = 1; period <= totalPeriods; ++period) {
double interestPayment = remainingPrincipal * rate;
double totalPayment = principalPayment + interestPayment;
remainingPrincipal -= principalPayment;
cout << setw(10) << period
<< setw(20) << principalPayment
<< setw(20) << interestPayment
<< setw(20) << totalPayment
<< endl;
}
}
// Main function
int main() {
double principal; // Loan amount
double annualInterestRate; // Annual interest rate in percentage
int years; // Loan term in years
int repaymentOption; // Repayment option (1 for Equal Principal, 2 for Equal Installments)
int frequency; // Repayment frequency (e.g., 12 for monthly, 4 for quarterly, 2 for semi-annually)
cout << "Enter the loan amount: ";
cin >> principal;
if (!validateInput(principal)) {
cerr << "Invalid loan amount. Must be greater than zero." << endl;
return 1;
}
cout << "Enter the annual interest rate (in percentage): ";
cin >> annualInterestRate;
if (!validateInput(annualInterestRate)) {
cerr << "Invalid annual interest rate. Must be greater than zero." << endl;
return 1;
}
cout << "Enter the loan term (in years): ";
cin >> years;
if (!validateInput(years)) {
cerr << "Invalid loan term. Must be greater than zero." << endl;
return 1;
}
cout << "Choose repayment option (1 for Equal Principal, 2 for Equal Installments): ";
cin >> repaymentOption;
if (repaymentOption != 1 && repaymentOption != 2) {
cerr << "Invalid option. Choose 1 or 2." << endl;
return 1;
}
cout << "Choose repayment frequency (12 for monthly, 4 for quarterly, 2 for semi-annually): ";
cin >> frequency;
if (frequency != 12 && frequency != 4 && frequency != 2) {
cerr << "Invalid frequency. Choose 12, 4, or 2." << endl;
return 1;
}
double payment;
if (repaymentOption == 1) {
payment = calculateEqualPrincipalPayment(principal, annualInterestRate, years);
cout << "Your average payment (Equal Principal) is: $" << payment << endl;
} else if (repaymentOption == 2) {
payment = calculateEqualInstallmentsPayment(principal, annualInterestRate, years);
cout << "Your monthly payment (Equal Installments) is: $" << payment << endl;
}
// Calculate total payment and total interest
int totalPeriods = years * frequency;
double totalPayment = payment * totalPeriods;
double totalInterest = totalPayment - principal;
cout << "Total payment over the life of the loan: $" << totalPayment << endl;
cout << "Total interest paid: $" << totalInterest << endl;
// Generate repayment schedule
generateRepaymentSchedule(principal, annualInterestRate, years, frequency);
return 0;
}
扩展说明
-
输入验证:
validateInput
函数检查输入值是否大于零,确保输入的有效性。
-
还款方式:
- 等额本金还款 :使用
calculateEqualPrincipalPayment
函数计算平均每月还款额。 - 等额本息还款 :使用
calculateEqualInstallmentsPayment
函数计算每月还款额。
- 等额本金还款 :使用
-
还款频率:
- 通过用户选择的频率(例如每月、每季度、每半年)来调整还款计划。
-
详细的还款计划表:
generateRepaymentSchedule
函数生成详细的还款计划,包括每期的本金支付、利息支付和总支付额。
编译和运行
-
保存代码:
- 将代码保存为一个C++源文件,例如
advanced_loan_calculator.cpp
。
- 将代码保存为一个C++源文件,例如
-
编译代码:
-
使用C++编译器进行编译,例如使用
g++
编译器:bashg++ -o advanced_loan_calculator advanced_loan_calculator.cpp
-
-
运行程序:
-
执行编译后的程序:
bash./advanced_loan_calculator
-
这个扩展后的程序提供了多种还款方式和详细的还款计划表,可以帮助用户更好地了解其贷款的还款情况。