目录
[2.1 构造函数( (constructor)) +接口说明](#2.1 构造函数( (constructor)) +接口说明)
[2.2 list iterator 的使用](#2.2 list iterator 的使用)
[2.3 list capacity](#2.3 list capacity)
[2.4 list element access](#2.4 list element access)
[2.5 list modifiers](#2.5 list modifiers)
前言
前面我们学习了vector,本节我们将对新的容器list进行拆分学习,并且有了string和vector的基础,list容器的方法学习起来就会轻松很多。
1.list的介绍
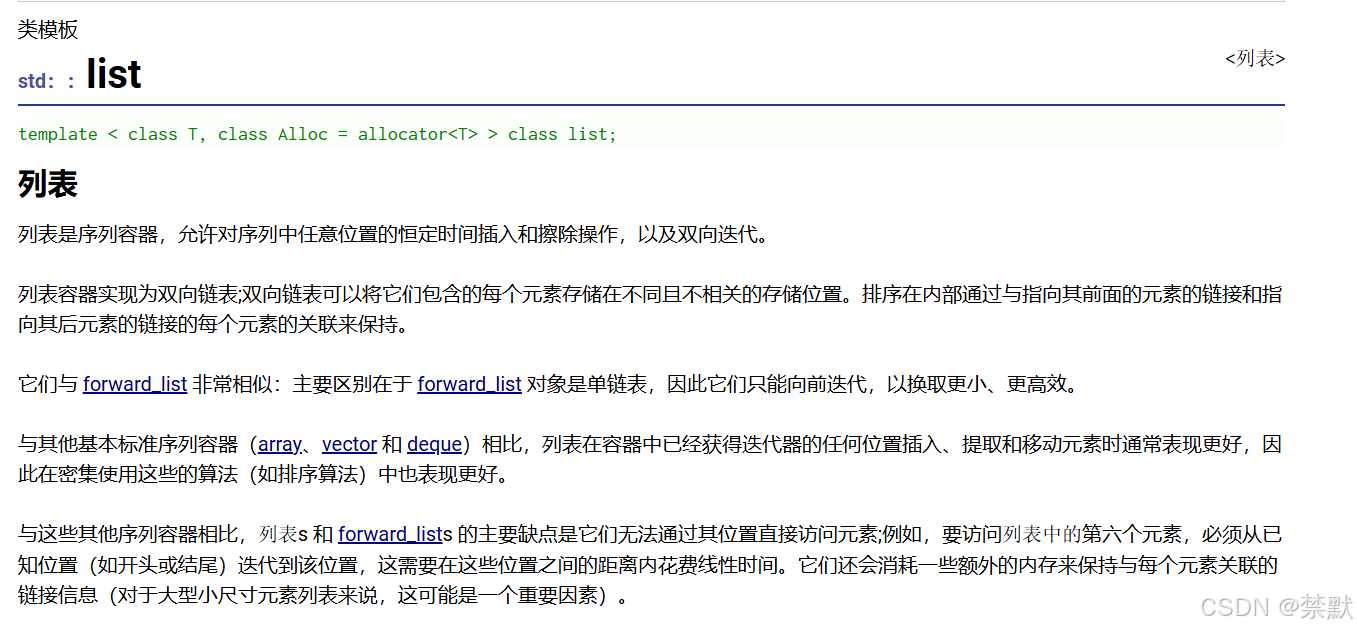
1. list是可以在常数范围内在任意位置进行插入和删除的序列式容器,并且该容器可以前后双向迭代。
2. list的底层是双向链表结构,双向链表中每个元素存储在互不相关的独立节点中,在节点中通过指针指向 其前一个元素和后一个元素。
3. list与forward_list非常相似:最主要的不同在于forward_list是单链表,只能朝前迭代,已让其更简单高 效。
4. 与其他的序列式容器相比(array,vector,deque),list通常在任意位置进行插入、移除元素的执行效率更好。
5. 与其他序列式容器相比,list和forward_list最大的缺陷是不支持任意位置的随机访问,比如:要访问list 的第6个元素,必须从已知的位置(比如头部或者尾部)迭代到该位置,在这段位置上迭代需要线性的时间 开销;list还需要一些额外的空间,以保存每个节点的相关联信息(对于存储类型较小元素的大list来说这可能是一个重要的因素)
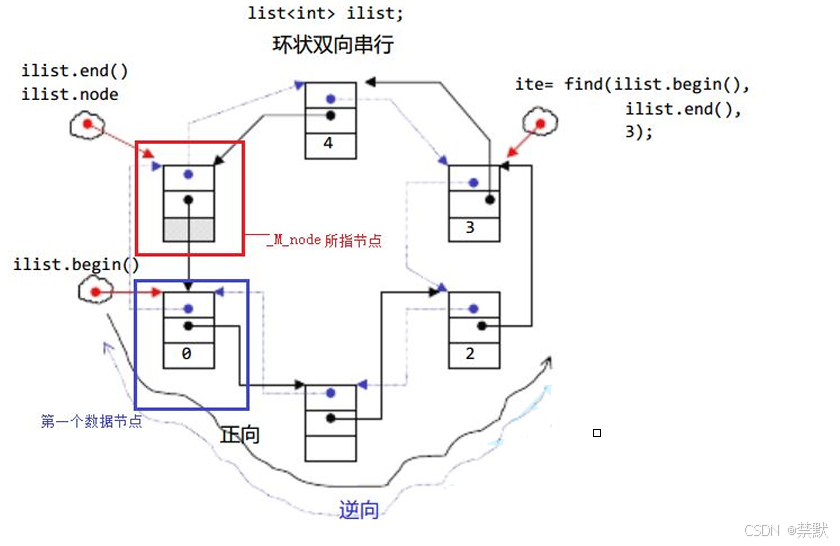
2.list的常见接口
2.1 构造函数( (constructor)) +接口说明
list (size_type n, const value_type& val = value_type()) 构造的list中包含n个值为val的元素
list() 拷贝空的list
list (const list& x) 拷贝构造函数
list (InputIterator first, InputIterator last) 用[first, last)区间中的元素构造list
cpp
void test1() {
list<int>l1;
list<int>l2(5, 10);
list<int>l3(l2.begin(), l2.end());//迭代器构造
list<int>l4(l2);//拷贝构造
//以数组区间迭代器构造list
float arr[] = { 5.20,13.14,9.99,8.88 };
list<float>l5(arr, arr + sizeof(arr) / sizeof(float));
// 列表格式初始化C++11
list<int> l6{ 1,2,3,4,5 };
// 用迭代器方式打印l5中的元素
list<float> ::iterator it = l5.begin();
while (it != l5.end()) {
cout << *it << " ";
it++;
}
cout << endl;
// C++11范围for的方式遍历
for (auto e : l6) {
cout << e << " ";
}
}
注意:遍历链表只能用迭代器和范围for
2.2 list iterator 的使用
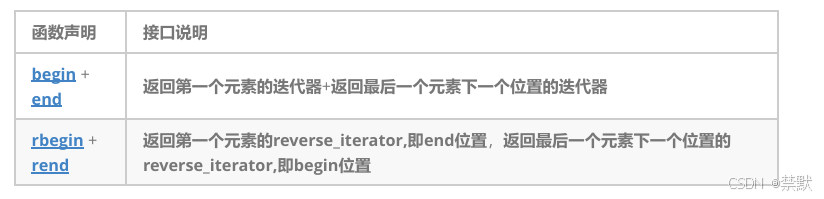
cpp
void TestList2()
{
int array[] = { 1, 2, 3, 4, 5, 6, 7, 8, 9, 0 };
list<int> l(array, array + sizeof(array) / sizeof(array[0]));
// 使用正向迭代器正向list中的元素
// list<int>::iterator it = l.begin(); // C++98中语法
auto it = l.begin(); // C++11之后推荐写法
while (it != l.end())
{
cout << *it << " ";
++it;
}
cout << endl;
// 使用反向迭代器逆向打印list中的元素
// list<int>::reverse_iterator rit = l.rbegin();
auto rit = l.rbegin();
while (rit != l.rend())
{
cout << *rit << " ";
++rit;
}
cout << endl;
}

跟vector几乎是一样的
注意:
1. begin与end为正向迭代器,对迭代器执行++操作,迭代器向后移动
2. rbegin(end)与rend(begin)为反向迭代器,对迭代器执行++操作,迭代器向前移动
2.3 list capacity
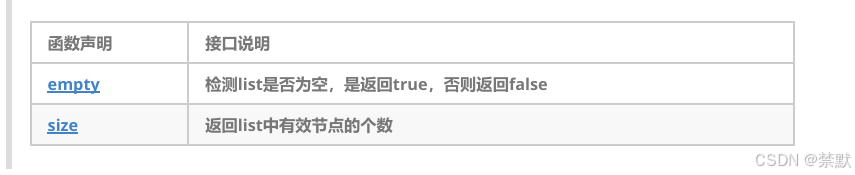
2.4 list element access
官方测试代码展示
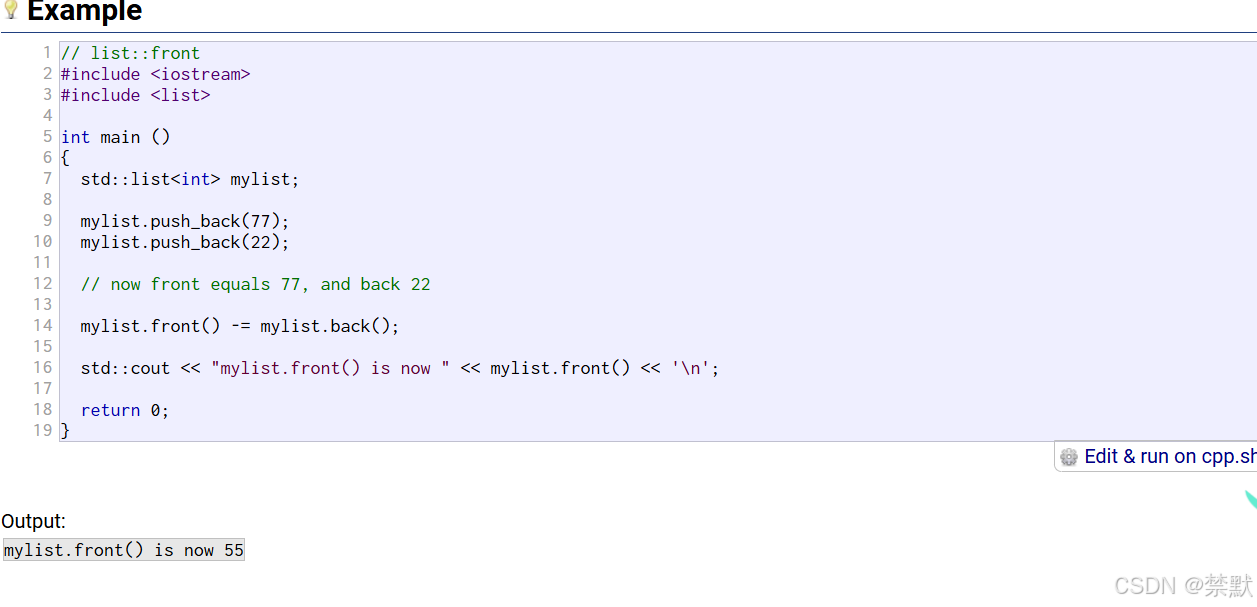
cpp
list<int> mylist;
mylist.push_back(10);
while (mylist.back() != 0)
{
mylist.push_back(mylist.back() - 1);
}
cout << "mylist contains:";
for (list<int>::iterator it = mylist.begin(); it != mylist.end(); ++it)
std::cout << ' ' << *it;
cout << '\n';

2.5 list modifiers
push_front 在list首元素前插入值为val的元素 pop_front 删除list中第一个元素
push_back 在list尾部插入值为val的元素 pop_back删除list中最后一个元素 insert 在list position 位置中插入值为val的元素 erase删除list position位置的元素
swap交换两个list中的元素 clear清空list中的有效元素
cpp
void print_list(const list<int>& ml) {
// 注意这里调用的是list的 begin() const,返回list的const_iterator对象
list<int>::const_iterator it = ml.begin();
while (it != ml.end()) {
cout << *it << " ";
it++;
}
cout << endl;
}
void TestList3() {
list<int>mylist{ 1,2,3,4,5 };
mylist.push_back(6);
mylist.push_front(0);
print_list(mylist);
mylist.pop_back();
mylist.pop_front();
print_list(mylist);
}
void TestList4()
{
int array1[] = { 1, 2, 3 };
list<int> L(array1, array1 + sizeof(array1) / sizeof(array1[0]));
// 获取链表中第二个节点
//auto pos = ++L.begin();
list<int>::iterator pos = ++L.begin();
cout << *pos << endl;
// 在pos前插入值为4的元素
L.insert(pos, 4);
print_list(L);
// 在pos前插入5个值为5的元素
L.insert(pos, 5, 5);
print_list(L);
// 在pos前插入[v.begin(), v.end)区间中的元素
vector<int> v{ 7, 8, 9 };
L.insert(pos, v.begin(), v.end());
print_list(L);
// 删除pos位置上的元素
L.erase(pos);
print_list(L);
// 删除list中[begin, end)区间中的元素,即删除list中的所有元素
L.erase(L.begin(), L.end());
print_list(L);
}
这里我们设置了一个打印链表值的函数,方便打印链表,只是打印整数,想打印其他值可以参考vector建立一个模版打印函数,让编译器自己推测打印数据的类型。
cpp
template <class Container>
void print(const Container& v) {
auto it = v.begin();
while (it != v.end()) {
cout << *it << " ";
it++;
}
cout << endl;
}
cpp
void TestList5()
{
// 用数组来构造list
int array1[] = { 1, 2, 3 ,4 ,5};
list<int> l1(array1, array1 + sizeof(array1) / sizeof(array1[0]));
print_list(l1);
list<int>l2{ 6,7,8,9,10 };
// 交换l1和l2中的元素
l1.swap(l2);
print_list(l1);
print_list(l2);
// 将l2中的元素清空
l2.clear();
cout << l2.size() << endl;
}
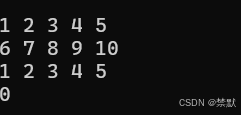
3.list的迭代器失效
此处可将迭代器暂时理解成类似于指针,迭代器失效即迭代器所指向的节点的无效,即该节 点被删除了。因为list的底层结构为带头结点的双向循环链表,因此在list中进行插入时是不会导致list的迭代器失效的,只有在删除时才会失效,并且失效的只是指向被删除节点的迭代器,其他迭代器不会受到影响。
cpp
void TestList() {
int arr[] = { 1,2,3,4,5,6,7,8,9 };
list<int>l1 (arr, arr + sizeof(arr) / sizeof(arr[0]));
auto it = l1.begin();
while (it != l1.end()) {
l1.erase(it);
it++;
}
}
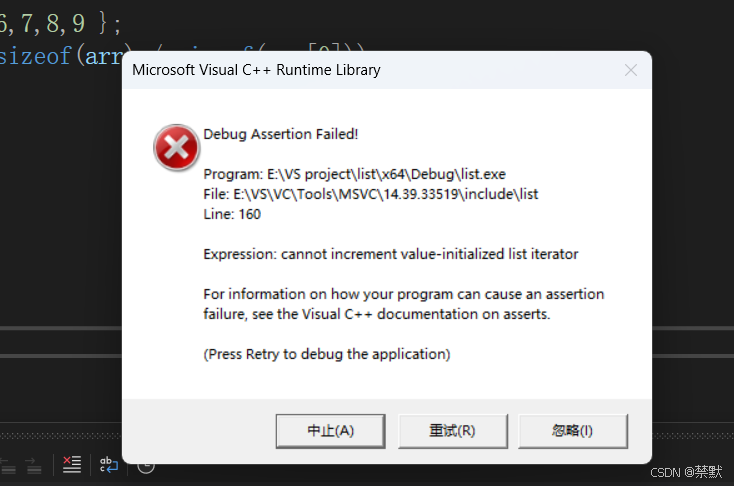
修改后:
cpp
void TestList() {
int arr[] = { 1,2,3,4,5,6,7,8,9 };
list<int>l1 (arr, arr + sizeof(arr) / sizeof(arr[0]));
print(l1);
auto it = l1.begin();
while (it != l1.end()) {
//等价于l1.erase(it++);
it = l1.erase(it);
}
print(l1);
}
变式删除偶数:
cpp
void TestList1() {
int arr[] = { 1,2,3,4,5,6,7,8,9 };
list<int>l1(arr, arr + sizeof(arr) / sizeof(arr[0]));
print(l1);
auto it = l1.begin();
while (it != l1.end()) {
if(*it%2==0)
it = l1.erase(it);
else
it++;
}
print(l1);
}

附整套练习源码
cpp
#define _CRT_SECURE_NO_WARNINGS
#include <iostream>
#include <list>
#include <vector>
using namespace std;
void test1() {
list<int>l1;
list<int>l2(5, 10);
list<int>l3(l2.begin(), l2.end());//迭代器构造
list<int>l4(l2);//拷贝构造
//以数组区间迭代器构造list
float arr[] = { 5.20,13.14,9.99,8.88 };
list<float>l5(arr, arr + sizeof(arr) / sizeof(float));
// 列表格式初始化C++11
list<int> l6{ 1,2,3,4,5 };
// 用迭代器方式打印l5中的元素
list<float> ::iterator it = l5.begin();
while (it != l5.end()) {
cout << *it << " ";
it++;
}
cout << endl;
// C++11范围for的方式遍历
for (auto e : l6) {
cout << e << " ";
}
cout << endl;
cout << l5.size() << endl;
}
void TestList2()
{
int array[] = { 1, 2, 3, 4, 5, 6, 7, 8, 9, 0 };
list<int> l(array, array + sizeof(array) / sizeof(array[0]));
// 使用正向迭代器正向list中的元素
// list<int>::iterator it = l.begin(); // C++98中语法
auto it = l.begin(); // C++11之后推荐写法
while (it != l.end())
{
cout << *it << " ";
++it;
}
cout << endl;
// 使用反向迭代器逆向打印list中的元素
// list<int>::reverse_iterator rit = l.rbegin();
auto rit = l.rbegin();
while (rit != l.rend())
{
cout << *rit << " ";
++rit;
}
cout << endl;
}
void test3() {
list<int> mylist;
mylist.push_back(10);
while (mylist.back() != 0)
{
mylist.push_back(mylist.back() - 1);
}
cout << "mylist contains:";
for (list<int>::iterator it = mylist.begin(); it != mylist.end(); ++it)
std::cout << ' ' << *it;
cout << '\n';
}
template <class Container>
void print(const Container& v) {
auto it = v.begin();
while (it != v.end()) {
cout << *it << " ";
it++;
}
cout << endl;
}
void print_list(const list<int>& ml) {
// 注意这里调用的是list的 begin() const,返回list的const_iterator对象
list<int>::const_iterator it = ml.begin();
while (it != ml.end()) {
cout << *it << " ";
it++;
}
cout << endl;
}
void TestList3() {
list<int>mylist{ 1,2,3,4,5 };
mylist.push_back(6);
mylist.push_front(0);
print_list(mylist);
mylist.pop_back();
mylist.pop_front();
print_list(mylist);
}
void TestList4()
{
int array1[] = { 1, 2, 3 };
list<int> L(array1, array1 + sizeof(array1) / sizeof(array1[0]));
// 获取链表中第二个节点
//auto pos = ++L.begin();
list<int>::iterator pos = ++L.begin();
cout << *pos << endl;
// 在pos前插入值为4的元素
L.insert(pos, 4);
print_list(L);
// 在pos前插入5个值为5的元素
L.insert(pos, 5, 5);
print_list(L);
// 在pos前插入[v.begin(), v.end)区间中的元素
vector<int> v{ 7, 8, 9 };
L.insert(pos, v.begin(), v.end());
print_list(L);
// 删除pos位置上的元素
L.erase(pos);
print_list(L);
// 删除list中[begin, end)区间中的元素,即删除list中的所有元素
L.erase(L.begin(), L.end());
print_list(L);
}
void TestList5()
{
// 用数组来构造list
int array1[] = { 1, 2, 3 ,4 ,5};
list<int> l1(array1, array1 + sizeof(array1) / sizeof(array1[0]));
//print_list(l1);
print(l1);
list<int>l2{ 6,7,8,9,10 };
// 交换l1和l2中的元素
l1.swap(l2);
//print_list(l1);
//print_list(l2);
print(l1);
print(l2);
// 将l2中的元素清空
l2.clear();
cout << l2.size() << endl;
}
void TestList() {
int arr[] = { 1,2,3,4,5,6,7,8,9 };
list<int>l1 (arr, arr + sizeof(arr) / sizeof(arr[0]));
print(l1);
auto it = l1.begin();
while (it != l1.end()) {
//等价于l1.erase(it++);
it = l1.erase(it);
}
print(l1);
}
void TestList1() {
int arr[] = { 1,2,3,4,5,6,7,8,9 };
list<int>l1(arr, arr + sizeof(arr) / sizeof(arr[0]));
print(l1);
auto it = l1.begin();
while (it != l1.end()) {
if(*it%2==0)
it = l1.erase(it);
else
it++;
}
print(l1);
}
int main() {
//test3();
TestList1();
return 0;
}
结束语
本节内容就到此结束啦,相信大家对list有了进一步的了解,下节我们将一步一步实现自己的list!
最后感谢各位友友的支持,给小编点个赞吧!!!