题意:文件上传 - 服务的端点和OpenAI生成
问题背景:
My Endpoint is a RestConroller with a Post-Method looking like this:
我的端点是一个带有 Post 方法的 RestController,如下所示
java
@PostMapping(path = "/xetra", consumes = {
MediaType.APPLICATION_JSON_VALUE,
MediaType.MULTIPART_FORM_DATA_VALUE})
public @ResponseBody void importXetra(@RequestParam("file") MultipartFile file) {
After OpenAI Generator i get the following signature for the endpoint:
使用 OpenAI 生成器后,我得到的端点签名如下
java
public importXetra(importXetraRequest?: ImportXetraRequest, observe?: 'body', reportProgress?: boolean, options?: {httpHeaderAccept?: undefined, context?: HttpContext}): Observable<any>;
public importXetra(importXetraRequest?: ImportXetraRequest, observe?: 'response', reportProgress?: boolean, options?: {httpHeaderAccept?: undefined, context?: HttpContext}): Observable<HttpResponse<any>>;
public importXetra(importXetraRequest?: ImportXetraRequest, observe?: 'events', reportProgress?: boolean, options?: {httpHeaderAccept?: undefined, context?: HttpContext}): Observable<HttpEvent<any>>;
public importXetra(importXetraRequest?: ImportXetraRequest, observe: any = 'body', reportProgress: boolean = false, options?: {httpHeaderAccept?: undefined, context?: HttpContext}): Observable<any> {
with
java
export interface ImportXetraRequest {
file: Blob;
}
My formular looks like: 我的表单如下:
XML
<p-fileUpload name="myfile[]" [customUpload]="true" (uploadHandler)="upload($event)">
<ng-template pTemplate="toolbar">
<div class="py-1">Upload 1 Files</div>
</ng-template>
<ng-template let-file pTemplate="file">
<div>Custom UI to display a file</div>
</ng-template>
my upload implementation: 我的上传实现
javascript
upload (event : {files: Blob[]}) : void {
let file = event.files[0] as File;
let request: ImportXetraRequest = ({} as any) as ImportXetraRequest;
request.file = file;
this.importEndpoint.importXetra(request).subscribe({
next : (res : void) => console.log("imported"),
error: (e: ErrorEvent) => console.error("error = " + e),
});
}
I don't know how exactly i can do the call to the backend, the backend says it is not a valid request. I get a 500-error. It also seems that the payload is not set/empty.
我不确定如何正确地调用后端,后端提示请求无效。我收到一个500错误。似乎负载没有设置或为空
问题解决:
The problem was, that the endpoint didn't have the correct MediaType Consumer:
问题在于,该端点没有正确的MediaType Consumer
javascript
@PostMapping(path = "/xetra", consumes = {
MediaType.MULTIPART_FORM_DATA_VALUE,
})
solved the problem. 解决了问题
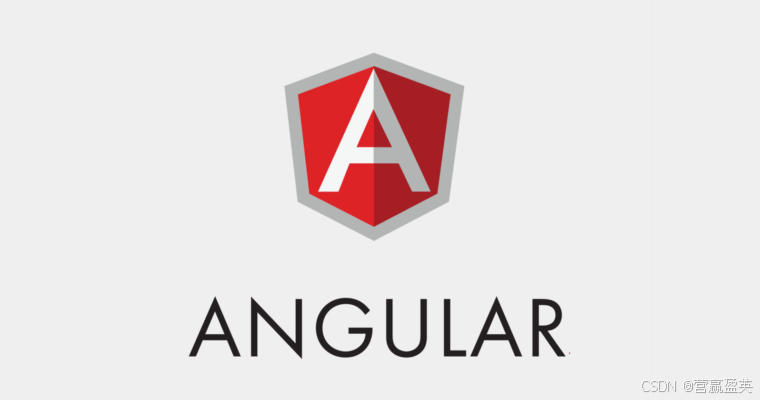